The `std::to_string` function in C++ is used to convert various data types, such as integers and floating-point numbers, into their corresponding string representations.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
int num = 42;
std::string strNum = std::to_string(num);
std::cout << "String representation: " << strNum << std::endl;
return 0;
}
Understanding Data Types in C++
In C++, the language supports various basic data types, but two of the most commonly used are integers and strings. Integers represent whole numbers, while strings represent sequences of characters.
Data type conversion, often referred to as type casting, is a critical concept in C++. It allows developers to transform one data type into another when necessary. Understanding how to convert integers to strings is particularly important for displaying, processing, and storing data effectively.
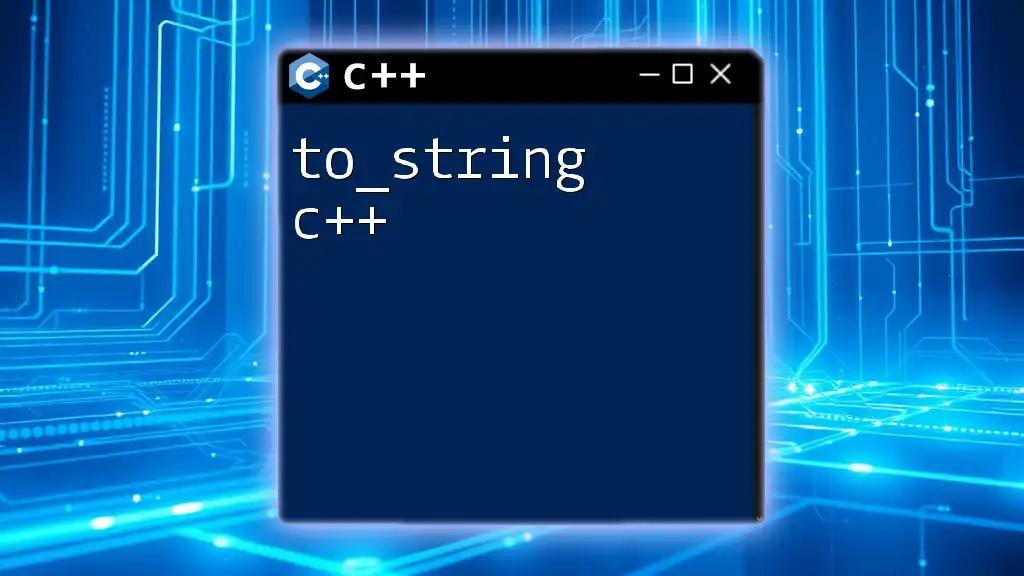
Why Convert int to string in C++
There are several key reasons to convert an integer to a string in C++.
-
Performance Considerations: Working with strings can sometimes lead to improved readability and easier debugging during the development process.
-
Data Presentation: In many applications, especially user interfaces, displaying integers as strings can make the output more user-friendly.
-
File I/O Operations: When writing integer data to files or transferring it over networks, strings are often required.
Consider a situation where you want to log numeric values alongside descriptive text. For example, if you’d like to log error messages that include specific integer error codes, converting these integers to strings becomes essential.

Methods to Convert int to string in C++
Using `std::to_string`
One of the simplest and most straightforward methods to convert an integer to a string in C++ is to use the `std::to_string()` function.
Overview of `std::to_string`:
- Simple Syntax: This function takes a numeric argument (int, float, etc.) and returns its string representation.
- Easy to Use: Minimizes the amount of boilerplate code you need to write.
Code Example:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string str = std::to_string(number);
std::cout << "The string representation is: " << str << std::endl;
return 0;
}
Explanation: In the example above, we convert the integer `42` into a string using `std::to_string()`. This method is efficient and reduces the likelihood of errors that could occur if you were to manage the conversion manually.
Using `std::stringstream`
Another versatile option for converting integers to strings is to utilize `std::stringstream`.
Overview of `std::stringstream`:
- Flexible Approach: Offers more control over formatting and is part of the C++ Standard Library.
- Chaining of Streams: Allows you to combine multiple types into a single string.
Step-by-Step Guide:
- Include the necessary headers.
- Create a `stringstream` object.
- Stream the integer into the `stringstream`.
- Retrieve the string from the `stringstream`.
Code Example:
#include <iostream>
#include <sstream>
int main() {
int number = 42;
std::stringstream ss;
ss << number; // Stream the integer into the stream
std::string str = ss.str(); // Retrieve the string
std::cout << "The string representation is: " << str << std::endl;
return 0;
}
In-depth Explanation: This method creates a `stringstream` object `ss`, into which the integer `number` is streamed. The `str()` function then produces the final string representation. This approach is very powerful, as you can easily concatenate multiple items in the stream and manage diverse data types seamlessly.
Using `sprintf` in C++
The `sprintf` function offers another way to convert integers to strings. While more traditional, it still finds relevance in many C++ applications.
Overview of `sprintf`:
- C-style Formatting: Allows for formatted output and is widely recognized among C and C++ programmers.
- Considerations for Buffer Sizes: Ensure buffers are adequately sized to avoid overflow.
Code Example:
#include <iostream>
#include <cstdio>
int main() {
int number = 42;
char buffer[50]; // Allocate buffer for the string representation
sprintf(buffer, "%d", number); // Format the integer as a string
std::string str(buffer); // Create a string from the buffer
std::cout << "The string representation is: " << str << std::endl;
return 0;
}
Discussion: While `sprintf` provides robust formatting options, it requires careful handling of buffer sizes. An insufficient buffer can lead to buffer overflow vulnerabilities. Due to these concerns, more modern methods like `std::to_string` and `std::stringstream` are often preferred.
Using `std::ostringstream`
Using `std::ostringstream` is similar to `std::stringstream`, but it’s explicitly designed for output operations.
Brief Overview:
- Object-Oriented Approach: Emphasizes C++ styling and is part of the Standard Library.
- Easy Integration: Naturally integrates with other C++ classes.
Code Example:
#include <iostream>
#include <sstream>
int main() {
int number = 42;
std::ostringstream oss;
oss << number; // Stream the integer into the ostringstream
std::string str = oss.str(); // Retrieve the string
std::cout << "The string representation is: " << str << std::endl;
return 0;
}
Clarification: Similar to the previous example using `std::stringstream`, `std::ostringstream` is dedicated to output and is well-suited for scenarios where you primarily want to transform various output types into strings.
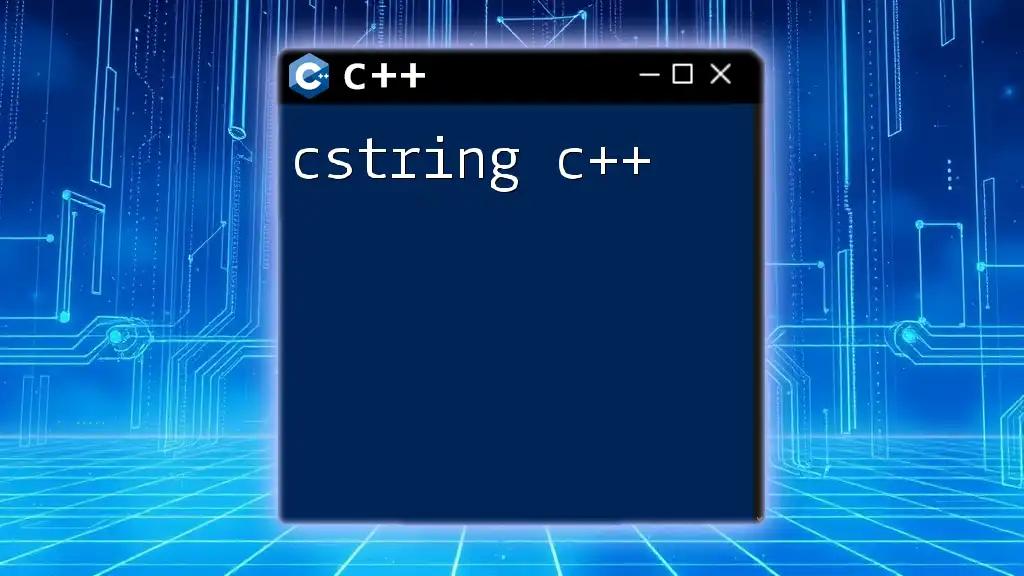
Best Practices for int to string Conversion
-
Avoiding Unnecessary Conversions: It's essential to be mindful of performance. Frequent conversions between types can lead to inefficiencies, so it's often best to perform conversions only when necessary.
-
Error Handling in Conversions: Always consider potential exceptions that might arise during conversion. Implement necessary checks or use try-catch blocks to handle unexpected cases gracefully.
-
Choosing the Right Method for the Task: Take into account the specific requirements of your application, such as performance, readability, and safety, before selecting a conversion method.

Conclusion
In summary, converting integers to strings in C++ offers flexibility and numerous options. Whether you choose to use `std::to_string`, `std::stringstream`, `sprintf`, or `std::ostringstream`, each method has its strengths and weaknesses. By understanding these various approaches and their respective use cases, you can effectively integrate int-to-string conversions into your coding workflows, enhancing the readability and functionality of your applications. Experiment with these methods, and see how they can improve your programming experience in C++.
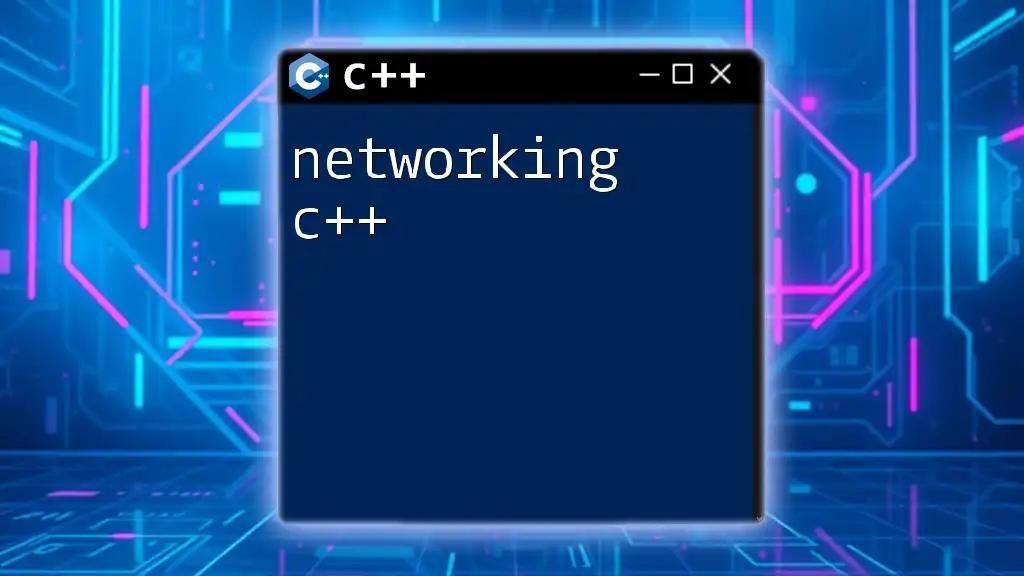
Additional Resources
For deeper learning and mastery over type conversions in C++, consider exploring recommended books and online courses on C++. Additionally, familiarize yourself with relevant official C++ documentation that can enhance your understanding of the intricacies of the C++ language.