Casting in C++ is the process of converting a variable from one data type to another, which can be achieved using static_cast, dynamic_cast, reinterpret_cast, or const_cast, depending on the use case.
#include <iostream>
int main() {
double num = 5.7;
int intNum = static_cast<int>(num); // Casting double to int
std::cout << "The integer value is: " << intNum << std::endl; // Outputs: The integer value is: 5
return 0;
}
What is Casting in C++?
Casting C++ refers to the process of converting a variable from one data type to another, allowing for greater flexibility in how data is manipulated and utilized in your code. This is a fundamental concept in C++ programming that enhances type safety and ensures that operations are applicable to the intended types. Casting is essential when dealing with different data types, especially in scenarios involving function parameters, class inheritance, and polymorphism.
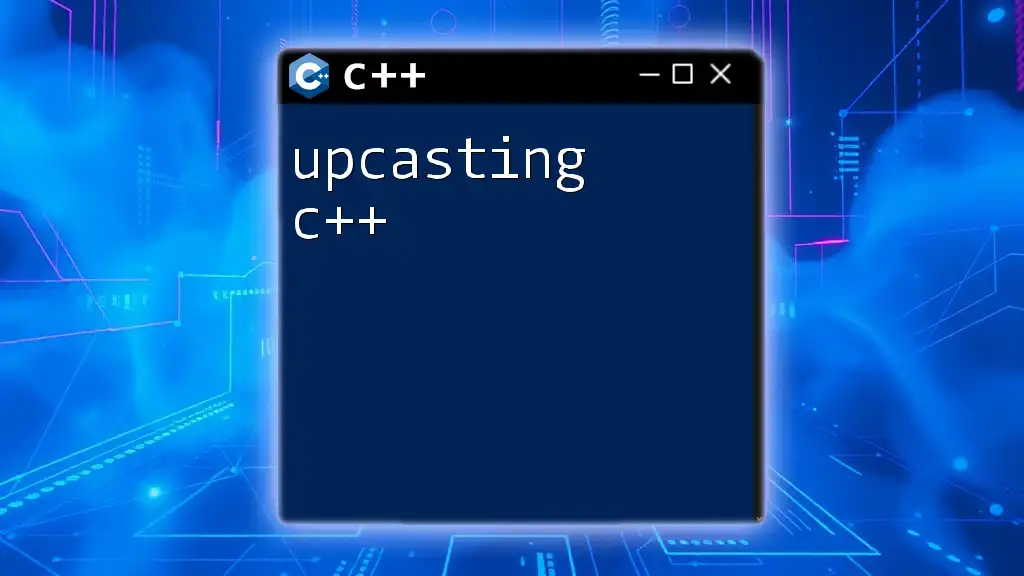
Why is Casting Necessary?
The necessity of casting arises from several key reasons:
-
Type Safety: C++ is a statically typed language which means type-checking is done at compile time. Casting ensures that the types of variables match the operations being performed, reducing the risk of type-related errors.
-
Data Manipulation: In many instances, variables need to be converted from one type to another for operations to be valid. For example, converting a `float` to an `int` when a whole number is required.
-
Performance Considerations: Proper casting can lead to optimized memory usage and performance by ensuring that the right data types are used according to the context of the program.
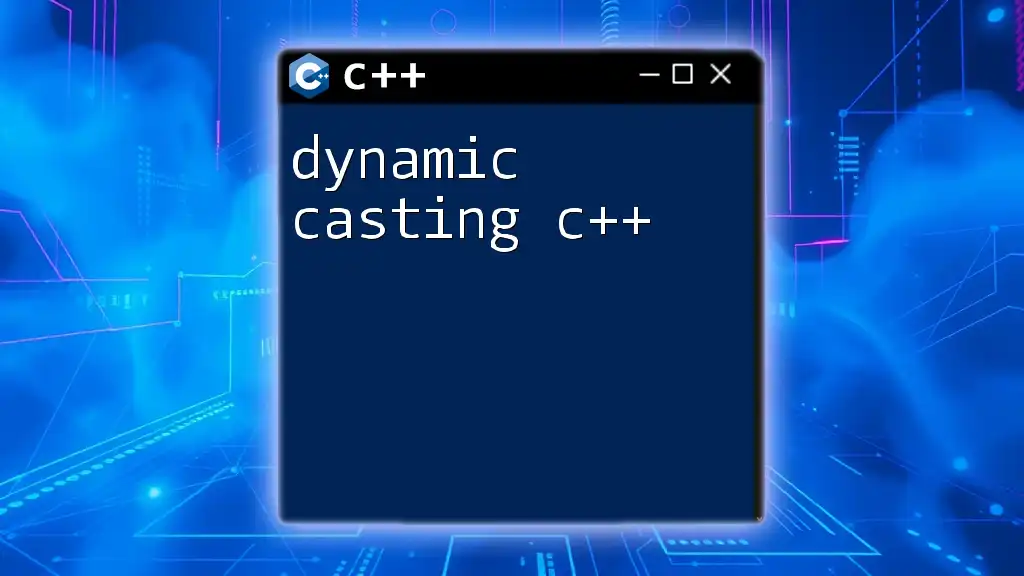
Types of Casting in C++
Implicit Casting
Implicit casting, also known as automatic or coercive casting, is performed by the compiler and does not require any additional syntax from the programmer. This occurs in situations where conversion is safe and clear:
For example, when assigning an `int` to a `float`:
int intValue = 10;
float floatValue = intValue; // Implicit casting from int to float
In this case, the integer is automatically converted to a floating-point number without losing information.
Explicit Casting
Explicit casting occurs when you manually specify the conversion. C++ offers several methods of explicit casting:
C-style Casting
Using the C-style casting involves using parentheses and the desired type:
double dValue = 9.8;
int intValue = (int)dValue; // C-style casting
While C-style casting is concise, it can be less clear and potentially dangerous as it allows for multiple types of casts.
Static Cast
The `static_cast` operator is a safer and more modern way to perform explicit casting. It can be used for conversions between related types, such as numeric types and base/derived class types:
float floatValue = 10.5;
int intValue = static_cast<int>(floatValue); // Convert float to int safely
With `static_cast`, if the conversion is not valid or dangerous, the compiler will throw an error, increasing type safety.
Dynamic Cast
The `dynamic_cast` is used extensively in scenarios involving polymorphism, specifically when working with class hierarchies. This cast checks at runtime whether the conversion is valid:
class Base {
virtual void func() {}
};
class Derived : public Base {
void func() override {}
};
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Safe downcasting
If the cast fails, `derivedPtr` will be `nullptr`, allowing for safe navigation through the class hierarchy.
Const Cast
The `const_cast` operator is used when you need to add or remove the `const` qualifier from a variable. This can be useful when interacting with APIs that require a non-const pointer:
const int constValue = 20;
int* nonConstValue = const_cast<int*>(&constValue); // Remove const qualifier
Care must be taken when using `const_cast` as modifying a const object can lead to undefined behavior.
Reinterpret Cast
The `reinterpret_cast` is used for low-level casting, allowing for conversion between incompatible types. This is powerful but can lead to dangerous code if not handled carefully:
int intValue = 65;
char* charPtr = reinterpret_cast<char*>(&intValue); // Reinterpret int as char pointer
std::cout << *charPtr; // Undefined behavior, may not output expected result
This cast does not perform any type checking, meaning that the programmer has to be cautious to avoid unsafe memory accesses.
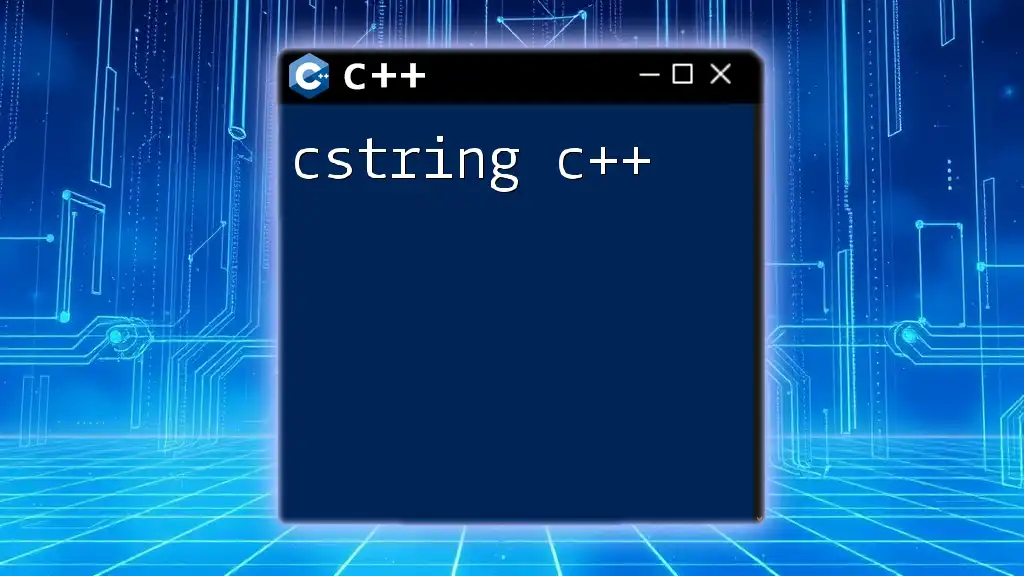
Best Practices for Casting
When to Use Casting
Casts should generally be discouraged unless absolutely necessary. When using casting, ensure:
- The conversion is logical and necessary for the operation
- The types are compatible and do not lead to information loss
- Alternatives (like templates) do not exist that accomplish the same goal without casting
Performance Considerations
Different types of casting can impact performance, especially when using `reinterpret_cast` or excessive dynamic casts. Favor `static_cast` and implicit casts for efficiency whenever possible, and remain mindful of using only what is needed in order to maintain flow and clarity in your code.
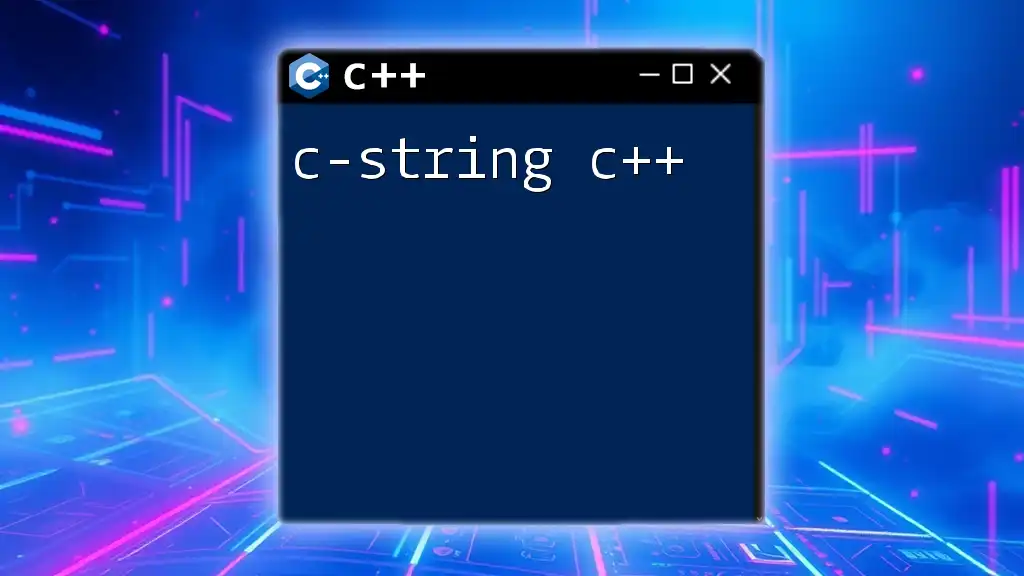
Common Mistakes in Casting
Overusing Casting
One significant risk in C++ programming is the overuse of casting. Relying heavily on casting can indicate that the code design needs reevaluation. If you find yourself frequently needing to cast, consider redesigning your classes or functions to work with better type compatibility inherently.
Failure to Check Types
Another frequent error is neglecting to check the validity of types prior to casting. For example, using `dynamic_cast` without checking for `nullptr` can cause runtime errors:
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (!derivedPtr) {
std::cerr << "Invalid cast!" << std::endl;
}
It is important always to check and ensure that the types are compatible before proceeding with the cast.
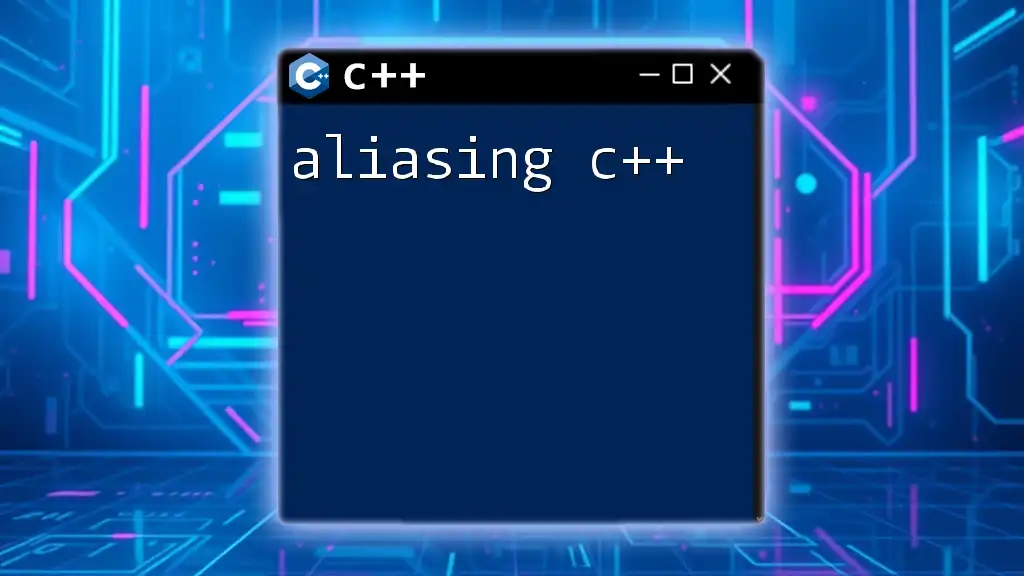
Conclusion
In summary, casting C++ is a crucial aspect that every C++ programmer must understand. Ranging from simple implicit conversions to safe explicit casts, each type has its usage and rules that govern its application. By adhering to best practices and avoiding common mistakes, developers can leverage casting effectively while maintaining code quality and prevent bugs related to type mismatches.
Final Thoughts
As you progress in your C++ journey, take the time to practice casting and explore how it fits within your broader programming paradigm. As you grow more comfortable with casting, you may find advanced topics, such as template programming and type traits, to further enhance your coding toolbox.
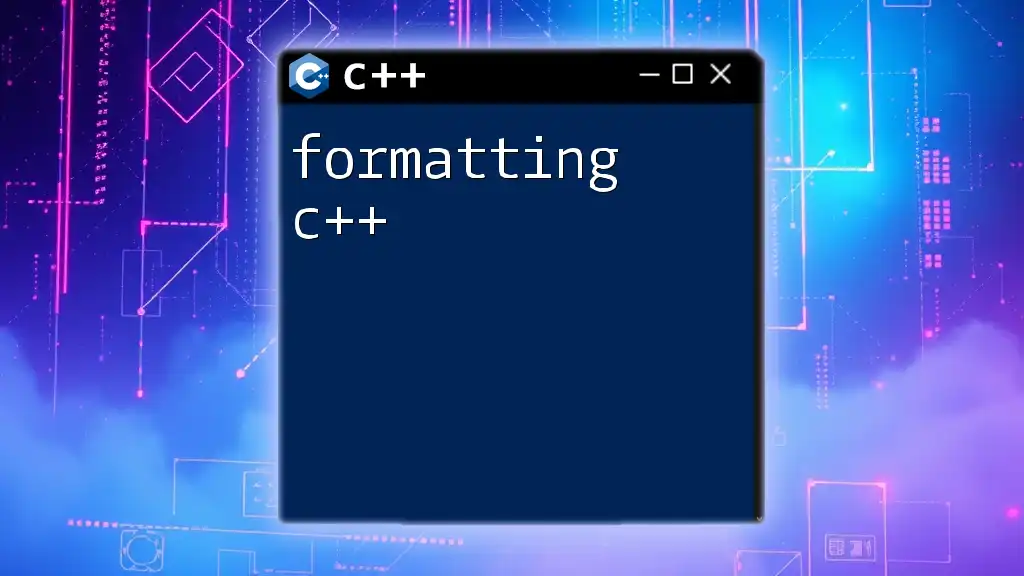
Additional Resources
For those looking to deepen their understanding, numerous resources are available. Recommended readings include books focused on C++ programming, online tutorials, and official C++ documentation. Engaging with the community through forums can also provide insights and real-world examples, enriching your learning experience.
Call to Action
Stay connected for more insights, tips, and comprehensive guides centered around mastering C++ casting and other fundamentals. Don’t hesitate to share your experiences or questions on the topic!