In C++, the `std::to_string` function converts various numerical types into their string representations for easier handling and display.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The number is: " << strNumber << std::endl;
return 0;
}
Understanding the to_string Function in C++
What is to_string in C++?
The `to_string` function in C++ is a built-in utility that facilitates the conversion of numerical values into a `std::string`. This is crucial because C++ recognizes various data types, and converting non-string types to string is often essential for display, logging, or concatenating with other strings.
The importance of `to_string` lies in its ability to simplify type conversions, reducing boilerplate code and potential bugs associated with manual conversions. Its typical use cases include formatting output for display purposes and preparing data for storage or transmission.
How to Use to_string in C++
Syntax Overview
The syntax of the `to_string` function is straightforward. It accepts numeric types as parameters and returns their string representation.
Data Types Supported by to_string Function
The `to_string` function supports a variety of data types, including:
- Integer types: `int`, `long`, `long long`
- Floating-point types: `float`, `double`, `long double`
Example Usage with Simple Data Types
Here's a basic example demonstrating how to convert an `int` to a string:
int num = 42;
std::string str_num = std::to_string(num);
In this example, the integer value `42` is converted into a string representation, `"42"`.
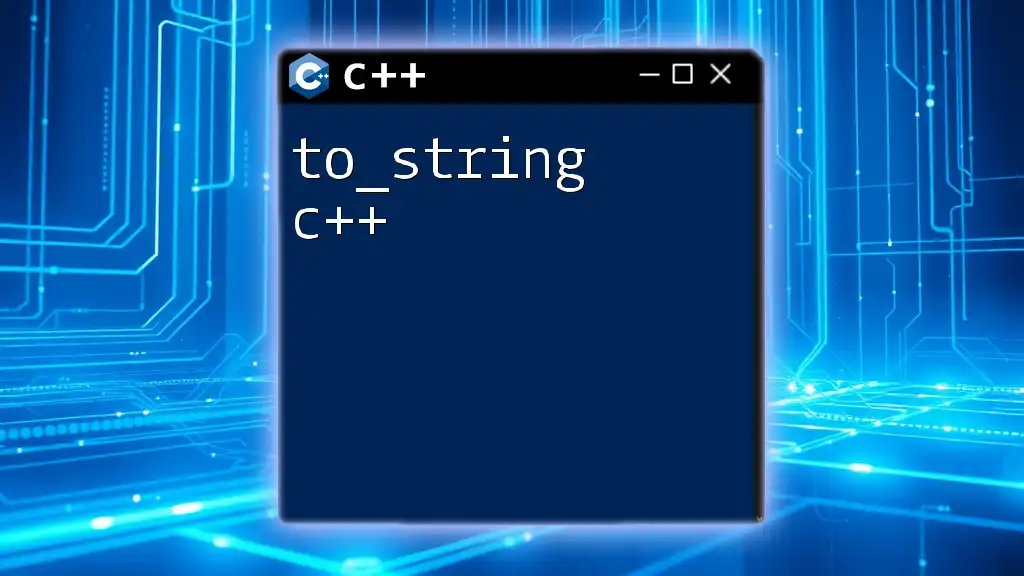
Detailed Breakdown of to_string Parameters
Parameter Types Supported
Integer Types
You can convert integers seamlessly:
int myInt = 100;
std::string myString = std::to_string(myInt);
Floating-Point Types
Similarly, the `to_string` function effectively handles floating-point numbers:
float myFloat = 3.14f;
std::string floatString = std::to_string(myFloat);
Return Value
The `to_string` function returns a `std::string`, providing the string representation of the input number. However, developers should be aware of potential downsides. For instance, precision issues can arise when converting floating-point numbers.
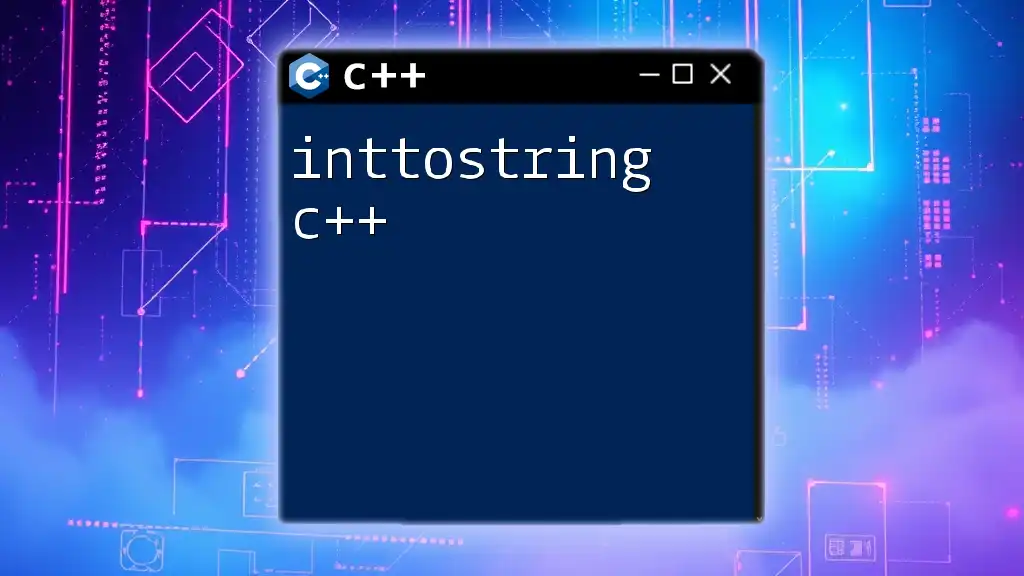
Practical Examples of to_string in C++
Converting Different Data Types
Converting an int to a string
int myInt = 150;
std::string myIntString = std::to_string(myInt);
Converting a double
double myDouble = 99.9999;
std::string myDoubleString = std::to_string(myDouble);
Combining with Other String Functions
The `to_string` function can be combined with other string manipulation methods to enhance its utility.
Example: Using to_string with string concatenation
Using `to_string` in a concatenation adds clarity to logged messages:
int score = 95;
std::string message = "Your score is " + std::to_string(score);
Example: Using to_string with std::cout
Another common scenario is directly using `to_string` with `std::cout` for display:
double pi = 3.14159;
std::cout << "The value of pi is " << std::to_string(pi) << std::endl;
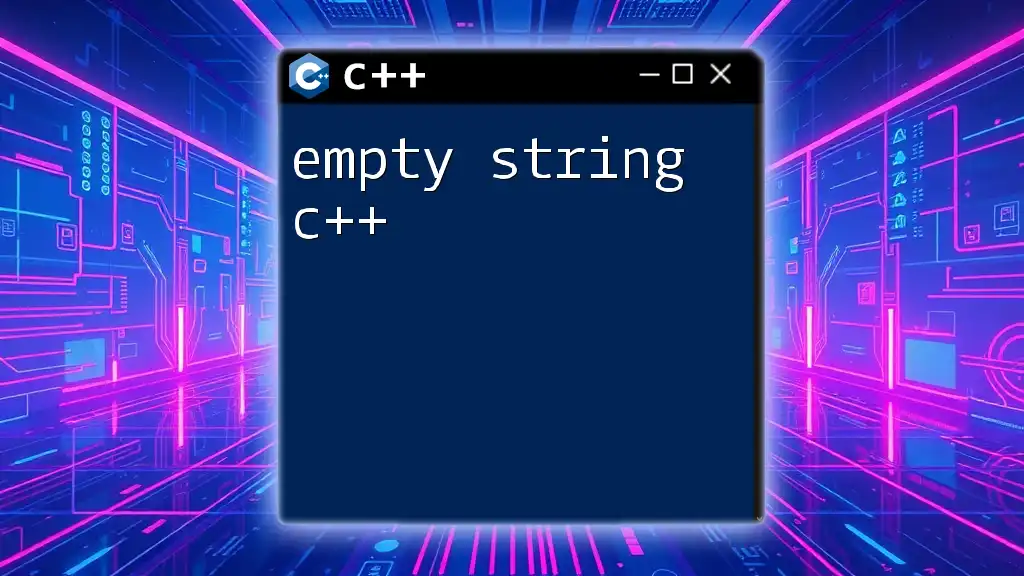
Error Handling and Limitations
Handling Edge Cases
While `to_string` is helpful, it may encounter edge cases. For instance, when converting large integers, it can lead to unexpected behavior. Similarly, floating-point conversions might lose precision if the number exceeds a specific threshold.
Common Errors when Using to_string
New developers might face type mismatches, where passing an unsupported type to `to_string` results in compilation errors. Additionally, during runtime, improper handling of numeric types could lead to crashes or undefined behavior. Always validate data types before invoking `to_string`.
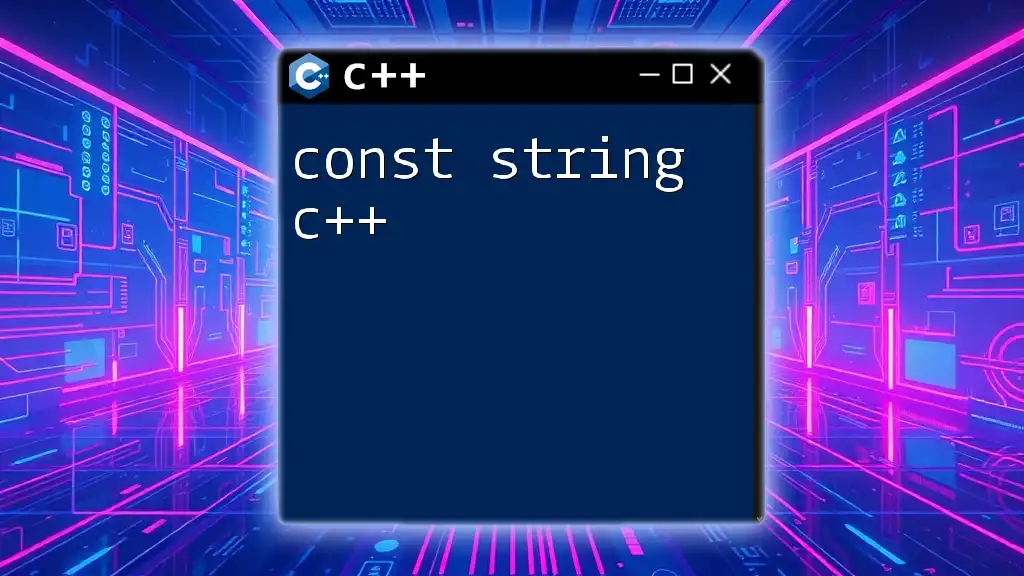
Best Practices when Using to_string in C++
When to Use to_string
`to_string` is ideally employed when formatting output to be human-readable or when preparing strings for UI components. Limiting its use to instances where clarity improves code readability can help maintain good coding practices.
Alternatives to to_string
For more complex string conversions or when handling various data types, `stringstream` may be a viable alternative. Using stringstreams provides greater control over the formatting and representation of numbers. Here’s how to do it:
#include <sstream>
double myDouble = 5.678;
std::ostringstream os;
os << myDouble;
std::string doubleStr = os.str();
For those utilizing external libraries, the Boost library offers robust features for string conversions and manipulations.
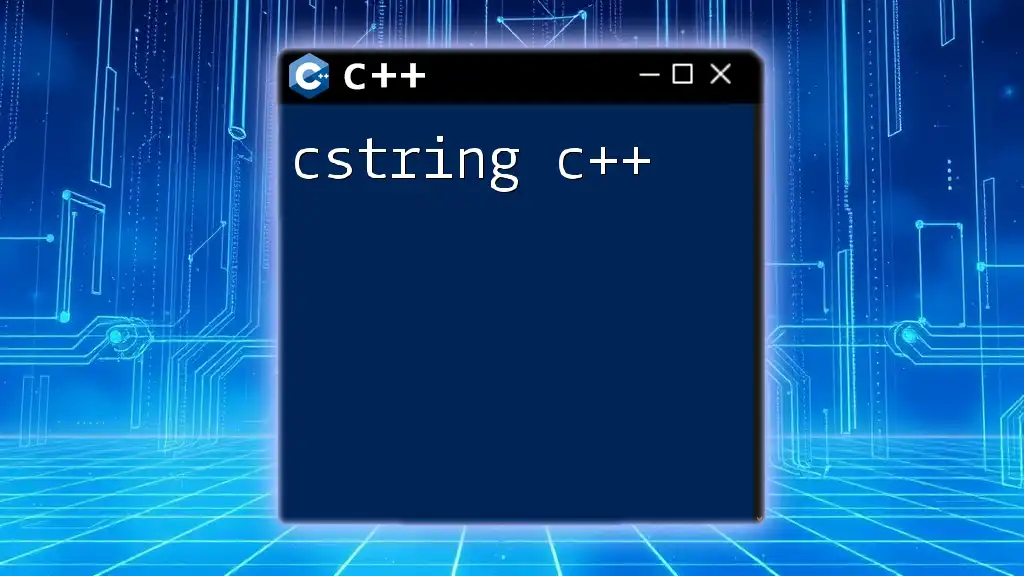
Conclusion
Recap of Key Points
In summary, the `to_string` function in C++ serves as an essential tool for converting numerical types into string representations. Its simplicity and efficiency make it an invaluable asset in everyday programming tasks. Understanding the nuances of `to_string`, including parameter support and potential pitfalls, is vital for effective C++ programming.
Additional Resources for Learning
For increased proficiency in C++, including using `to_string`, consider checking out official documentation, reputable programming books, or enrolling in online courses focusing on C++. Exploring more on string manipulation techniques will deepen your understanding and enhance your skill set.