In C++, the `std::to_string` function is used to convert numerical values into string format for easier manipulation and display.
Here’s an example code snippet:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The string representation is: " << strNumber << std::endl;
return 0;
}
What is `to_string`?
The `to_string` function in C++ is a powerful tool that allows developers to convert various data types into a string representation. This function simplifies the process of turning integers, floating-point numbers, and other numeric types into strings, which can be particularly useful in user interfaces, data storage, and when performing string concatenations.

Understanding Data Types in C++
C++ supports a variety of fundamental data types, including:
- Integers (e.g., `int`, `long`)
- Floating-point numbers (e.g., `float`, `double`)
- Characters and Booleans
Converting these data types into strings is crucial when you need to present or manipulate data as text. The `to_string` function streamlines these conversions, helping to create clear and efficient code.
Why Use `to_string`?
Purpose of Conversion
Data conversion becomes essential in scenarios such as:
- Display: When you want to present numeric data as text in user interfaces.
- Data Storage: When saving numeric values as part of formatted strings.
- String Operations: When concatenating numbers with other strings.
Advantages of Using `to_string`
Using `to_string` has several advantages:
- Simplicity: The function abstracts the complexities of data conversion, allowing you to write code that is easier to read and maintain.
- Error Reduction: It reduces the likelihood of bugs that can arise from manual conversion methods.
- Consistency: It ensures a uniform way of converting different data types into strings.
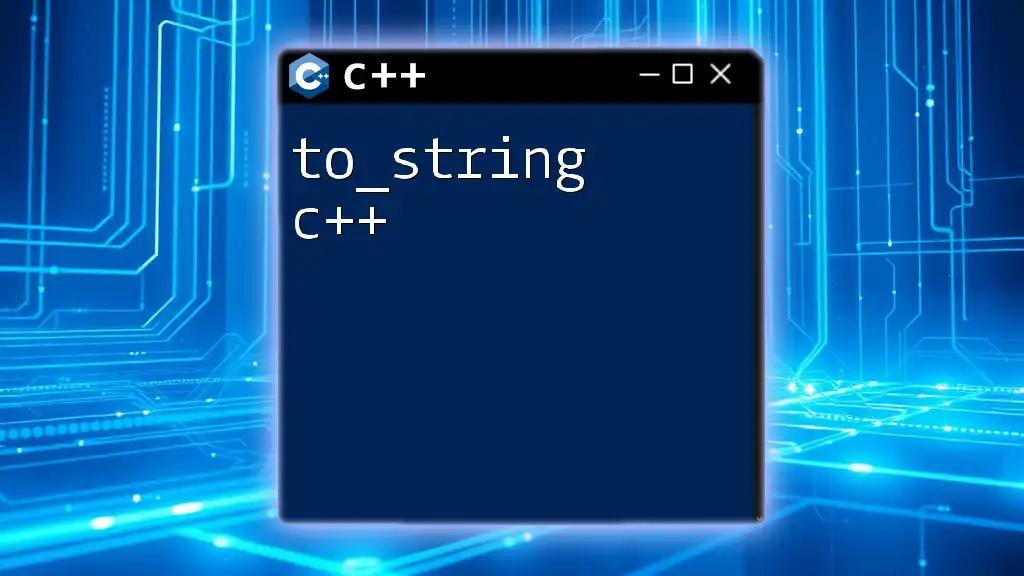
The Syntax of `to_string`
The syntax for using `to_string` is straightforward:
std::string to_string(T value);
Available Variants
The function supports various data types. Here are some common examples:
- `int`: Converts an integer to a string.
- `float`: Converts a floating-point number to a string.
- `double`: Converts a double-precision floating-point number to a string.
This functionality makes `to_string` versatile and applicable in multiple scenarios of coding.

Examples of Using `to_string`
Converting an Integer to String
Converting an integer to a string is a common task. Here’s a simple example:
int num = 42;
std::string strNum = std::to_string(num);
In this case, `strNum` will contain the string "42", effectively converting the integer using `to_string`.
Converting a Floating Point Number to String
For floating-point numbers, the process is equally simple:
float pi = 3.14;
std::string strPi = std::to_string(pi);
As a result, `strPi` will hold the string "3.140000". This conversion provides a full representation including trailing zeros.
Converting a Double to String
For double values, the syntax remains the same:
double e = 2.71828;
std::string strE = std::to_string(e);
This would yield `strE` as "2.718280". It's essential to note how the precision is managed automatically by `to_string`.

Handling Special Cases
Precision Control in Floating Point Numbers
When you need specific control over how many decimal places appear in your string representation, you can use `std::setprecision`. Here’s an example:
#include <iomanip> // For std::setprecision
float pi = 3.14159;
std::ostringstream out;
out << std::fixed << std::setprecision(2) << pi;
std::string strPi = out.str();
Now `strPi` will be "3.14", allowing you to specify the exact level of precision you desire.
Converting Negative Numbers and Edge Cases
Converting negative numbers is equally straightforward:
int negativeNum = -10;
std::string strNegativeNum = std::to_string(negativeNum);
The value of `strNegativeNum` will be "-10". This capability ensures that `to_string` can handle both positive and negative values seamlessly.
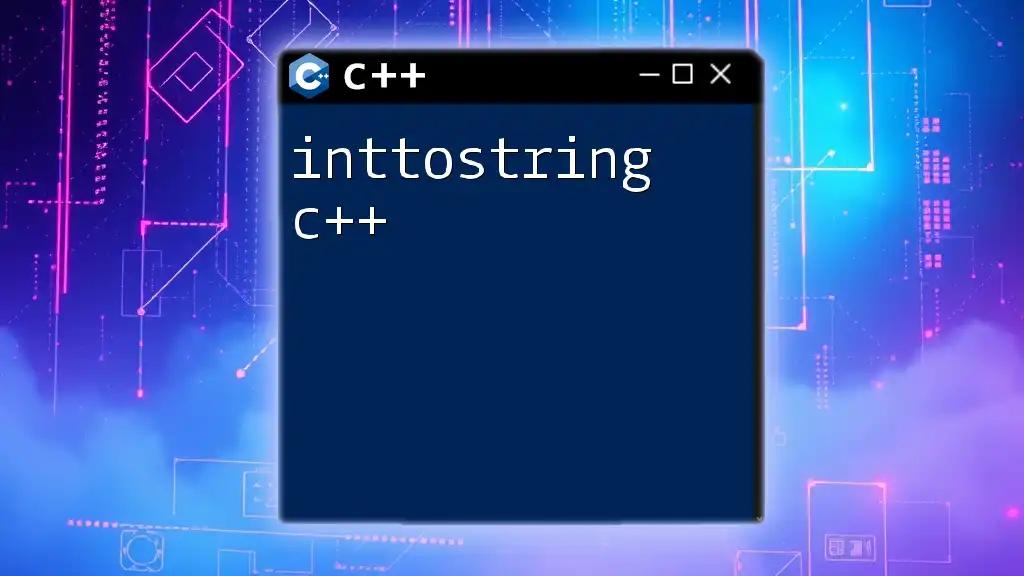
Errors and Limitations
Potential Pitfalls
While `to_string` streamlines many conversions, it’s essential to be aware of its limitations. If you attempt to use `to_string` with unsupported types, it will produce compile-time errors. Thus, it’s crucial to ensure the type being passed is one of the supported basic types (e.g., int, float, double).
Return Values
Note that `to_string` will return an empty string if an unsupported type is processed, leading to potential confusion in your code. Always ensure the types are compatible.

When Not to Use `to_string`
Performance Considerations
In highly performance-sensitive applications, especially in scenarios that involve numerous conversions, `to_string` may not be the optimal solution due to potential overhead. It's a good practice to profile your code and ascertain performance using benchmarks.
Alternative Methods
For cases where performance is paramount, consider using other libraries or functions, such as `stringstream`. This method may provide greater control and efficiency when crafting strings:
#include <sstream>
int number = 100;
std::stringstream ss;
ss << number;
std::string result = ss.str();
This approach can be particularly useful when building complex strings or when concatenating multiple values.
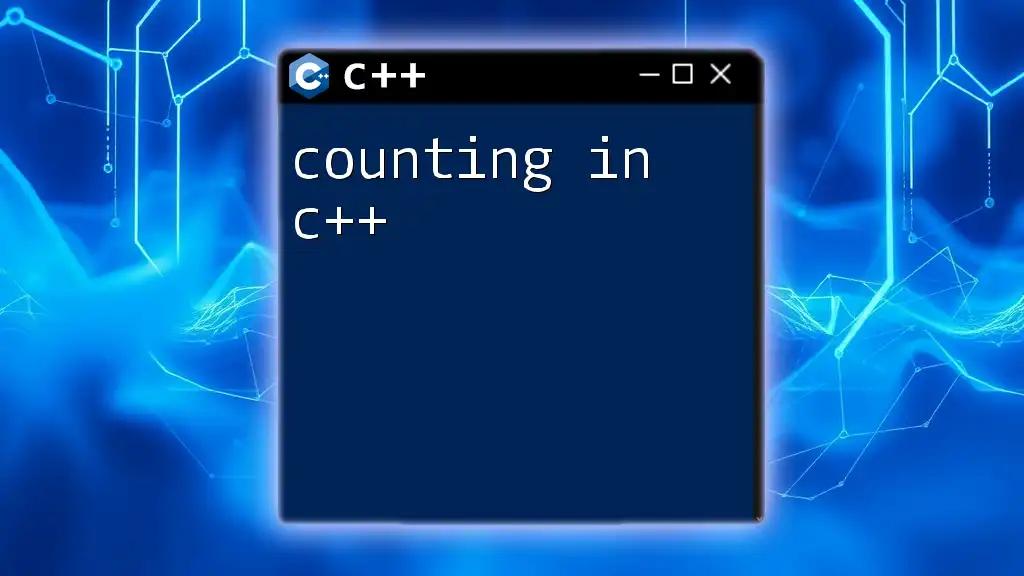
Best Practices for Using `to_string`
Keep It Simple
Maintain a focus on simplicity and readability in your code. Using `to_string` promotes clear, self-documenting code that can easily be understood by other developers.
Use Cases to Consider
When deciding to use `to_string`, consider the following typical scenarios:
- Building strings for logging messages
- Preparing data for user input or display
- Concatenating numeric values with strings in complex outputs

Conclusion
The `to_string` function in C++ is an invaluable tool for developers, simplifying the process of converting numeric types to string representations. Understanding its usage, advantages, and best practices empowers you to improve code readability and reduce errors. By practicing and experimenting with `to_string`, you can integrate this function into your coding toolkit for efficient data handling and representation.
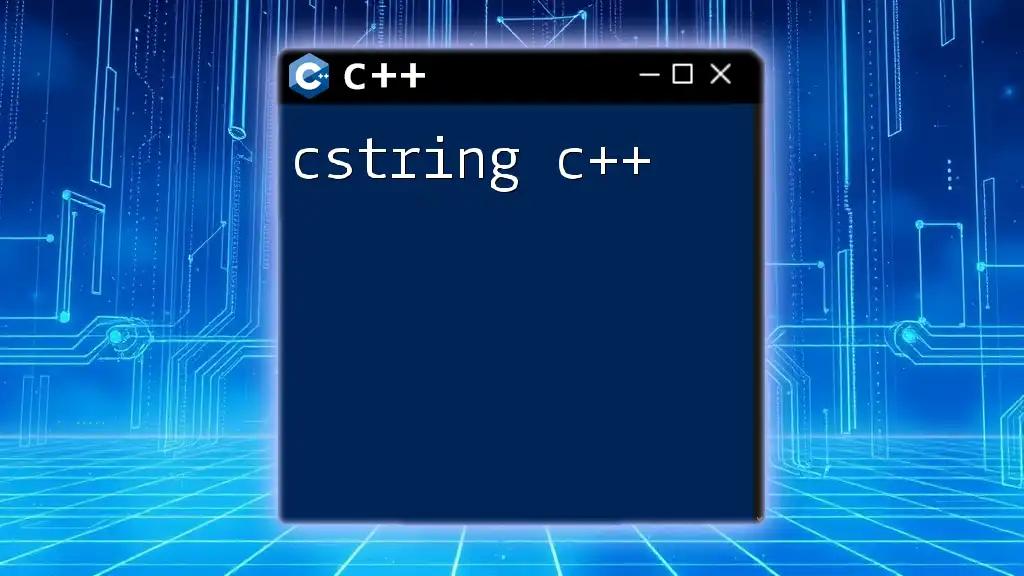
Additional Resources
For further learning, refer to the official C++ STL documentation. Online courses or textbooks on C++ will provide deeper insights into string manipulation and broader programming principles.