To reverse a string in C++, you can use the `std::reverse` function from the `<algorithm>` header. Here’s a simple code snippet demonstrating this:
#include <iostream>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end());
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
What Does It Mean to Reverse a String?
In C++, a string is essentially a sequence of characters represented as an object of the `std::string` class. When we talk about reversing a string, we refer to the process of rearranging the characters in the reverse order. For instance, the string "hello" would become "olleh".
Reversing strings is significant in programming because it finds applications in various algorithms, data processing tasks, and problem-solving challenges. Knowing how to reverse a string efficiently enables developers to handle scenarios like palindrome checking, cryptography, and data transformations seamlessly.

Basic Methods to Reverse a String in C++
Using Loops
One of the simplest methods to reverse a string is by using a loop. This approach is straightforward and gives you a clear understanding of how string indexing works.
Code Snippet: Basic Loop Method
#include <iostream>
#include <string>
using namespace std;
string reverseString(string str) {
string reversed = "";
for (int i = str.length() - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
In this snippet, we define a function `reverseString` that takes a string as input. The loop iterates through the string from the last character to the first, appending each character to `reversed`. The final output is a new string with characters in reverse order.
Using `std::reverse` Function
The C++ Standard Library includes a powerful utility for reversing collections, including strings, via the `<algorithm>` header. This method is efficient and clean.
Code Snippet: Using std::reverse
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
string reverseString(string str) {
reverse(str.begin(), str.end());
return str;
}
Here, the `reverse` function automatically swaps elements in place between the beginning and end of the string. This method is preferable because it leverages built-in functionality, making your code cleaner and often faster.
Using Recursion
Recursion can be both elegant and intuitive for certain problems, including string reversal. By defining a base case and simplifying the string step by step, we can achieve the desired output.
Code Snippet: Recursive Method
#include <iostream>
#include <string>
using namespace std;
string reverseString(string str) {
if (str.empty()) {
return str;
}
return str.back() + reverseString(str.substr(0, str.size() - 1));
}
In this method, if the string is empty, it simply returns the empty string. Otherwise, it takes the last character of the string and concatenates it with the result of a recursive call to `reverseString` using a substring that excludes the last character. This approach showcases the power of recursion, though it may introduce a stack overhead for large strings.
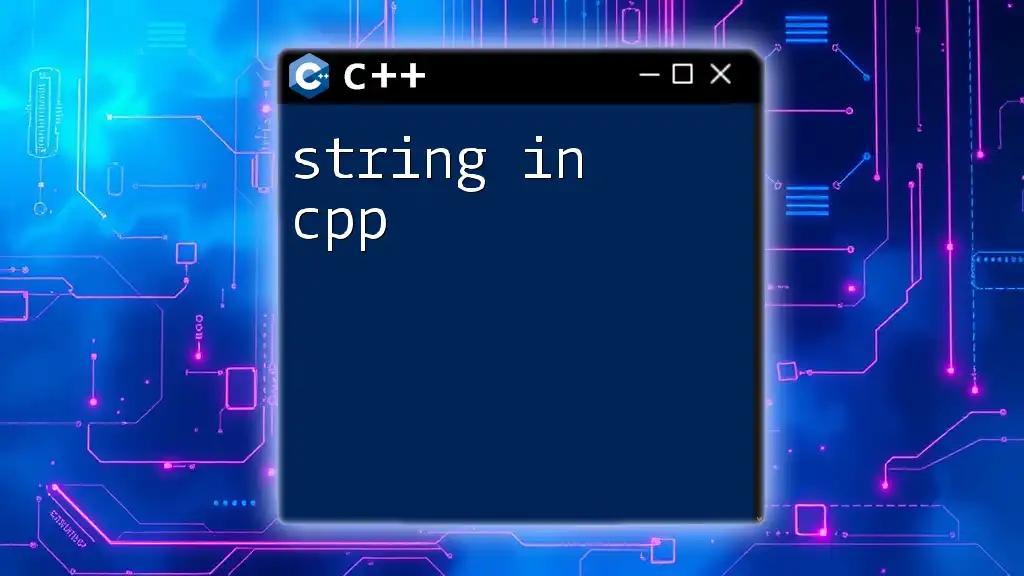
Tips for Choosing the Right Method
When deciding on which method to use for reversing a string in C++, consider the following factors:
- Efficiency: The loop and `std::reverse` methods typically provide better time complexity compared to recursion, which can suffer from stack overflow errors with extensive string lengths.
- Clarity: If you prioritize readability and simplicity, `std::reverse` offers a clean solution. Loops, while slightly more verbose, are very straightforward for beginners.
- Recursion: This method is elegant but should be used with caution, especially with large strings due to its potential for high memory usage.

Common Pitfalls in String Reversal
Handling Edge Cases
When implementing string reversal in C++, it's essential to consider edge cases such as:
- Empty Strings: An empty string should return an empty output.
- Single-Character Strings: These should return the same string, as reversing makes no change.
- Special Characters: Ensure that punctuation and whitespace are preserved in their respective places.
Example Scenarios:
- Input: `"hello"` ➔ Output: `"olleh"`
- Input: `""` ➔ Output: `""`
- Input: `"a"` ➔ Output: `"a"`
Memory Management
When working with strings in C++, it's crucial to understand the differences between `std::string` (which manages memory automatically) and C-style strings (character arrays). Using standard strings is generally safer and more manageable, as they help prevent memory leaks and buffer overflows.
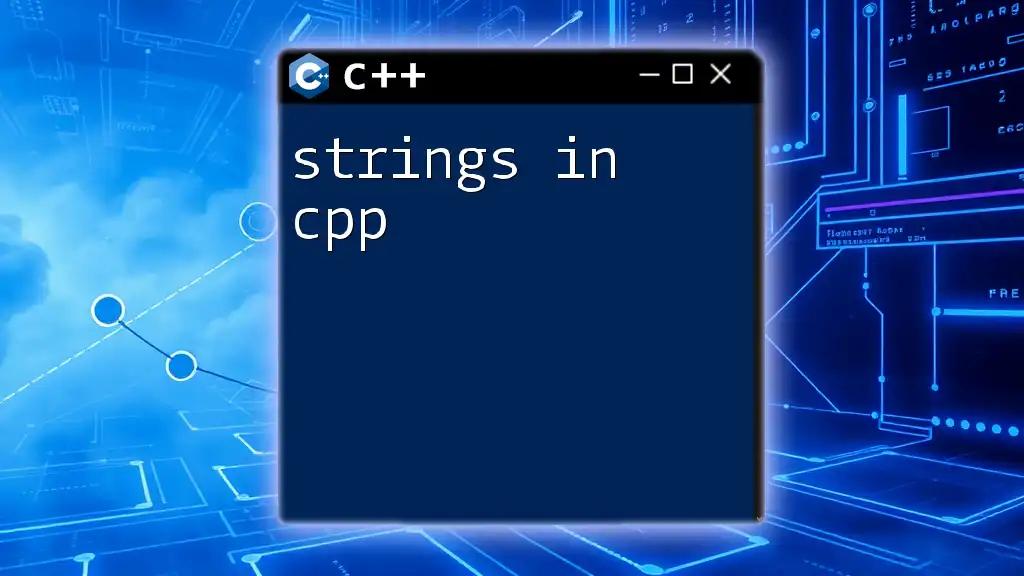
Practical Application of Reversed Strings
Palindrome Check
One of the intriguing applications of reversed strings is checking whether a string is a palindrome—meaning it reads the same forwards and backwards. We can leverage our string reversal technique to efficiently verify this.
Code Snippet: Palindrome Check
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
bool isPalindrome(string str) {
string reversed = str;
reverse(reversed.begin(), reversed.end());
return str == reversed;
}
In this example, we first create a copy of the input string and reverse it. By comparing the original string with the reversed version, we can determine if it is a palindrome. This technique is concise and leverages the power of the `std::reverse` function.

Conclusion
Reversing a string in C++ is a fundamental skill worth mastering as it has several practical applications in programming. From basic loops to leveraging the C++ Standard Library, there are multiple methods to perform this task efficiently. Understanding when and how to use each method can significantly enhance your string manipulation skills.
As you explore string reversal, consider the different scenarios you encounter in programming. Practice with various methods, and don't shy away from diving into algorithmic problems that involve string manipulations. This engagement will deepen your understanding and expertise in C++.