In C++, a `char` represents a single character, while a `string` is a sequence of characters, enabling handling text data more efficiently.
#include <iostream>
#include <string>
int main() {
char letter = 'A'; // single character
std::string word = "Hello"; // sequence of characters
std::cout << "Char: " << letter << std::endl;
std::cout << "String: " << word << std::endl;
return 0;
}
What is a Char in C++?
Definition of Char
In C++, a `char` is a data type that is typically used to store a single character. A `char` variable can hold any valid character defined in the character set (usually ASCII). For example, you can declare a `char` variable as follows:
char letter = 'A';
In this case, `letter` holds the character 'A'. It’s important to note that characters are enclosed in single quotes.
Characteristics of Char
The `char` data type is quite efficient in terms of memory usage. It occupies 1 byte of memory and is great for scenarios where memory conservation is a priority. Character encoding plays a critical role here; for instance, the ASCII value for 'A' is 65. Understanding these values can help you perform low-level operations as well.
Common Use Cases for Char
`Char` variables are often used in several practical scenarios:
- Storing Single Characters: When you need to store individual characters like letters, numbers, or symbols.
- Using in Switch Statements: They can effectively work in switch statements for decision-making processes.
- Creating Character Arrays: You can create arrays of `char` to represent strings.
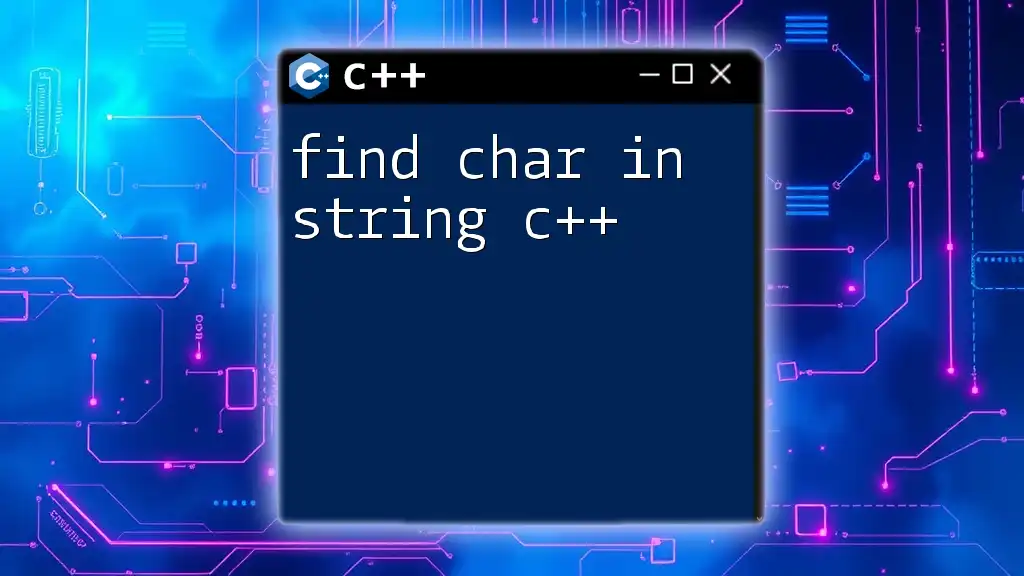
What is a String in C++?
Definition of String
In C++, a `string` is a class in the Standard Template Library (STL), providing a way to manipulate sequences of characters easily. Unlike `char`, which holds a single character, a `string` can store an entire sequence, allowing for complex text manipulations. Here’s how to declare and initialize a string:
#include <string>
std::string greeting = "Hello, World!";
In this case, `greeting` holds the entire phrase.
Characteristics of String
Strings are dynamic in nature; they can adjust their size as required. This flexibility allows you to store varying lengths of text without worrying about buffer sizes that come with `char` arrays. Strings usually grow and shrink automatically with operations performed on them, eliminating many sword-and-shield memory management issues.
Common Use Cases for String
Strings serve a variety of purposes in programming:
- Storing User Input: Easily capture and manipulate text from user interfaces.
- Text Processing: They are essential for tasks involving search, replace, substring extraction, and more.
- Handling Complex Data: When dealing with sentences or paragraphs, strings become invaluable.
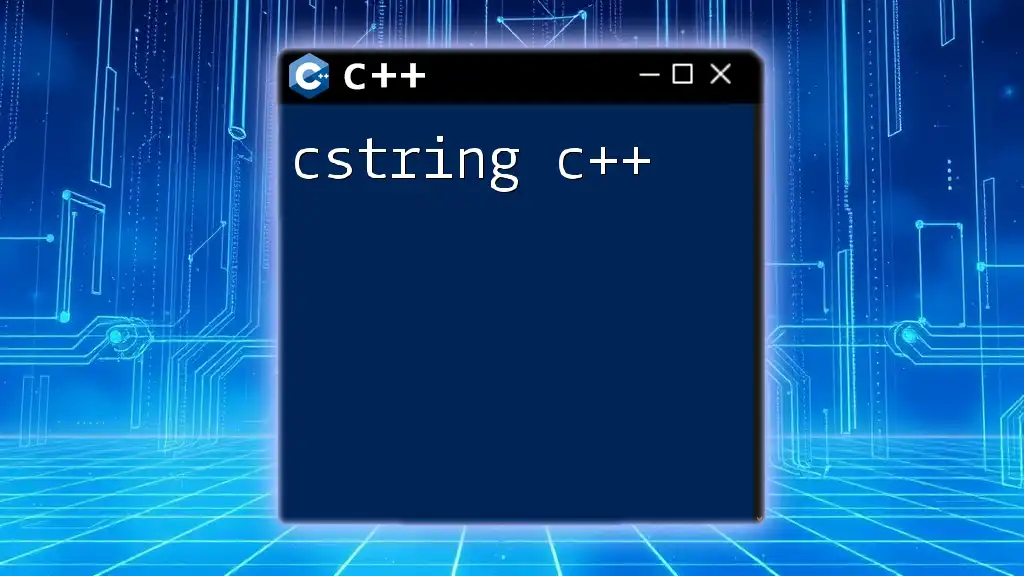
Differences Between Char and String
Memory Usage
When discussing char vs string in C++, one of the primary differences is their memory usage:
- A `char` always occupies 1 byte.
- A `string`, however, is dynamically sized, meaning its storage grows based on its content, often utilizing additional bytes for management.
Operations and Manipulation
Char Operations
Basic operations for `char` include:
- Assignment: Assigning values to chars.
- Comparisons: You can directly compare `char` variables to determine equality or order.
Example: Comparing characters:
char char1 = 'A';
char char2 = 'B';
if (char1 == char2) {
// Do something if they are equal
}
String Operations
Strings, equipped with rich member functions, support numerous operations:
- Concatenation: Combine two strings easily.
- Finding Length: Quickly obtain the size of a string.
Example: String concatenation:
std::string combined = greeting + " How are you?";
This example connects `greeting` with additional text effortlessly.
Performance Considerations
When it comes to performance, `char` can be more efficient for fixed-size tasks because of its minimal overhead. In contrast, `string` is better for dynamic scenarios where text manipulation is required, despite potentially incurring higher computational costs due to resizing activities.
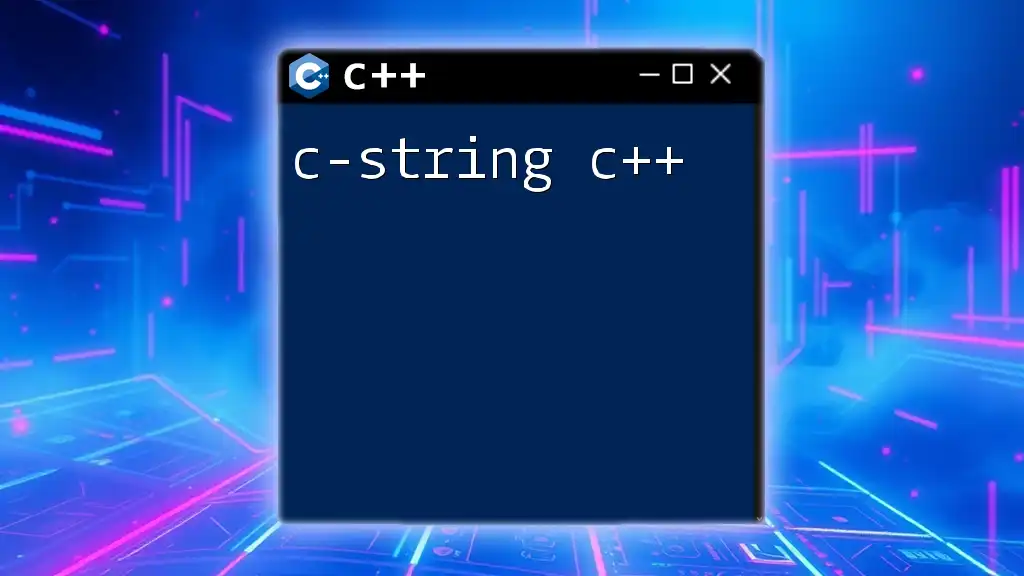
When to Use Char vs String
Scenarios for Using Char
Use `char` when:
- You are working in memory-constrained environments.
- You only need to store single characters.
- You are performing low-level programming tasks, such as in embedded systems.
Scenarios for Using String
Opt for `string` when:
- You need to handle user input.
- You want to perform complex manipulations, like searching or substring extraction.
- You anticipate variable or dynamic text lengths.
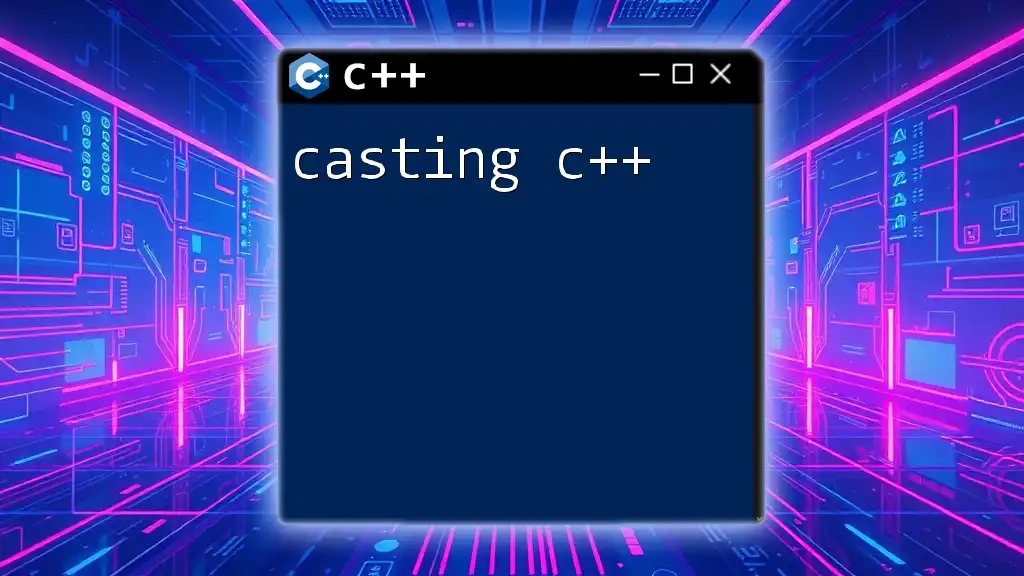
Best Practices for Using Char and String
Choosing the Right Type
To choose between `char` and `string`, consider the requirements of your application. If you foresee a necessity for sequences of text, strings are the way to go. If you only need to store isolated characters, chars suffice.
Memory Management Tips
- Char: Since it’s a primitive type, it doesn’t require any special memory management.
- String: Always ensure proper initialization to avoid undefined behavior, especially if you’re manipulating memory directly or interfacing with C-style strings.
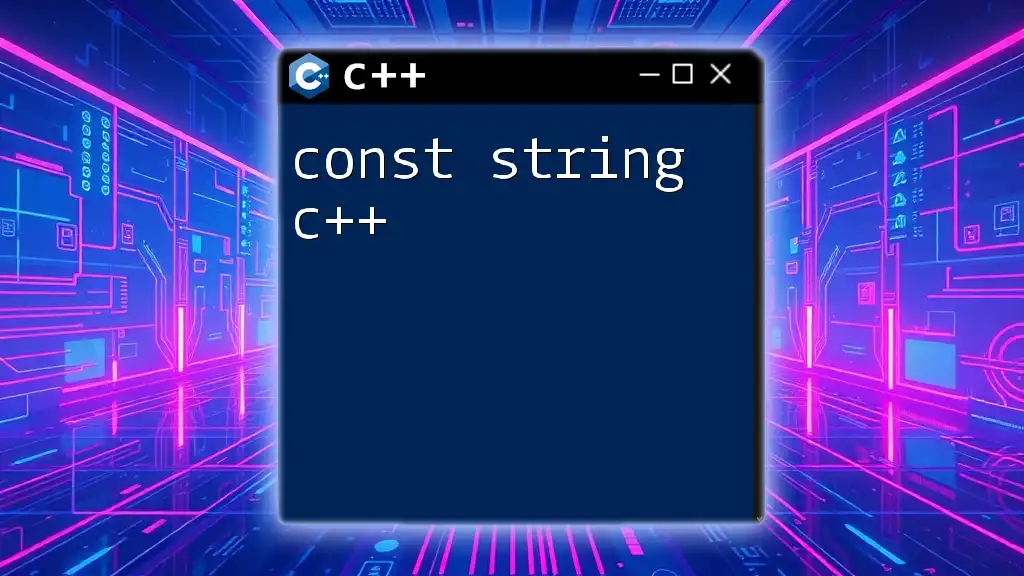
Tips for Working with Strings in C++
Common String Functions
The string class comes equipped with various functions. Familiarize yourself with essential methods like:
- `length`: To get the size of a string.
- `find`: To locate substrings within a string.
Example: Using the `find` method:
size_t pos = greeting.find("World");
This finds the position of "World" within the greeting.
String Iteration Techniques
Iterating over characters in a string can be accomplished using a loop:
for (char& c : greeting) {
// Process character c
}
This allows you to inspect, transform, or build custom logic for each character.
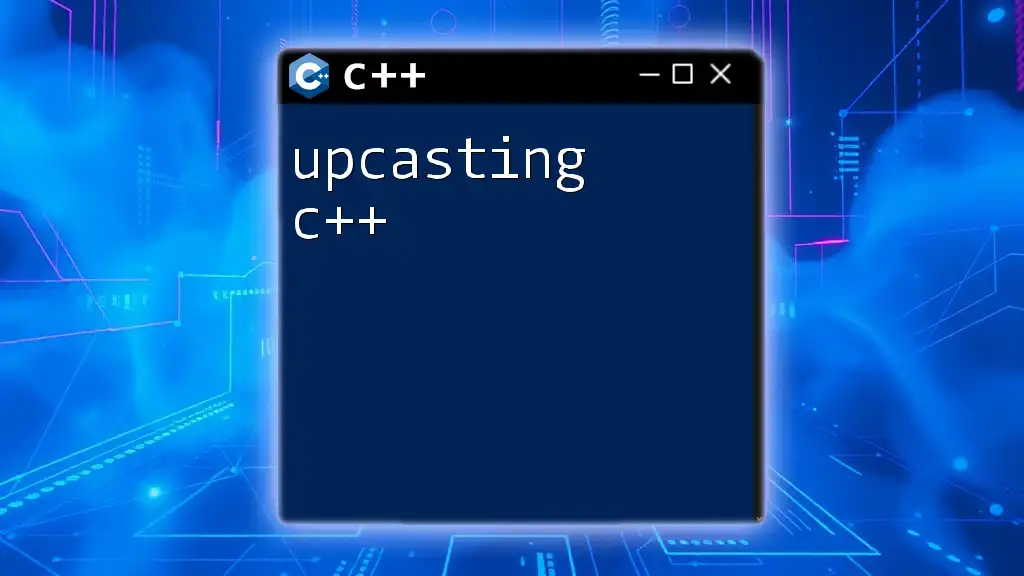
Conclusion
In the grand debate of char vs string in C++, understanding their distinct characteristics is crucial for effective programming. `Char` provides a compact, efficient means of handling individual characters, while `string` offers a versatile, expandable approach for sequences of text. By discerning when to use each type and following best practices, you can enhance the efficiency and readability of your C++ code.
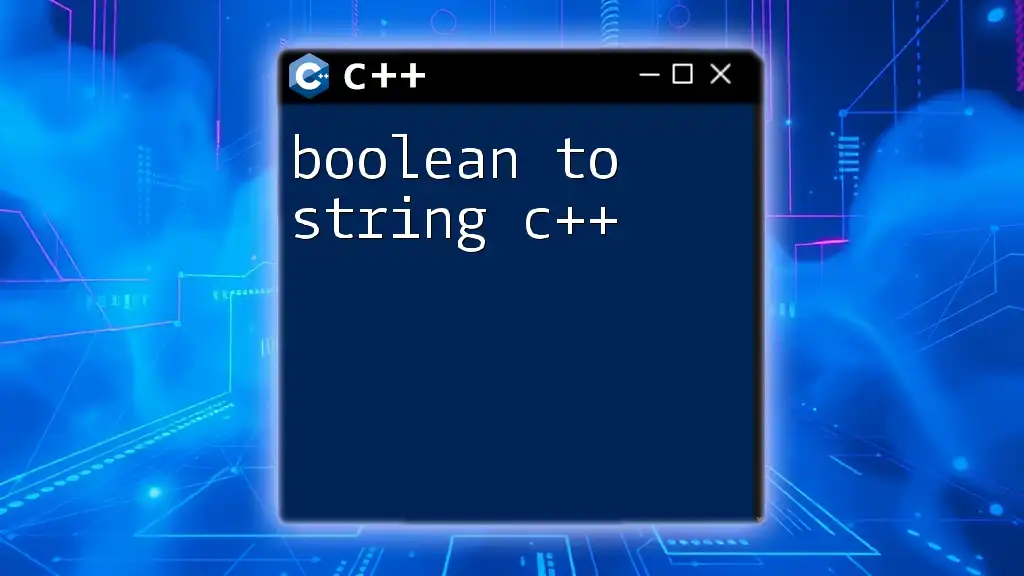
Additional Resources
Further Reading
Explore documentation and tutorials on C++ `char` and `string` for deeper insights into usage and best practices.
Exercises
To reinforce your understanding, consider attempting exercises involving both `char` and `string`. Write small programs that implement string manipulations and character comparisons to solidify your grasp on the concepts discussed.