In C++, you can convert a boolean value to a string using the `std::to_string` function alongside a conditional operator to represent `true` as "true" and `false` as "false". Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
std::string boolToString(bool value) {
return value ? "true" : "false";
}
int main() {
bool myBool = true;
std::cout << boolToString(myBool) << std::endl; // Output: true
return 0;
}
Understanding Boolean in C++
What is a Boolean?
A boolean is a fundamental data type in C++ that can hold one of two values: `true` or `false`. This binary state is crucial for controlling the flow of a program, facilitating decision-making processes in conditional statements and loops.
Boolean Representation in C++
In C++, a boolean is represented internally as an integer, where `0` is equivalent to `false`, and `1` is equivalent to `true`. This representation allows C++ to perform various operations efficiently, yet working with boolean values directly provides clarity and intention in your code.
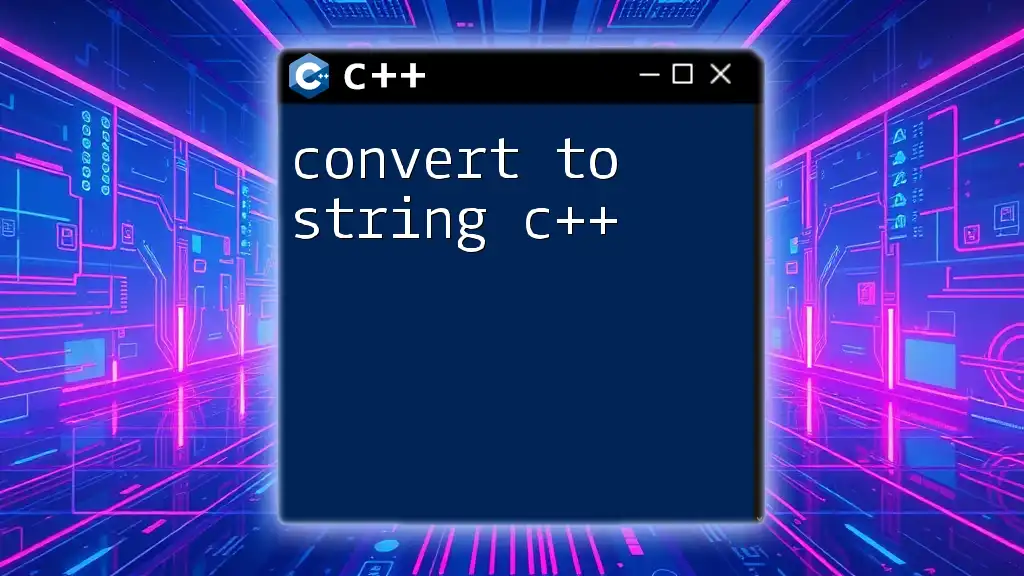
Why Convert Boolean to String?
Practical Applications
Converting a boolean to a string is essential in several scenarios. For instance, when logging application statuses, displaying results in user interfaces, or even debugging, you may need a human-readable format for boolean values. A string representation enhances readability, making it easier to communicate information to users or developers.
Benefits of String Representation
String representations not only provide clarity but also improve the user experience within applications. For example, instead of displaying `0` or `1` to indicate a boolean state, you can show `"true"` or `"false"`, which is more intuitive.
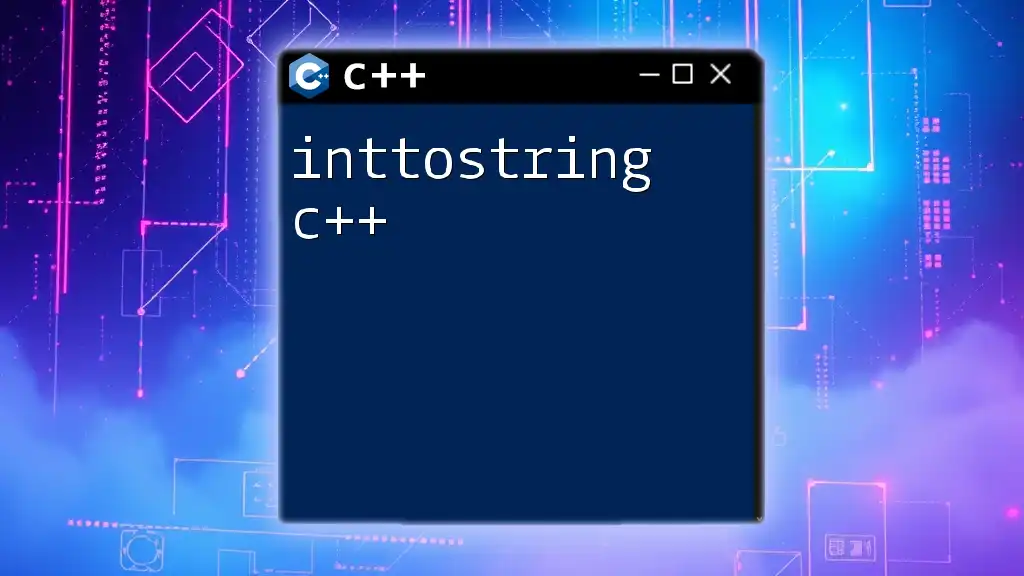
Conversion Methods
Standard Method: Using `std::to_string()`
One straightforward way to convert a boolean to a string is by utilizing `std::to_string()`. This method takes advantage of the ternary operator to return a string representation of the boolean value.
Here’s how it can be done:
#include <iostream>
#include <string>
std::string boolToString(bool value) {
return value ? "true" : "false";
}
int main() {
bool myBool = true;
std::cout << boolToString(myBool) << std::endl; // Output: true
return 0;
}
In this snippet, the `boolToString` function checks the value of `value` and returns the respective string. This method is both concise and easy to understand.
Custom Function for Conversion
Creating a custom function allows for flexibility and control over the formatting of the output. You might want to ensure that the casing is consistent or add any specific prefixes.
Consider this example:
std::string customBoolToString(bool value) {
if (value) {
return "True";
}
return "False";
}
In this function, the boolean is checked, and the corresponding string with a specific casing is returned.
Using C++ Streams for Conversion
Using `std::ostringstream` for conversion provides a more versatile approach, especially when you want to maintain specific formatting or append other strings.
Here’s how to use streams for this purpose:
#include <sstream>
std::string streamBoolToString(bool value) {
std::ostringstream oss;
oss << std::boolalpha << value; // std::boolalpha gives textual representation
return oss.str();
}
// Sample usage
In this method, `std::boolalpha` is used to print boolean values as `true` or `false` instead of `1` or `0`. This approach integrates well into larger applications where such output formatting is beneficial.
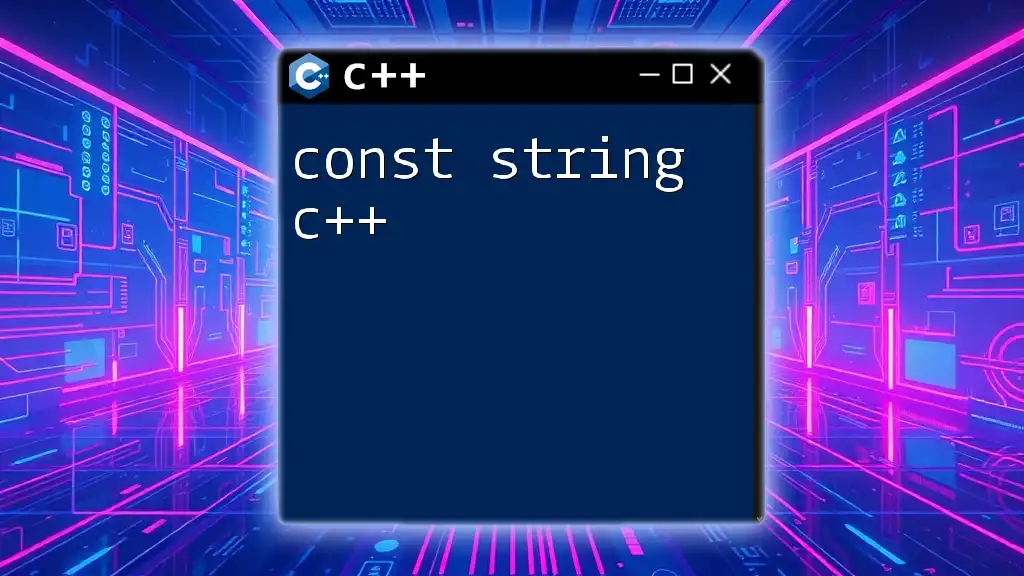
Example Use Cases
Logging and Debugging
In practical scenarios, converting booleans to strings can simplify logging. For instance, consider the following function designed to log the status:
void logStatus(bool status) {
std::cout << "Status: " << boolToString(status) << std::endl;
}
This implementation ensures that the log output is straightforward, making it easier to review logs during debugging.
User Interface Display
When building user interfaces, the clarity of boolean data is paramount. For instance, if a checkbox indicates whether a feature is enabled or disabled, converting its state into a string allows you to convey this more effectively to end-users.
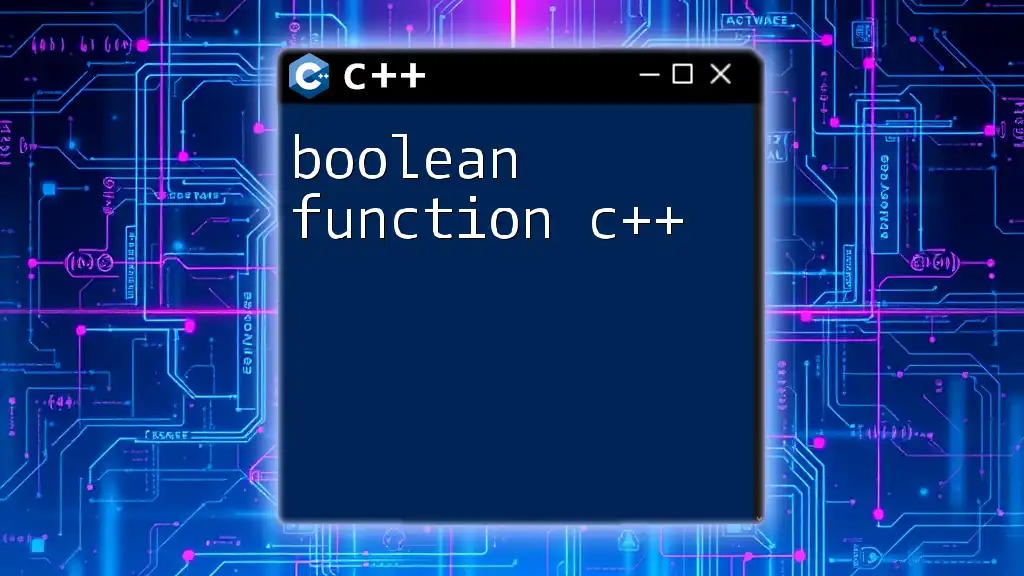
Performance Considerations
Efficiency of Different Methods
Each conversion method has its efficiency and performance characteristics. The standard method using `std::to_string()` is quick and convenient for simple conversions. In contrast, using `std::ostringstream` may introduce slight overhead but offers more formatting options.
Trade-offs
When deciding which method to implement, consider the trade-offs between performance and readability. While performance may be critical in time-sensitive applications, clarity often enhances maintainability and aids long-term project success.
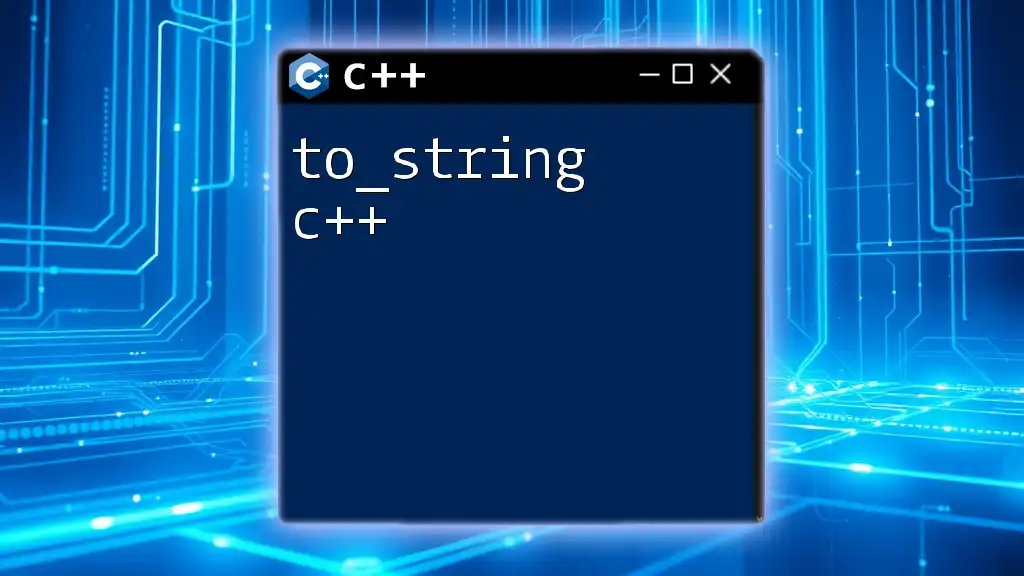
Conclusion
Converting a boolean to string in C++ is a fundamental skill that can enhance both the readability and functionality of your code. By employing various methods such as the standard approach, custom functions, or streams, programmers can easily adapt their solutions to fit different needs. Experimenting with these examples can provide deeper insights into effectively managing boolean values in your applications.
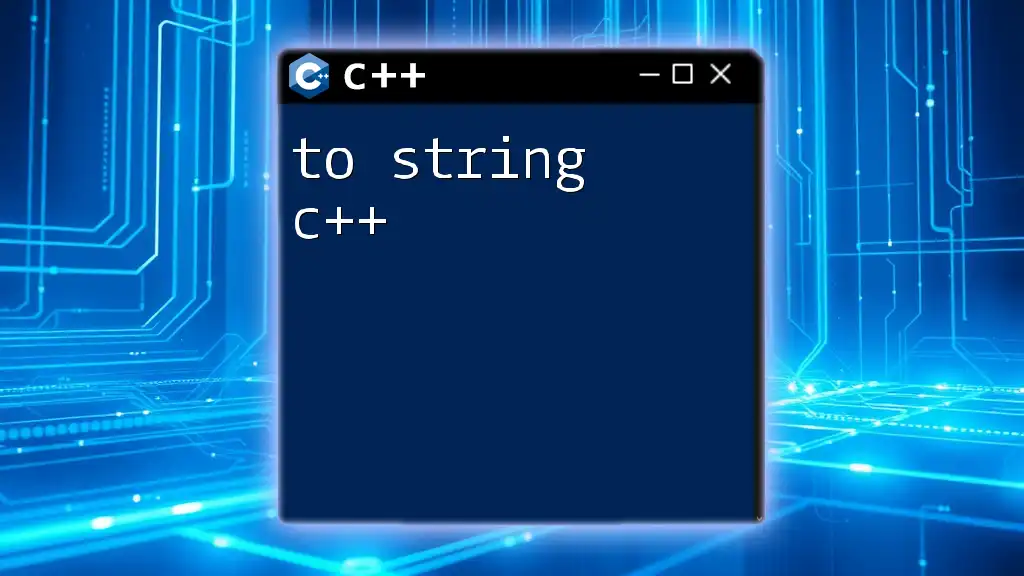
Additional Resources
References
For further reading, consult the official C++ documentation for `std::to_string()` and `std::ostringstream`, as well as other resources that delve into effective C++ programming practices.
Frequently Asked Questions
You might also explore how to convert other data types to strings and the best practices for formatting output in C++. This knowledge can greatly bolster your understanding of string manipulations in C++.