A boolean function in C++ is a function that returns a value of either `true` or `false`, often used for conditions and logic in programming.
Here's a simple example:
#include <iostream>
using namespace std;
// Function that checks if a number is even
bool isEven(int number) {
return number % 2 == 0;
}
int main() {
int num = 4;
cout << num << " is even: " << isEven(num) << endl;
return 0;
}
Understanding Boolean Functions in C++
A Boolean function is a mathematical function that returns either true or false based on the input parameters. In programming, especially in C++, Boolean functions play a crucial role in decision-making processes, where they determine the flow of control in the application.
Role of Boolean Functions in Decision Making
Boolean functions are essential in implementing conditional statements, enabling programs to branch based on logical evaluations. For instance, when you check user inputs or when making game-related decisions, Boolean functions can dramatically streamline the application’s logic.
Consider a scenario where a user needs to input an age. A Boolean function could be used to check whether their input meets a specific criterion, such as being old enough to access a service.
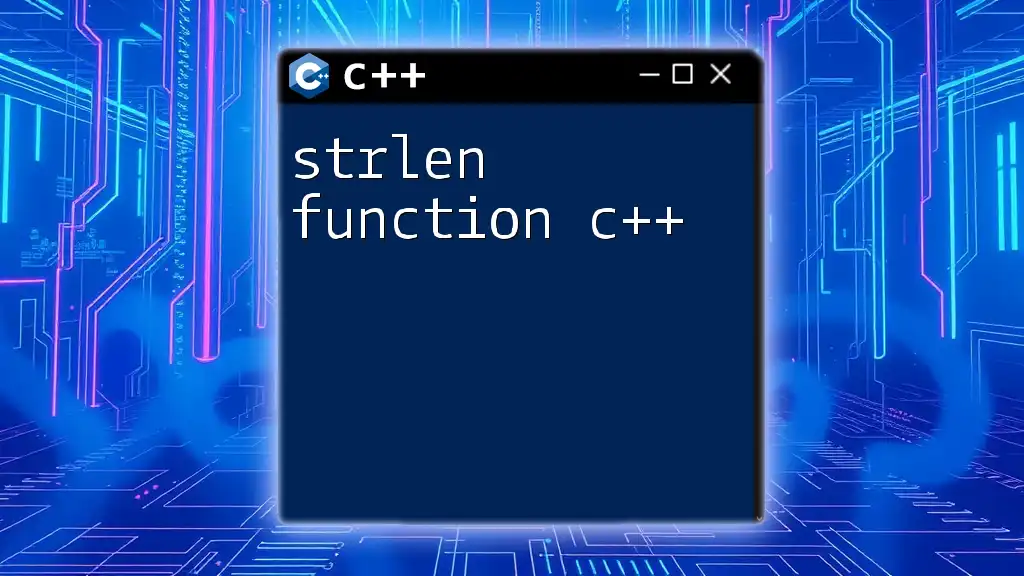
Creating Boolean Functions in C++
Syntax of a Boolean Function
Creating a Boolean function in C++ is straightforward. The basic structure consists of the return type, which is specified as `bool`, followed by the function name and any parameters. Here's the syntax:
bool functionName(parameters) {
// function body
}
Example of a Simple Boolean Function
Let’s define a basic Boolean function to check if a number is even:
bool isEven(int number) {
return number % 2 == 0;
}
In this code snippet, the function `isEven` takes an integer as input and returns true if the number is even (i.e., divisible by 2), and false otherwise. By leveraging the modulo operator (`%`), we can easily ascertain the evenness of the number.
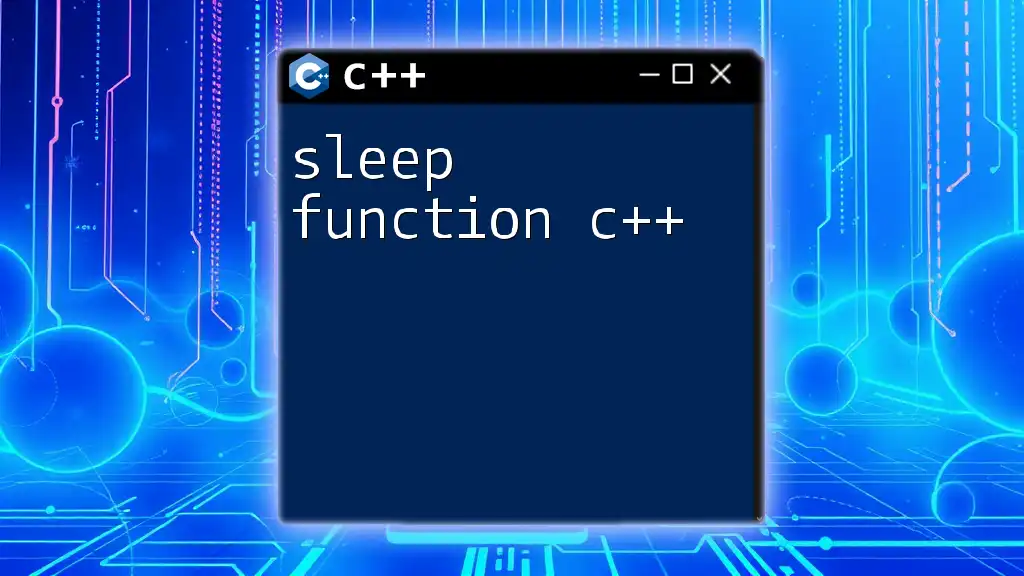
Function bool C++
More Elaborate Boolean Functions
Boolean functions can also be designed to handle multiple conditions. For example, we can create a function that checks if a number is both positive and even:
bool isPositiveAndEven(int number) {
return number > 0 && isEven(number);
}
In this case, the `isPositiveAndEven` function utilizes logical operators (`&&`, which stands for AND) to combine two conditions. It returns true only if both conditions are satisfied.
Best Practices for Writing Boolean Functions
When writing Boolean functions, follow these best practices:
-
Use clear and descriptive function names: This enhances readability and maintainability. For instance, `isEven` conveys precisely what the function does.
-
Keep functions concise: Each function should ideally focus on a single task or condition. This helps in easier debugging and testing.
-
Avoid side effects: Boolean functions should not modify global states or have unintended consequences. Keeping functions pure improves reliability.
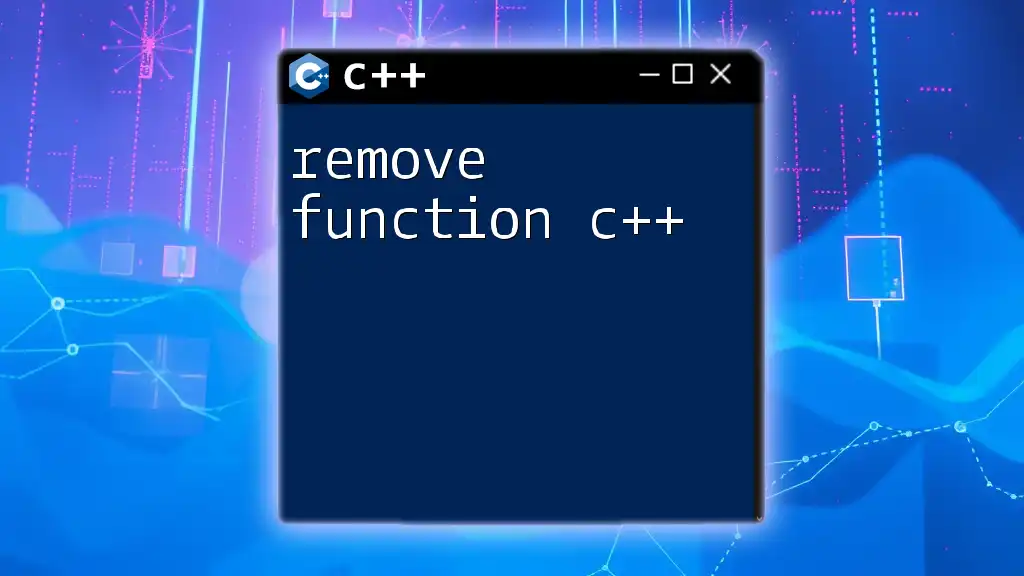
Utilizing Boolean Functions in C++
Implementing Boolean Functions in Control Structures
Boolean functions find significant application in control structures, notably `if` statements. The following example illustrates how to use a Boolean function within an `if` statement:
if (isEven(number)) {
cout << "The number is even." << endl;
}
This code checks whether the `number` is even and, if so, prints a message. This illustrates how the program branches based on the output of the Boolean function.
Combining Multiple Boolean Functions
You can also combine multiple Boolean functions to create complex conditions.
if (isPositiveAndEven(number) && isLessThanHundred(number)) {
cout << "The number is positive, even, and less than 100." << endl;
}
In this example, the function checks if the number meets two conditions, demonstrating how Boolean logic can be leveraged to refine decision-making.
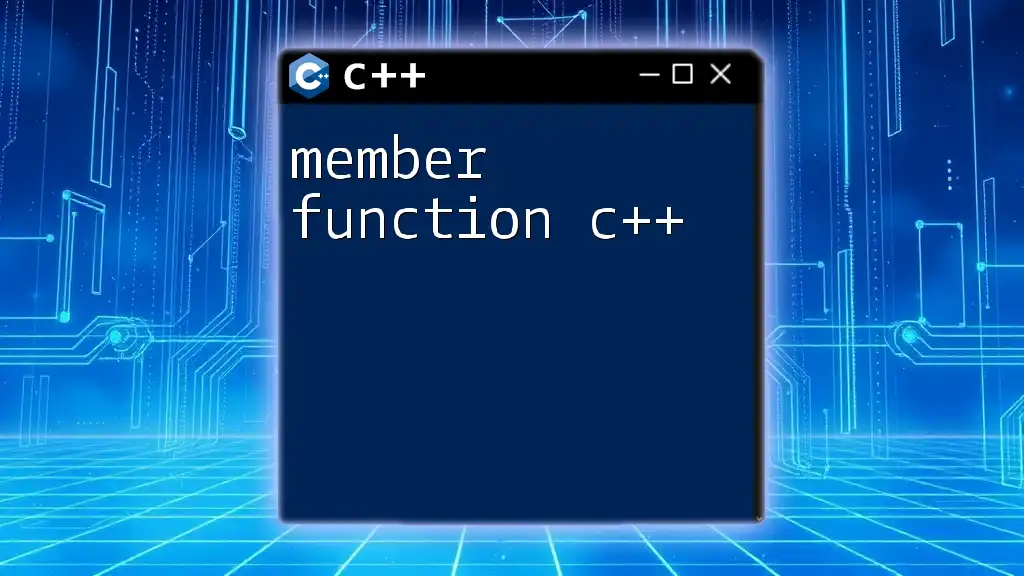
Advanced Topics in Boolean Functions
Overloading Boolean Functions
C++ allows for function overloading, which is the ability to define multiple functions with the same name but different signatures. This is particularly useful for Boolean functions that handle varied input types:
bool isEven(double number) {
return static_cast<int>(number) % 2 == 0;
}
This overloaded version of `isEven` accepts a `double`, converting it to an `int` before performing the even check. Function overloading provides flexibility and usability in your functions.
Boolean Algebra in C++
Understanding basic principles of Boolean algebra can enhance your programming skills in C++. It involves logical operations and identities that help in optimizing Boolean expressions. Familiarizing yourself with concepts such as De Morgan's laws can lead to more efficient code and better performance in systems where complexity arises from numerous conditions.
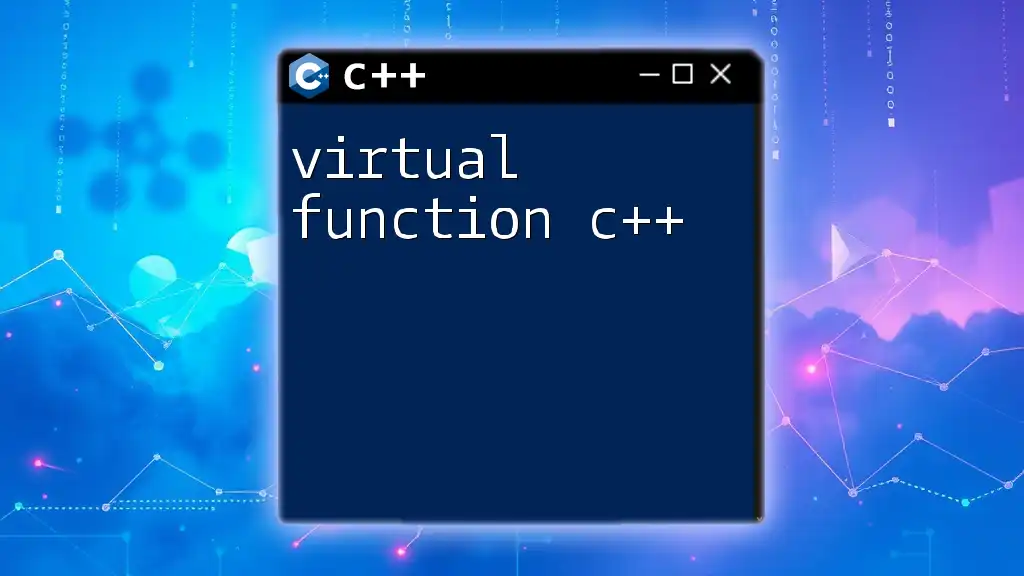
Debugging and Troubleshooting Boolean Functions
When debugging Boolean functions, several common mistakes often occur. Here are tips to help you troubleshoot effectively:
-
Common Mistakes: Watch out for incorrect logical operators (e.g., using `&&` instead of `||`). Such oversights can cause unexpected results.
-
Debugging Tips: Utilize `cout` statements or breakpoints to track how input data flows through your functions and what values are returned. This can aid in understanding the function's behavior and isolating issues.
By following these tips, you can avoid many pitfalls associated with writing and implementing Boolean functions in C++.
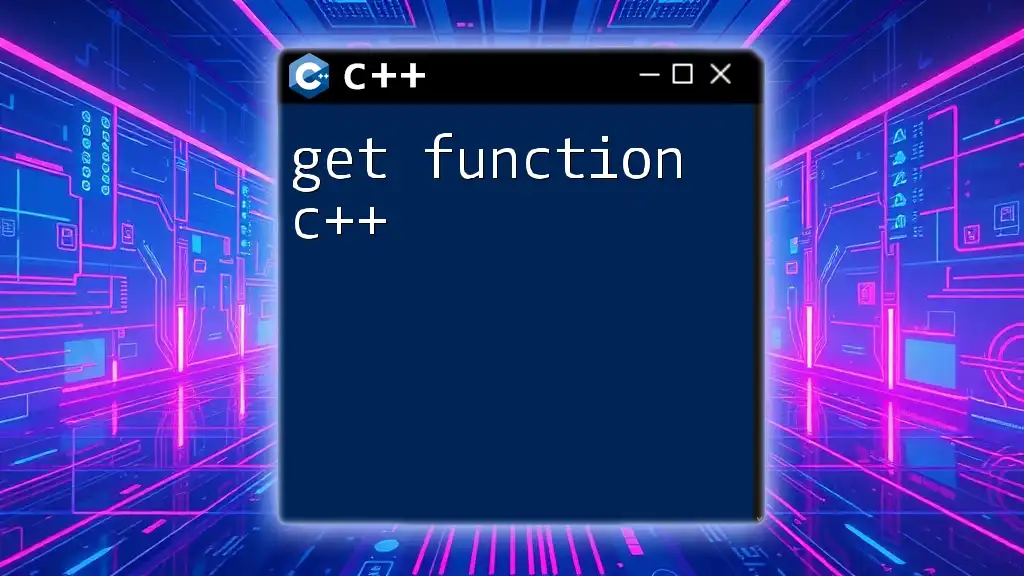
Conclusion
Mastering Boolean functions in C++ is vital for any programming endeavor. Understanding how to create, combine, and implement these functions can significantly improve the logical structure of your code. Moreover, by applying good practices and learning to debug effectively, you create robust applications that can handle a wide range of scenarios. Remember, practice is key, so start applying these concepts in your C++ coding projects today.
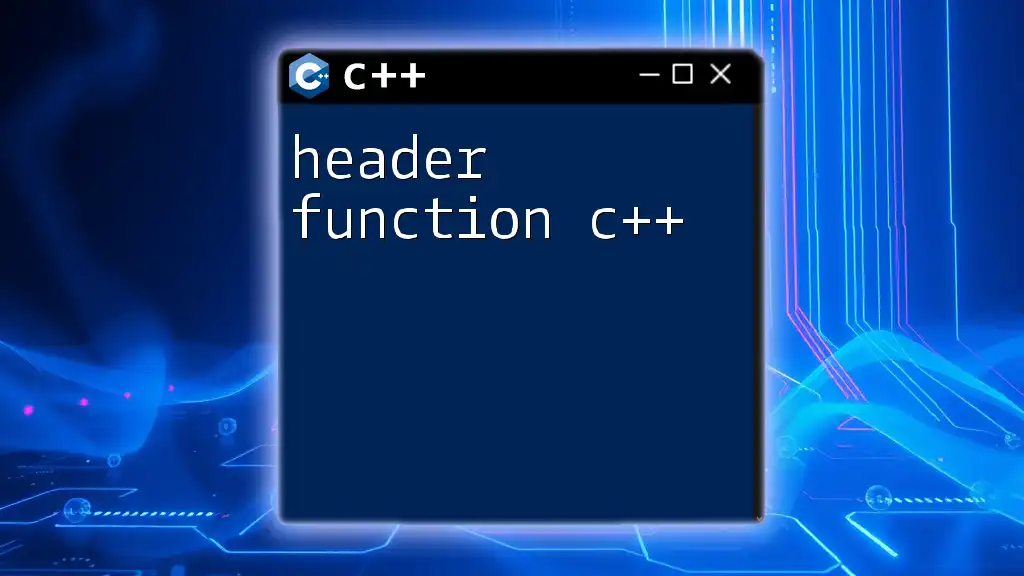
Additional Resources
For further exploration, consider looking at online tutorials, coding exercises, or literature on C++ that focuses on more advanced Boolean logic and applications to deepen your understanding.