A member function in C++ is a function that is defined within a class and can operate on its instances, allowing the function to access and modify the class's member variables.
Here's a simple example:
#include <iostream>
using namespace std;
class MyClass {
public:
void displayMessage() {
cout << "Hello from MyClass member function!" << endl;
}
};
int main() {
MyClass obj;
obj.displayMessage();
return 0;
}
Understanding Member Functions in C++
A member function is a function that is defined within a class and operates on its member variables. Member functions are integral to object-oriented programming in C++, as they encapsulate both the data and behavior relevant to a class, enabling data abstraction and encapsulation.
Key Characteristics of Member Functions
- Scope: Member functions have access to the class's private and protected members. This allows for controlled access to the internal state of an object.
- Binding: Member functions can be invoked on instances of the class they belong to, usually referred to as objects.
- Overloading: You can define multiple member functions with the same name but different parameters, allowing for flexibility through function overloading.
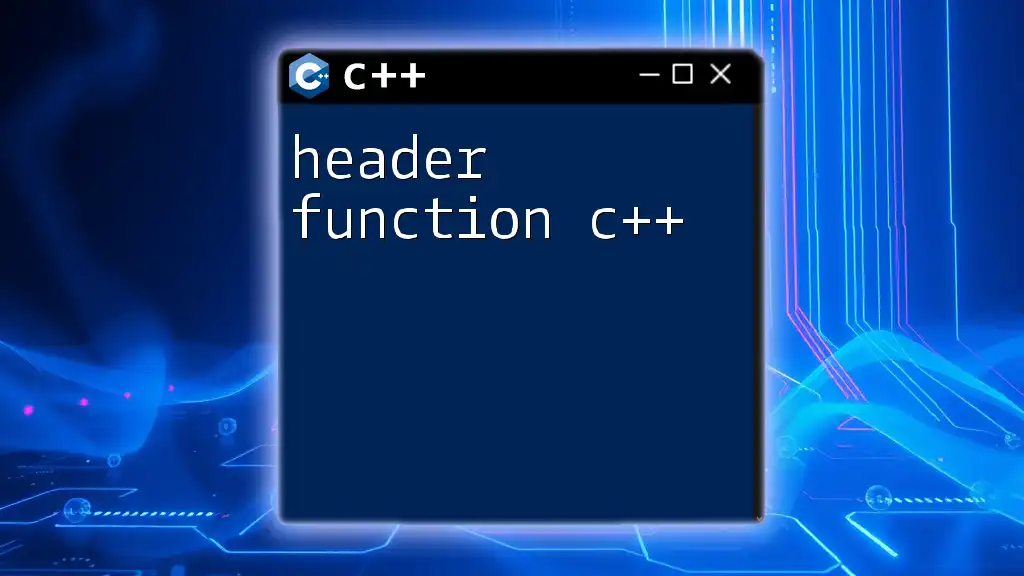
Types of Member Functions
Regular Member Functions
Regular member functions are the most common type. They operate on the instance of the class, using its member variables to perform operations.
The syntax for defining a regular member function typically follows this structure:
returnType ClassName::functionName(parameters) {
// function body
}
Example:
class Box {
public:
int volume() {
return length * width * height;
}
private:
int length;
int width;
int height;
};
In this example, the `volume` function computes and returns the volume of a `Box` object by multiplying its private member variables.
Static Member Functions
Static member functions belong to the class rather than any particular object. This means they can be called without creating an instance of the class.
The syntax for a static member function is similar, but you use the `static` keyword:
static returnType functionName(parameters);
Example:
class Math {
public:
static int add(int a, int b) {
return a + b;
}
};
You can call `Math::add(5, 3);` without needing to create an object of `Math`, highlighting the utility of static member functions for utility functions.
Const Member Functions
A const member function guarantees that it will not modify any member variables of the class, allowing developers to safely call it on const objects.
To declare a const member function, add the `const` keyword after the function signature:
returnType functionName() const;
Example:
class Circle {
public:
double getArea() const {
return 3.14 * radius * radius;
}
private:
double radius;
};
Calling `getArea()` on a `const Circle` instance ensures that the state of the object remains unchanged.
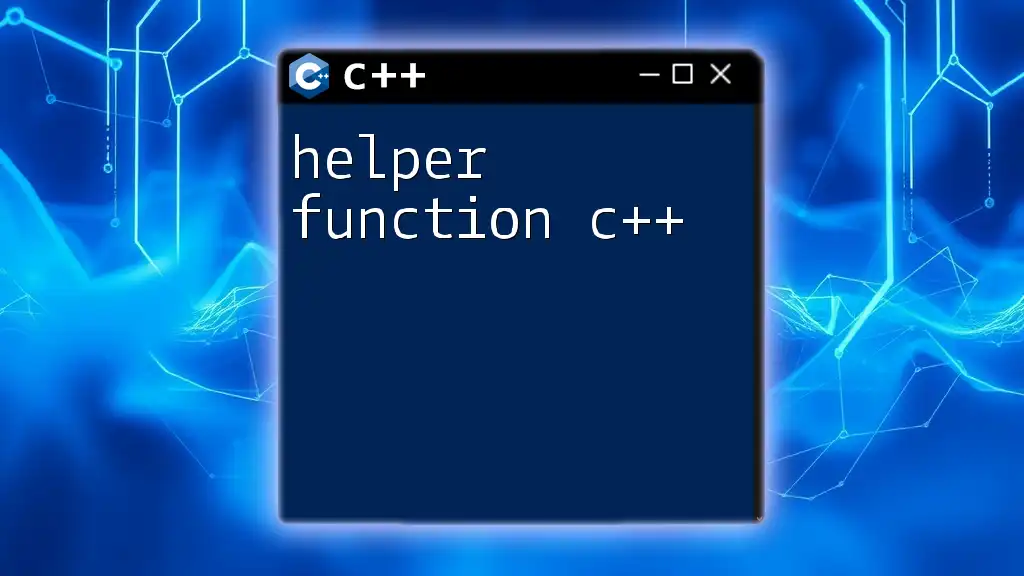
Defining Member Functions
Syntax for Declaring Member Functions
When you declare a member function, include its return type, the class name followed by the scope resolution operator (`::`), and the function signature containing the parameters (if any). This structure differentiates member functions from regular functions.
Defining Member Functions Outside the Class
You can also define member functions outside the class declaration. This keeps class declarations clean and separates interface from implementation.
Example:
class Employee {
public:
void setSalary(double s);
private:
double salary;
};
void Employee::setSalary(double s) {
salary = s;
}
In this example, `setSalary` is declared in the class but defined outside, making it clear where the class is declared and where its implementation lies.
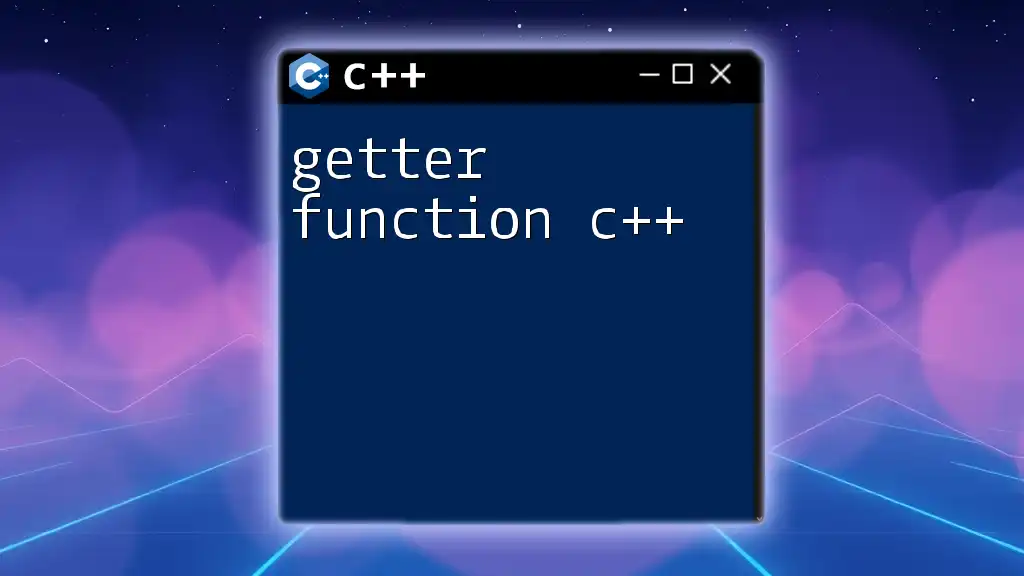
Access Specifiers in Member Functions
Public Member Functions
Public member functions can be accessed from outside the class. They are the primary means by which outside code interacts with an object. This accessibility allows the best practices of encapsulation and abstraction to be maintained.
Private Member Functions
Private member functions are hidden from the outside world and can only be called from other member functions of the class. This encapsulation provides more control and helps maintain the integrity of the object's state.
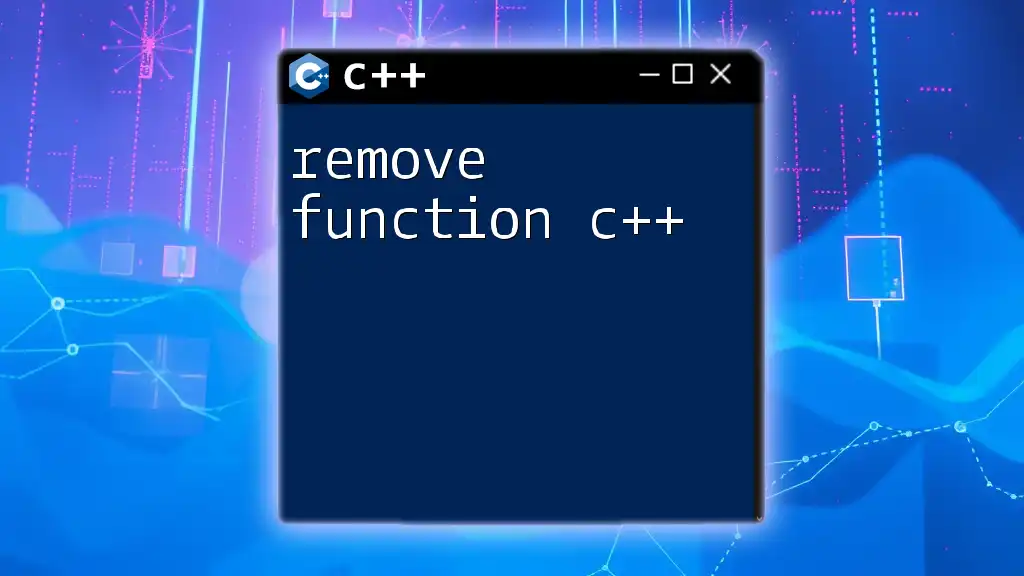
Need for Member Functions
Member functions encapsulate functionality within a class, allowing for better organization of code. By grouping related data and behavior, they promote code reusability and clarity. This structure makes understanding and maintaining codebases more straightforward.
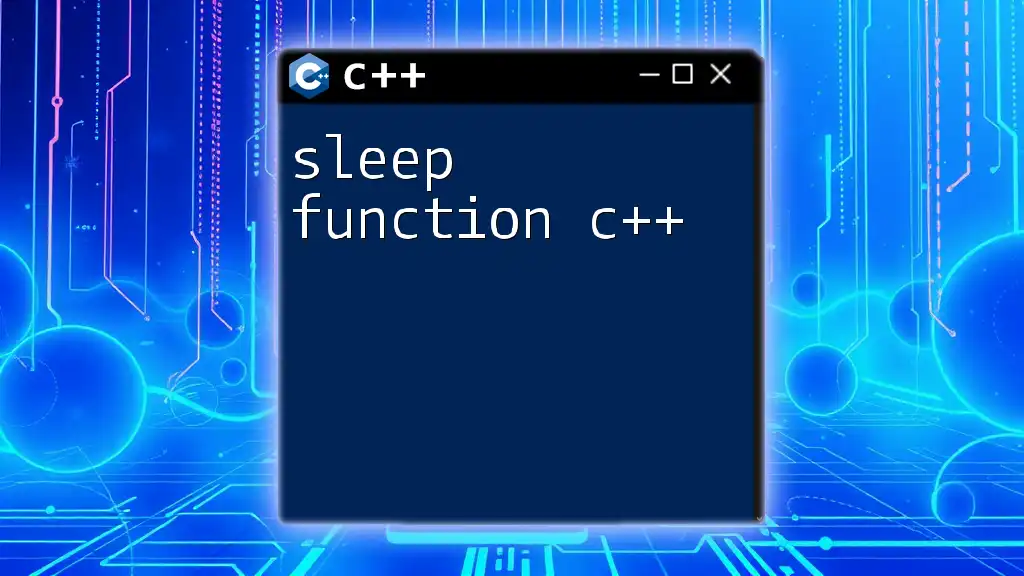
Member Function Overloading
Member function overloading allows you to define multiple functions with the same name but differing parameter lists. Overloading enhances the flexibility of your classes by enabling operations to accept various types.
Example:
class Printer {
public:
void print(int i) {
std::cout << "Integer: " << i << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
};
In this example, both `print` function overloads can be called depending on whether an integer or a double is passed.
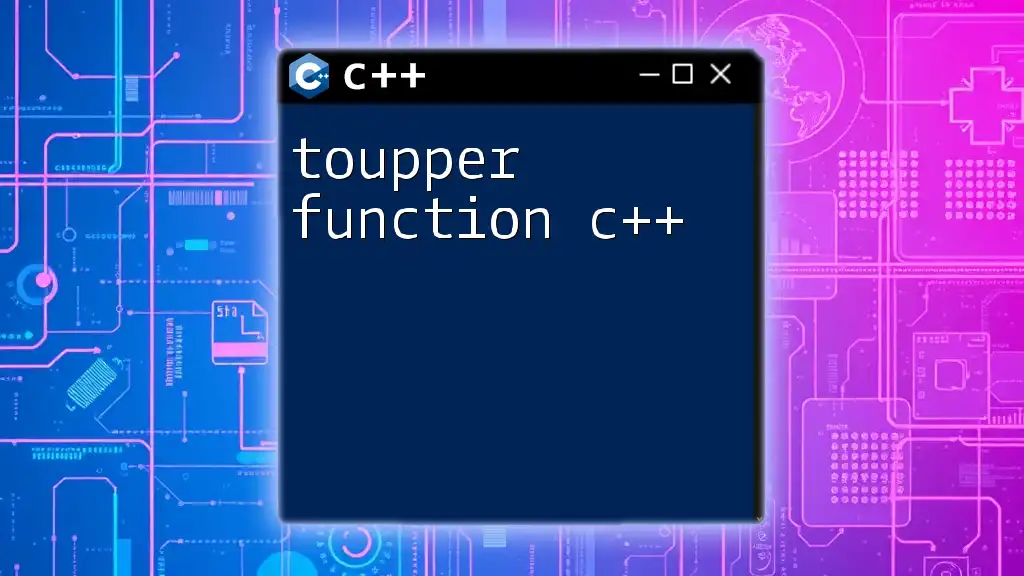
The Role of `this` Pointer in Member Functions
The `this` pointer is a special pointer that points to the object for which a member function is called. It allows member functions to access member variables and other member functions of the same class.
When working with member functions, you often see the `this` pointer used when parameters shadow member variables. Using `this` makes it clear that you are referring to the member variable.
Example:
class Car {
public:
void setSpeed(int speed) {
this->speed = speed; // Distinguishing between the parameter and the member variable
}
private:
int speed;
};
Using `this->speed` clarifies that you are assigning the value from the parameter `speed` to the member variable `speed`.
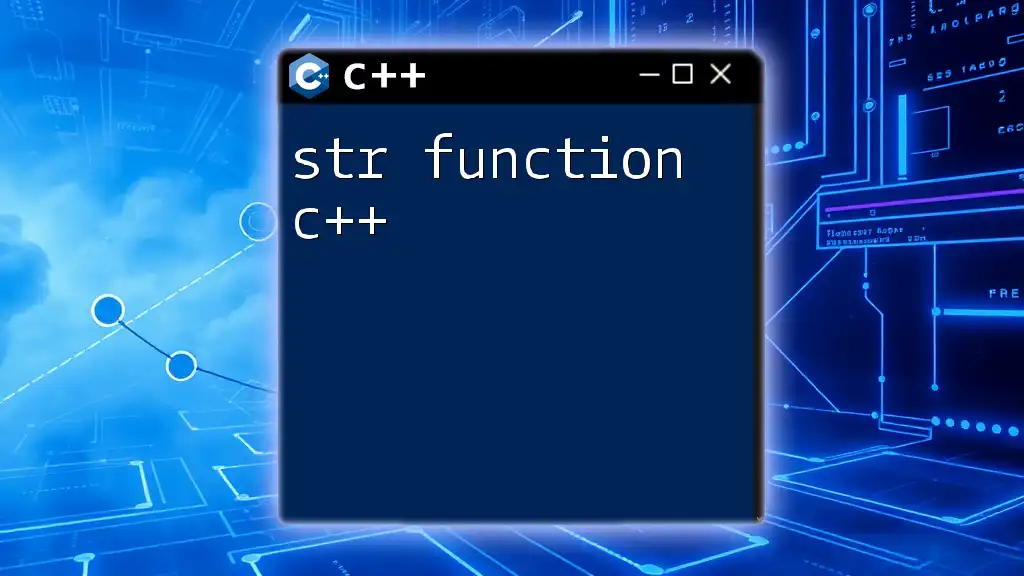
Conclusion
In summary, understanding member functions in C++ is crucial for effective software development. They are pivotal in enhancing code readability, functionality, and reusability. Familiarity with different types of member functions—regular, static, and const—along with their appropriate usage, is essential for every C++ programmer.
Embrace the power of member functions, and don't hesitate to practice implementing them in your own classes. Exploring more resources will deepen your understanding and expertise in using member functions effectively. From small utility functions to complex class designs, mastering this concept will elevate your C++ programming skills to new heights.
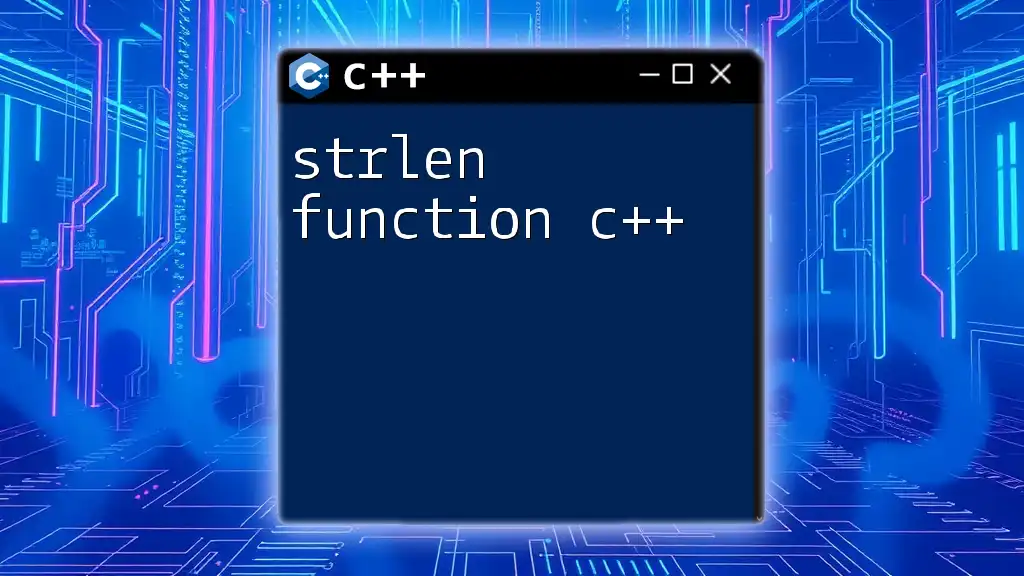
Additional Resources
To further your knowledge about member functions in C++, consider checking out reputable online C++ tutorials, books, and courses specifically designed to enhance your understanding of this topic.
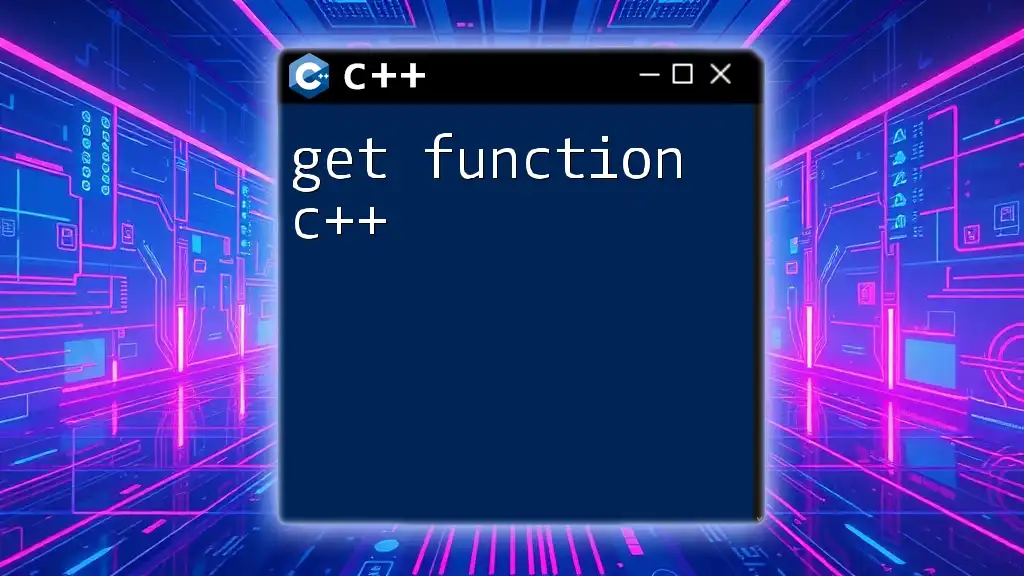
FAQs
-
What is a member function in C++? A member function is a function defined inside a class that operates on the class's data members.
-
Can member functions access private variables? Yes, member functions can access private and protected data members of their own class.
-
How do you call a static member function? You can call a static member function using the class name followed by the scope resolution operator, e.g., `ClassName::functionName()`.
By grasping these basics, you can effectively navigate the realm of member functions in C++, driving you towards becoming a proficient C++ developer.