A helper function in C++ is a small, reusable function that simplifies common tasks or calculations within a program to enhance code readability and maintainability.
Here's an example of a helper function that calculates the square of an integer:
int square(int num) {
return num * num;
}
What are Helper Functions?
Helper functions are specialized functions that assist in performing specific tasks within a program. They help break down complex operations into smaller, more manageable pieces, ultimately making code easier to read, understand, and maintain. In the context of C++ programming, helper functions are invaluable for keeping code organized and modular, allowing programmers to focus on different parts of a solution without overwhelming themselves with complexity.

Importance of Helper Functions in C++
Using helper functions in C++ offers several key advantages:
-
Code Organization and Modularity: By grouping related code into smaller functions, developers can easily navigate their programs. This modularity makes it easier to isolate and fix bugs.
-
Enhanced Readability and Maintainability: Code that employs helper functions tends to be more readable. Developers can quickly grasp the intent behind a function name, which aids in understanding the overall flow of the program.
-
Code Reuse and Reduction of Duplication: When common tasks are encapsulated in helper functions, the same code does not need to be rewritten multiple times. This promotes reusability and keeps the codebase cleaner.

Characteristics of a Good Helper Function
Simplicity and Clarity
A hallmark of a good helper function is its simplicity. It should focus on completing one specific task efficiently, avoiding overly complex operations. A clear and concise implementation allows other developers (and your future self) to follow the logic without getting lost in unnecessary details.
Proper Naming Conventions
Choosing appropriate names for helper functions is crucial. The function name should describe its purpose clearly. For instance, a function named `calculateArea` conveys its behavior more effectively than a vague name like `func1`. By adhering to good naming practices, you enhance the code's readability and facilitate collaboration among developers.

Creating Helper Functions in C++
Defining a Helper Function
Defining a helper function in C++ follows a straightforward syntax:
return_type function_name(parameters) {
// function body
}
Let’s take a basic example to illustrate how to define a helper function that adds two integers:
int add(int a, int b) {
return a + b;
}
Calling Helper Functions
To use the helper function inside your main program, you simply call it by its name, providing the requisite arguments. Here’s how you would do that with the `add` function:
int main() {
int sum = add(5, 3);
cout << "The sum is: " << sum;
return 0;
}
When executed, this snippet would output: "The sum is: 8", demonstrating the simple yet effective utility of a helper function.

Common Use Cases for Helper Functions
Performing Calculations
Helper functions shine when handling calculations. For instance, a function that computes the factorial of a number can be defined as follows:
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
This recursive function provides a clean solution to a commonly encountered mathematical problem.
Handling Input and Output
When managing user inputs or outputs, helper functions can significantly streamline the process. For example, formatting output can be simplified with a dedicated helper function:
void displayMessage(const string& message) {
cout << "Message: " << message << endl;
}
Data Processing
Helper functions are essential for data processing tasks, such as finding the maximum value in an array. Consider this example:
int findMax(int arr[], int size) {
int max = arr[0];
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This function iterates through the array, showcasing how helper functions can enhance data manipulation tasks.

Best Practices for Writing Helper Functions
Minimize Side Effects
Avoid functions that modify global variables or maintain state outside their scope. Doing so can lead to unpredictable behavior. Aim for pure functions that produce the same output for a given input without causing side effects.
Keep Functions Small
Small, focused functions are easier to debug and test. As a guiding principle, each helper function should ideally perform one distinct task. This approach leads to cleaner code and simplifies the debugging process.
Use Constants Instead of Magic Numbers
Using constants instead of arbitrary values (or magic numbers) enhances code readability. For example, replacing a hard-coded value with a named constant can clarify the function's intent:
const double PI = 3.14159;
double areaOfCircle(double radius) {
return PI * radius * radius;
}
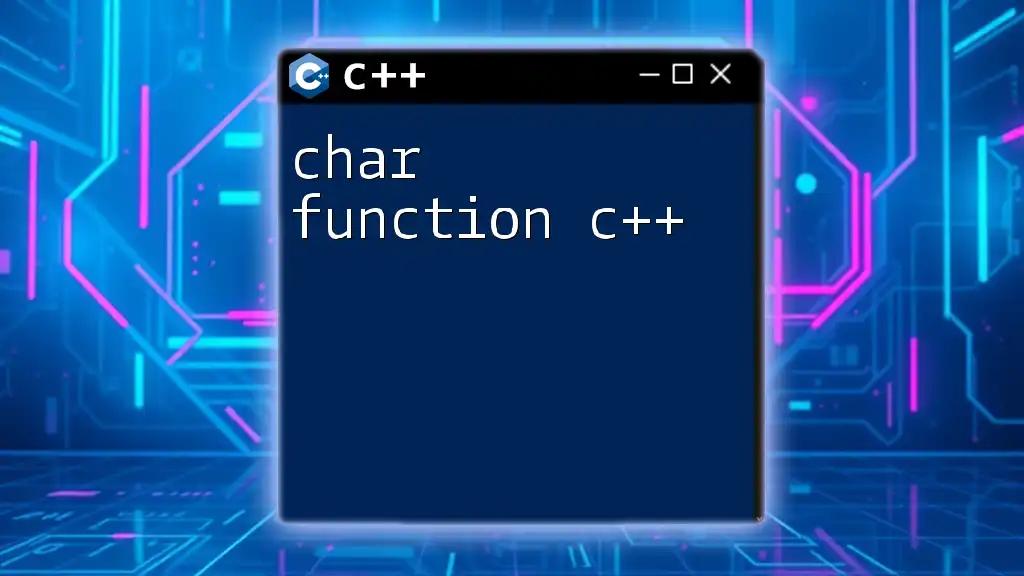
Debugging Helper Functions
Common Issues and Solutions
When developing with helper functions, common pitfalls such as incorrect bit manipulation or inadvertently altering variable values can occur. Implementing print statements to log variable values or revisiting function signatures can be an effective strategy for debugging.
Testing Helper Functions
Testing is crucial for ensuring functionality and reliability. Use simple unit tests to validate behavior. For example, when testing the `add` function, one could write:
assert(add(2, 3) == 5);
assert(add(-1, 1) == 0);
This practice confirms that the helper function behaves as expected.
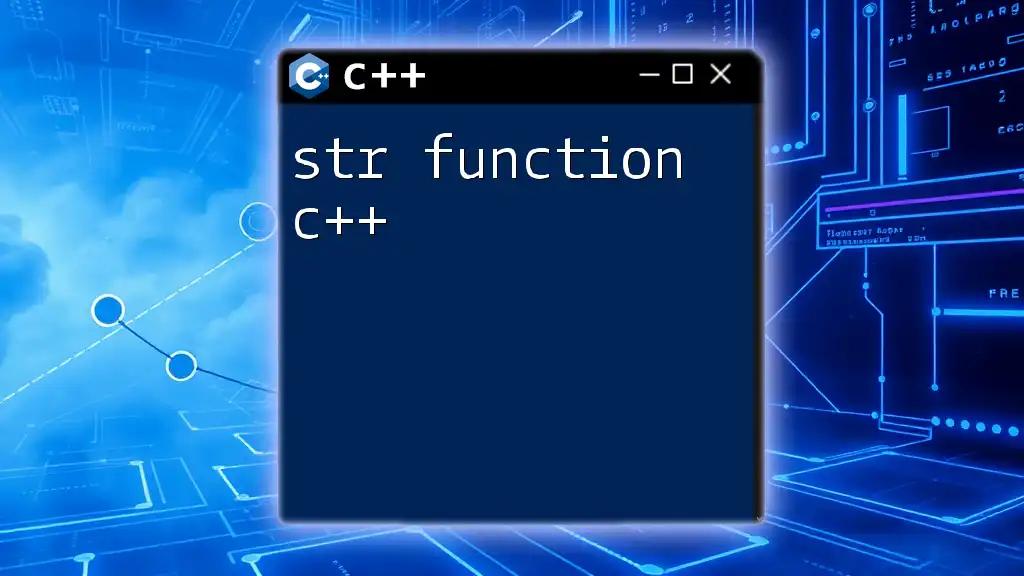
Conclusion: Embracing Helper Functions in C++
Helper functions are a powerful tool in C++ programming. By promoting code organization, enhancing readability, and encouraging code reuse, they significantly contribute to maintaining a clean and efficient codebase. Embracing the use of helper functions can drastically improve your coding experience, guiding you toward more structured and manageable code.
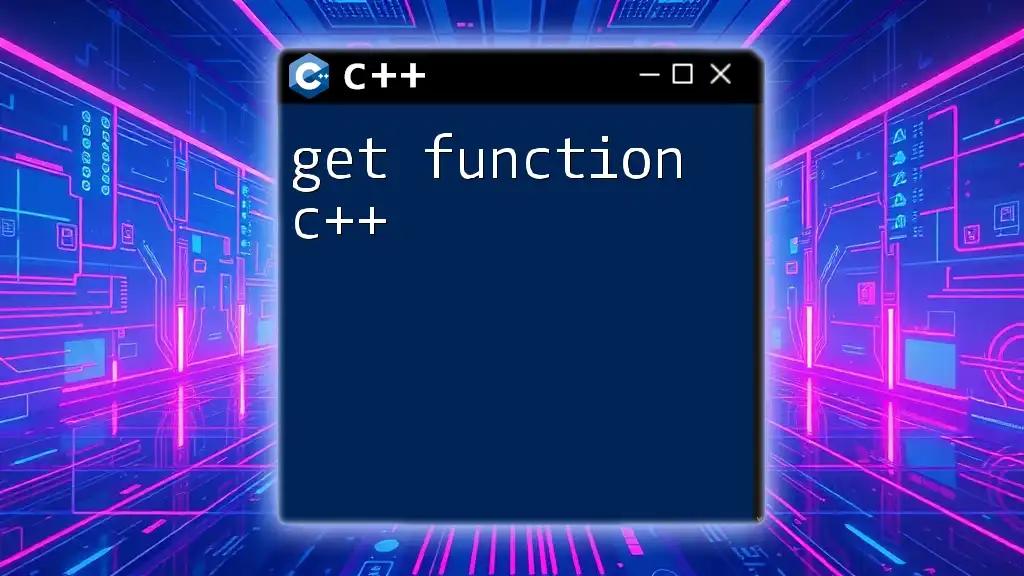
Additional Resources
To further your understanding of C++ and the implementation of effective functions, consider exploring recommended books and online tutorials. Engaging with online communities can also provide valuable insights and support as you navigate your journey in programming.