The `replace` function in C++ replaces occurrences of a specified substring within a string with another substring, as demonstrated in the following code snippet:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
str.replace(7, 5, "C++"); // Replaces "World" with "C++"
std::cout << str; // Output: Hello, C++!
return 0;
}
Understanding the Replace Function in C++
What is the Replace Function?
The replace function in C++ is an essential tool for manipulating strings. This function enables programmers to replace specific portions of a string with another substring. String manipulation is crucial in programming, as it allows you to efficiently modify and manage textual data within your applications.
Overview of String Manipulation in C++
In C++, strings can be represented in two primary forms: C-style strings (character arrays) and std::string objects, which are part of the C++ Standard Library. The std::string provides a higher level of abstraction, making it easier to perform operations like concatenation, comparison, and replacement. The standard library's versatile functionality simplifies string manipulation, allowing developers to focus on logic rather than low-level memory management.

The Syntax of the Replace Function in C++
General Syntax of string.replace()
The syntax for the replace function in C++ is as follows:
std::string& std::string::replace(size_type pos, size_type count, const std::string& str);
This function replaces count characters in the string starting from position pos with the string str. It returns a reference to the modified string.
Key Parameters Explained
- Position (pos): The index where the replacement begins.
- Length (count): The number of characters to be replaced.
- Substring (str): The new string that will replace the specified segment.
Here’s a code snippet demonstrating the parameters in action:
std::string text = "Hello, World!";
text.replace(7, 5, "C++"); // Replaces "World" with "C++"
In this example, starting from index 7, the function replaces five characters ("World") with "C++".

Implementing the Replace Function in C++
Simple Example of Using string.replace()
When using the replace function in C++, a straightforward application might look like this:
std::string phrase = "The quick brown fox.";
phrase.replace(4, 5, "slow"); // Replaces "quick" with "slow"
In this example, the text now reads "The slow brown fox." The essence of utilizing this function lies in its flexibility and ease of use.
Replacing Multiple Occurrences of a Substring
To replace multiple occurrences of a substring, you can employ a loop. For example, if you want to replace all instances of "fox" in a sentence, consider the following approach:
std::string text = "The fox jumps over the lazy fox.";
std::string to_replace = "fox";
std::string replacement = "dog";
size_t pos = 0;
while ((pos = text.find(to_replace, pos)) != std::string::npos) {
text.replace(pos, to_replace.length(), replacement);
pos += replacement.length(); // Move past the replacement
}
In this code, a while loop finds and replaces every instance of "fox" with "dog", transforming the string into "The dog jumps over the lazy dog."

Advanced Usage of The Replace Function
Replacing with Different Data Types
The replace function in C++ can handle both constant character strings (C-style strings) and std::string objects. Note the difference in usage:
std::string str = "Hello World";
str.replace(6, 5, "C++"); // Using std::string
char original[] = "Hello World";
strncpy(original + 6, "C++", 4); // Using C-style string (not using string.replace)
While the first example showcases std::string's capabilities, the second manipulates a C-style string using `strncpy`. While it works, using std::string is generally safer and more manageable.
Case Sensitivity in Replacement
It's crucial to remember that string replacements in C++ are case-sensitive. If you attempt to replace "fox" with another word and the string contains "Fox", it won't work. To address this, a case-insensitive approach can be implemented using `std::transform`:
#include <algorithm>
#include <cctype>
std::string caseInsensitiveReplace(std::string text, const std::string& to_replace, const std::string& replacement) {
std::string::iterator it = std::search(
text.begin(), text.end(),
to_replace.begin(), to_replace.end(),
[](char a, char b) { return tolower(a) == tolower(b); }
);
while (it != text.end()) {
text.replace(it - text.begin(), to_replace.length(), replacement);
it = std::search(it + replacement.length(), text.end(), to_replace.begin(), to_replace.end(),
[](char a, char b) { return tolower(a) == tolower(b); });
}
return text;
}
This function searches for a specified substring in a case-insensitive manner and replaces it wherever it finds a match.

Common Mistakes When Using string.replace()
Off-by-One Errors
A frequent mistake programmers make is off-by-one errors, where they miscalculate the index of the characters. For example:
std::string sentence = "The cat sat on the mat.";
sentence.replace(8, 3, "dog"); // Incorrectly replaces "cat" with "dog"
Here, it replaces "cat" starting from the index for "sat," leading to unexpected results. Ensuring accurate indexes is crucial to avoid such pitfalls.
Forgetting to Handle Different String Lengths
Another common error entails neglecting the length of the strings involved. If a new substring is longer than the substring being replaced, it can lead to runtime errors. For instance:
std::string text = "Hello!";
text.replace(1, 4, "beautiful"); // Attempts to replace "ello" with "beautiful"
This can cause issues, as the resultant string length exceeds the original.

Performance Considerations in Using Replace Function
Time Complexity Analysis
Performance is a relevant factor when dealing with extensive strings. The replace function in C++ operates in linear time complexity O(n), meaning its efficiency may degrade with larger strings. Be mindful of using this function in performance-critical applications.
Best Practices for Large Scale Replacements
When replacing within large datasets, consider the following tips:
- Precompute string lengths to avoid additional computations.
- Use efficient search algorithms to optimize the replacement process.
- Where feasible, minimize the number of replacements in a single pass.
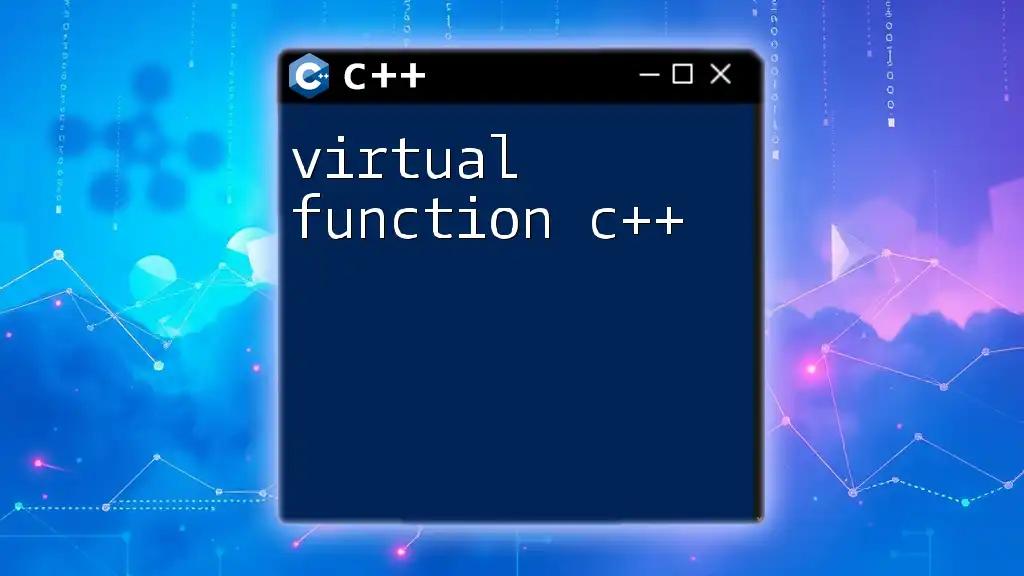
Conclusion: Mastering the Replace Function in C++
In summary, the replace function in C++ is a vital component for anyone working with strings. By understanding its parameters, potential pitfalls, and best practices, developers can effectively manipulate text within their applications.
Encourage practice with real-world examples to fully grasp the intricacies of the replace function and integrate it into your programming skills seamlessly.

Additional Resources
For those looking to delve deeper into C++ string manipulation, consider exploring recommended books, online tutorials, and official documentation for the std::string class.

Acknowledgments
Thank you for your commitment to mastering C++. We encourage you to reach out with any feedback or questions about the replace function in C++!