The `remove` function in C++ is used to delete elements from a container or to remove a file from the file system, depending on the context.
Here's a code snippet demonstrating its usage to remove a file:
#include <iostream>
#include <cstdio> // for remove function
int main() {
const char* filename = "example.txt";
if (remove(filename) != 0) {
perror("Error deleting file");
} else {
std::cout << "File successfully deleted" << std::endl;
}
return 0;
}
Understanding the `remove` Function
What is the `remove` Function?
The `remove` function is a part of the C++ Standard Library, specifically found in the `<algorithm>` header. Its primary purpose is to remove elements that match a specific value from a sequence, effectively repositioning the remaining elements so that they appear at the beginning of the container. This function is especially handy when working with collections of data where you need to purge unwanted items efficiently.
Why Use the `remove` Function?
By utilizing the `remove function c++, you can achieve cleaner and more efficient code without having to manually traverse through containers to eliminate unwanted elements. Whether you're developing an application that handles large datasets or refining smaller collections, `remove` streamlines the process, saving both time and effort.
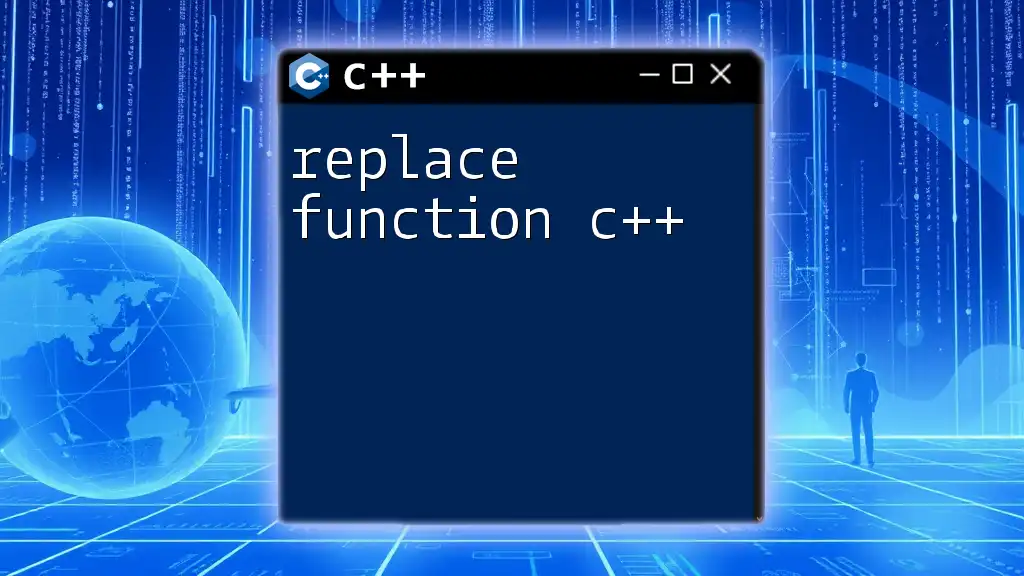
Syntax of the `remove` Function
Basic Syntax
The general syntax of the `remove` function is as follows:
std::remove(iterator first, iterator last, const T& value);
- `first`: This is the starting iterator of the range you want to consider for removal.
- `last`: This is the ending iterator (which is non-inclusive) for the range.
- `value`: The value you wish to eliminate from the given range.
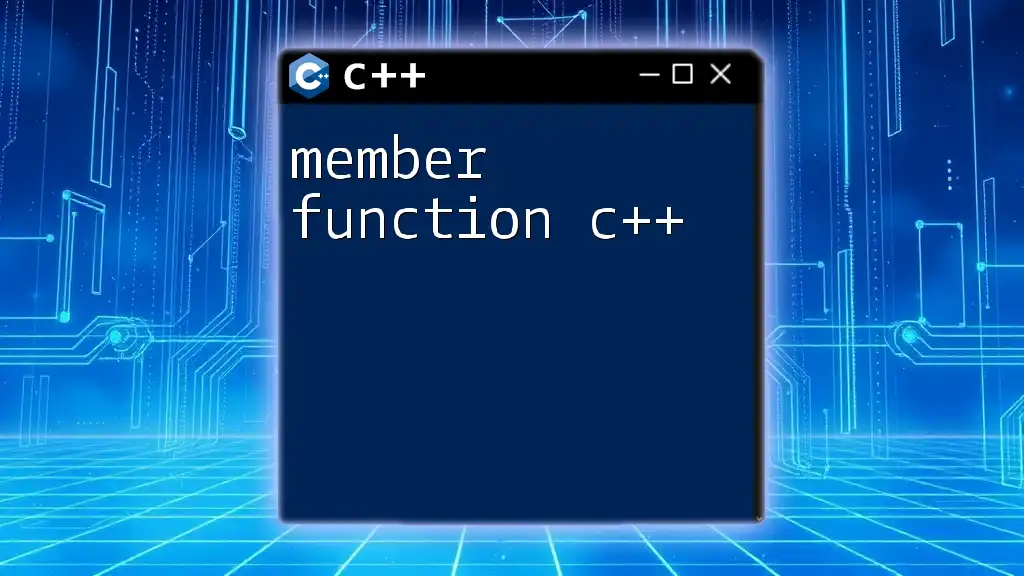
Working with the `remove` Function
How `remove` Works under the Hood
When you invoke the `remove` function c++, it does not directly remove elements from the container. Instead, it shifts all the elements that do not match the specified value to the front of the container and returns an iterator pointing to the new logical end of the range. This means the unwanted elements are still present but are considered "removed" for any operations or iterations performed after the call.
Example: Removing Elements from a Vector
Here’s a practical example that showcases how the `remove` function can be utilized effectively in a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 2, 5};
auto end = std::remove(numbers.begin(), numbers.end(), 2);
numbers.erase(end, numbers.end());
for (int num : numbers) {
std::cout << num << " "; // Output: 1 3 4 5
}
return 0;
}
In this code:
- We start with a vector containing duplicates.
- The `remove` function effectively shifts all elements except `2` to the front.
- The call to `erase` then physically removes the "removed" elements from the container.
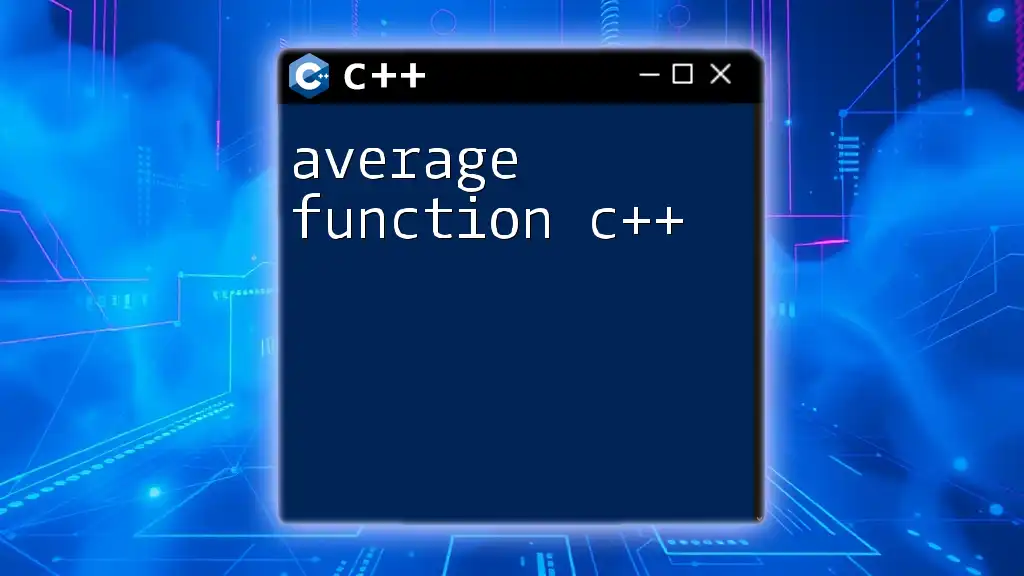
Important Considerations
Understanding the Return Value
A critical aspect of the `remove` function c++ is understanding its return value. The function returns an iterator pointing to the new logical end of the range, which signifies where the valid elements end. Thus, merely using `remove` without the subsequent `erase` does not actually modify the container; instead, it leaves the unwanted elements lingering beyond the new end of the logical sequence.
The Importance of `erase` After `remove`
Since `remove` only repositions the elements, you must follow up with the `erase` function to truly eliminate those unwanted elements. Here’s why it's crucial: without `erase`, your data structure still contains the original values, which can lead to confusion and incorrect results in further operations.
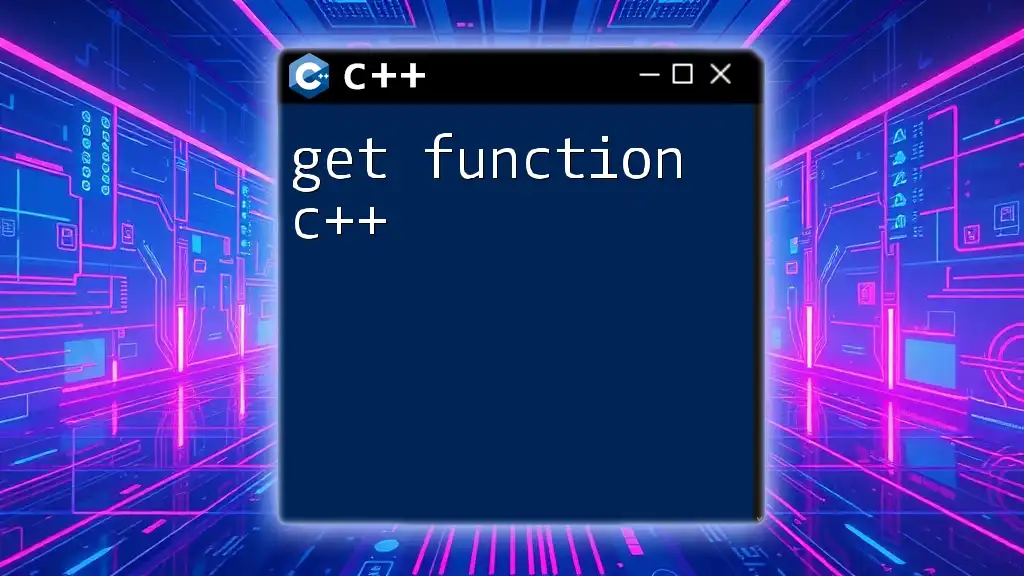
Common Use Cases for `remove`
Removing Duplicates
It's common to face scenarios where you need to remove duplicate values from a collection. The `remove` function helps streamline this process:
std::sort(numbers.begin(), numbers.end());
auto newEnd = std::unique(numbers.begin(), numbers.end());
numbers.erase(newEnd, numbers.end());
In the code above:
- We first sort the vector to bring duplicates next to each other.
- `std::unique` then rearranges the elements, making duplicates consecutive.
- Finally, we utilize `erase` to eliminate the redundant elements.
Conditional Removal
In many situations, you may wish to remove elements based on custom conditions. The `remove_if` function paired with a lambda expression allows you to achieve this seamlessly.
numbers.erase(std::remove_if(numbers.begin(), numbers.end(), [](int num) {
return num % 2 == 0; // Remove even numbers
}), numbers.end());
Here, we remove all even numbers from the vector by incorporating conditional logic directly into the `remove_if` function.
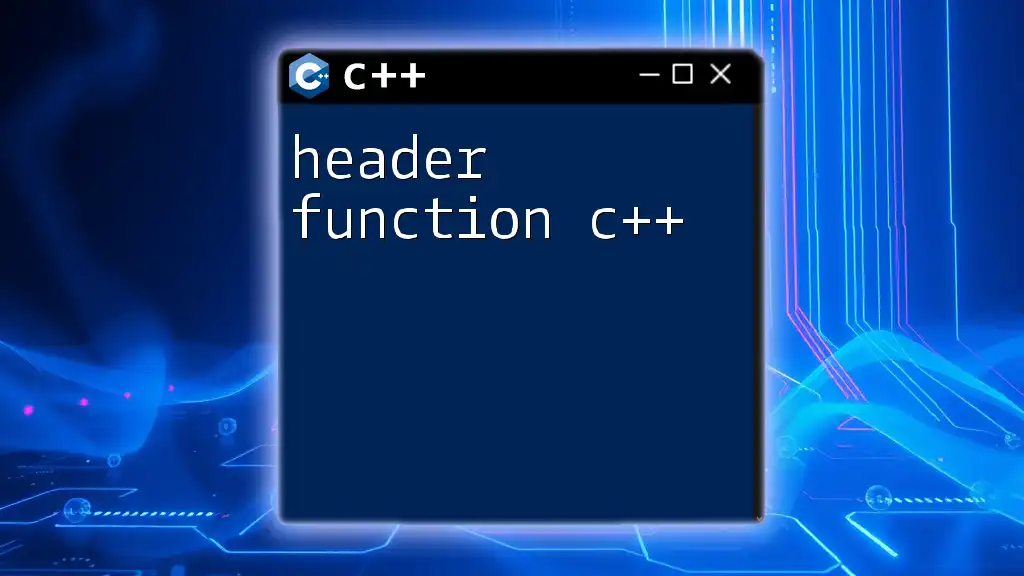
Performance Considerations
Time Complexity
One of the key factors when working with the `remove` function c++ is its time complexity. The function generally operates with a linear time complexity, O(n), as it traverses the entire range. However, do keep in mind that the erase operation also has a linear complexity based on the number of elements being removed.
Memory Management
Proper memory management is essential if you intend to modify collections frequently. Understanding how `remove` shifts data allows you to predict and control memory usage effectively, preventing memory leaks and fragmentation issues.
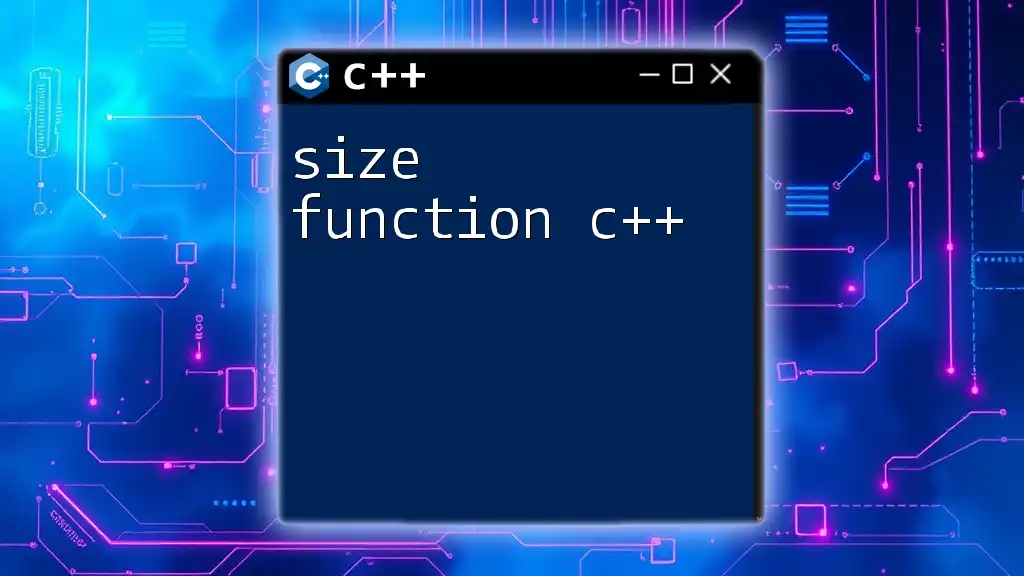
Alternatives to `remove`
Using `std::remove_if` for More Complex Conditions
For scenarios requiring more nuanced removal strategies, `std::remove_if` expands upon the basic functionality of `remove` by enabling condition-based filtering. This offers improved flexibility to cater to a wider range of applications.
Using Other Containers
The behavior of `remove` may vary subtly depending on the container type one utilizes. For instance, `std::list` has its unique characteristics that differentiate its implementation of removal operations, making it essential to grasp how various containers relate to the `remove function c++.
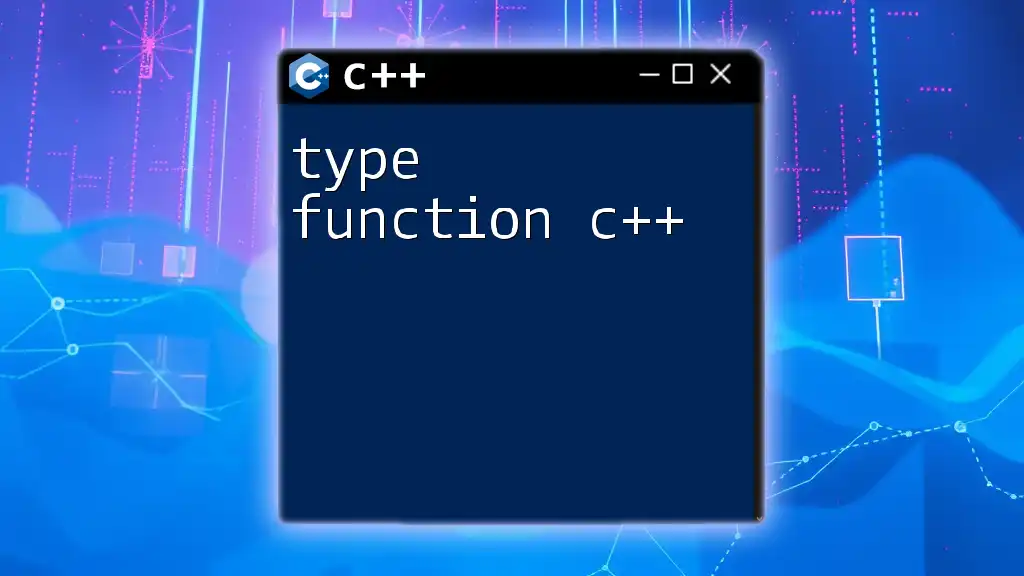
Conclusion
Summarizing Key Points
In conclusion, the `remove function c++ stands out as an efficient tool for eliminating unwanted values from collections. Understanding its syntax, functionality, and nuances empowers you to manipulate data effectively, ensuring cleaner code and improved application performance.
Additional Resources
To deepen your comprehension of the `remove function c++, consider exploring the C++ Standard Library documentation and various online tutorials that provide additional examples and best practices. This will enhance your fluency in applying this function in real-world coding scenarios.