The `std::string` class in C++ serves as a powerful and flexible way to handle strings, providing numerous functions for manipulation, including conversion from other types via the `std::to_string()` function.
Here’s a simple example of using the `std::to_string()` function to convert an integer to a string:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The number as a string: " << strNumber << std::endl;
return 0;
}
Understanding the C++ String Class
What is a String in C++?
In C++, a string is a sequence of characters used to represent text. This can encompass everything from names and phrases to more complex data types. The ability to manipulate strings is crucial in programming as they form the basis of user interactions, data storage, and much more. Understanding how to effectively use strings can significantly enhance the functionality of your applications.
The C++ String Class
C++ provides a powerful string class, `std::string`, which is part of the Standard Library. This class simplifies string operations compared to traditional C-style strings, which are essentially arrays of characters.
Unlike C-style strings, which require manual memory management and can lead to buffer overflows and other issues, `std::string` handles memory allocation automatically and provides a rich set of methods for string manipulation. This allows developers to focus on logic rather than memory management, making C++ much more user-friendly for string operations.
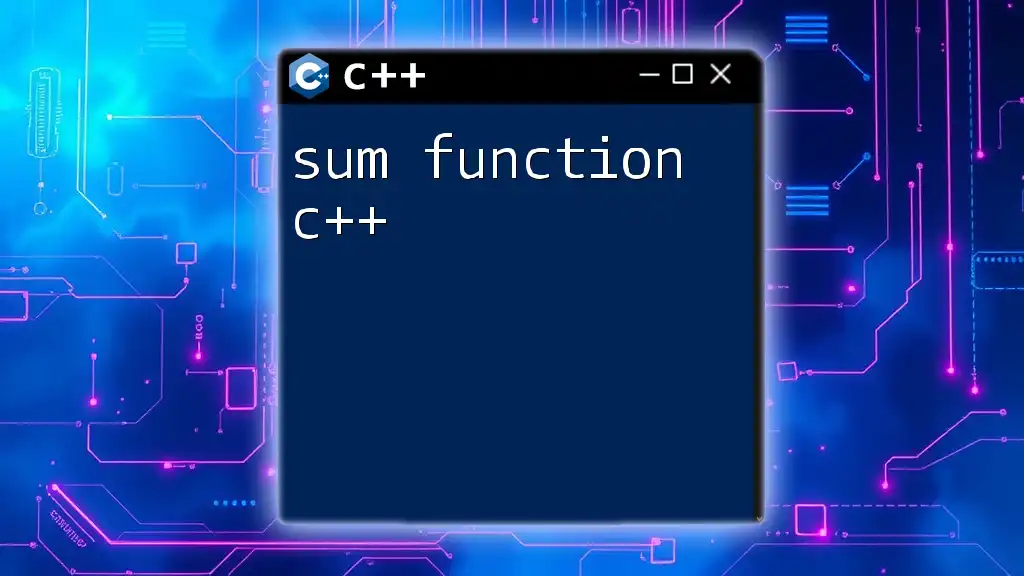
C++ String Functions and Methods
Introduction to C++ String Functions
C++ string functions allow you to perform a wide variety of operations on strings, from measuring their length to completely transforming their content. Mastering these functions is essential for any programmer working with text processing, user input, or even data storage.
Common C++ String Functions
Length and Size Functions
The `length()` and `size()` methods are used to determine the number of characters in a string. Both functions return the same value, so you can use whichever you prefer.
std::string str = "Hello, World!";
std::cout << str.length(); // Output: 13
These functions are fundamental, especially when validating user data or processing text in applications.
String Access Functions
You can access individual characters in a string using the `at()` method or the `operator[]`. The `at()` method checks bounds and throws an exception if the index is out of range, whereas `operator[]` does not.
std::string str = "Hello";
char ch1 = str.at(1); // 'e'
char ch2 = str[1]; // 'e'
Knowing how to access and manipulate string characters is crucial for tasks such as character replacement or pattern matching.
Modifying Strings
C++ provides several methods to modify strings, including `append()`, `insert()`, and `replace()`.
The `append()` method allows you to concatenate another string or characters to the end of an existing string:
std::string str = "Hello";
str.append(", World!"); // "Hello, World!"
The `insert()` method adds a substring at a specified position:
str.insert(5, " Beautiful"); // "Hello Beautiful, World!"
The `replace()` method allows you to replace a part of a string with another substring:
str.replace(6, 9, "CPP"); // "Hello CPP, World!"
Mastering these methods is vital for creating dynamic string content in programs.
Searching and Substring Functions
Finding Substrings with `find()`
The `find()` function is instrumental in searching for a substring within a string. It returns the starting position of the first occurrence of the substring, or `std::string::npos` if not found.
std::string str = "Hello, World!";
size_t pos = str.find("World");
if (pos != std::string::npos) {
std::cout << "Found 'World' at position: " << pos; // Output: 7
}
This function is extensively used in text analysis and data validation scenarios.
Extracting Substrings using `substr()`
To extract a substring from a string, you can use the `substr()` method. This method takes two parameters: the starting index and the length of the substring.
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // "World"
Using `substr()` is particularly helpful when you need specific segments from larger strings, such as whole words or phrases.
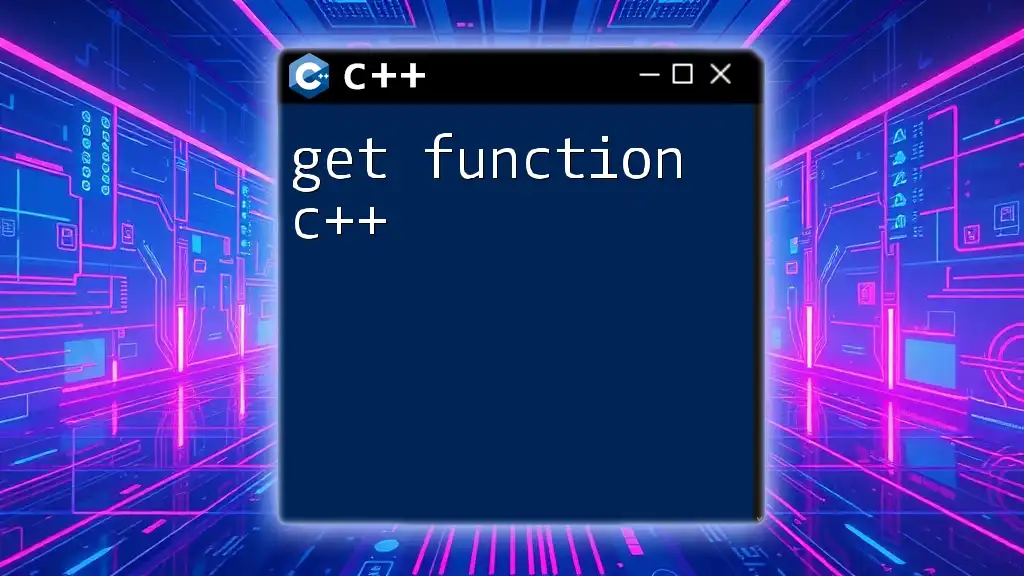
C++ String Operations
Concatenation and Comparison
String Concatenation using `+` Operator
C++ allows you to concatenate strings using the `+` operator, which simplifies combining multiple strings.
std::string str1 = "Hello";
std::string str2 = "World";
std::string combined = str1 + ", " + str2; // "Hello, World"
This operator is intuitive and makes the joining of strings fluid and easy to understand.
Comparing Strings using `compare()`
To compare two strings lexicographically, use the `compare()` method. It returns an integer: less than zero if the first string is less than the second, zero if they are equal, and greater than zero if it's greater.
std::string str1 = "apple";
std::string str2 = "banana";
if (str1.compare(str2) < 0) {
std::cout << "apple is less than banana";
}
Understanding string comparison is essential for sorting data and handling conditional logic based on string values.
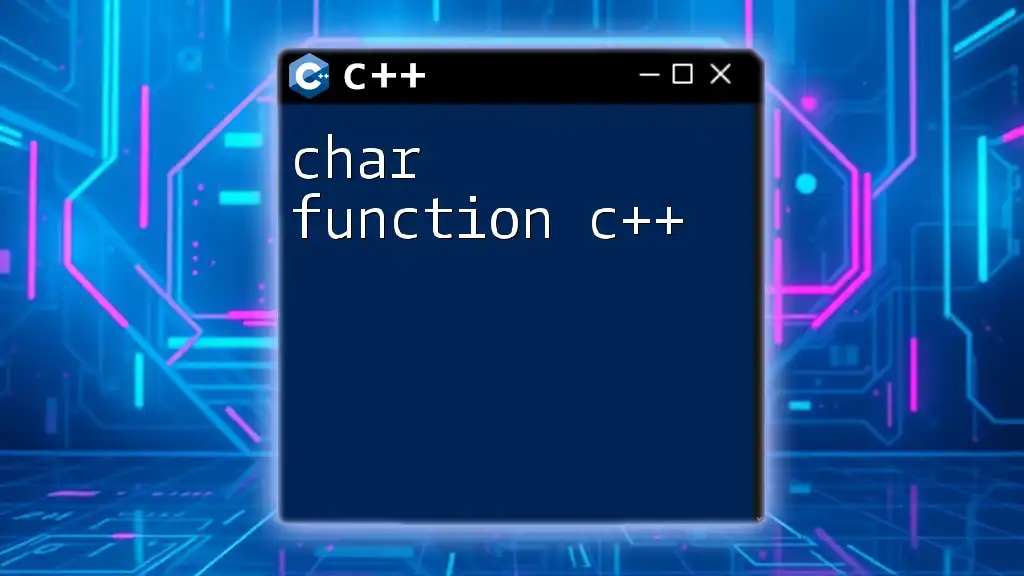
Advanced C++ String Methods
C++ String Class Methods
Conversion Functions
C++ provides several conversion methods within the `std::string` class, primarily `c_str()` and `data()`. These functions convert a C++ string to a C-style string, essential when interfacing with libraries that require character arrays.
std::string str = "Hello";
const char* cStr = str.c_str(); // C-style string
Utilizing these conversion functions enables you to integrate C++ strings with functions expecting C-string arguments effortlessly.
Other Useful String Methods
C++ strings also come with other useful methods like `clear()`, which empties the string, and `empty()`, which checks if the string is empty.
std::string str = " Hello ";
str.clear(); // Clears the string, now str is empty
bool isEmpty = str.empty(); // returns true if string is empty
These functionalities help ensure your string manipulations are handled effectively, allowing for cleaner and more manageable code.
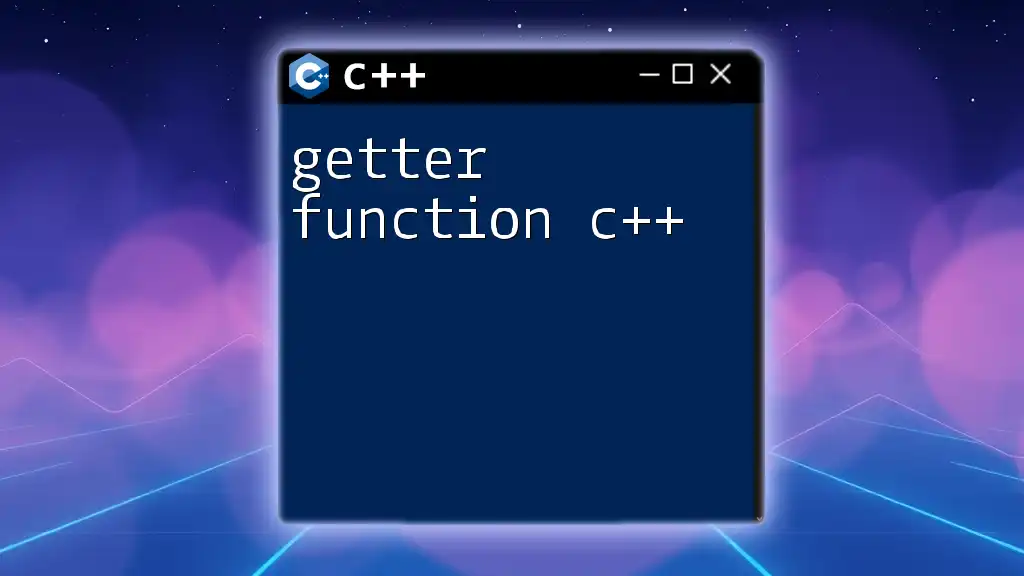
Debugging Common String Issues
Common Pitfalls with Strings in C++
When working with strings, beginners may face challenges such as:
- Incomplete initialization, leading to unexpected behavior.
- Misuse of string methods which can lead to out-of-bound errors.
Tips for Effective String Manipulation
To avoid common pitfalls associated with C++ strings, consider the following best practices:
- Always validate the input before performing operations.
- Prefer using `std::string` over C-style strings to reduce the complexity of memory management.
Additionally, be mindful of performance considerations when manipulating large strings, as excessive copying can lead to inefficiencies.
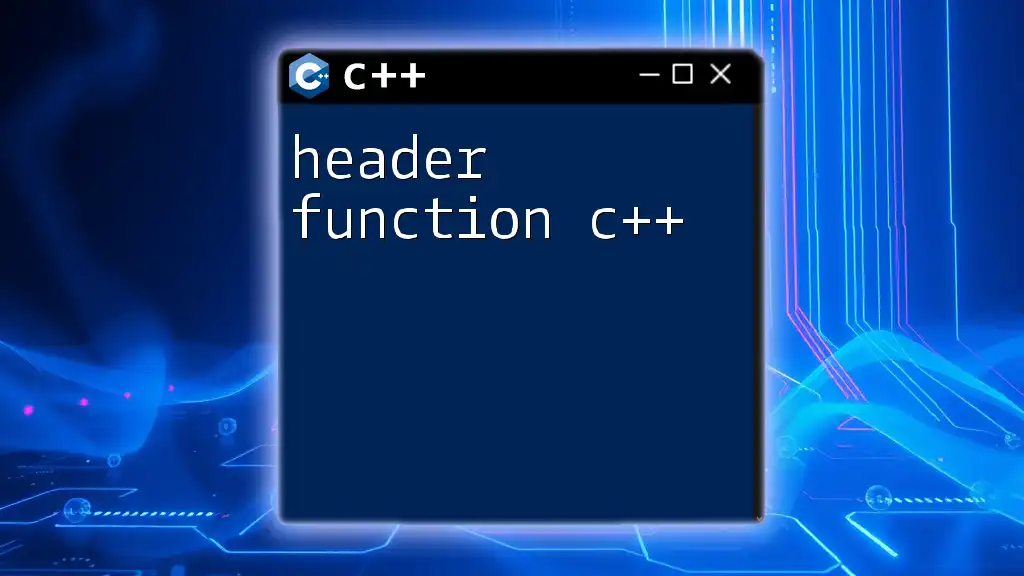
Conclusion
Recap of Key Points
This guide has provided a comprehensive overview of the `str function c++`, detailing the various string functions and methods available in C++. Effective manipulation of strings is a critical skill for C++ programmers, facilitating various applications and user interactions.
Further Learning Resources
For those seeking to deepen their understanding of C++ strings, numerous resources are available, from online courses to programming books. Engaging with these resources will solidify your expertise in string manipulation and enhance your overall programming skills.
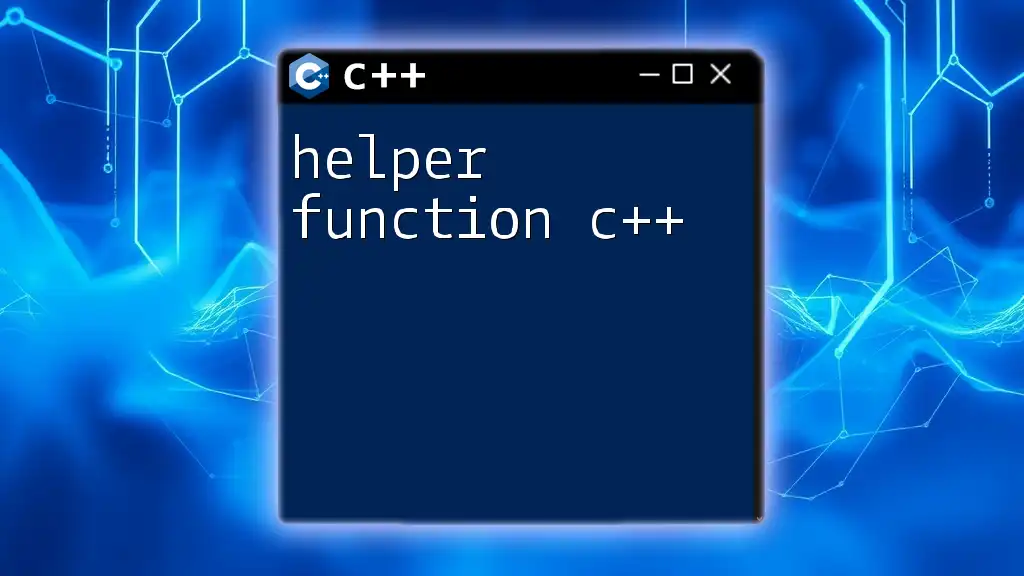
Call to Action
Start practicing these C++ string functions today! Experiment with different examples and create your own mini-projects to solidify your understanding. By continually honing your skills, you will be well-equipped to handle any text-based challenge you encounter in C++.