The `char` function in C++ is used to represent single character data types, which can be manipulated and stored as variables. Here's a code snippet demonstrating how to declare and initialize a `char` variable:
char letter = 'A';
What is a Char in C++?
Definition of Char Data Type
In C++, `char` is one of the fundamental data types used to store a single character. The `char` type is often referred to as a character data type, and it typically occupies 1 byte of memory. Unlike strings, which can hold multiple characters, `char` can hold only a single character like `'A'`, `'1'`, or `'#'`.
It is essential to understand that `char` can hold both letters and digits. However, there is a key difference between storing a number as a character (like `'5'`) versus storing it as an integer (like `5`). This distinction is crucial in programming, as it affects how you manipulate and evaluate these values.
Memory Representation of Char
Each character in C++ is stored using a specific numerical representation, primarily based on the ASCII (American Standard Code for Information Interchange) or Unicode systems. In ASCII, for example, the character `'A'` is represented by the number `65`, while `'a'` is represented by `97`. This numerical representation allows for various character manipulation operations and comparisons.
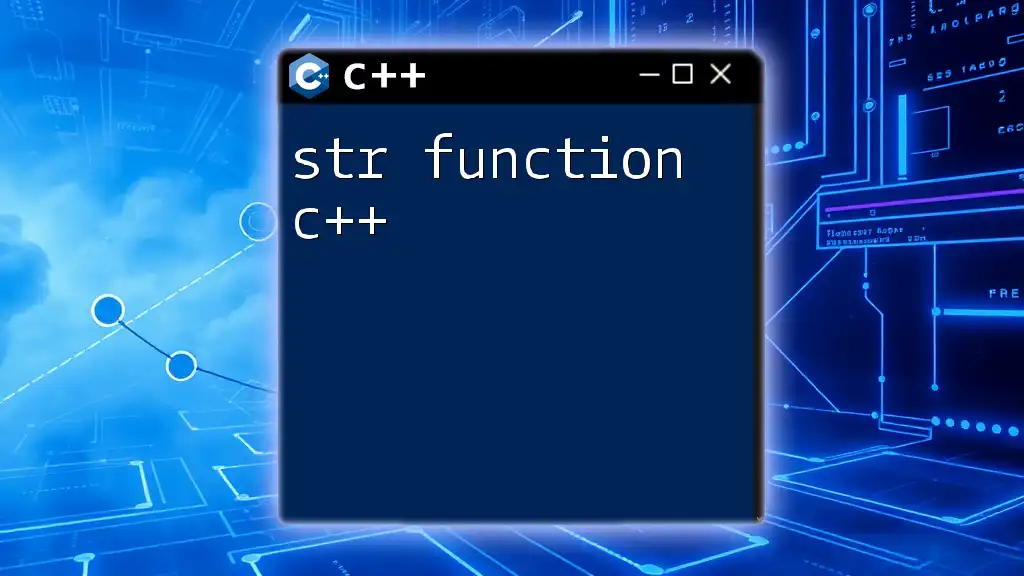
Understanding Char Functions in C++
What is a Char Function?
A char function in C++ is a function designed to operate on `char` data types. These functions are primarily found within the `<cctype>` library and are critical for performing tasks such as checking character types and manipulating character cases. Understanding these functions is essential for efficient character handling in your C++ programs.
Common Char Functions in C++
Several functions are frequently used when working with the `char` data type. Below are some widely adopted functions and their purposes:
Frequently Used Char Functions
isalpha()
The `isalpha()` function checks whether a character is an alphabetic letter. It returns a non-zero value if the character is alphabetic, otherwise, it returns zero.
Example Usage:
#include <cctype>
#include <iostream>
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabet." << std::endl;
} else {
std::cout << ch << " is not an alphabet." << std::endl;
}
isdigit()
Similar to `isalpha()`, the `isdigit()` function checks for numeric digits. If the character is a digit, it returns a non-zero value; otherwise, it returns zero.
Example Usage:
#include <cctype>
#include <iostream>
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a digit." << std::endl;
} else {
std::cout << ch << " is not a digit." << std::endl;
}
tolower() and toupper()
These functions are pivotal for case manipulation. `tolower()` converts a character to lowercase, while `toupper()` converts it to uppercase.
Example Usage:
#include <cctype>
#include <iostream>
char ch = 'D';
std::cout << "Lowercase: " << (char)tolower(ch) << std::endl; // Output: d
std::cout << "Uppercase: " << (char)toupper(ch) << std::endl; // Output: D
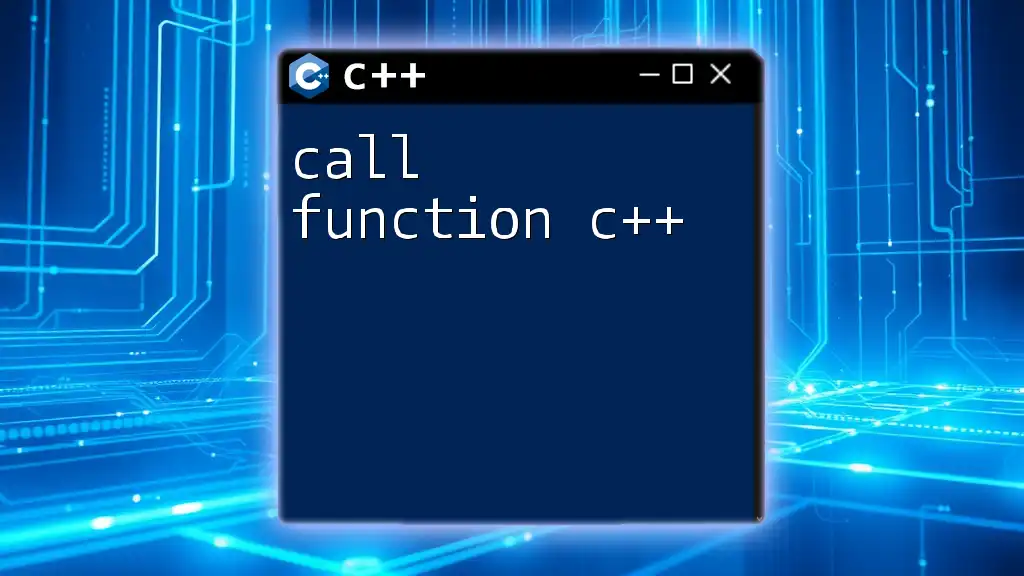
Advanced Char Functions and Techniques
Combining Char Functions
Proficiently utilizing char functions can lead to more robust programs. For instance, you can combine `isalpha()` and `tolower()` to filter input and convert letters to lowercase simultaneously.
Example of Combining Functions:
#include <cctype>
#include <iostream>
#include <string>
std::string input = "AbcD123";
for (char c : input) {
if (isalpha(c)) {
std::cout << tolower(c); // Outputs: abcd
}
}
Char Function Performance Considerations
Understanding the performance implications of char functions can help you write efficient code. While most char functions execute quickly, using them in tight loops over large datasets could lead to performance bottlenecks.
Best Practices:
- Always validate inputs with functions like `isalpha()` or `isdigit()` before processing them further.
- Use char functions selectively based on the need for clarity and performance.
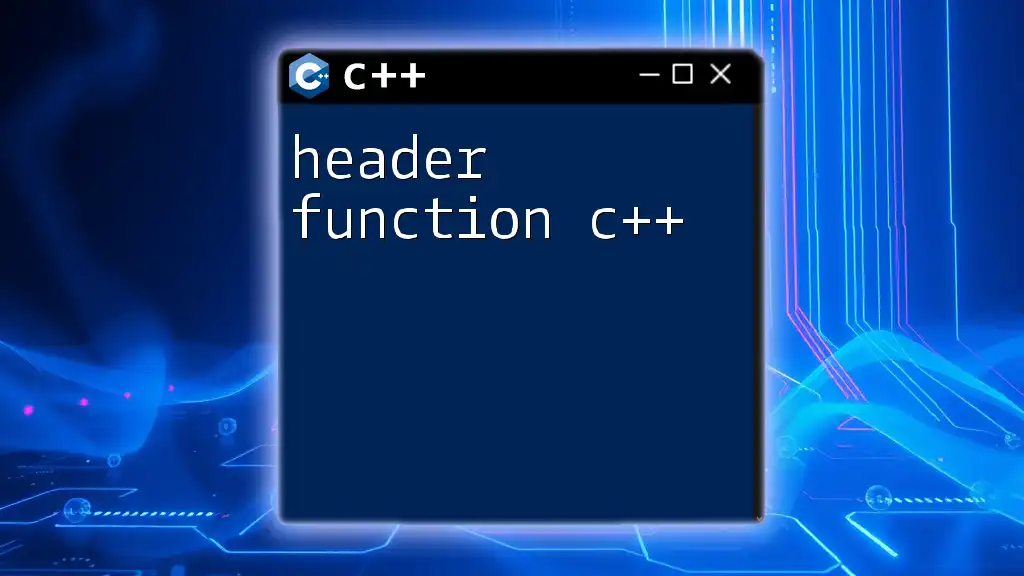
Practical Examples and Use Cases
User Input Validation
When developing applications, input validation is crucial for ensuring data integrity. Char functions like `isalpha()` and `isdigit()` can validate user inputs effectively.
Example of Input Validation:
#include <cctype>
#include <iostream>
char ch;
std::cout << "Enter a character: ";
std::cin >> ch;
if (isalpha(ch)) {
std::cout << ch << " is a valid letter." << std::endl;
} else if (isdigit(ch)) {
std::cout << ch << " is a valid digit." << std::endl;
} else {
std::cout << ch << " is not valid." << std::endl;
}
String Manipulation Techniques
Transforming Strings
Using char functions, you can efficiently transform strings from uppercase to lowercase or vice versa.
Example of String Transformation:
#include <cctype>
#include <iostream>
#include <string>
std::string str = "Hello World!";
for (char &c : str) {
c = tolower(c); // Transforms all characters to lowercase
}
std::cout << str << std::endl; // Output: hello world!
Reversing a String
Char functions also come handy when manipulating strings, such as reversing them character-by-character.
Example of Reversing a String:
#include <iostream>
#include <string>
std::string str = "C++ Programming";
std::string reversed = "";
for (int i = str.length() - 1; i >= 0; --i) {
reversed += str[i]; // Append characters in reverse order
}
std::cout << reversed << std::endl; // Output:gnimmargorP ++C
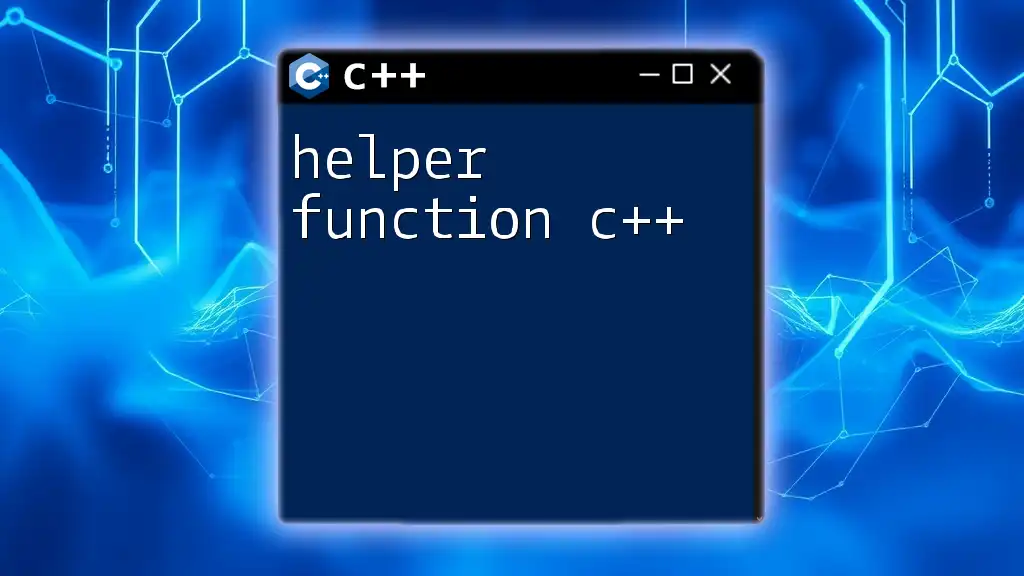
Conclusion
In this comprehensive guide on the char function in C++, we explored the fundamentals of the `char` data type, common char functions, and various practical applications. Mastering these char functions is essential for any programmer who aims to enhance their skills in C++. We encourage you to practice with the provided examples and explore further to solidify your understanding of character manipulation in C++.
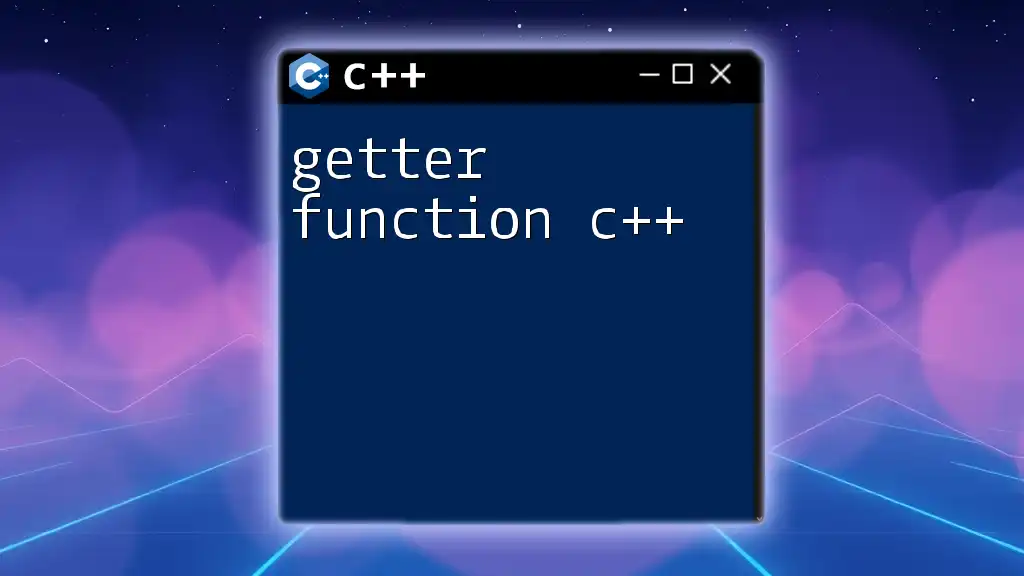
Additional Resources
Book Recommendations
For further learning about C++, consider these foundational texts that cover character manipulation and data types extensively.
Online Courses
We also recommend several online courses focused on C++ programming, which include lessons on char functions.
Practice Platforms
To develop your skills, utilize multiple online platforms that offer coding challenges and exercises specifically for practicing C++ char functions.
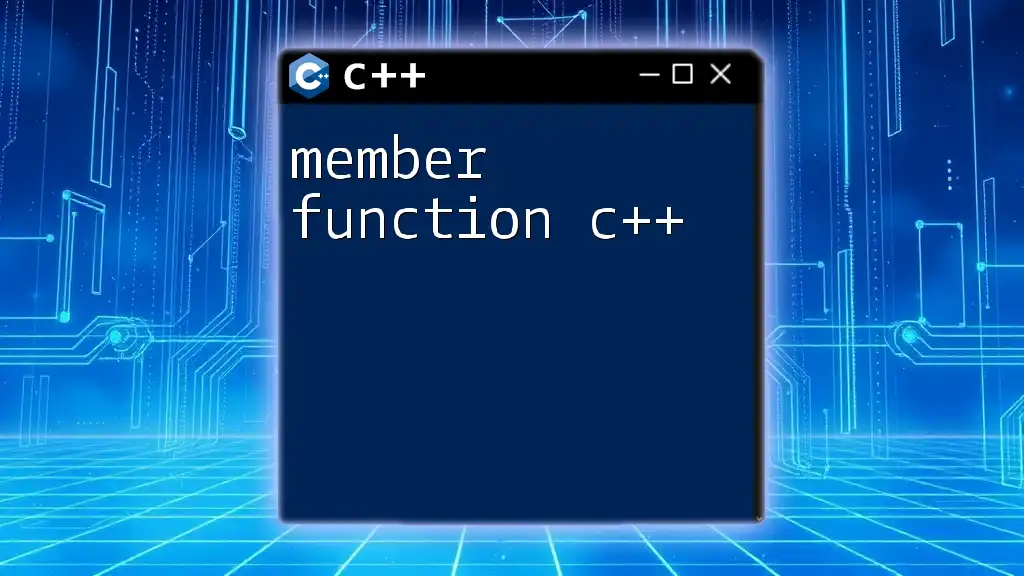
FAQs
Throughout this article, we've tackled various aspects of char functions. Below are some commonly asked questions that can help clarify any uncertainties you may have about using char in C++.