A virtual function in C++ allows derived classes to override a base class function, enabling polymorphic behavior and ensuring the correct function is called for an object, regardless of the type of reference or pointer used for the object.
#include <iostream>
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class show function called." << std::endl;
}
};
int main() {
Base* b = new Derived();
b->show(); // Calls Derived class show()
delete b;
return 0;
}
Understanding Virtual Functions
Definition of Virtual Function
In C++, a virtual function is a member function in a base class that you expect to override in derived classes. When you use a virtual function, you enable the C++ runtime to use a mechanism called dynamic binding (or late binding), allowing the program to decide at runtime which function to invoke based on the type of the object pointed to or referenced rather than the type of the pointer or reference.
The basic syntax for declaring a virtual function in a class is:
class Base {
public:
virtual void functionName() {
// Default implementation
}
};
How Virtual Functions Work
When a class declares a virtual function, the compiler creates a vtable (virtual method table) for that class. Each object of a class containing virtual functions has a pointer to this vtable, commonly referred to as vptr. When you call a virtual function through a base class pointer or reference, the runtime checks the vtable to determine which derived class function should actually be executed.
This mechanism allows for dynamic dispatch, meaning that even when using a base class pointer, the derived class version of the function is invoked if it has been overridden.
Here’s a simple code example demonstrating this dynamic binding:
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class show function called." << std::endl;
}
};
int main() {
Base* b = new Derived();
b->show(); // Output: Derived class show function called.
delete b;
}
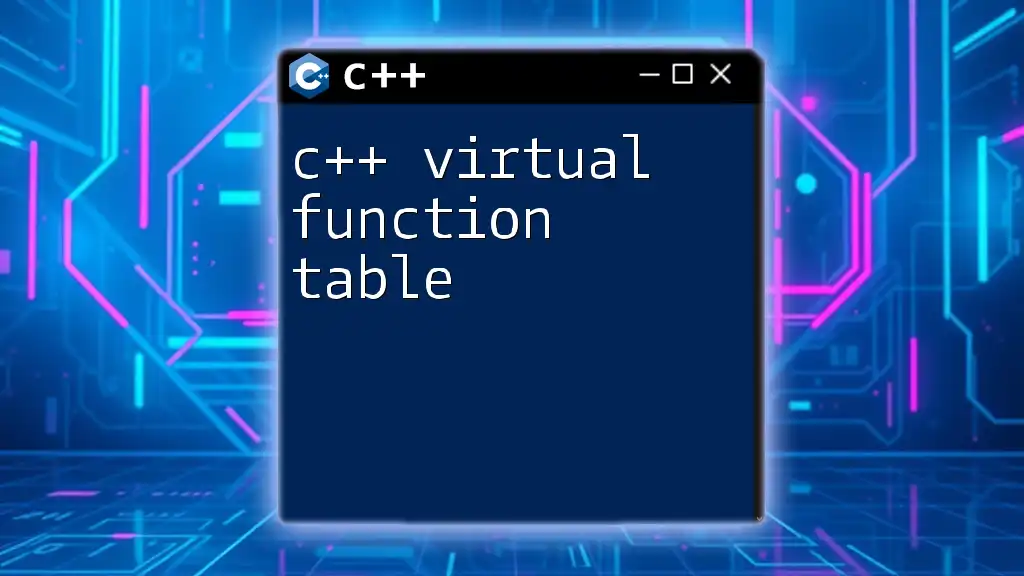
Creating and Using Virtual Functions
Basic Example of Virtual Functions
To illustrate the usage of virtual functions, let’s create a simple inheritance hierarchy where a base class has a virtual function that is overridden in a derived class.
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class show function called." << std::endl;
}
};
In this example, calling the `show` function on a pointer or reference of type `Base` will lead to the derived class's implementation being executed, thanks to the virtual function mechanism.
Calling Virtual Functions
When invoking virtual functions, the derived class's method is executed even when accessed via a base class pointer. This is one of the main reasons virtual functions are so powerful in achieving polymorphic behavior.
int main() {
Base* basePointer;
Derived derivedObject;
basePointer = &derivedObject;
basePointer->show(); // Output: Derived class show function called.
}
This illustrates how polymorphism allows a program to process objects of different classes through a common interface, enhancing flexibility and scalability.
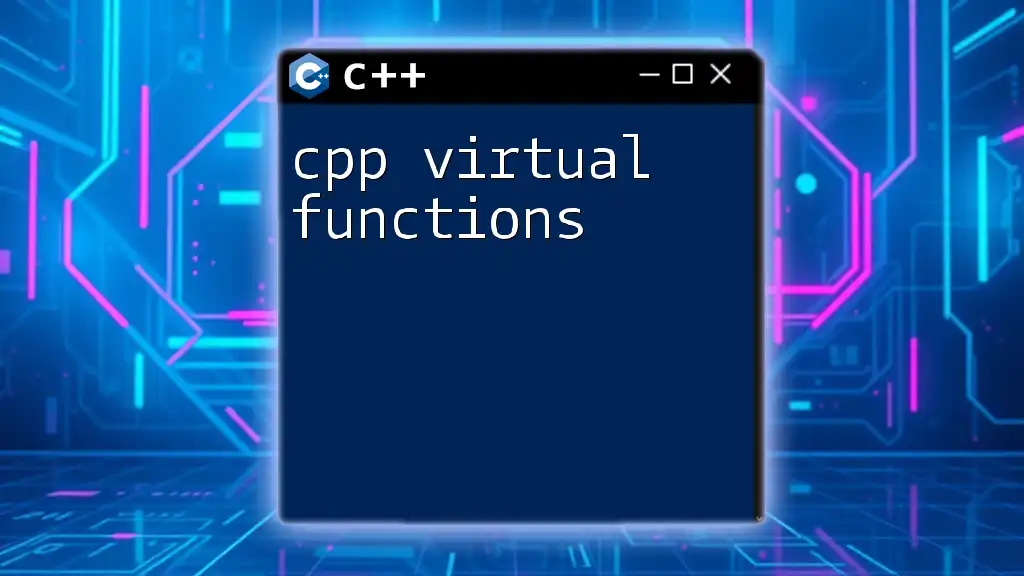
Characteristics of Virtual Functions
Declaration
In addition to being declared as virtual, derived functions that are meant to override a base class’s virtual function can use the `override` keyword. This helps the compiler catch mistakes if the function signature does not match.
class Base {
public:
virtual void display() {
std::cout << "Base display function." << std::endl;
}
};
class Derived : public Base {
public:
void display() override { // Correctly overriding
std::cout << "Derived display function." << std::endl;
}
};
Virtual Destructor
It is crucial to declare destructors as virtual in base classes that will be inherited from. Failing to do so can lead to resource leaks since the derived class's destructor will not be called when an object is deleted through a base class pointer.
class Base {
public:
virtual ~Base() {
std::cout << "Base destructor called." << std::endl;
}
};
class Derived : public Base {
public:
~Derived() {
std::cout << "Derived destructor called." << std::endl;
}
};
In this case, deleting an object of `Derived` through a `Base` pointer will ensure both the `Derived` and `Base` destructors are called, avoiding resource leaks and ensuring proper cleanup.
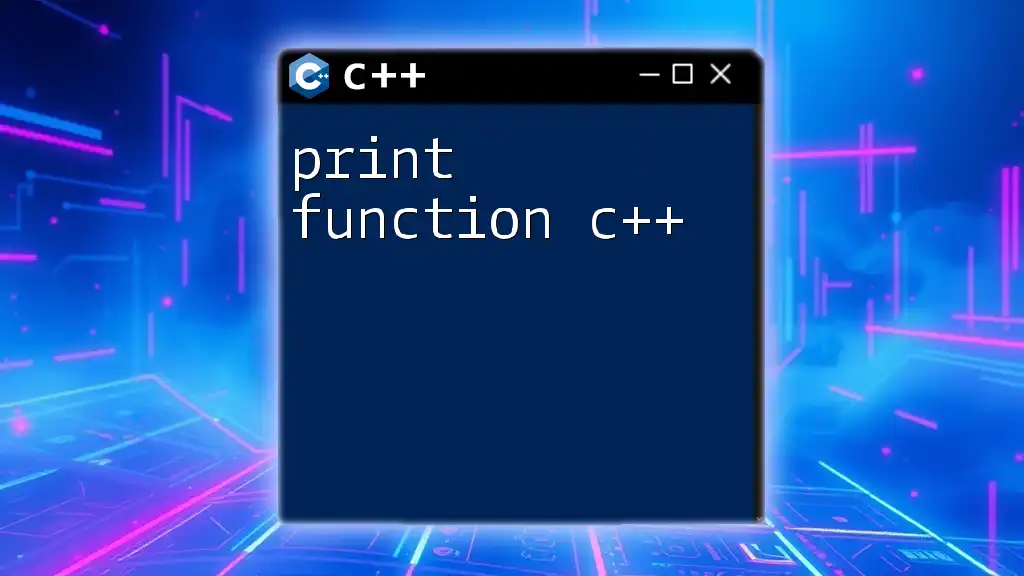
Common Challenges and Solutions
Diamond Problem
One common challenge with inheritance in C++ is the diamond problem, which occurs in cases of multiple inheritance. This happens when two base classes inherit from the same parent class, and a derived class inherits from both of the base classes.
For instance:
class A {};
class B : public A {};
class C : public A {};
class D : public B, public C {}; // Diamond shape
Without a proper mechanism, the compiler cannot determine whether to use the base class `A`’s instance once or twice. To solve this issue, C++ provides virtual inheritance, allowing you to specify that the base class should only be instantiated once.
class A {};
class B : virtual public A {};
class C : virtual public A {};
class D : public B, public C {}; // No ambiguity now
Performance Considerations
While virtual functions offer great flexibility and polymorphism, they do incur some overhead due to the additional lookup required to access the correct function. It's important to consider this trade-off, especially in performance-sensitive applications. In situations where polymorphism isn’t required, it's generally better to use non-virtual functions.
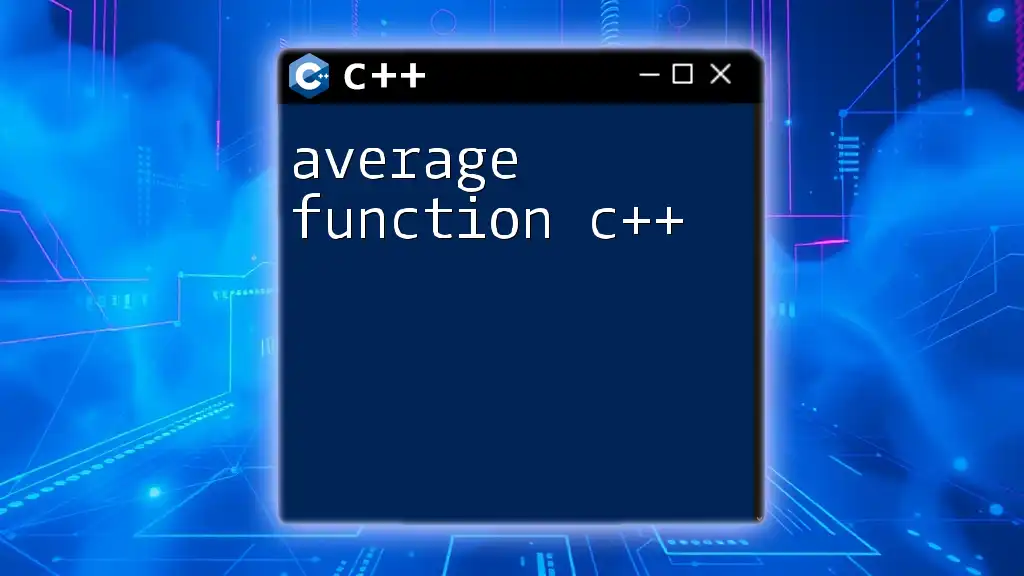
Best Practices for Using Virtual Functions
When to Use Virtual Functions
Virtual functions are best used when you anticipate that derived classes will provide their own version of a function. They facilitate code extensibility and maintainability, reducing the need for scattered changes across various parts of the codebase.
Alternative Approaches
Besides virtual functions, C++ also supports static polymorphism through templates and function overloading. You may also consider using pure virtual functions for defining interfaces in C++. A pure virtual function is declared by assigning `0`, indicating that the function must be overridden in derived classes.
class AbstractBase {
public:
virtual void pureVirtualFunc() = 0; // Pure virtual function
};
This forces any derived class to provide its implementation, enforcing a contract of functionality.
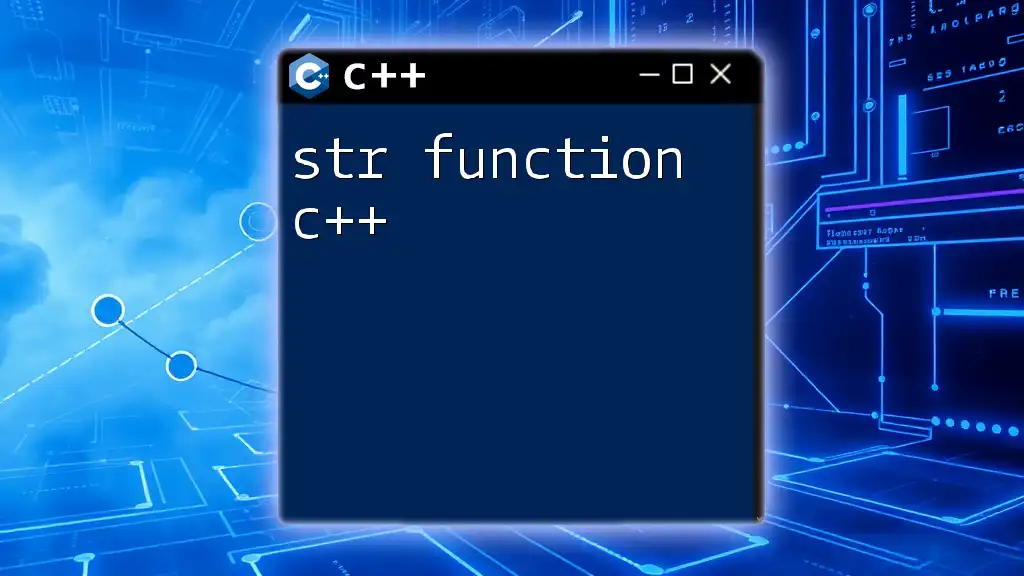
Conclusion
Understanding virtual functions in C++ is crucial for harnessing the power of polymorphism in your applications. They enable you to write flexible, reusable code and ensure that the correct version of a function is executed at runtime. Remember to utilize virtual destructors in base classes and be mindful of performance implications when using them. As you deepen your understanding of virtual functions, you will find them to be an indispensable tool in your C++ programming arsenal.
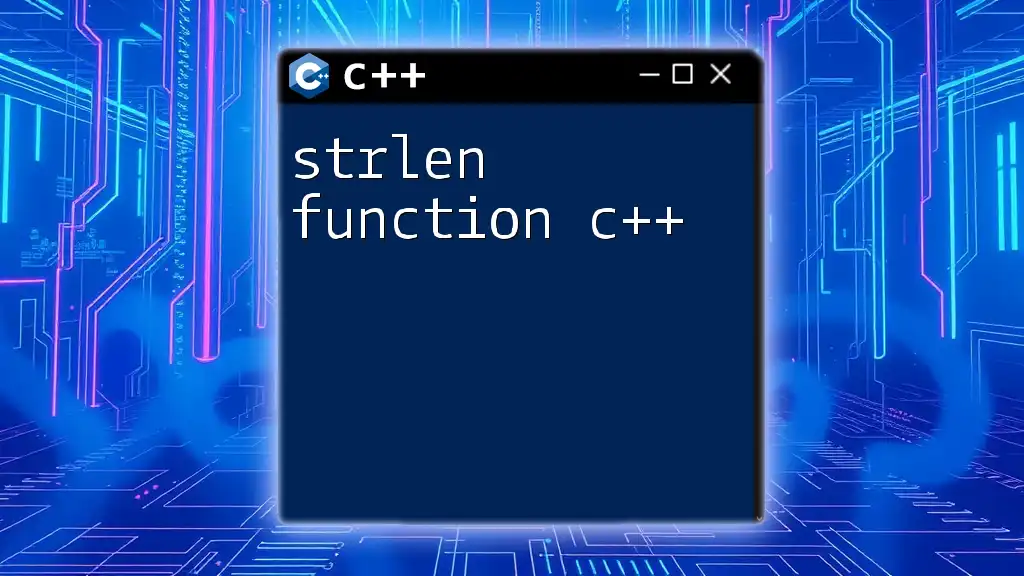
Additional Resources
For readers looking to further their knowledge on the topic, consider exploring select books, online courses, and tutorials designed to enhance your C++ skills. Additionally, hands-on coding practices such as contributing to GitHub repositories can provide beneficial real-world experience.
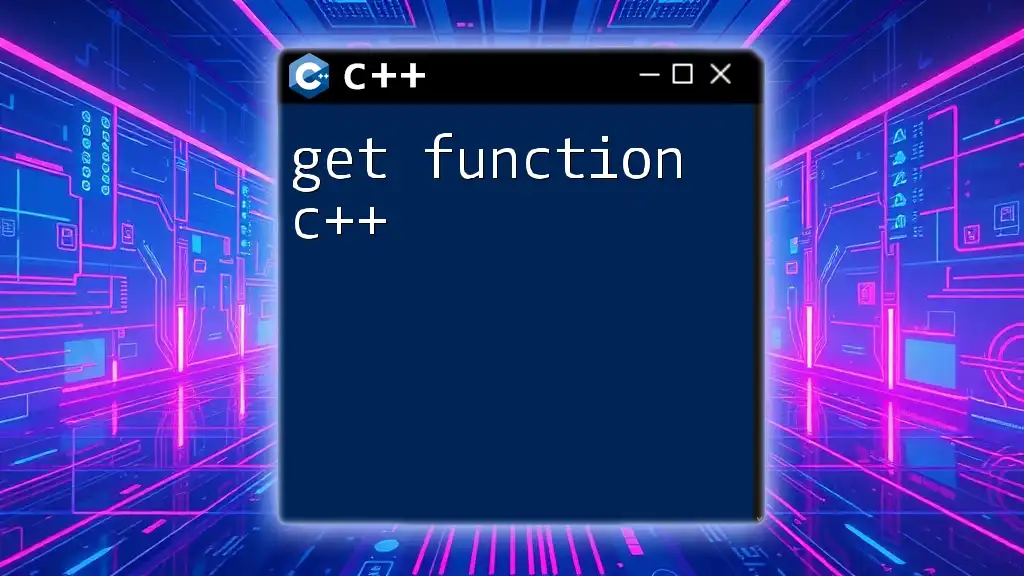
Call to Action
Have you had experiences with virtual functions in your coding journey? Share your thoughts and insights in the comments below. Alternatively, consider signing up for our lessons on advanced C++ topics to expand your proficiency even further!