The `size()` function in C++ is used to obtain the number of elements in a container, such as a vector or string.
Here's an example using the `size()` function with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Size of vector: " << numbers.size() << std::endl;
return 0;
}
What is the Size Function?
The size function in C++ serves as an essential tool for developers, allowing them to determine the amount of space occupied by variables, data types, and containers. This function plays a crucial role in memory management and data structure operations by providing crucial insights about how much data is stored, which in turn helps optimize resource usage and debug programs more effectively.
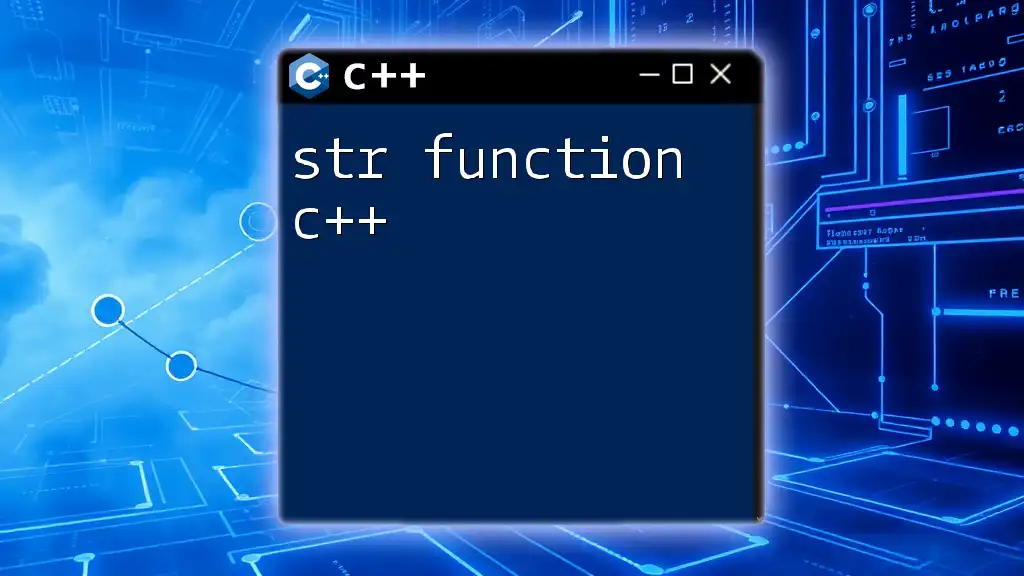
Why is Understanding Size Functions Important?
Understanding the size function in C++ is vital for several reasons:
- It aids in memory management, allowing developers to allocate only the necessary amount of memory for their programs.
- Knowledge of the actual size of data structures is essential for creating efficient algorithms, thus helping in both performance tuning and debugging.
- In real-world applications, efficient memory usage can lead to more scalable and reliable software.
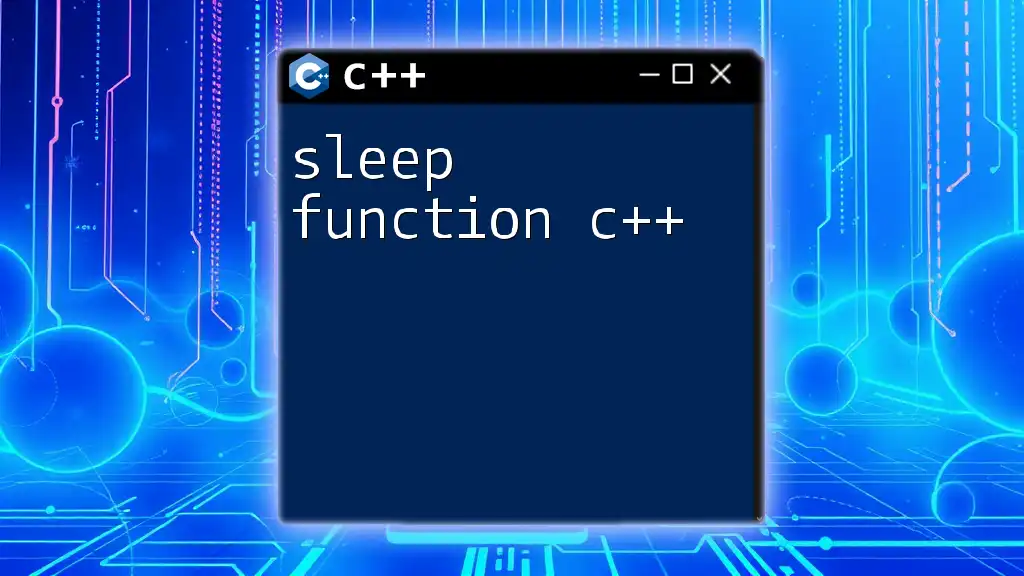
The Basics of the Size Function in C++
What Does the Size Function Do?
The size function is primarily designed to retrieve the size of a data type or data structure in bytes. This functionality extends across various contexts, including basic data types, arrays, and standard template library (STL) containers, among others.
Syntax of the Size Function
The syntax for determining the size varies slightly based on what you’re working with. Some of the general syntaxes include:
- For built-in data types:
sizeof(data_type)
- For STL containers like vectors and strings:
container.size()
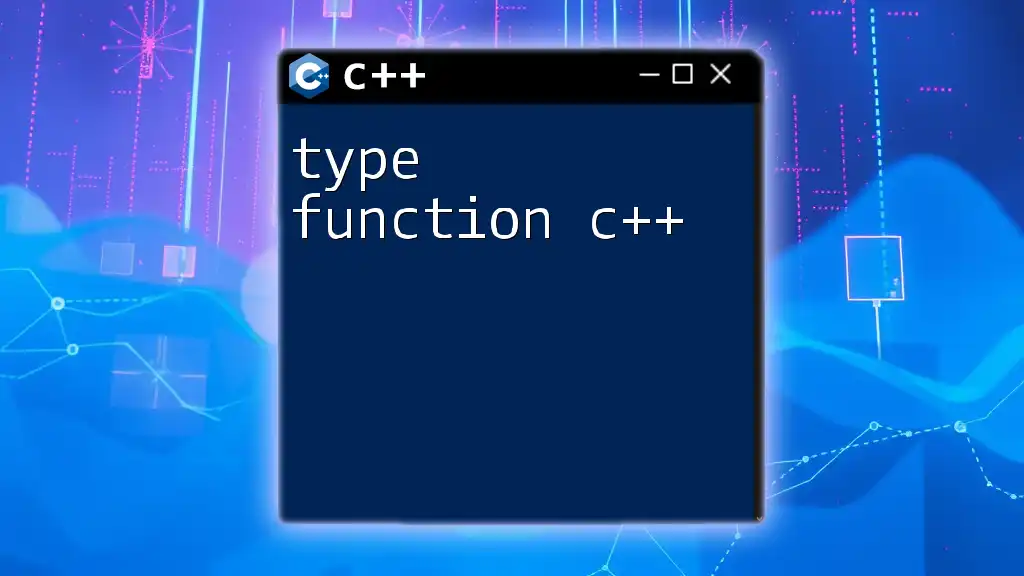
Using Size Function with Basic Data Types
Size of Fundamental Data Types
When dealing with fundamental data types, the size function can help unearth information about the memory footprint of variables. For example:
int a;
std::cout << "Size of int: " << sizeof(a) << " bytes." << std::endl;
This line of code retrieves the size of the `int` type, which is typically 4 bytes on most platforms.
Size of User-Defined Types
Understanding the size of user-defined types like structs and classes is crucial, especially when you're developing systems where memory consumption is a concern. Here’s an example:
struct Node {
int data;
Node* next;
};
std::cout << "Size of Node: " << sizeof(Node) << " bytes." << std::endl;
This code snippet illustrates how to calculate the size of a user-defined structure, which is essential when implementing linked lists or other data structures.
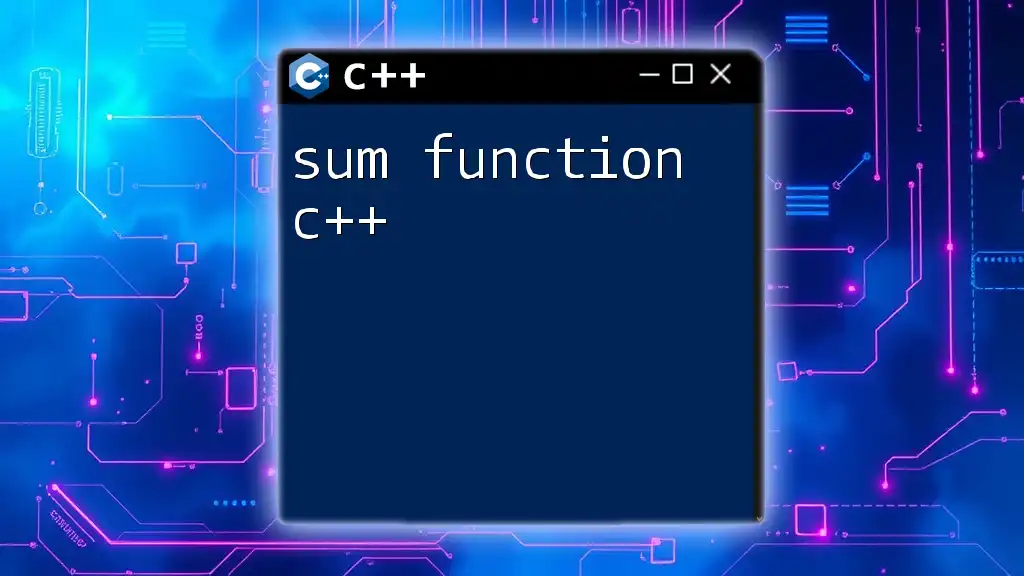
The Size Function with Standard Library Containers
Size Function with Arrays
When working with arrays, determining the size can be tricky due to how pointers operate. A common method to find the size of an array is shown below:
int arr[10];
std::cout << "Size of array: " << sizeof(arr) / sizeof(arr[0]) << std::endl;
This example shows how to divide the total byte size of the array by the byte size of a single element to find the total number of elements.
Size Function with `std::vector`
The `std::vector` class from the C++ Standard Library provides a simple way to find the number of elements contained:
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Size of vector: " << vec.size() << std::endl;
Using `vec.size()` allows developers to know exactly how many items are in the vector without needing to perform any additional calculations.
Size Function with `std::string`
In C++, strings are first-class citizens with their methods for size retrieval:
std::string str = "Hello, World!";
std::cout << "Size of string: " << str.size() << std::endl;
This code extracts the size of the string variable, which includes the length of the string without the null-terminating character.
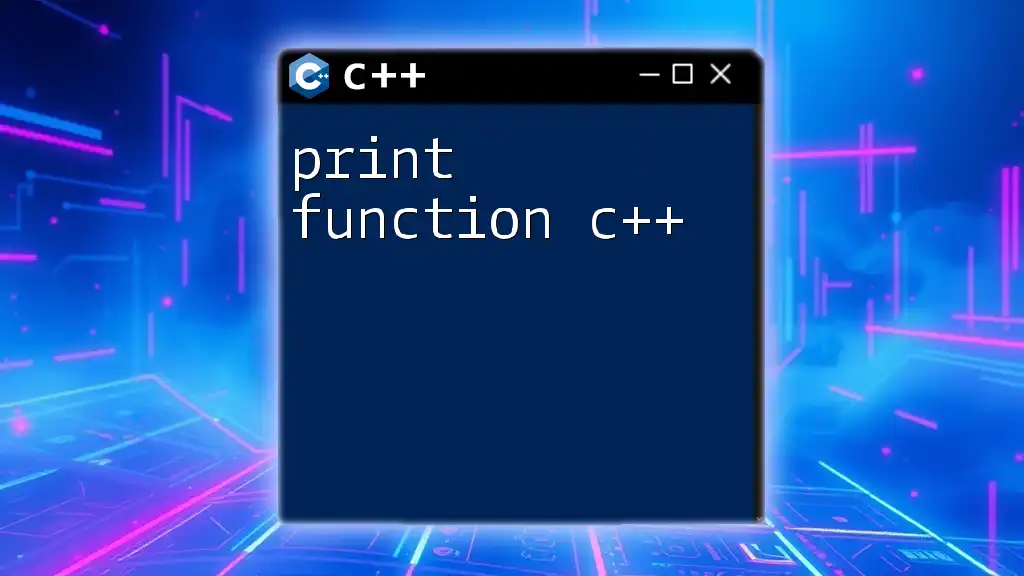
Advanced Usage of Size Functions
Dynamic vs Static Sizing
It's important to differentiate between dynamic and static sizes. Static sizes are determined at compile time, while dynamic sizes may change during the program's execution. For instance, the size of an array defined as `int arr[10]` is static and does not change. In contrast, a `std::vector` can resize itself, so its size is inherently dynamic.
Understanding the Limitations of Size Functions
While the size function provides useful information, it's crucial to understand its limitations. The size returned by `sizeof` for a pointer does not necessarily reflect the amount of memory allocated at runtime. For instance, when using dynamic memory allocation with `new`, the size calculation may lead you to patterns that cause memory leaks if not properly managed.
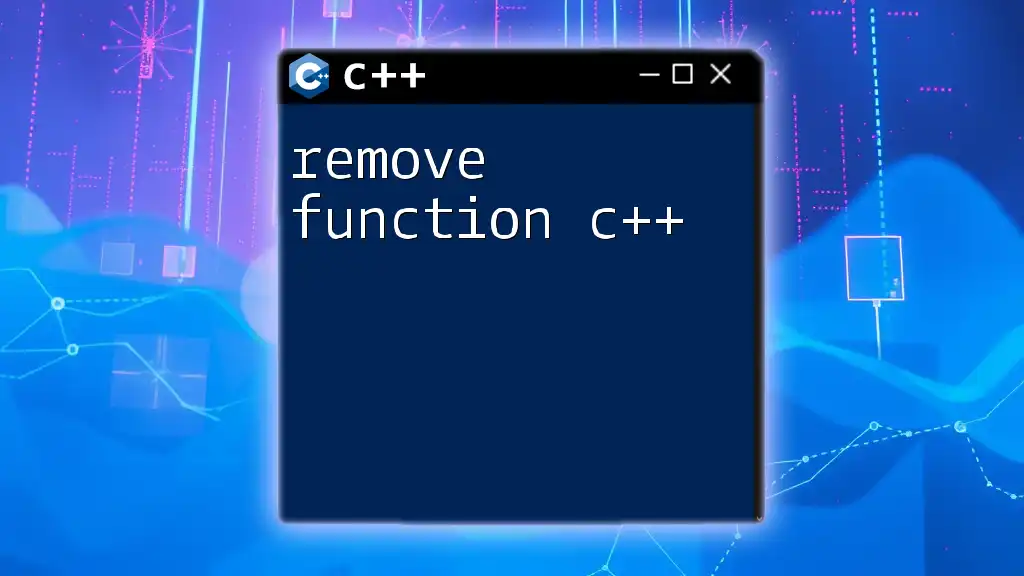
Best Practices Using Size Function in C++
Writing Clean and Efficient Code
To write clean and efficient code, it's advisable to leverage the size function when iterating through data structures. For example, when looping through a `std::vector`, keep the size accessible:
for (size_t i = 0; i < vec.size(); ++i) {
// process vec[i]
}
This approach not only makes your code clearer but also more efficient by avoiding repetitive size calculations within the loop.
Avoiding Common Mistakes
One common mistake is misunderstanding how arrays decay to pointers. For a static array like `int arr[10]`, using `sizeof(arr)` will yield the total size, but just passing `arr` to a function will convert it into a pointer, leading to misleading size calculations. Always be cautious of how you are obtaining sizes, especially in function calls.
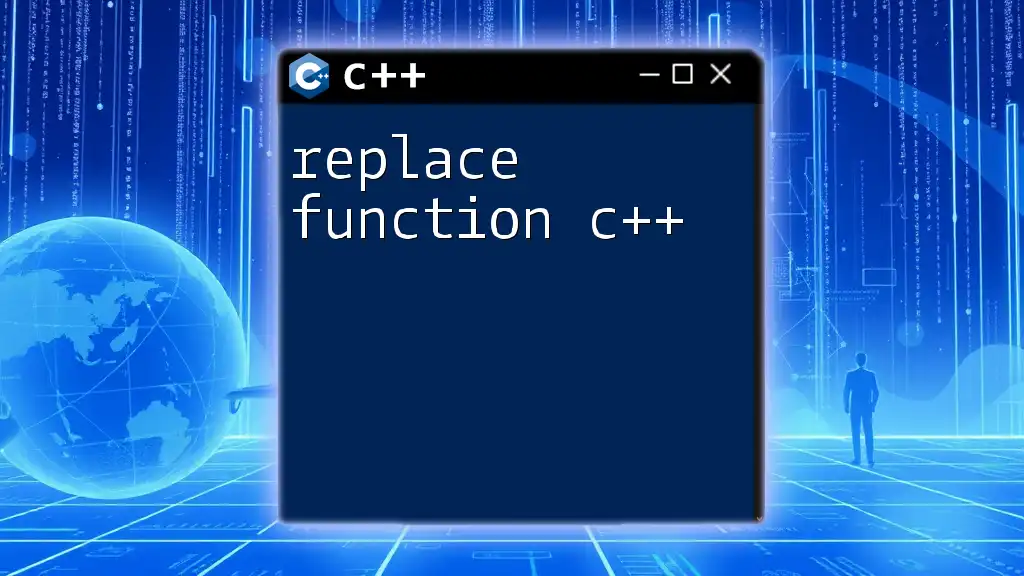
Performance Considerations
Impact of Size on Performance
The size of your data structures can significantly affect program performance. Larger data containers may require more time to traverse and manipulate. Understanding the size can help you optimize algorithms, especially when dealing with large datasets.
Optimizing for Size in Design
Designing data structures with size considerations in mind can lead to more efficient applications. Consider using deques or linked lists if you frequently modify the size of your datasets, instead of vectors or arrays, which may require expensive reallocation.
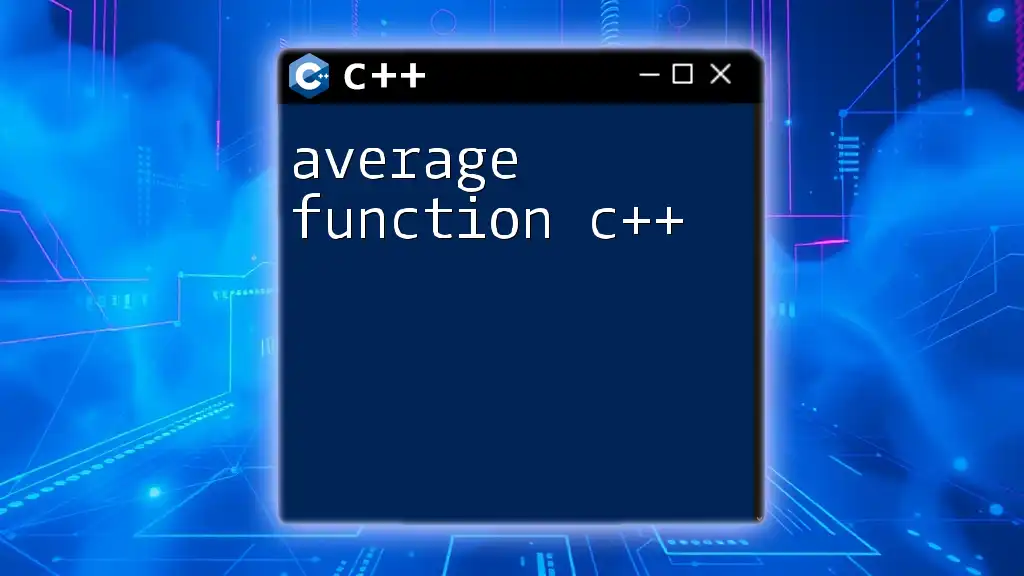
Conclusion
The size function in C++ is a powerful tool that every developer should be familiar with. It provides essential insights into memory consumption and performance, thereby allowing for optimized and reliable code. By understanding its use across various contexts, you can enhance your programming skills and ensure that your applications are both efficient and effective.
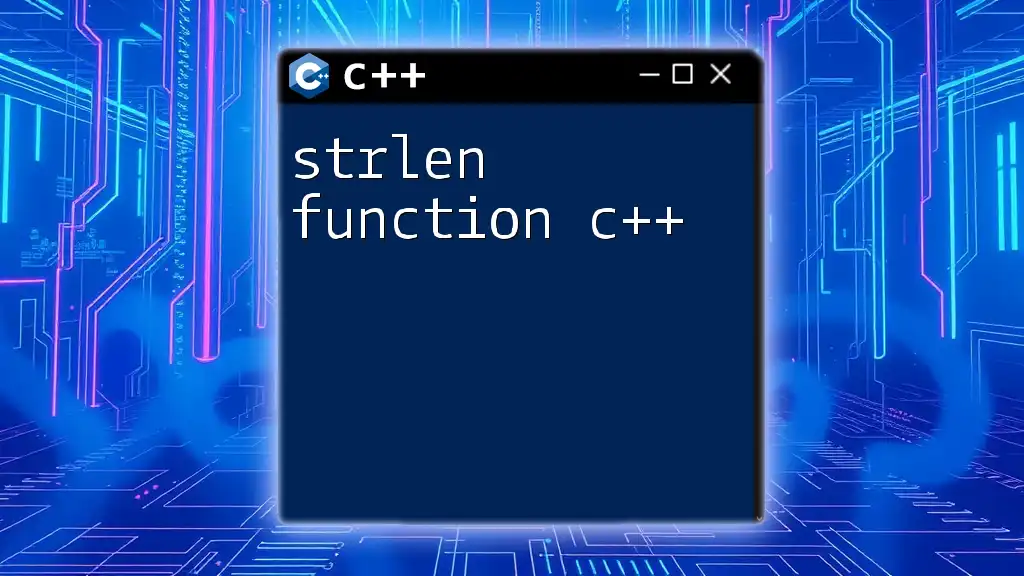
Additional Resources
To broaden your knowledge and enhance your expertise in using the size function in C++, it is beneficial to refer to the official C++ documentation and reliable programming literature. Exploring examples in depth and experimenting with different data structures can significantly improve your proficiency in this essential area.