In C++, a header file is used to declare functions, classes, and other identifiers which can be reused across multiple source files, enhancing modularity and organization in the code.
Here’s a basic example of how to create and include a header file in C++:
// my_functions.h
#ifndef MY_FUNCTIONS_H
#define MY_FUNCTIONS_H
void greet();
#endif // MY_FUNCTIONS_H
// main.cpp
#include <iostream>
#include "my_functions.h"
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
What are Header Files?
Header files are an essential component of C++ programming. They serve as containers for declarations of functions, classes, and variables. By including header files, programmers can leverage predefined functionalities, which facilitates code reusability and modular programming. The most common file extensions associated with header files are `.h` and `.hpp`.
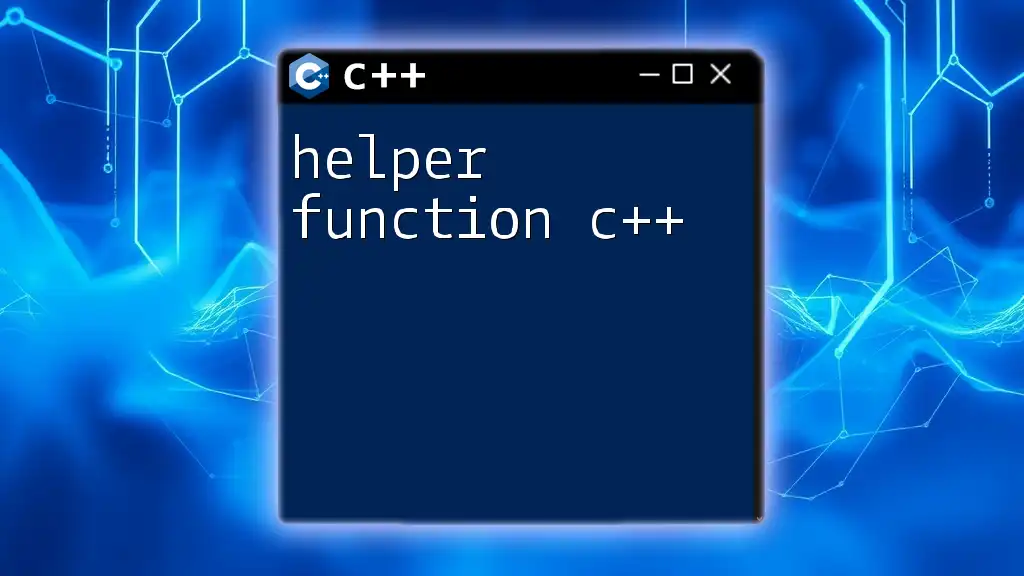
Types of Header Files
Standard Library Headers
C++ offers a rich Standard Library, which includes a variety of headers that provide a wide array of functionalities. These include:
- Input/Output: The `<iostream>` header is fundamental for input and output operations.
- Containers: Headers like `<vector>` and `<map>` provide dynamic array and associative array functionalities, respectively.
- Strings: The `<string>` header allows for robust string manipulation capabilities.
By including these headers, you can directly access their functions and classes without needing to write them from scratch.
User-Defined Headers
User-defined headers are created by programmers to encapsulate their own functions or classes. This practice promotes modularity and keeps the codebase organized. For instance, if you are developing a project with several utility functions, you can group all of these functions in a single header file to simplify your code structure.
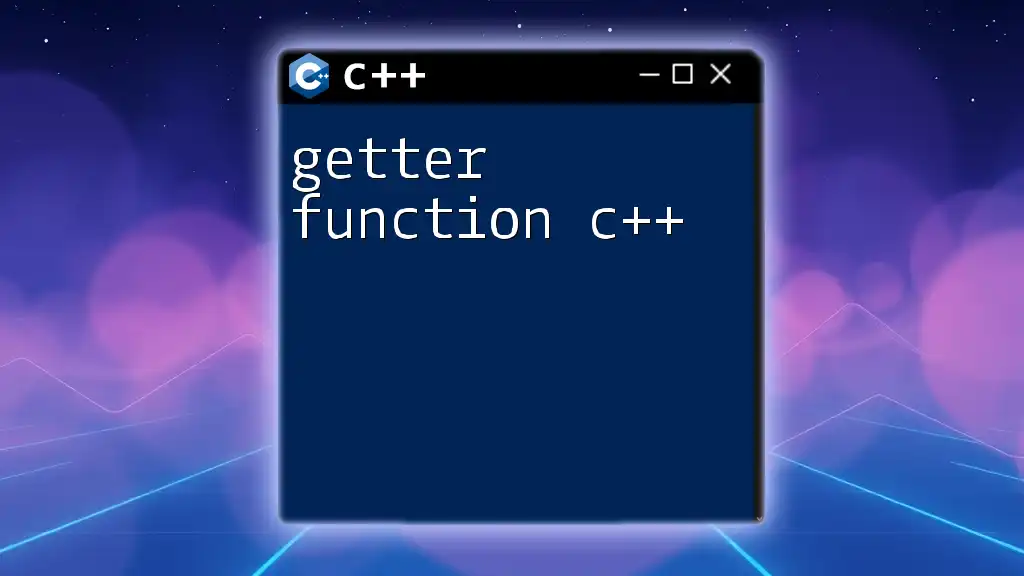
Structure of a Header File
Basic Syntax
The basic structure of a header file follows a simple syntax that includes declarations without definitions (the latter typically resides in the corresponding source file). A minimal user-defined header file might look like this:
// my_header.h
#ifndef MY_HEADER_H
#define MY_HEADER_H
void myFunction();
#endif
In this example, `myFunction()` is declared in the header file, while its definition would ideally be found in a corresponding source file, such as `my_header.cpp`.
Include Guards
Include guards prevent a header file from being included multiple times in the same compilation unit, which could lead to redefinition errors. The use of `#ifndef`, `#define`, and `#endif` effectively wraps your header content and ensures it’s processed only once during compilation. Here’s another example demonstrating include guards:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Header content here
#endif
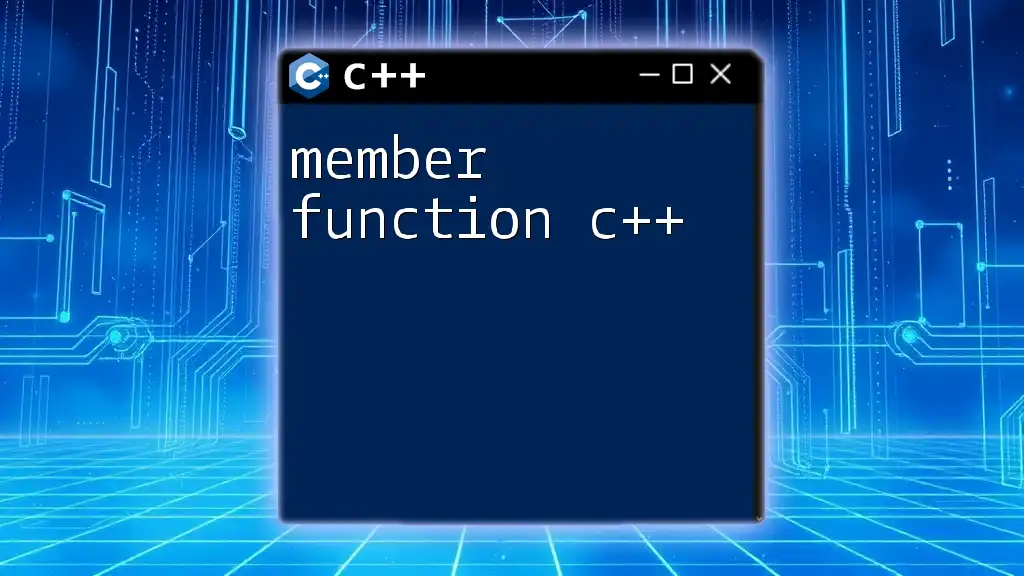
How to Include Header Files in C++
Using `#include`
The `#include` directive is the means by which header files are included in C++ programs. You can include standard libraries or user-defined headers using two different styles: angle brackets (`<>`) or double quotes (`""`).
-
Angle brackets are used for standard headers:
#include <iostream>
-
Double quotes are used for user-defined headers:
#include "my_header.h"
The distinction in quotation styles helps the compiler identify the header locations: it looks in system directories for angle-bracketed files, while it searches the current directory for quoted files.
Importing Specific Functions
Instead of importing the entire header file, you can choose to import specific functions using the `using` directive. This approach minimizes namespace pollution and promotes clarity in your code. Here’s an example:
#include <iostream>
using std::cout;
using std::endl;
This will allow you to use `cout` and `endl` directly without prefixing them with `std::`.
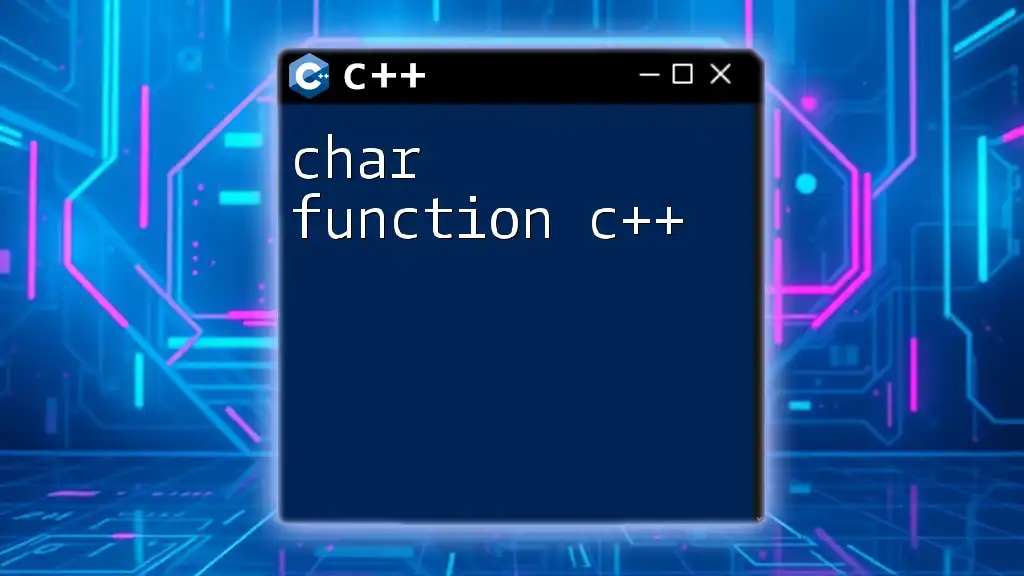
Best Practices for Using Header Files
Organizing Your Code
Effective code organization is paramount in programming. Group related functions into dedicated header files, such as `math_utilities.h` for mathematical functions. This logical categorization increases readability and maintainability.
Avoiding Name Clashes
Name clashes can be a source of confusion, especially in larger projects. A good practice to prevent this is to utilize namespaces. For example, by defining your functions inside a namespace:
namespace MyNamespace {
void myFunction();
}
This encapsulation of names ensures that functions defined in different files do not conflict.
Commenting and Documentation
Having well-commented header files enhances team collaboration and self-documentation in your code. Always describe the purpose of your functions, expected parameters, and return values. This level of detail makes it easier for others (or yourself in the future) to understand and utilize the functions.
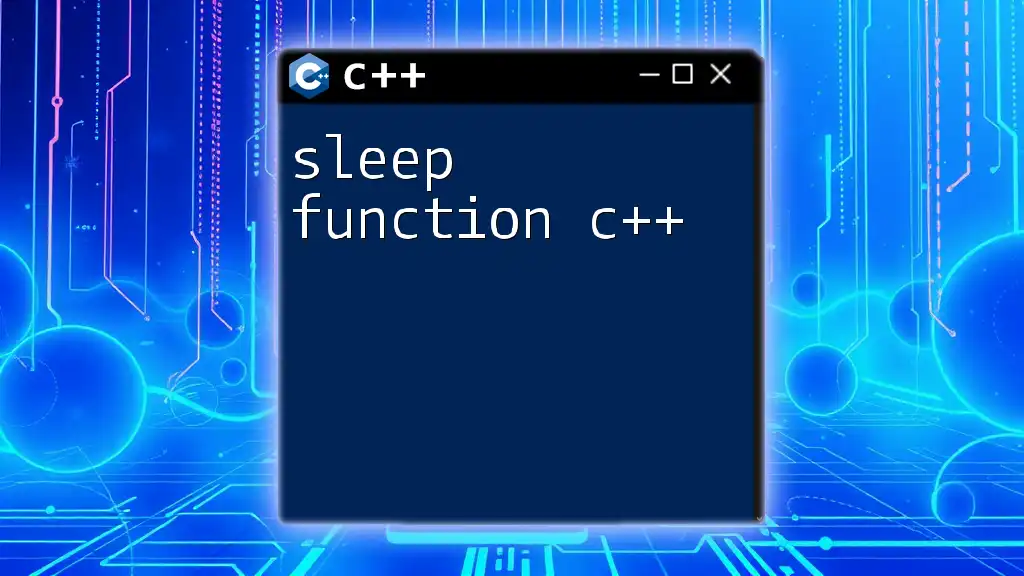
Common Issues with Header Files
Circular Includes
Circular includes occur when two or more header files include each other, leading to compilation issues. This usually disrupts the compilation process and can cause confusion. A common solution to this problem is to redesign the dependencies of your header files. Using forward declarations can also alleviate this issue, allowing you to define a class/interface without providing the complete declaration.
Compilation Errors
Compilation errors related to headers usually arise from improper inclusion practices or naming conflicts. For example, a circular include scenario might look like this:
// A.cpp
#include "B.h"
// B.h (Circular Include)
#include "A.h"
This kind of structure can lead to complications during the compilation phase, emphasizing the need for careful management of header dependencies.
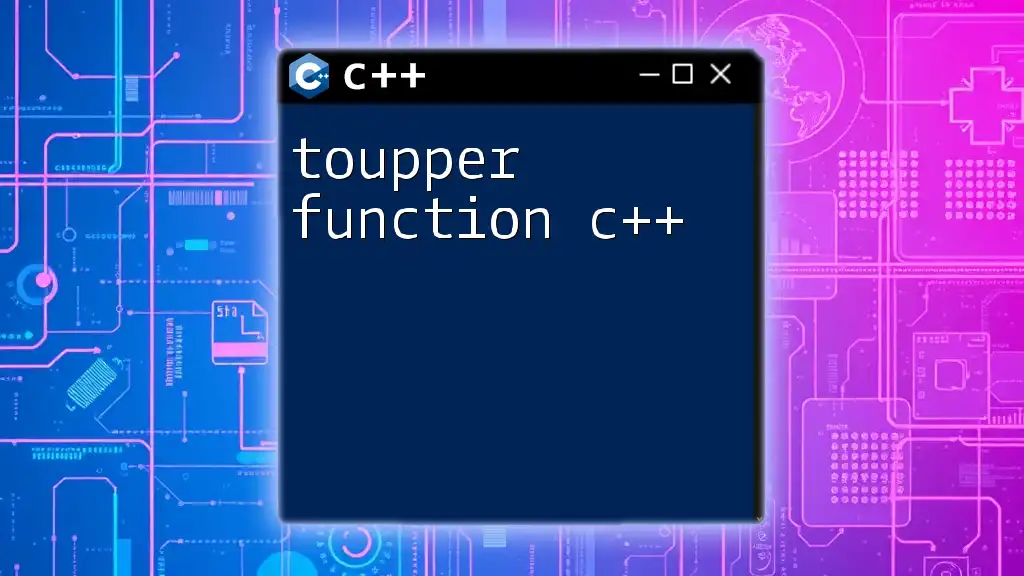
Conclusion
Header files are an integral part of C++ programming that allows for organized, efficient, and modular coding practices. By mastering their usage, you enable yourself to write better, cleaner, and more maintainable code. As you deepen your experience with C++, practice using header files to enhance both the functionality and clarity of your projects.
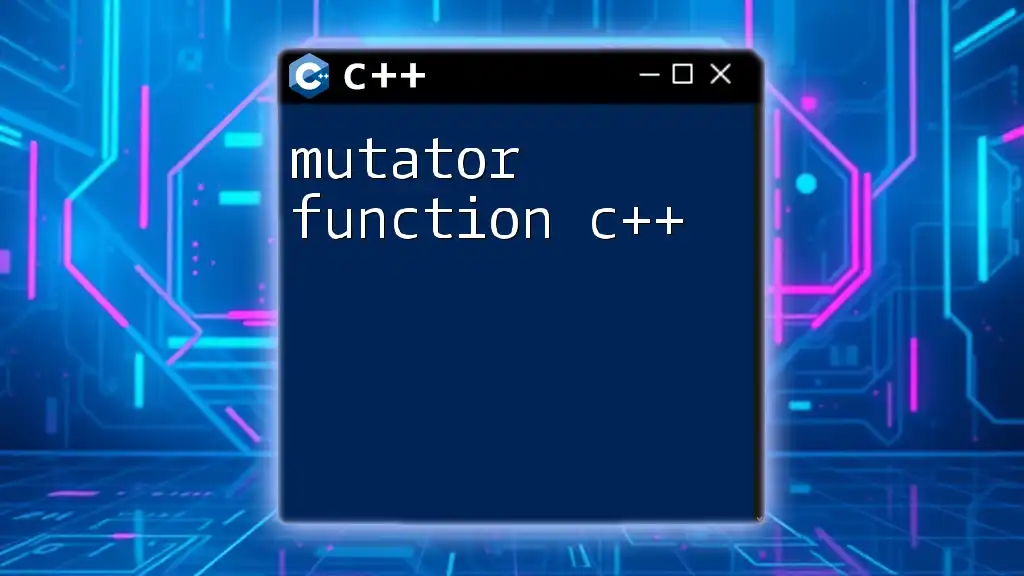
Further Reading and Resources
For more in-depth understanding, consider exploring the official C++ documentation on header files. Supplement your learning with books and online courses that focus on C++ programming, as they can offer valuable insights and tips on best practices.