In C++, using `const` after a member function declaration signifies that the function does not modify the object on which it is called, ensuring the integrity of the object's state.
Here's a code snippet to illustrate this:
class MyClass {
public:
int getValue() const { // 'const' indicates this function does not modify the object
return value;
}
private:
int value = 42;
};
What is the Purpose of 'const'?
In C++, the keyword `const` is pivotal for creating immutable data. By signifying that a variable or function parameter is constant, it informs the compiler—and other programmers—that the value cannot change. This leads to safer code and promotes better programming practices.
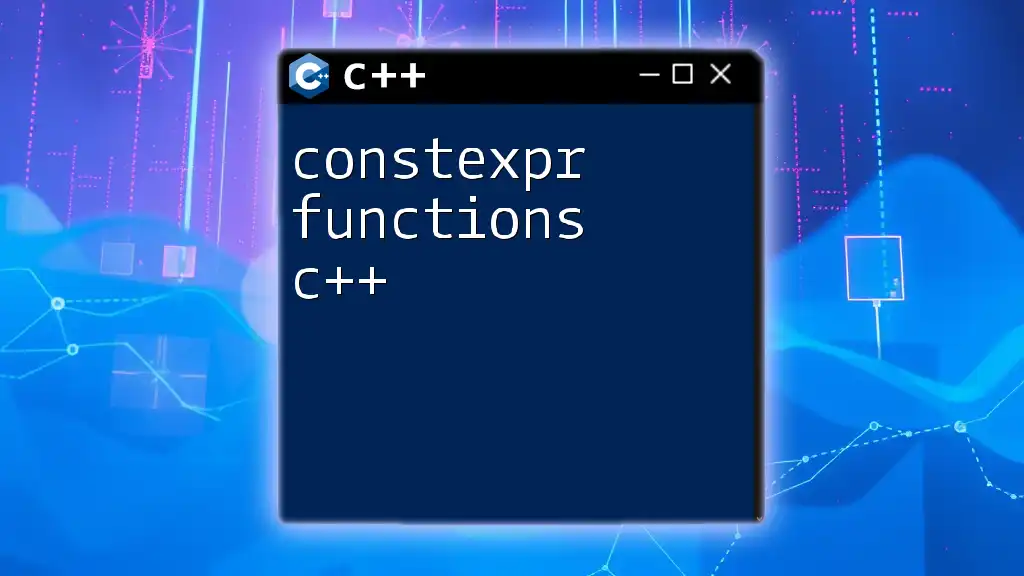
Understanding 'const' in C++
Definition of 'const'
The `const` keyword serves as a safeguard against modification. This means that once a data structure or variable is declared as `const`, attempts to modify its value will result in compilation errors. This immutability feature makes `const` particularly useful for preventing accidental changes that could disrupt the flow of a program.
Why Use 'const'?
Using `const` provides several advantages:
- Safety: It reduces the risk of unintentional modification of data.
- Readability: Code becomes easier to read, as developers understand which variables are meant to remain unchanged.
- Optimization: The compiler can optimize code better, knowing certain values will not change.
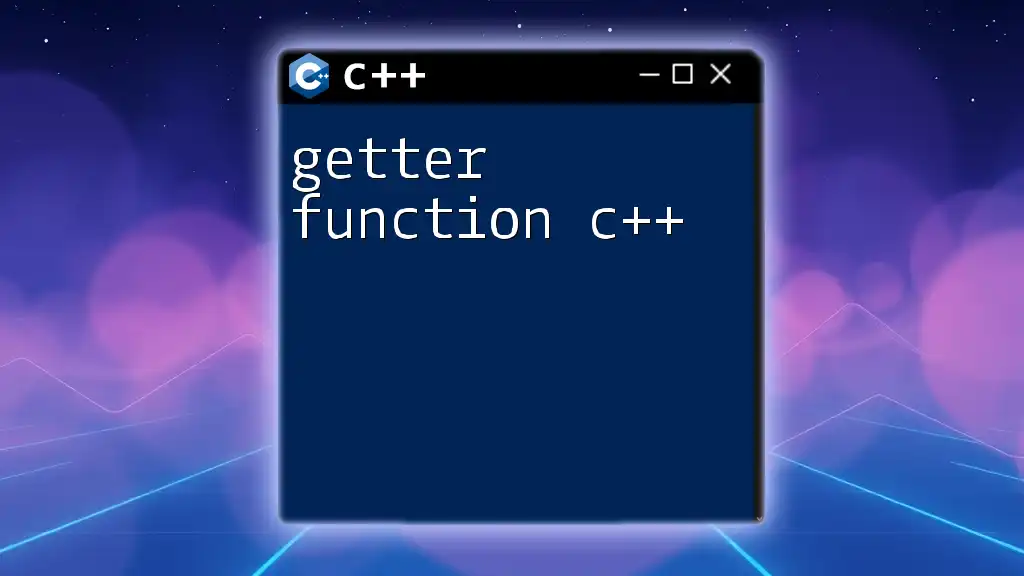
Applying 'const' After Functions
What Does 'const' After a Function Mean?
When the `const` keyword is placed after a member function declaration in a class, it specifies that the function will not modify any member variables of that class.
Characteristics of Const Member Functions
In a `const` member function, attempting to change any member variables will result in an error. This feature is essential for ensuring that member functions carry no side effects. Here’s a practical example:
class Example {
public:
void print() const {
// this->memberVariable = 5; // Error: cannot modify
std::cout << "Const member function" << std::endl;
}
private:
int memberVariable;
};
In this code, the `print()` function is defined as `const`. This means that it doesn't have the ability to modify the state of the `Example` object, ensuring integrity and predictability.
Understanding 'this' Pointer in Const Functions
In a `const` member function, the `this` pointer is treated as a pointer to a constant object. This distinction means that you cannot alter any member variables of the object within the function. For instance:
class Example {
public:
void modify() const {
// this->memberVariable = 1; // Error: cannot modify
}
private:
int memberVariable;
};
Here, attempting to modify `memberVariable` leads to a compilation error, reinforcing the concept of const-correctness.
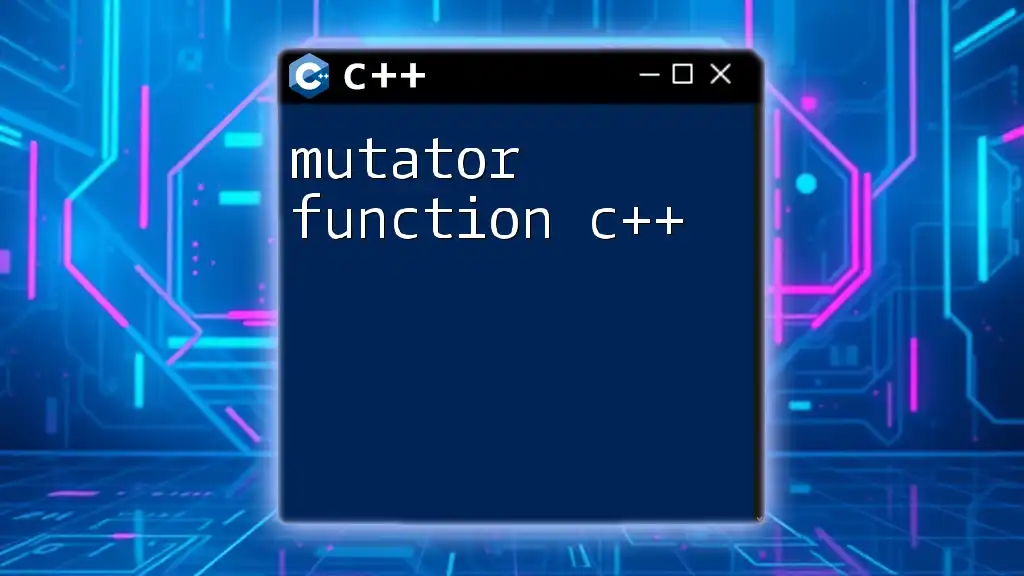
When to Use 'const' After a Function
Readability and Intent
Making member functions `const` signals to other developers that the function is intended to read rather than modify the object's state. This clarity enhances both the understanding and maintainability of the code.
Const-correctness
Const-correctness is the practice of using `const` consistently in your code. It prevents logical errors and safeguards against unwanted changes. By adhering to this principle, developers can ensure that their code behaves in a predictable manner.
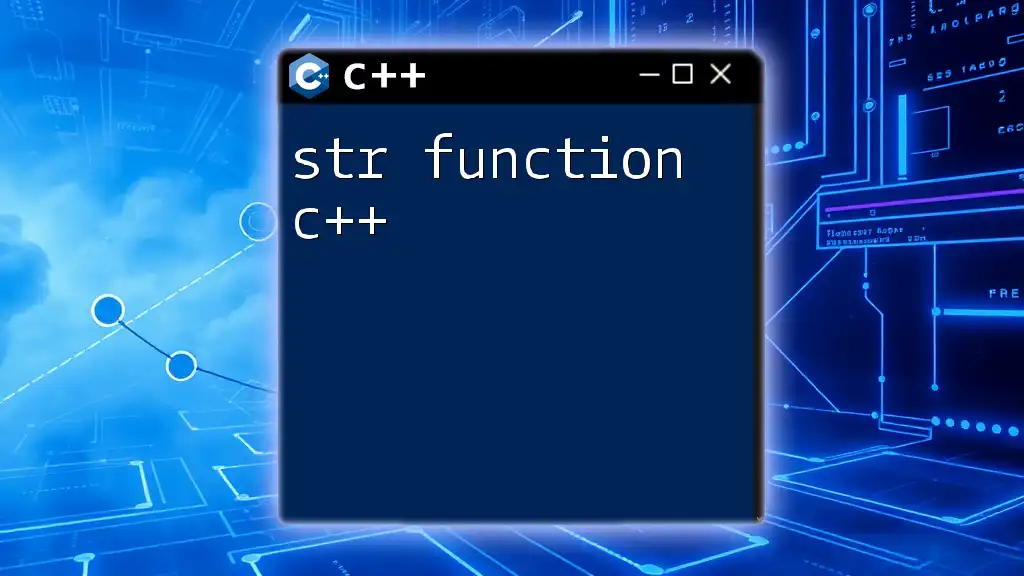
Real-World Examples
Using 'const' in Getter Functions
Getter functions often serve as a prime example of where `const` should be used. By using `const` in a getter, you assure that fetching the value does not alter the object's state. Here's how to implement it:
class Sample {
public:
int getValue() const {
return value;
}
private:
int value = 42;
};
In this example, `getValue()` is a `const` member function that safely returns the value without risking any modifications to it or the object.
Using 'const' in Operator Overloading
When overloading operators, using `const` is essential. It helps maintain the integrity of the original object while allowing for controlled modifications in a copy. Here’s a practical implementation:
class Box {
public:
Box operator+(const Box& other) const {
Box newBox;
// Add properties of *this and other to newBox
return newBox;
}
};
In this snippet, the `operator+` function allows for the calculation of a new `Box` object while ensuring that neither operand is modified.
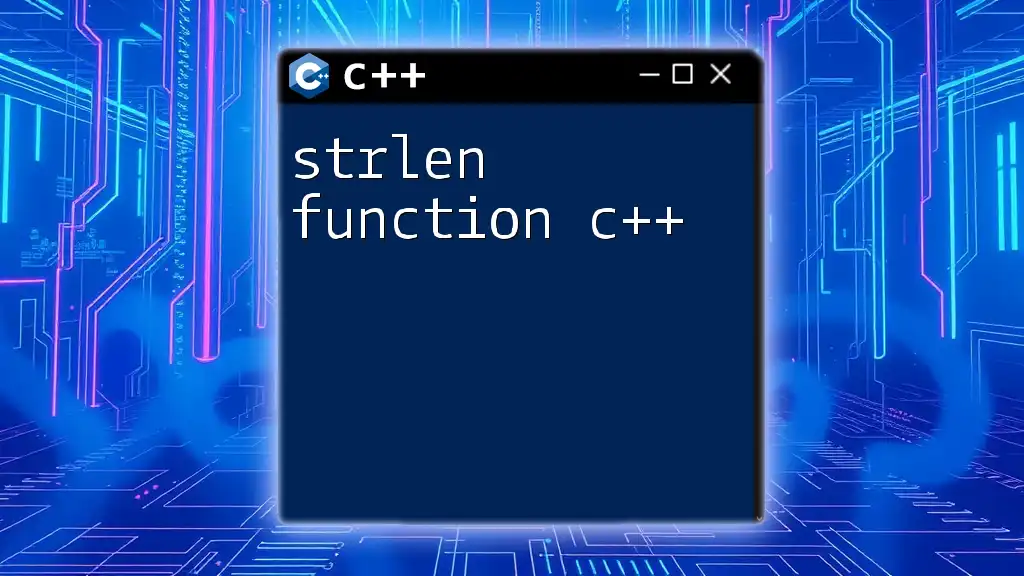
Best Practices for Using 'const'
General Guidelines
- Declare member functions as `const` whenever they do not modify the object's state.
- Use `const` for local variables in functions when they should remain unchanged.
- Employ const parameters in function signatures wherever possible to ensure that passed arguments are not modified.
Combining 'const' with Pointers and References
Understanding how `const` interacts with pointers and references is crucial. For instance, consider the differences:
- `const T*`: Pointer to a constant object, the object cannot be modified through the pointer.
- `T* const`: A constant pointer, which means the pointer itself cannot be changed to point to another object, but the object it points to can be modified.
- `const T* const`: A constant pointer to a constant object, neither the pointer nor the object can be modified through this pointer.
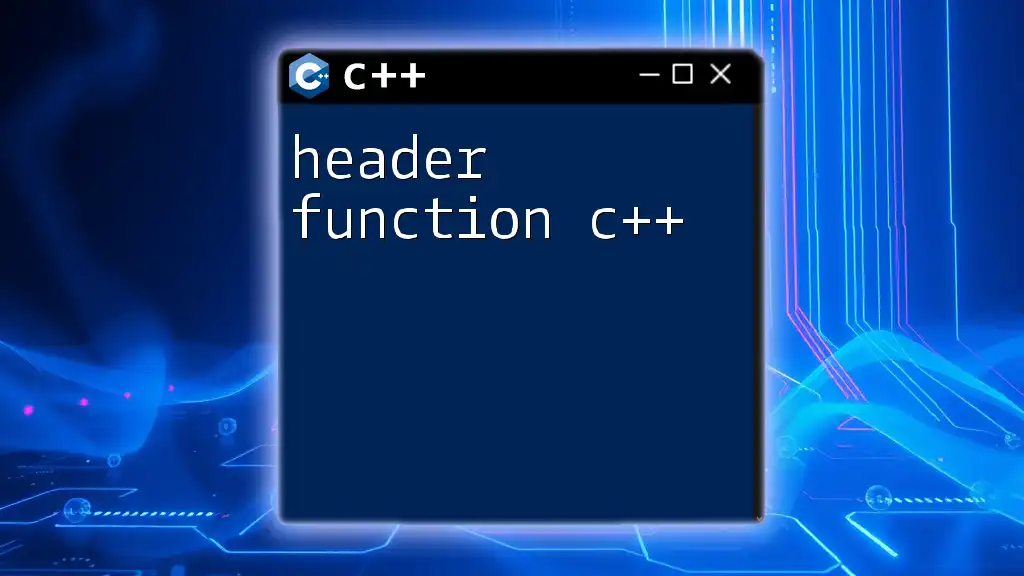
Common Pitfalls and Mistakes
Misunderstanding the Role of 'this'
Many developers new to `const` may mistakenly believe they can change member variables within a `const` member function. Forgetting that the `this` pointer, by default, becomes `const` within such functions leads to confusion and errors.
Neglecting Const-Correctness
Ignoring `const` can lead to bugs that are often difficult to trace. Functions that should not modify internal state can inadvertently do so, resulting in unpredictable behavior. Following the principle of const-correctness ensures your program's reliability and predictability.
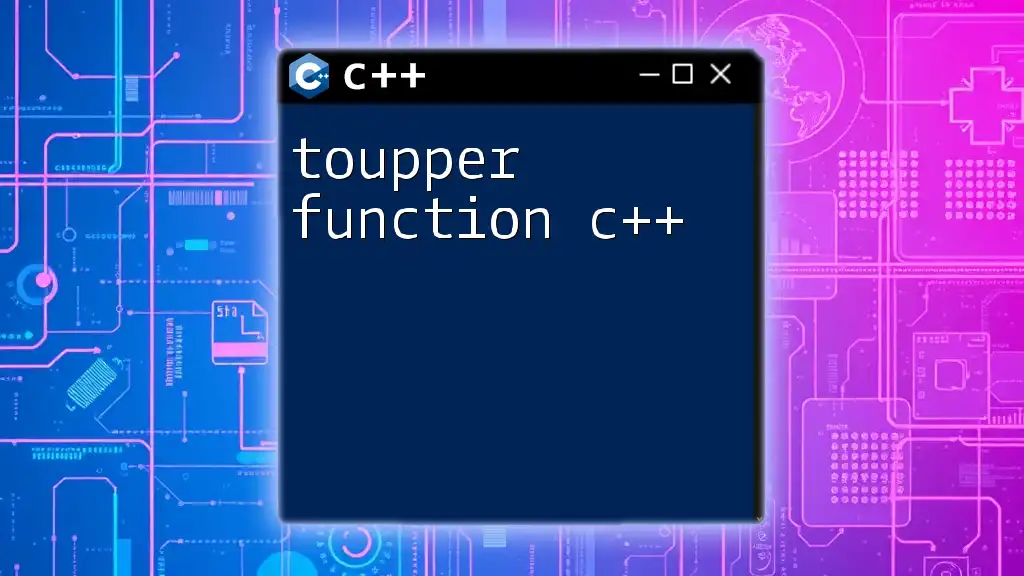
Conclusion
Utilizing `const` appropriately in C++ is foundational for creating safe, efficient, and maintainable code. By following the principles of const-correctness and understanding when to apply `const` after functions, you enhance the overall quality of your programs. Embrace these practices in your coding journey and see the difference in code clarity and reliability.