In C++, a string can be passed to a function as an argument, allowing the function to manipulate or utilize the string data effectively. Here's a simple example:
#include <iostream>
#include <string>
void printString(const std::string &str) {
std::cout << str << std::endl;
}
int main() {
printString("Hello, World!");
return 0;
}
Understanding the String Data Type
A string in C++ is a standard library object that represents a sequence of characters. Unlike traditional character arrays (C-style strings), which require manual memory management, C++ strings handle memory automatically and provide a wide range of functionalities. This automatic management simplifies coding and reduces the chances of memory leaks and buffer overflows.
Strings are widely used in programming for various tasks, such as user input, file manipulation, and network communications. Understanding how to manipulate strings effectively is crucial for any C++ programmer.

Importance of Strings in Functions
Strings enhance the capabilities of functions significantly. They allow you to pass user-defined data, perform string manipulations, and return meaningful textual information. Real-world applications like text processing, data parsing, and user interfaces benefit enormously from efficient string handling. Functions processing strings can transform user input into structured data, making them an essential tool in any developer's arsenal.

Basic String Operations
Declaring and Initializing Strings
Declaring a string in C++ is straightforward. You typically use the `std::string` class from the Standard Template Library (STL).
Example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << myString << std::endl;
return 0;
}
In this example, we declared a string variable `myString` and initialized it with "Hello, World!". You can also initialize strings using different methods, such as from character arrays or through user input.
String Length and Capacity
Understanding the length and capacity of strings is key to efficient programming. The `size()` or `length()` method returns the number of characters in the string, which is useful for iterations or validation.
Code snippet:
std::cout << "Length: " << myString.length() << std::endl;
The capacity of a string refers to the size of the allocated memory. You can check it using the `capacity()` method. Knowing both length and capacity can help you optimize performance, especially in function calls.
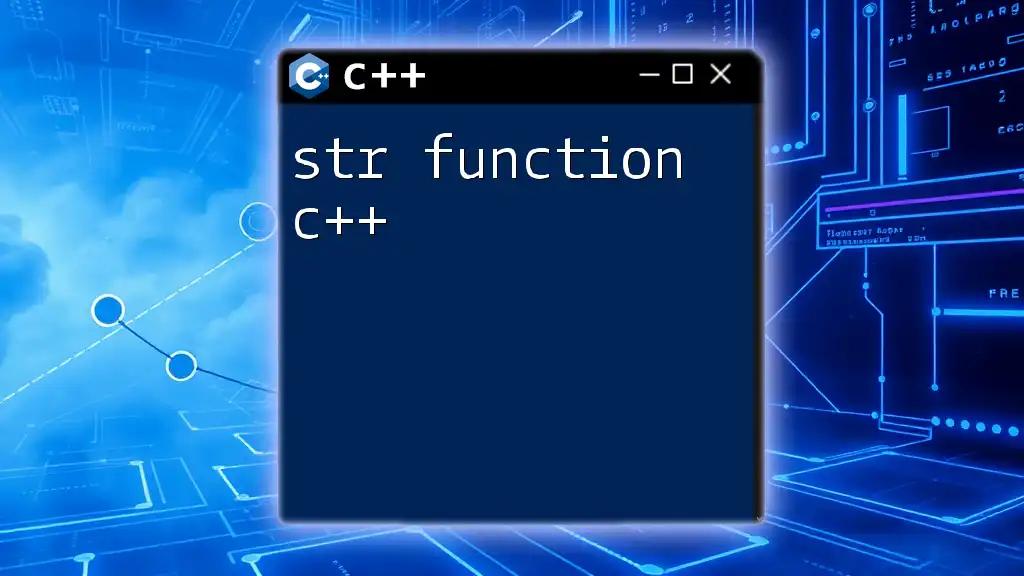
Using Strings as Function Parameters
Passing Strings by Value
When you pass a string by value, a copy of the string is created.
Advantages:
- Simple syntax
- Original string remains unmodified
Disadvantages:
- Performance overhead, especially with large strings
Code Example:
void greet(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
In this function, the string `name` is passed by value. If a large string is passed, it duplicates the string's data in memory, increasing overhead.
Passing Strings by Reference
Passing by reference allows the function to work with the original string without creating a copy. This is especially useful for large strings.
Code Example:
void modifyString(std::string& str) {
str += " Welcome!";
}
Here, the function `modifyString` takes a string reference. Any changes made to `str` directly affect the original string, which is efficient for memory usage and performance.
Using Constant References
To ensure that a function doesn’t modify a string while still allowing efficient memory usage, you can pass it as a constant reference.
Code Example:
void printString(const std::string& str) {
std::cout << str << std::endl;
}
In this example, `str` is a constant reference. The function can read from `str` but cannot modify it, thus ensuring safety while keeping performance intact.

String Manipulation Functions
Common String Functions
C++ provides various functions for easy string manipulation.
- Concatenation can be performed using the `+` operator or the `+=` operator.
Example:
std::string fullGreeting = myString + " How are you?";
- Finding substrings uses the `find()` method. This is helpful when you need to locate a specific part of a string.
Code snippet:
size_t position = myString.find("World");
- Substring extraction can be done with `substr()`.
Example:
std::string sub = myString.substr(7, 5); // Returns "World"
Modifying Strings
Strings can be modified dynamically using various methods.
- Replacing content within a string is handled via the `replace()` method.
Code snippet:
myString.replace(0, 5, "Hi");
- Inserting and erasing can be done using `insert()` and `erase()`.
Inserting Example:
myString.insert(5, "Beautiful ");
These manipulations make strings incredibly versatile and allow dynamic changes to string content.

String Streams in C++
Introduction to String Streams
String streams offer a way to perform input and output operations on strings, just like you would with standard input and output streams. They allow users to combine strings and various data types smoothly.
Using `std::stringstream`
Using `std::stringstream` makes it possible to construct strings incrementally.
Code example:
#include <sstream>
std::stringstream ss;
ss << "Age: " << 25;
std::string ageString = ss.str(); // ageString now contains "Age: 25"
This is particularly useful when you need to format data before storing it as a string, providing flexibility in constructing text outputs.
Practical Example: Converting Types
String streams simplify type conversion. You can extract other data types from strings seamlessly.
Example:
int number;
std::stringstream ss("42");
ss >> number; // number is now 42
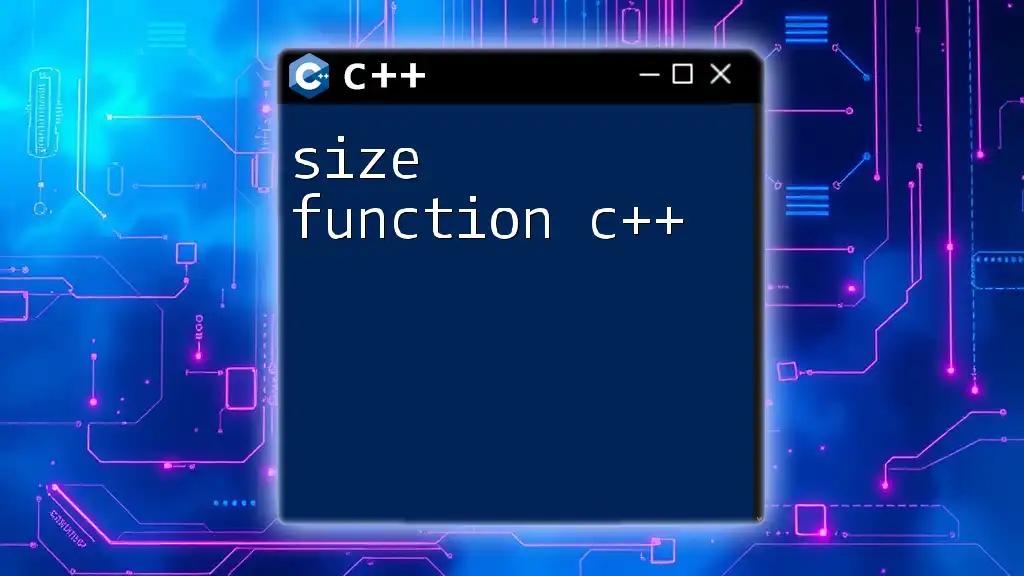
Error Handling with Strings
When dealing with strings, you should also be aware of common pitfalls. For example, managing memory correctly is vital. Using `std::string` helps mitigate many issues associated with C-style strings, which necessitate manual memory management and can lead to safety risks.
Example of Common Pitfalls:
char cStr[50];
std::strcpy(cStr, myString.c_str()); // Safe way to convert
Using `c_str()` method helps convert a `std::string` to a C-style string when necessary without losing the safety net provided by `std::string`.

Conclusion
In summary, understanding strings in function C++ empowers you to write more efficient and robust applications. Mastering basic operations, effective parameter passing, and string manipulation will enhance your programming skills significantly. Strings are not just a simple sequence of characters; they embody a range of functionalities that every effective C++ function can harness for better data handling and user interaction.
As you continue your journey in C++, it’s beneficial to practice these concepts frequently and explore more advanced string operations to enrich your coding expertise.