The C++ string constructor is used to create a string object from various inputs like a C-style string or by specifying a string length, allowing for flexible string initialization.
Here’s an example of using the string constructor with a C-style string:
#include <iostream>
#include <string>
int main() {
const char* cstr = "Hello, World!";
std::string str(cstr); // String constructor from a C-style string
std::cout << str << std::endl; // Outputs: Hello, World!
return 0;
}
Understanding the C++ String Class
The C++ string class is a powerful tool for managing and manipulating sequences of characters. Unlike C-style strings, which rely on character arrays and require manual memory management, the C++ string class abstracts these complexities, providing a range of features that simplify string handling. Key features of the C++ string class include:
- Dynamic sizing: Strings can grow or shrink as needed, eliminating concerns about buffer overflows.
- Memory management: Automatic handling of memory allocation and deallocation, reducing the risk of memory leaks.
- Convenience and functionality: A rich set of built-in methods for string manipulation, such as concatenation, comparison, and searching.
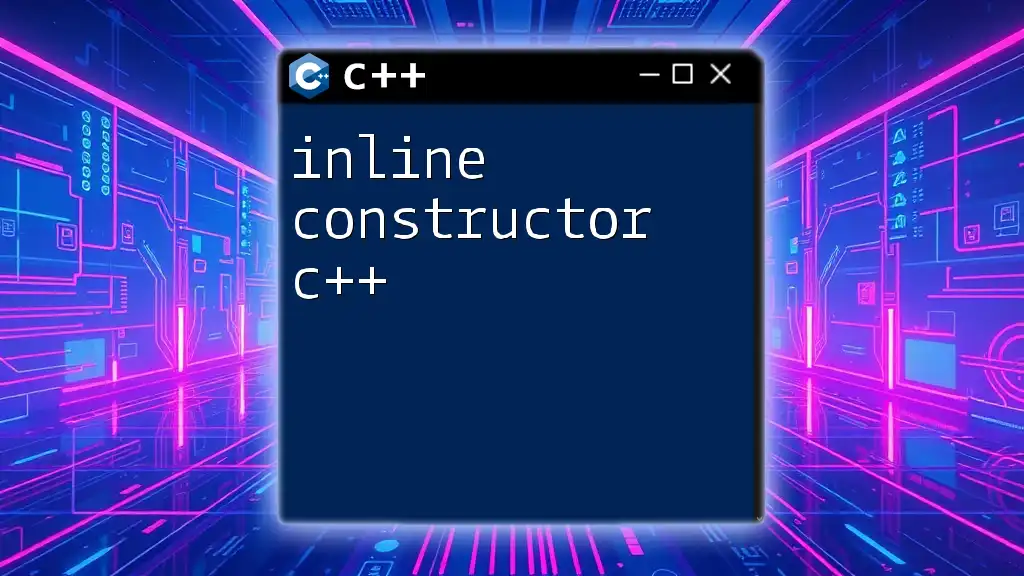
The C++ String Constructor
When we discuss the string constructor in C++, we refer to the various ways we can initialize a string object. Constructors play a crucial role in setting up the initial state of an object, enabling developers to create strings in a way that best suits their needs. Constructors allow strings to be initialized from character arrays or even with a specified number of repeated characters. The introduction of move semantics in C++11 further improved the performance and flexibility of string initialization.
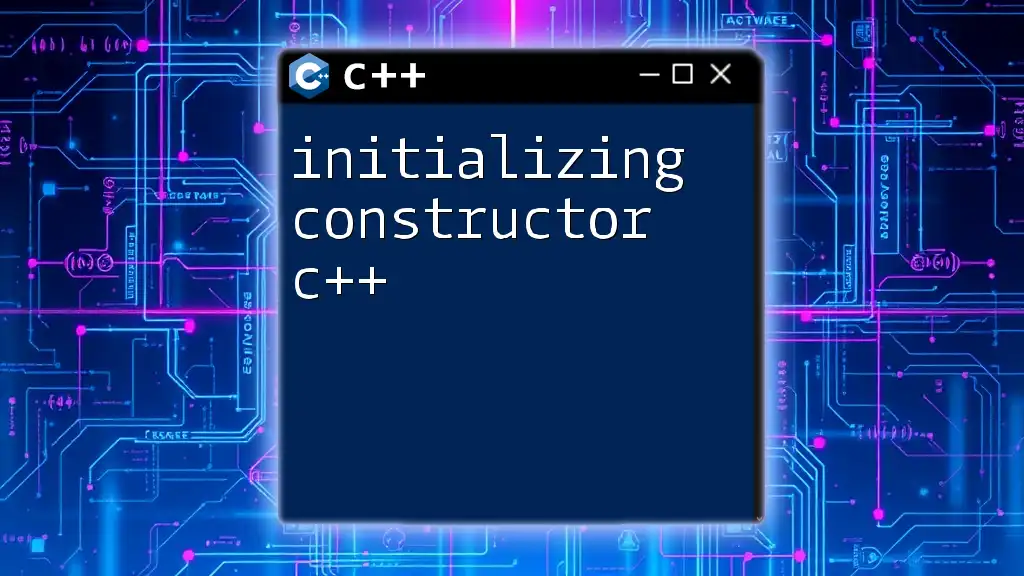
Types of C++ String Constructors
Default Constructor
The default string constructor creates an empty string. This is useful when you want to declare a string variable without assigning it a specific value initially.
std::string str1; // Creates an empty string
The empty string can later be assigned a different value using assignment operators or functions like `append()`. This constructor initializes the string to a zero-length state, ensuring that no garbage values are present.
Constructor with a Character Array
Another common way to initialize a string is by using a character array. This constructor converts a null-terminated C-style string into a C++ string.
const char* text = "Hello, World";
std::string str2(text); // Creates a string initialized with the character array
With this constructor, developers can directly convert legacy C-style strings into the more robust C++ string objects, allowing for easy integration of older C code within C++ applications.
Constructor with Repeated Characters
Sometimes, you might find yourself in a situation where you need a string initialized with repeated characters. The C++ string constructor allows you to specify both the character to repeat and the number of repetitions.
std::string str3(5, 'A'); // Creates "AAAAA"
This constructor is particularly useful for scenarios where rapid string initialization is necessary, such as placeholder strings in user interfaces or test data preparation.
Constructor with Substring
You can also construct a string by using a substring from another string or character array. This constructor is valuable when you want only a portion of a string.
std::string str4(text, 5); // Creates "Hello"
By specifying the starting point and the length, this constructor allows developers to efficiently extract substrings without needing to manually manipulate pointers or character arrays.
Copy Constructor
The copy constructor is used to initialize a new string object as a copy of an existing one. This is essential for ensuring that a new string has its unique copy of the data.
std::string str5 = str2; // Copying str2 into str5
With the copy constructor, changes made to `str5` will not affect `str2`, highlighting the importance of C++ string constructors in managing the integrity of string data.
Move Constructor (C++11 and beyond)
With the introduction of C++11, the move constructor emerged, allowing for more efficient resource management. Unlike the copy constructor, the move constructor 'steals' the resources of an existing string rather than duplicating them.
std::string str6 = std::move(str5); // Transfers ownership of str5's resources
This approach significantly increases performance, particularly for large strings, by avoiding expensive deep copies. Only the internal pointers are moved, and the original object is left in a valid but unspecified state, often leading to more efficient memory usage.
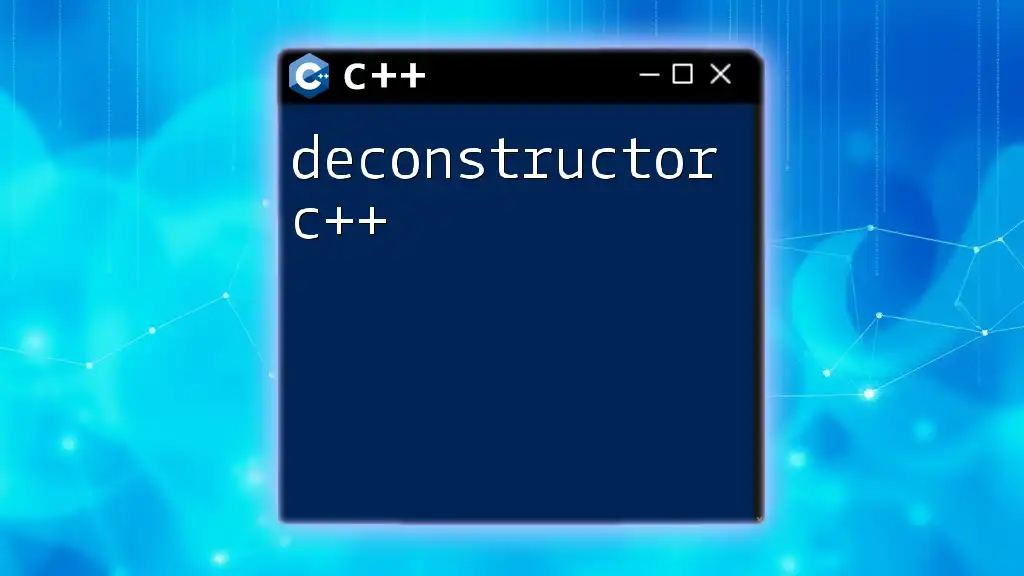
Practical Applications of String Constructors
C++ string constructors have numerous applications in real-world programming. They can be used whenever strings need to be created, modified, or manipulated, such as in user input handling, special formatting requirements, or data processing tasks.
For instance, when parsing data from a file, string constructors allow for the efficient reading and storing of individual lines or words. Initialization of formatted strings, like generating messages or reports, can also leverage these constructors' flexibility.
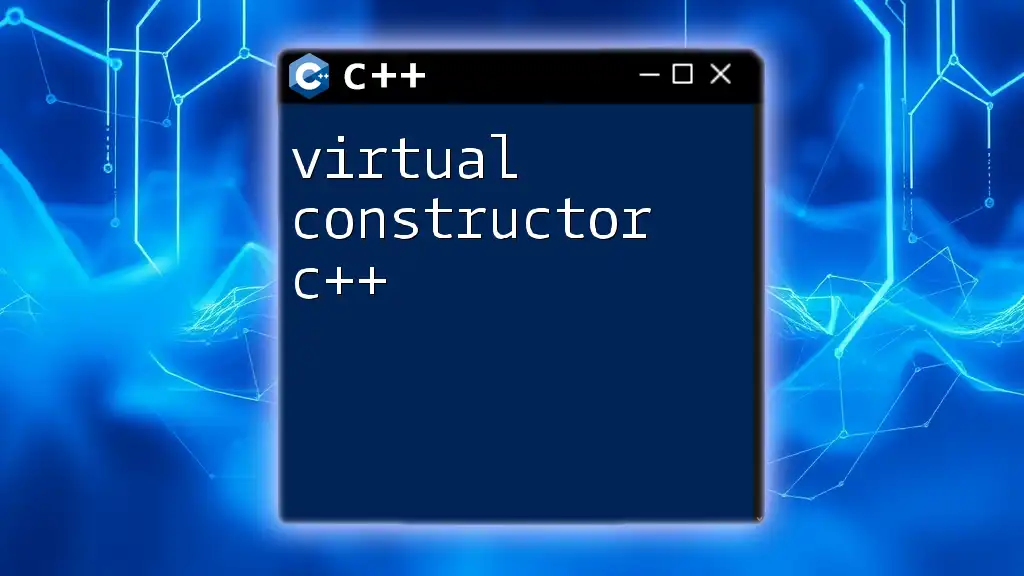
Best Practices for Using String Constructors
When utilizing string constructors in C++, follow these best practices to maximize performance and maintainability:
-
Choose the appropriate constructor: Select the constructor that best fits your needs. For example, prefer the move constructor for large objects where appropriate to avoid unnecessary copying.
-
Avoid potential pitfalls: Be mindful of initializing strings with character arrays that may not be null-terminated, as this can lead to undefined behavior. Ensure to validate or appropriately manage input data.
-
Leverage modern features: With C++11 and beyond, take full advantage of move semantics and the variety of constructors provided by the string class to enhance your programs' efficiency.
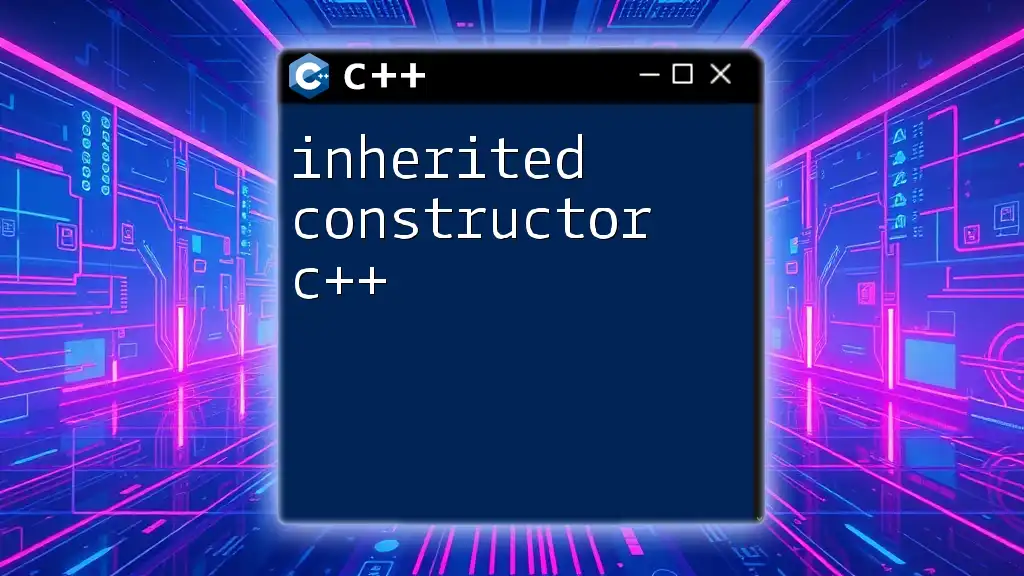
Conclusion
Understanding the various string constructors in C++ can significantly enhance your ability to work with string data. This flexibility not only simplifies string manipulation but also ensures that you can write efficient and maintainable code. Whether initializing an empty string, copying an existing one, or utilizing modern move semantics, mastering these constructs will pave the way for more effective C++ programming.
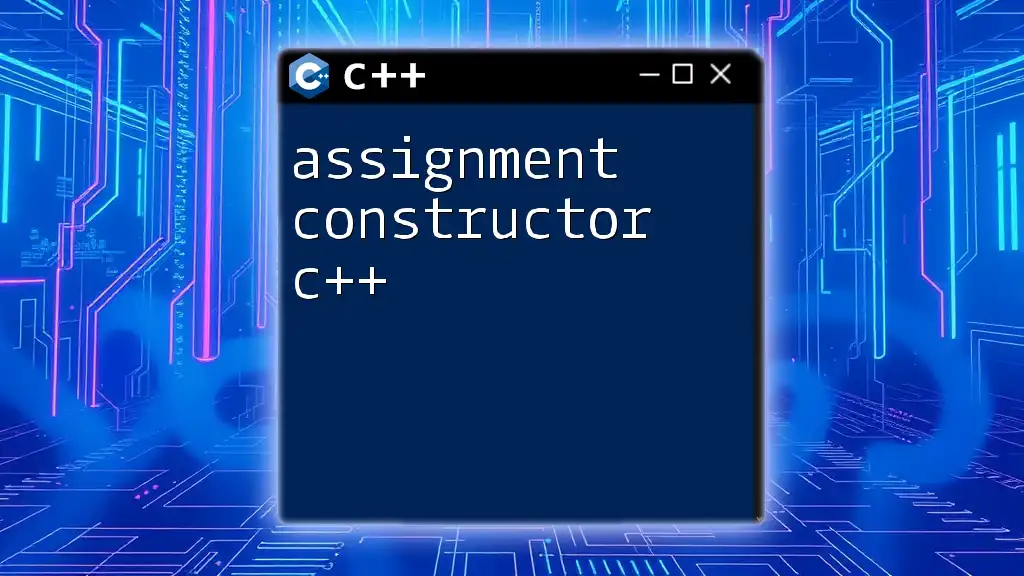
Additional Resources
For those looking to dive deeper into C++ string handling and explore related topics, consider referring to the official documentation, community forums, or comprehensive guides on C++ programming. Each of these resources will provide you with additional insights and practical examples to further solidify your understanding.