In C++, you can convert a `std::string` to a `double` using the `std::stod` function, which parses the string and returns the corresponding double value.
Here’s a simple example:
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double dbl = std::stod(str);
std::cout << "The double value is: " << dbl << std::endl;
return 0;
}
Understanding Strings and Doubles in C++
What is a String?
A string in C++ is a sequence of characters represented by the `std::string` class from the C++ Standard Library. This class makes it easier to handle and manipulate texts. A `std::string` can dynamically adjust its size to accommodate the characters stored in it. Here’s an example of declaring a string:
std::string str = "123.45";
What is a Double?
In C++, a double is a data type used for representing floating-point numbers. It is capable of storing a larger range of values compared to `float` due to its increased precision, making it ideal for calculations where accuracy is critical. Here's how you can declare a double:
double num = 123.45;
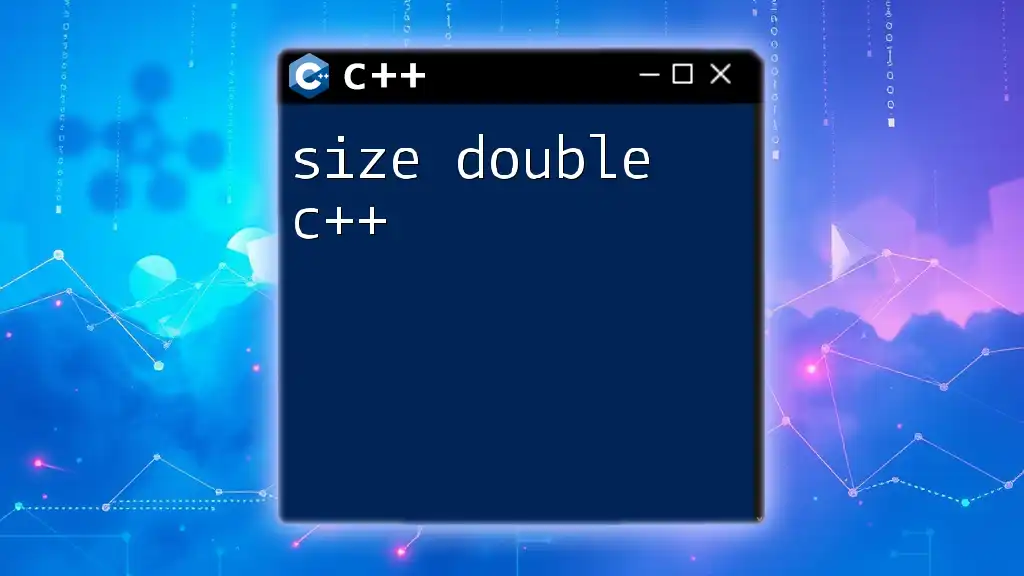
Reasons for Converting String to Double
Converting a string to double in C++ is crucial in various situations:
- Data input: When receiving user input that needs to be processed as a numerical value for computations or calculations.
- File operations: Reading data from files often involves strings that represent numerical values, where conversion is necessary for further processing.
- Validation: Before performing any numerical operations, ensuring that the string can be converted to a double helps maintain program stability and correctness.
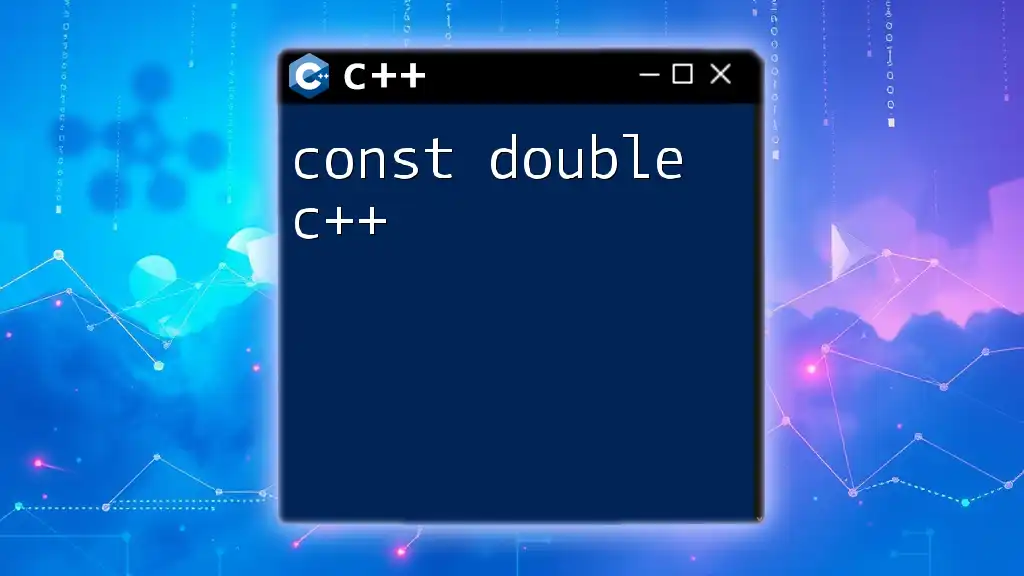
Methods to Convert String to Double in C++
Using `std::stod()`
Syntax and Basic Usage
The `std::stod()` function is a convenient way to convert a `std::string` to a double. It is part of the `<string>` header file and has a straightforward syntax:
double num = std::stod("123.45");
Example of `std::stod()`
To illustrate its use, here’s a simple example that demonstrates how to convert a string representation of a number into a double:
#include <iostream>
#include <string>
int main() {
std::string str = "123.45";
double num = std::stod(str);
std::cout << "String converted to double: " << num << std::endl; // Output: 123.45
return 0;
}
Handling Exceptions
While `std::stod()` is effective, it can throw exceptions when invalid input is encountered. The `std::invalid_argument` exception is thrown when the provided string does not represent a valid number, while `std::out_of_range` is thrown if the converted value falls outside the range of representable values. Here’s an example of how to handle these exceptions gracefully:
try {
double num = std::stod("invalid");
} catch (const std::invalid_argument& e) {
std::cout << "Invalid input: " << e.what() << std::endl;
}
Using `std::stringstream`
The Concept of String Stream
`std::stringstream` is another robust way to convert strings to doubles. It is part of the `<sstream>` header and allows for more complex conversion scenarios, especially when additional formatting is required.
Syntax and Basic Usage
Using `std::stringstream`, the conversion can be performed as follows:
#include <sstream>
std::stringstream ss("123.45");
double num;
ss >> num;
Example of `std::stringstream`
Here’s a complete example showcasing how `std::stringstream` can be used for conversion:
#include <iostream>
#include <sstream>
int main() {
std::string str = "123.45";
std::stringstream ss(str);
double num;
ss >> num;
std::cout << "String converted to double: " << num << std::endl; // Output: 123.45
return 0;
}
Using `strtod()`
Overview of `strtod()`
The `strtod()` function from the `<cstdlib>` header is a C-style approach to converting strings to doubles. It provides a way to convert a C-style string (const char*) and offers additional functionality over `std::stod`, such as handling pointers to track where parsing ends.
Syntax and Example
Here’s how to use `strtod()`:
#include <cstdlib> // for strtod
#include <iostream>
int main() {
const char* str = "123.45";
char* ptr;
double num = strtod(str, &ptr);
std::cout << "String converted to double: " << num << std::endl; // Output: 123.45
return 0;
}
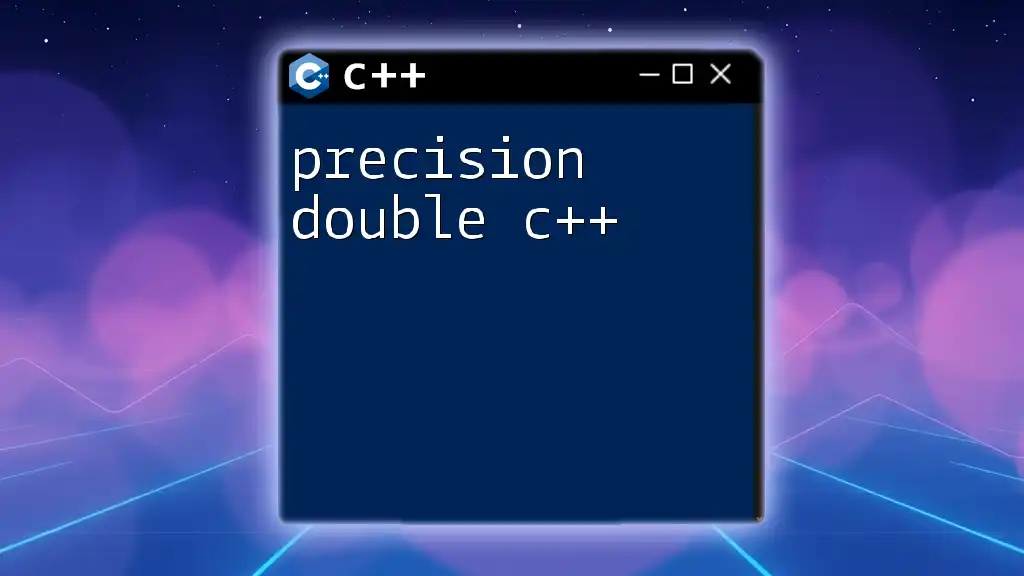
Performance Considerations
When converting strings to doubles in C++, performance may vary depending on the method used. While `std::stod()` offers simplicity and safety, `std::stringstream` and `strtod()` can be preferred for scenarios that require high performance or additional control over parsing. It’s essential to consider your specific use case when choosing the best method.
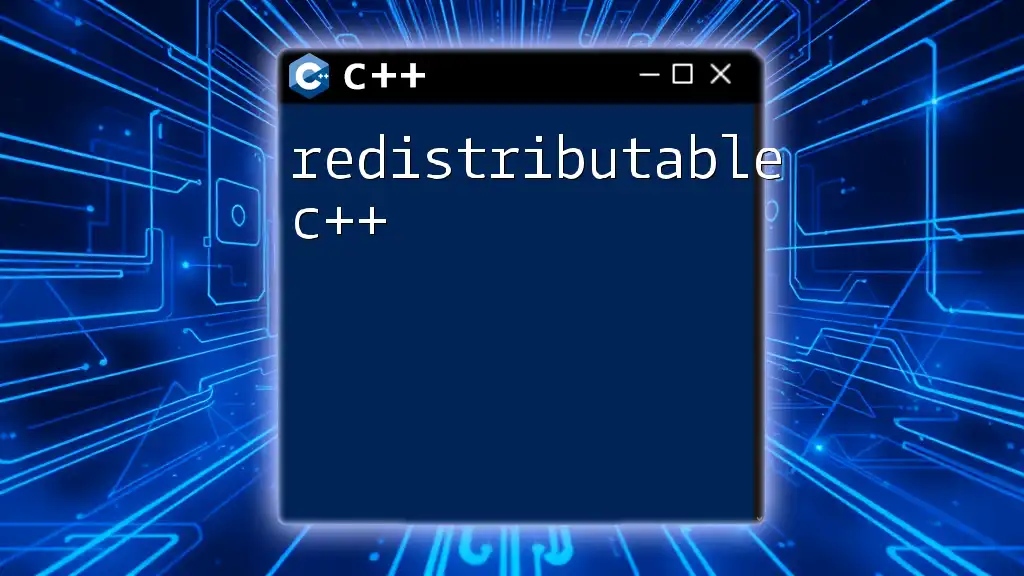
Common Pitfalls and How to Avoid Them
Handling Invalid Inputs
One of the most common issues when dealing with string-to-double conversions is the presence of invalid inputs. Examples include empty strings, non-numeric characters, and unexpected formats. Always validate input before conversion to avoid runtime exceptions and make sure to provide user feedback when invalid input is detected.
Locale and Number Formats
The locale settings can significantly impact how number formats are interpreted. For instance, in certain locales, a comma may be used as a decimal separator instead of a dot. When converting strings in varying locales, consider implementing locale-aware parsing to ensure that numbers are correctly interpreted.
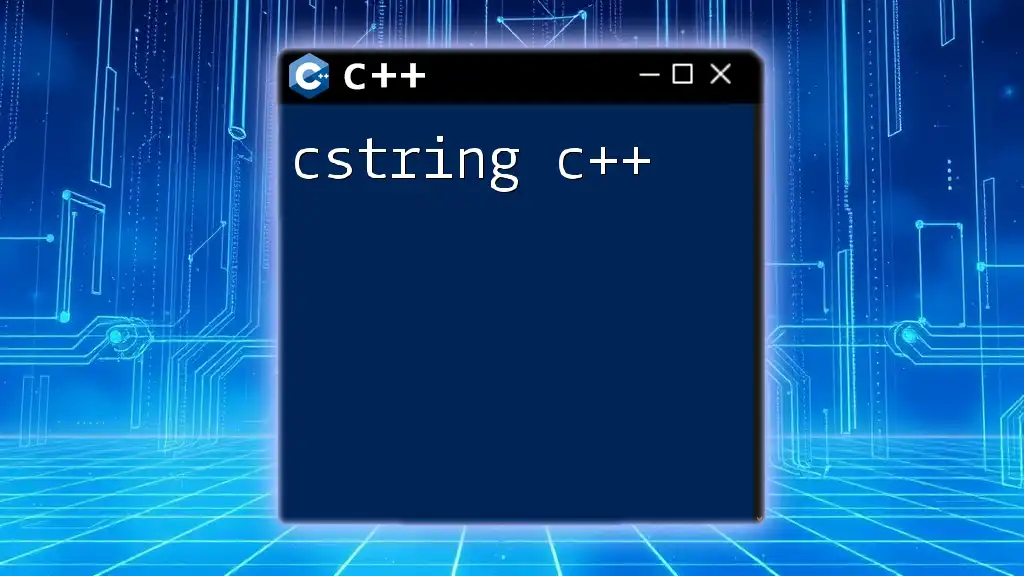
Best Practices for String to Double Conversion
Incorporating effective practices when converting strings to doubles can enhance your C++ programs:
- Validate strings before attempting conversion to catch potential issues early.
- Handle exceptions appropriately to maintain program stability.
- Use the right method based on performance needs and context.
- Normalize input data to account for locale differences and ensure consistent interpretation.
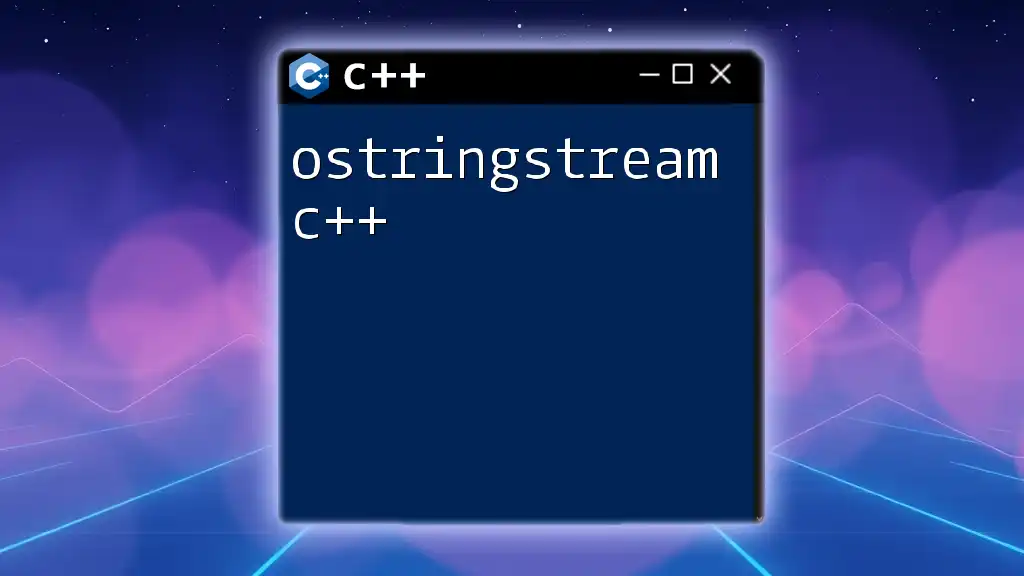
Conclusion
Understanding how to convert string double c++ is a crucial skill for any developer working with user input and data processing. By mastering the various methods available, such as `std::stod()`, `std::stringstream`, and `strtod()`, you can write more robust and flexible programs. Practice with the examples provided, and explore more advanced string manipulation and numeric conversions to enhance your C++ capabilities.
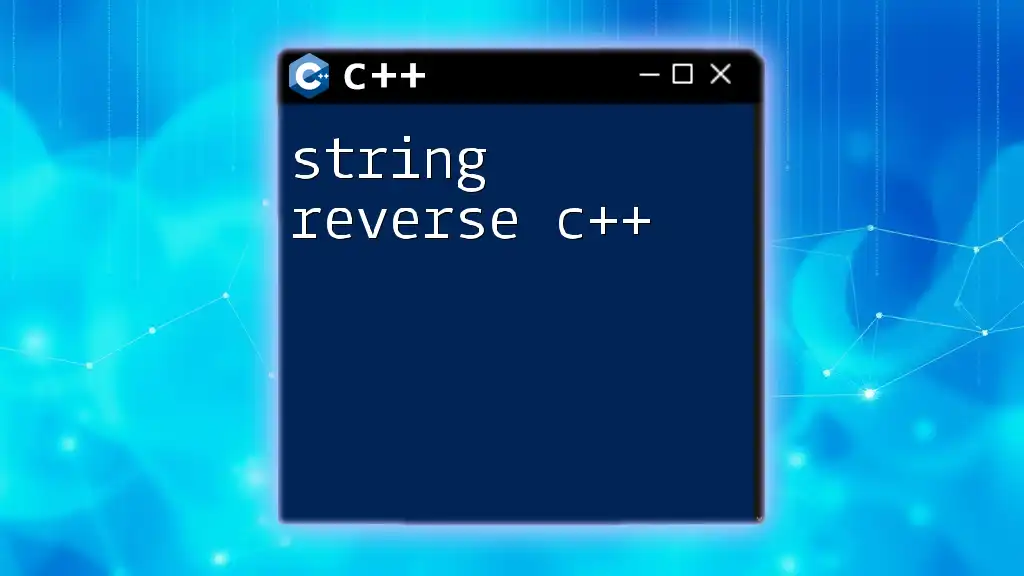
Additional Resources
For further learning, consider exploring the following resources:
- C++ Reference: Detailed documentation for standard functions and classes.
- Online tutorials that delve into string manipulation, input/output, and error handling.
- Recommended books on C++ programming to deepen your understanding of the language.