In C++, `const double` is used to define a constant variable of type double, meaning its value cannot be changed once initialized.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
int main() {
const double pi = 3.14159;
std::cout << "The value of pi is: " << pi << std::endl;
// pi = 3.14; // This line would cause a compile-time error
return 0;
}
Understanding `const` Keyword
What Does `const` Mean?
The `const` keyword in C++ indicates that a variable's value cannot be changed after its initial assignment. This concept of immutability ensures that once you define a constant, it retains its value throughout its scope, enhancing data reliability. By enforcing immutability, the `const` keyword helps developers avoid unintended side effects and improves the overall robustness of the code.
Benefits of Using `const`
Utilizing `const double` makes your code clearer. By defining numbers that should not change, you communicate your intentions more explicitly to others reading or maintaining your code. This clarity can greatly simplify debugging efforts, making it easier to identify potential issues.
Moreover, using constants can lead to compiler optimizations. When the compiler recognizes a value as constant, it can make specific optimizations, possibly improving runtime efficiency. This ensures that your application runs smoother and faster.
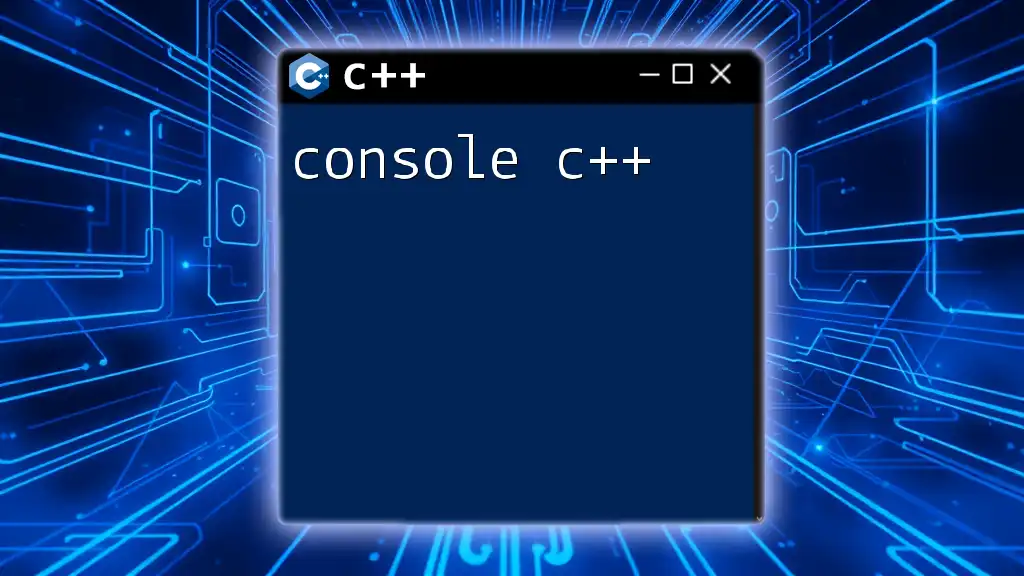
Usage of `const double`
Declaring `const double`
Declaring a `const double` is straightforward and follows a simple syntax. You specify the `const` keyword, followed by the type, the variable name, and its value.
Here's a basic example:
const double PI = 3.14159;
In this example, `PI` is a constant and its value cannot be changed throughout its lifetime.
Scenarios for Using `const double`
Mathematical Constants
Using `const double` is particularly useful for defining mathematical constants like `PI`, which are used in calculations. For instance, when calculating the area of a circle, you can utilize `PI` as follows:
double areaOfCircle(double radius) {
return PI * radius * radius;
}
Fixed Configuration Values
Constants are also essential when you have configuration settings that should remain unchanged. For example, if you want to define the maximum speed of a vehicle:
const double MAX_SPEED = 120.5;
This ensures that `MAX_SPEED` retains its value across various parts of your code, preventing accidental modifications that could lead to unexpected behavior.
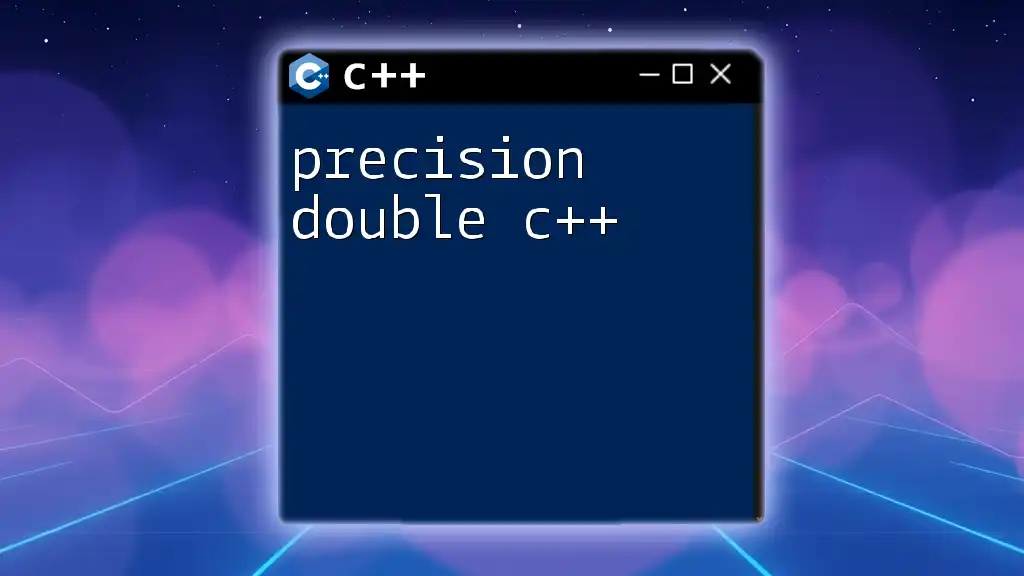
Scope and Lifetime of `const double`
Local vs Global Constants
The scope of your variables plays a crucial role in their usability. A local constant only exists within the function or block where it was declared, while a global constant is accessible throughout the program.
Here's a demonstration of a local constant:
const double LOCAL_VALUE = 20.0;
void func() {
const double FUNC_VALUE = 10.0;
// FUNC_VALUE is accessible here
}
In this case, `FUNC_VALUE` is unique to `func()` and cannot be accessed outside of it.
Static Constants
The `static` keyword can be combined with `const` to create `static const double` variables. A static constant retains its value and scope across different function calls, but is not visible outside the file.
Here's how you might declare a static constant:
static const double GRAVITY = 9.81;
Benefits of Static Constants:
Using static constants can be especially beneficial for constants that should not change and are not meant to be accessed externally. This enhances encapsulation and helps to avoid naming conflicts.
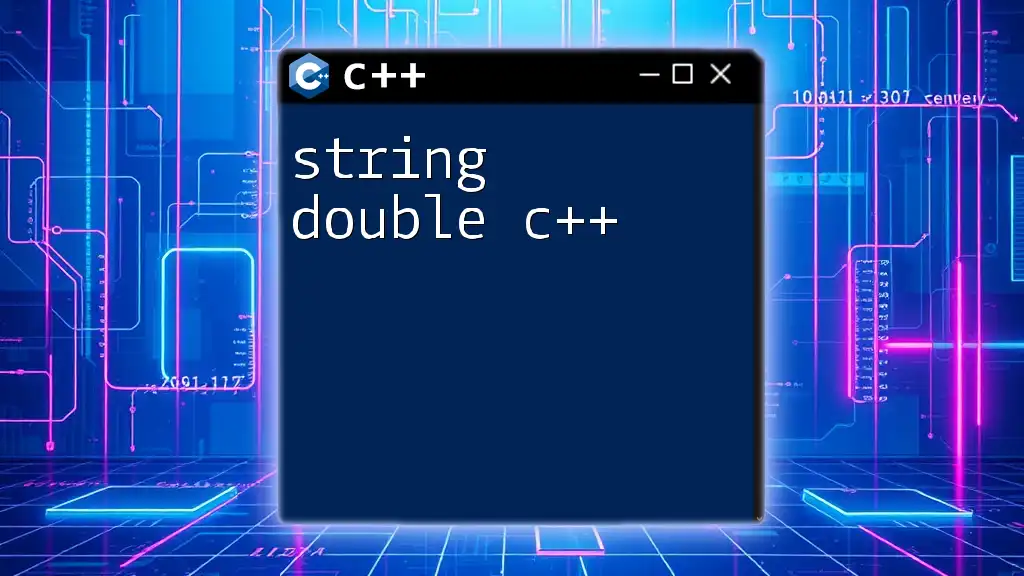
Best Practices for Using `const double`
When to Choose `const double` Over Regular `double`
When defining values that are known to be constant, opting for `const double` is a best practice. Such usage includes mathematical constants, configuration values, or any other scenario where the value's immutability is essential.
Potential Pitfalls
Overusing or mistakenly using `const` can lead to complications. For example, declaring a variable `const` that should be changeable can hinder functionality and lead to logic errors. It’s crucial only to use `const` when it makes sense and adds value to your code.
Code Snippet Example
Here’s a complete code example demonstrating the concept of `const double` in action:
#include <iostream>
const double FEET_TO_METERS = 0.3048;
double convertFeetToMeters(double feet) {
return feet * FEET_TO_METERS;
}
int main() {
double feet = 10.0;
std::cout << feet << " feet is " << convertFeetToMeters(feet) << " meters." << std::endl;
return 0;
}
In this example, the `convertFeetToMeters` function uses a constant factor, `FEET_TO_METERS`, to accurately convert feet to meters, showcasing efficient and clear coding practices.
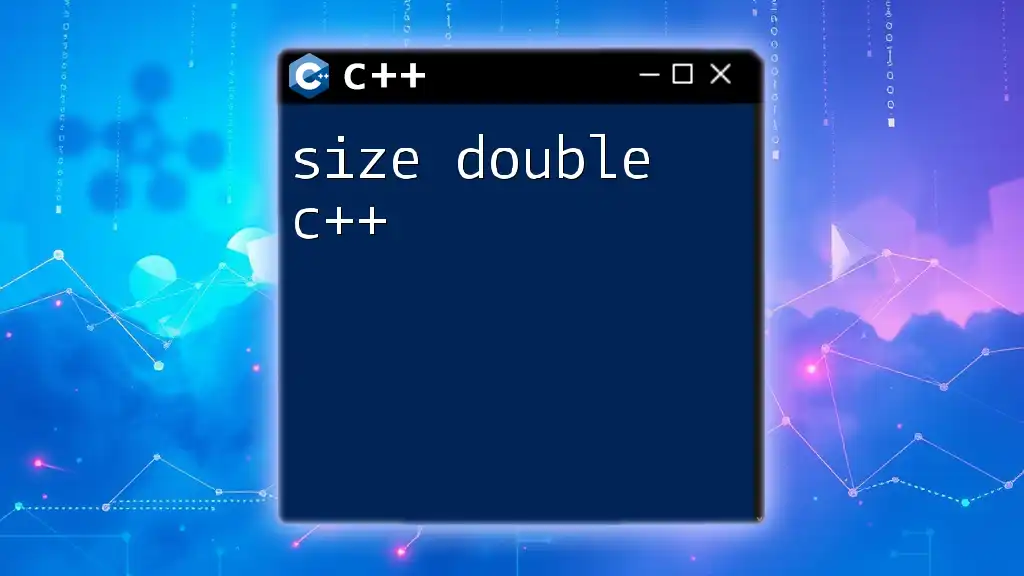
Common Mistakes with `const double`
Misunderstanding Scope and Lifetime
A frequent error occurs when programmers misunderstand the scope and lifetime of constants. For example, attempting to access a local `const double` outside its function leads to compilation errors. Being aware of where constants can and cannot be accessed is key to effective C++ programming.
Modifying `const double`
Attempting to change a `const double` will result in a compilation error. For instance:
const double TEMP = 100.0;
// TEMP = 200.0; // Compilation error
This strict enforcement prevents accidental modifications that could result in unstable applications, highlighting the importance of using `const` in your code.
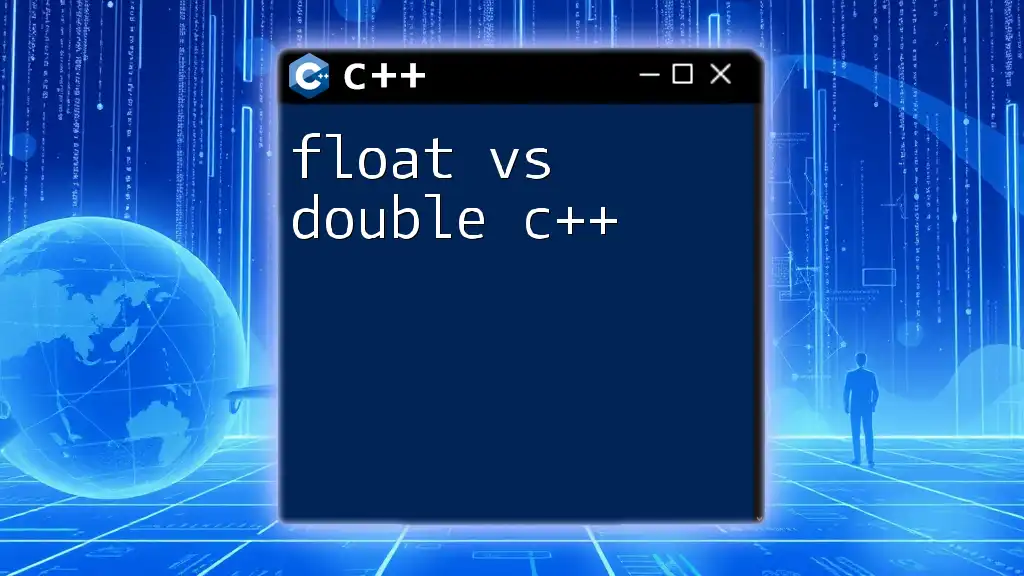
Conclusion
In summary, understanding and properly utilizing `const double` in C++ can significantly enhance the clarity, reliability, and performance of your code. By defining constants, you prevent accidental changes, communicate your intentions clearly, and allow compilers to optimize your code for efficiency.
Embrace the use of `const double` in your projects, and watch your programming practices improve tremendously. For further exploration, consider diving into additional resources or tutorials that enhance your understanding of constant variables in C++.
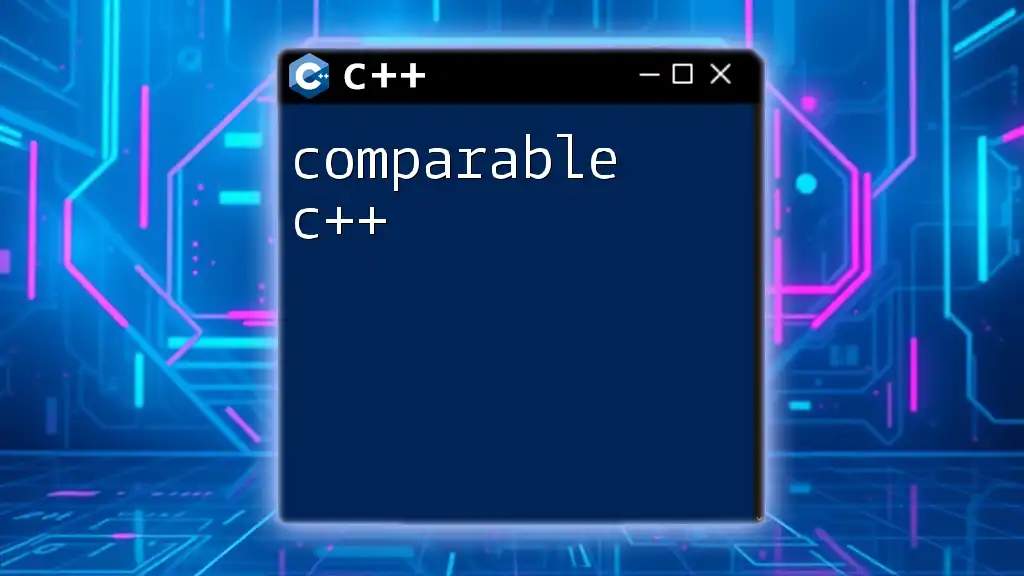
Additional Resources
For more information, you can refer to the C++ reference documentation on constants. Additionally, exploring recommended books and courses will further strengthen your C++ programming skills.
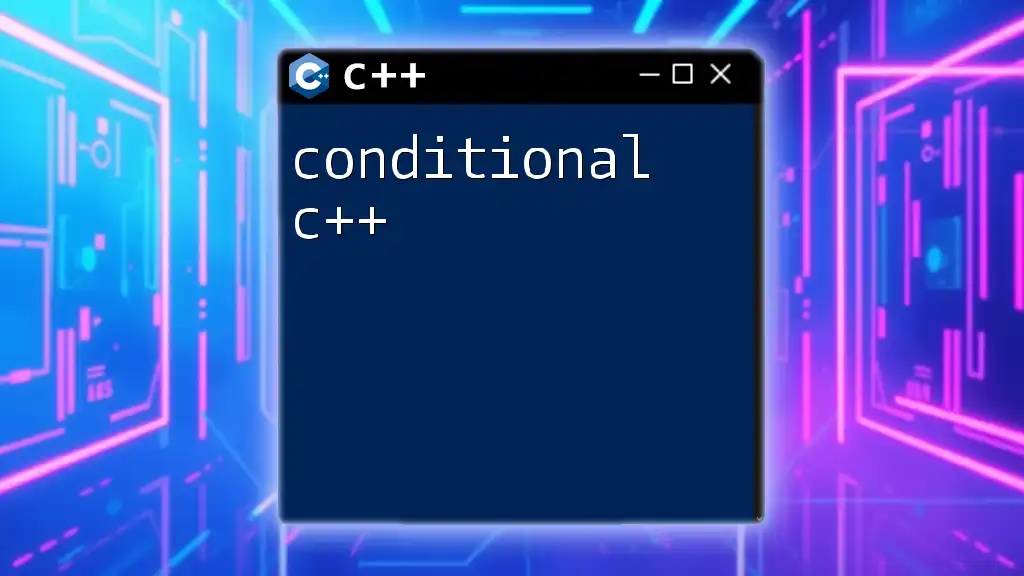
FAQs
Consider adding a section on frequently asked questions to address common concerns or misconceptions that beginners might have regarding `const double`. This can serve as a helpful guide for new learners.