In C++, `const` indicates that a variable's value cannot be changed, while `noexcept` specifies that a function does not throw exceptions, enhancing performance by avoiding unnecessary exception-handling overhead.
Here’s a code snippet demonstrating both concepts:
#include <iostream>
class MyClass {
public:
const int value;
MyClass(int val) : value(val) {}
void display() const noexcept {
std::cout << "Value: " << value << std::endl;
}
};
int main() {
MyClass obj(10);
obj.display();
return 0;
}
Understanding const in C++
What is const?
In C++, the `const` keyword is a modifier used to declare constants, which are variables whose values cannot be changed after their initialization. When a variable is declared as `const`, any attempt to modify its value will lead to a compilation error. This feature is crucial for maintaining immutability and ensuring that your code behaves predictably.
For example:
const int x = 10;
// Trying to modify x will result in an error
// x = 15; // Error: cannot modify a const variable.
Benefits of using const
Utilizing `const` in your code provides several advantages:
- Prevents accidental modification: Declaring variables as `const` helps protect against changes that could inadvertently introduce bugs.
- Enhances code optimization: Compilers can optimize better when they know that certain variables will not change. This helps improve runtime performance.
- Improves safety in multithreaded environments: By marking shared variables as `const`, you can avoid race conditions that arise from concurrent write operations.
Const with Pointers and References
The `const` keyword can also be applied to pointers and references, leading to varying semantics:
- Const pointers: Allows the pointer to point elsewhere, but the value at the pointed location cannot be modified.
- Pointer to const: The pointed value can be modified, but the pointer cannot change its address.
- Const pointer to const: Neither the pointer can be reassigned nor the pointed value modified.
Consider this code example:
int value = 20;
const int* ptr = &value; // Can read but not modify
int* const cptr = &value; // Can modify value but not reassign ptr
Using these const qualifiers correctly can clarify intent and improve code reliability.
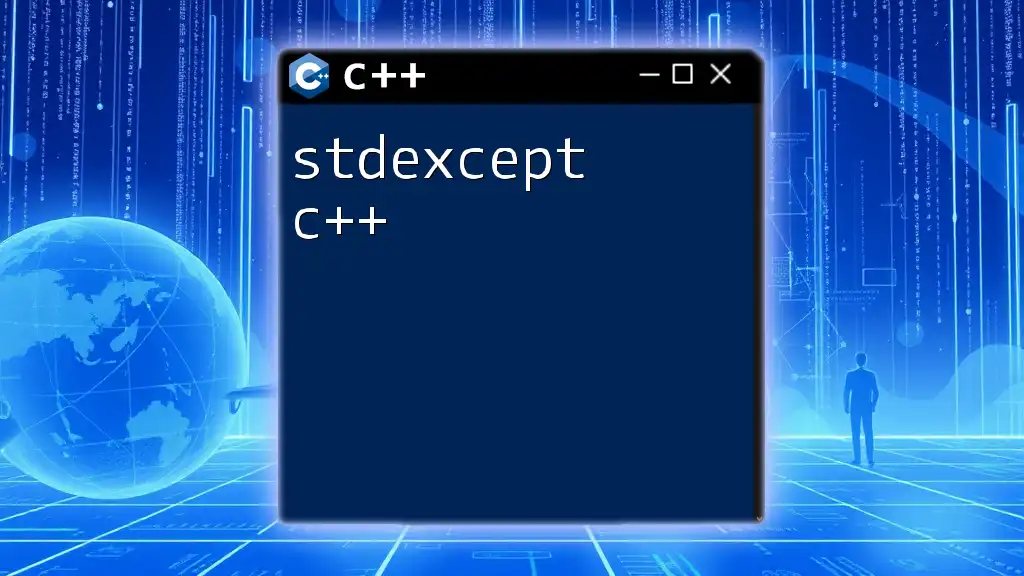
Understanding noexcept in C++
What is noexcept?
The `noexcept` specifier is a relatively newer addition to C++. It is used to indicate that a particular function is guaranteed not to throw exceptions. By marking functions as `noexcept`, developers signal their intent, improving both performance and reliability.
When to Use noexcept
Employing `noexcept` can yield significant performance advantages. Functions declared as `noexcept` allow the compiler to optimize exception handling more efficiently. For instance, operations like `std::vector` can avoid unnecessary checks for exceptions during runtime.
Here’s a simple example of a `noexcept` function:
void function() noexcept {
// Function guarantee: won't throw exceptions
}
Checking the noexcept status
You can check whether a function is `noexcept` using the `noexcept` operator. This operator returns `true` if the expression inside is declared as `noexcept`:
constexpr bool is_noexcept = noexcept(function());
This can be particularly useful in template programming or for generic functions where runtime behavior is conditionally dependent.
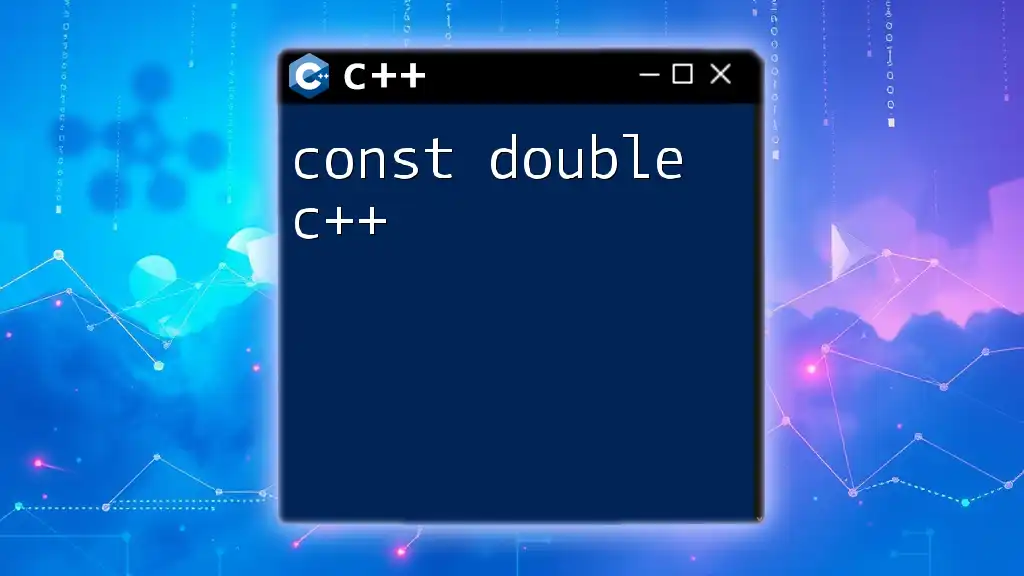
The Combination of const and noexcept
Why combine const and noexcept?
Combining `const` and `noexcept` provides an effective way to enhance your code’s safety and performance. Declaring member functions as both `const` and `noexcept` indicates that they will not modify the state of the object and will not throw exceptions. This can help you create cleaner interfaces with robust error handling, and it often yields performance improvements.
Consider the following example:
class MyClass {
public:
const int getValue() const noexcept {
return value;
}
private:
int value = 42;
};
In this case, the `getValue` function promises not to alter the object's state or throw exceptions, making it a safe choice for users accessing it.
Benefits of const noexcept member functions
Using `const noexcept` member functions has several benefits:
- Improved performance in STL containers and algorithms: The Standard Template Library (STL) can leverage `noexcept` guarantees to optimize memory management and reduce overhead during operations.
- Better integration with modern C++ features: As the C++ standard evolves, using these keywords can help you adopt new features that further leverage safety and performance.
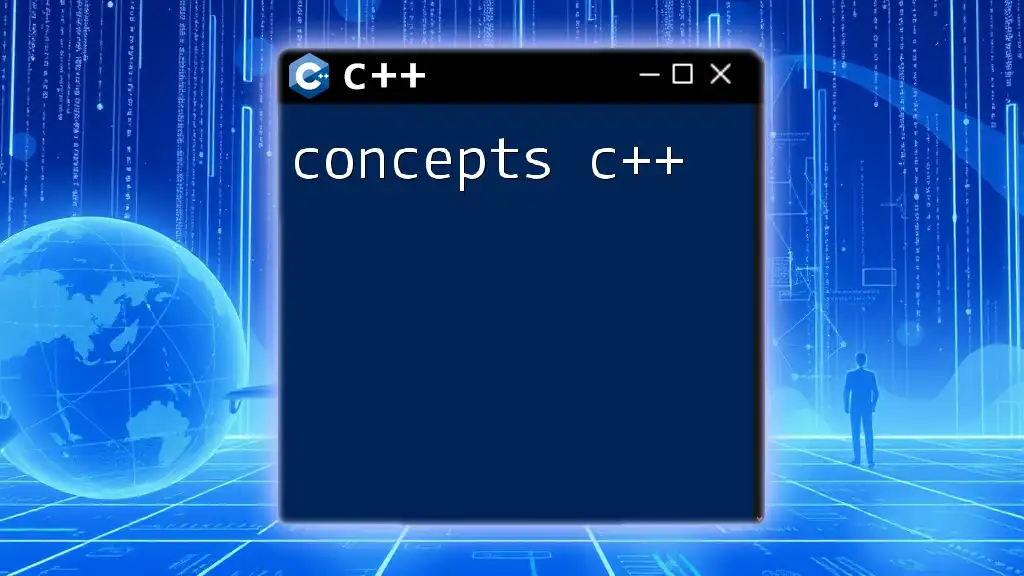
Best Practices for Using const noexcept
When to use const
To maximize the benefits of `const`, consider these guidelines:
- Always declare objects and parameters `const` when they do not need to be modified.
- Use `const` with pointers and references to protect against unintentional changes.
- Avoid applying `const` excessively, as doing so can lead to code that is harder to read.
When to use noexcept
Understanding when to mark functions as `noexcept` is equally important:
- Functions that do not perform operations that could throw exceptions, such as simple getter methods or functions that involve no dynamic memory allocation, should generally be declared `noexcept`.
- However, avoid marking functions as `noexcept` when it's not reasonable, such as when dealing with operations that can throw (like memory allocation) to avoid unexpected behavior.
Performance Considerations
The correct application of `const` and `noexcept` not only promotes intent but also has tangible performance benefits. Functions declared with `noexcept` can minimize performance penalties caused by exception handling. Similarly, using `const` throughout your code can lead to better optimization opportunities for compilers.
For example, consider functions:
void process(const std::vector<int>& data) noexcept {
// Process data without modifying it or throwing exceptions
}
This approach encapsulates both clear semantics and performance.
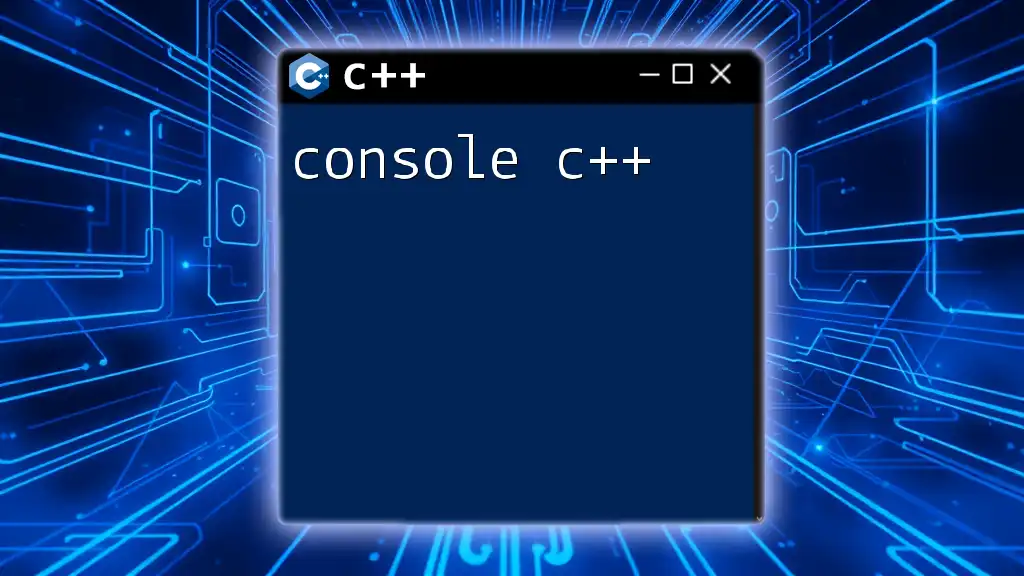
Conclusion
The combined use of `const noexcept` in C++ offers a dual approach to enhancing code quality and performance. Recognizing the significance of these keywords allows developers to write cleaner, safer, and more efficient code. As you continue to grow your mastery of modern C++, embracing `const` and `noexcept` will place you ahead in crafting high-quality software solutions.
Fostering an in-depth understanding of these concepts will help streamline your coding practices. For those looking to dive deeper, a variety of resources are available, from authoritative texts on C++ standards to engaging community forums where experienced developers share their insights and tips.