`stdexcept` in C++ provides a standardized way to report errors via exceptions, enabling safer and cleaner error handling throughout your code.
Here's a simple code snippet demonstrating the use of `std::runtime_error` from the `stdexcept` header:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error& e) {
std::cerr << "Caught exception: " << e.what() << std::endl;
}
return 0;
}
What is stdexcept?
The `stdexcept` header in C++ provides a set of standard exception classes meant specifically for error handling. These classes help to signal that an exceptional situation has occurred and manage errors in a clean and structured manner. Using `stdexcept` allows programmers to separate error handling from the main logic of their code, making it easier to read and maintain.
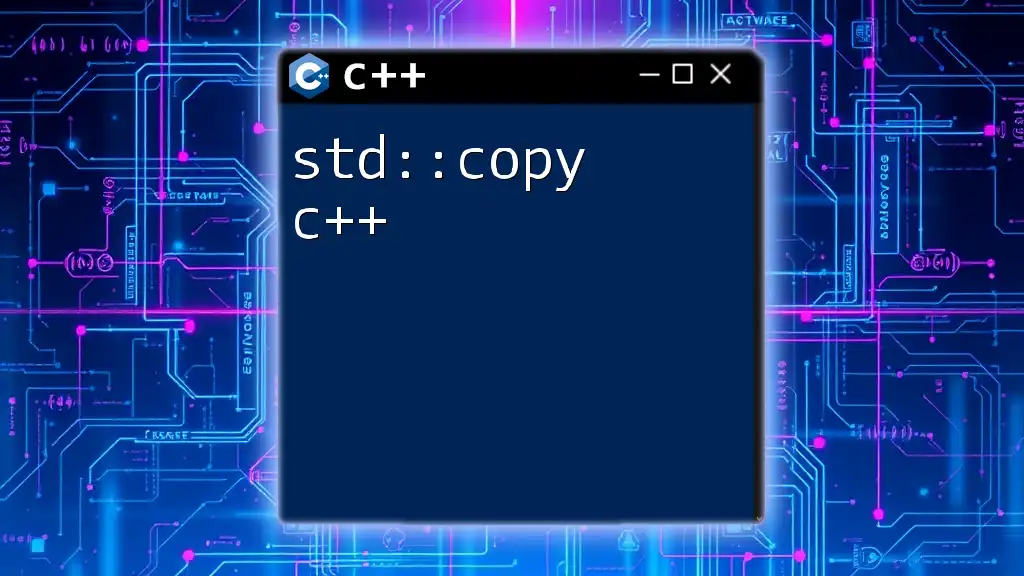
Why Use stdexcept?
Using `stdexcept` introduces significant benefits to your C++ programs:
- It standardizes error handling, making it easier for other programmers to understand exceptions in your code.
- It enhances code readability and maintainability, as common error conditions are encapsulated within standard exception classes.
- It allows for better debugging, since the semantics of each exception class provide meaningful messages related to the type of error encountered.
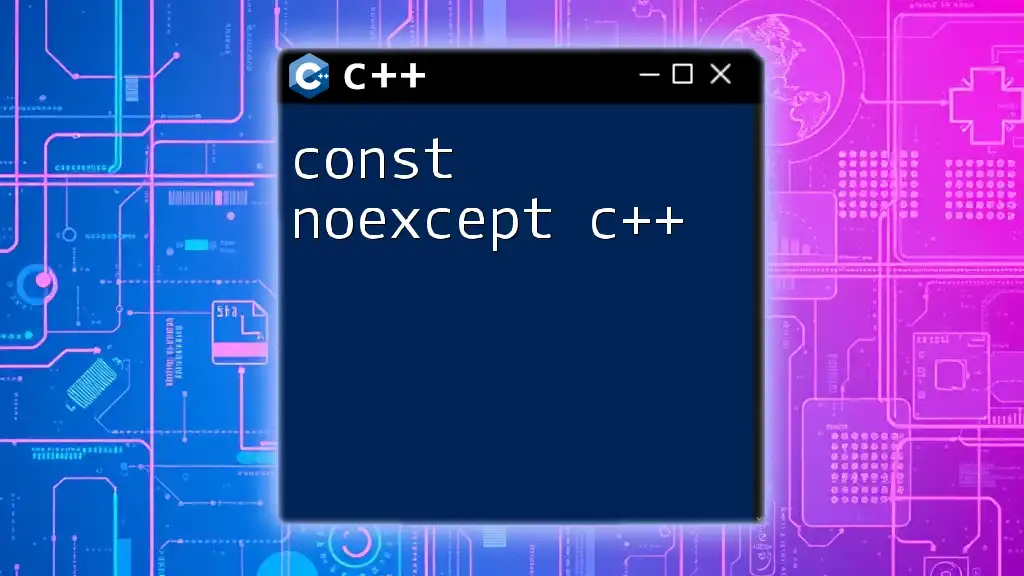
Understanding Exceptions in C++
What Are Exceptions?
An exception in C++ is a runtime error condition that disrupts the normal flow of a program's execution. When an error occurs, an exception is thrown, and the control flow will go to the nearest catch block that can handle it. This mechanism helps you separate error handling code from regular logic, making your programs cleaner.
The Exception Handling Mechanism
C++ employs a simple yet powerful error handling structure utilizing `try`, `catch`, and `throw`:
- try: This block contains code that may potentially throw an exception.
- throw: This statement triggers an exception, signaling that an error condition exists.
- catch: This block catches exceptions that are thrown, allowing you to handle them gracefully.
In essence, the flow when an exception is encountered is as follows: the program will jump from the point of the exception (where it is thrown) to the nearest catch block, where the error can be processed.
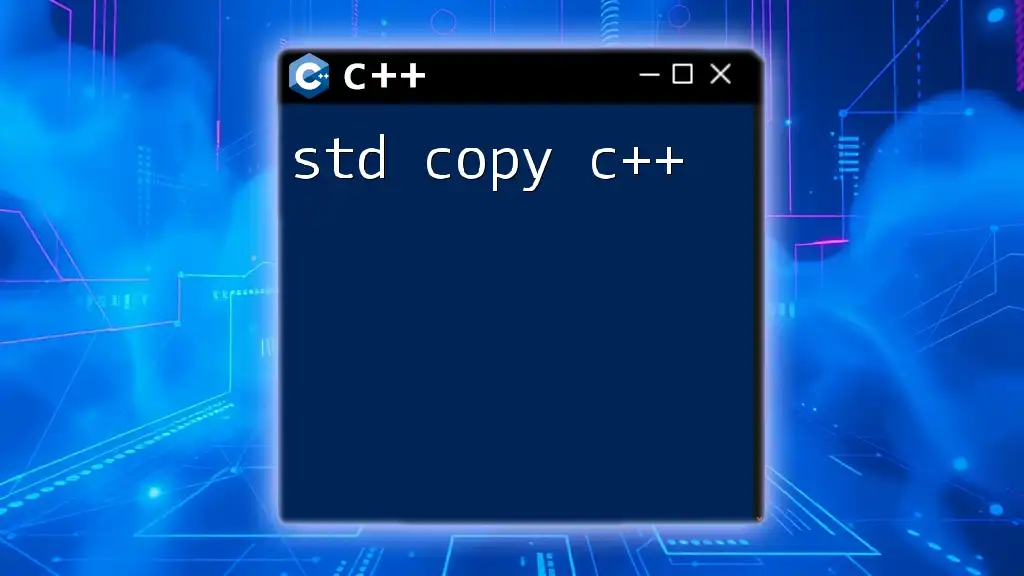
Overview of stdexcept
What is stdexcept?
The `stdexcept` header defines standard exception classes that are intended to provide a clear and standard way to handle exceptional circumstances. These exceptions inherit from `std::exception`, which is the base class for all standard exceptions in C++.
Standard Exception Classes
The exception classes defined in `stdexcept` are structured into a hierarchy, covering both logic and runtime errors. This categorization helps developers choose the right type of exception for their specific needs.
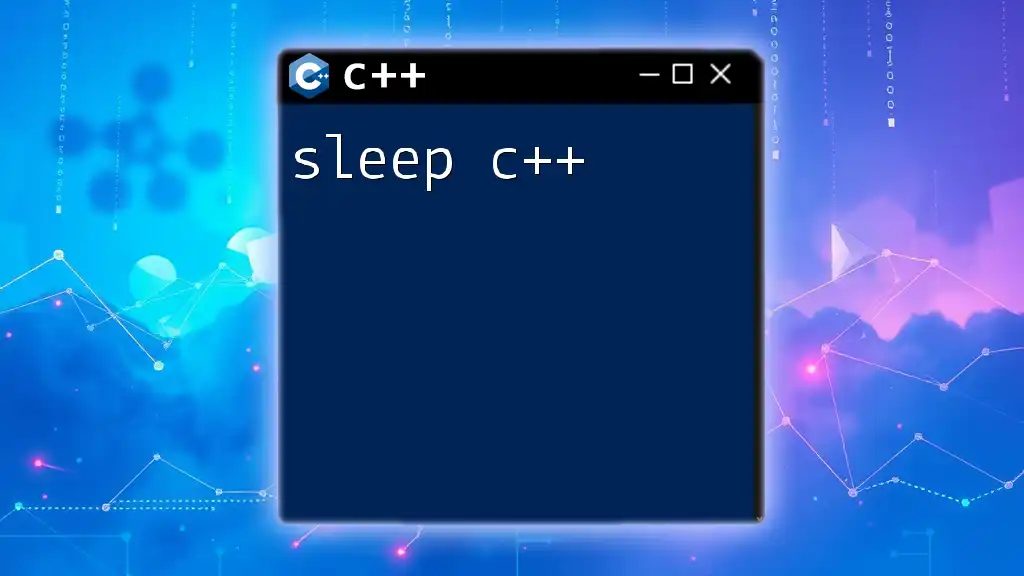
Common Types of Exceptions in stdexcept
Logic_error
The `logic_error` class is used to signal errors in the internal logic of a program. It should be thrown when the program’s logic is violated.
Example code snippet:
#include <iostream>
#include <stdexcept>
void testLogicError() {
throw std::logic_error("This is a logic error!");
}
int main() {
try {
testLogicError();
} catch (const std::logic_error& e) {
std::cerr << e.what() << '\n';
}
}
In this example, the function `testLogicError` throws a `logic_error`. It's caught in `main`, where the error message is printed out.
Runtime_error
The `runtime_error` exception is intended for errors that occur while the program is running, which are usually outside the programmer's control.
Example code snippet:
#include <iostream>
#include <stdexcept>
void testRuntimeError() {
throw std::runtime_error("This is a runtime error!");
}
int main() {
try {
testRuntimeError();
} catch (const std::runtime_error& e) {
std::cerr << e.what() << '\n';
}
}
This code similarly captures and outputs the runtime error to the console.
Invalid_argument
The `invalid_argument` exception is used to indicate that an invalid argument was passed to a function, which can prevent it from executing successfully.
Example code snippet:
#include <iostream>
#include <stdexcept>
void validateInput(int value) {
if (value < 0)
throw std::invalid_argument("Negative value not allowed.");
}
int main() {
try {
validateInput(-1);
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << '\n';
}
}
Here, trying to validate a negative number leads to an `invalid_argument` exception being thrown and subsequently handled.
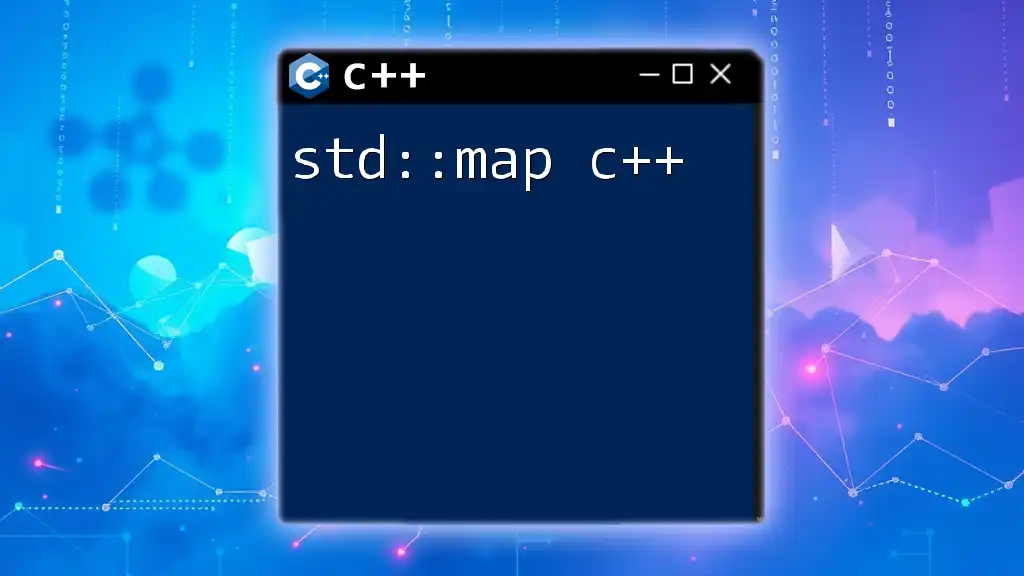
Customizing Exceptions
Creating Your Own Exception Class
Sometimes, the standard classes may not cover a specific situation in your program. In such cases, you can create custom exceptions by inheriting from `std::exception`.
Example code snippet:
#include <exception>
#include <iostream>
class MyException : public std::exception {
const char* what() const noexcept override {
return "My custom exception occurred!";
}
};
int main() {
try {
throw MyException();
} catch (const MyException& e) {
std::cerr << e.what() << '\n';
}
}
This custom exception class `MyException` provides a specific error message when it is caught, allowing tailored error handling.
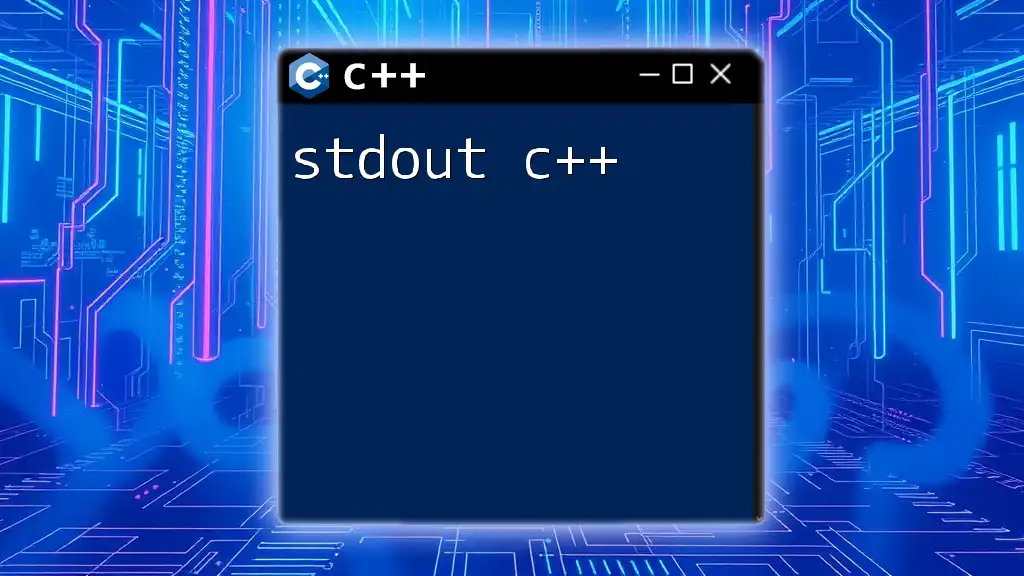
Best Practices for Using stdexcept
When to Throw Exceptions
Throw exceptions when encountering situations that cannot be handled locally. This includes invalid inputs, state violations, or operations that exceed operational boundaries. Remember, throwing exceptions should not be used for control flow; they should only be used for unexpected errors.
Catching Exceptions Effectively
It's crucial to catch exceptions as specifically as possible. Catching specific exception types is preferable since it allows you to handle various error conditions differently. For example:
try {
// Code that may throw exceptions
} catch (const std::logic_error& e) {
// Handle logic error
} catch (const std::runtime_error& e) {
// Handle runtime error
}
Re-throwing Exceptions
Re-throwing exceptions is also useful, especially when you want to perform some cleanup or logging before propagating an error further up the call stack. Using the `throw;` statement within a catch block allows this behavior.
Example code snippet:
try {
// Code that may throw exceptions
} catch (const std::exception& e) {
// Log the error
std::cerr << "Error occurred: " << e.what() << '\n';
throw; // Re-throw the exception
}
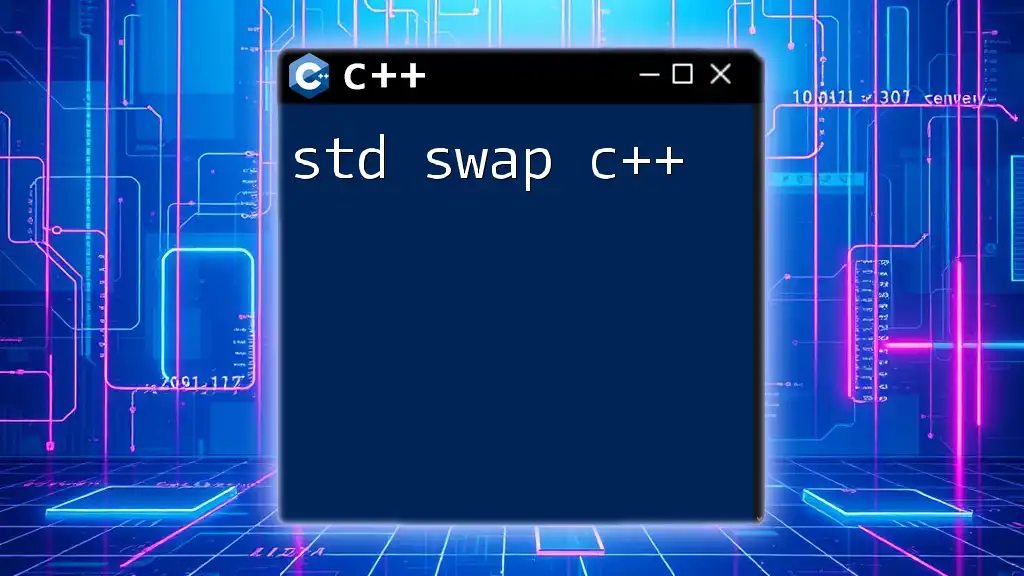
Conclusion
Understanding and implementing `stdexcept` in C++ is essential for robust exception handling in your applications. By leveraging the standardized exception classes provided by `stdexcept`, you can create cleaner, more maintainable, and more readable code. Embrace these tools to enhance your programming practice and improve the error-handling mechanisms within your applications.
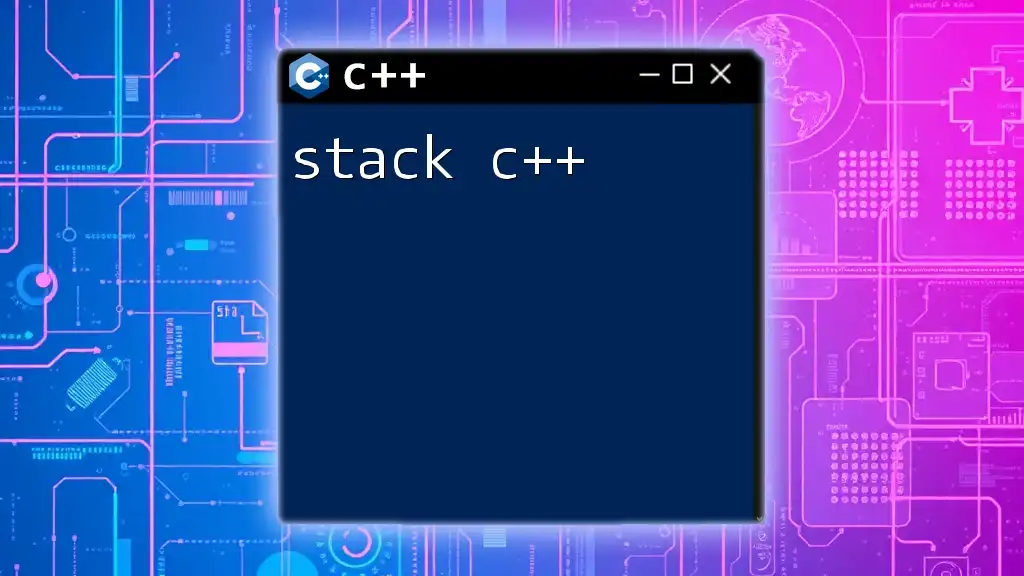
References for Further Reading
Books and Resources
- Look for books focused on C++ programming and design patterns that include sections on exception handling.
- Explore online platforms that offer C++ courses, with specific modules dedicated to exception handling.
Documentation
- Official C++ documentation provides an in-depth exploration of `stdexcept` and standard C++ exceptions, making it a valuable resource for any programmer wishing to enhance their understanding of error handling in C++.