The `extern "C"` declaration in C++ is used to indicate that the following declarations should use C linkage, allowing C++ code to call C functions seamlessly.
extern "C" {
void myFunction(int a);
}
What is the Extern Keyword in C++?
The `extern` keyword in C++ is a declaration specification that indicates a variable or function is defined in another translation unit, allowing it to be accessible across multiple files. This is crucial for establishing links between different components of a program, especially in larger C++ applications that are built using multiple source files.
By marking a variable or function as `extern`, you inform the compiler that the definition exists elsewhere, thus preventing it from attempting to allocate storage for it in the current translation unit. This could be viewed as a way to communicate across file boundaries.
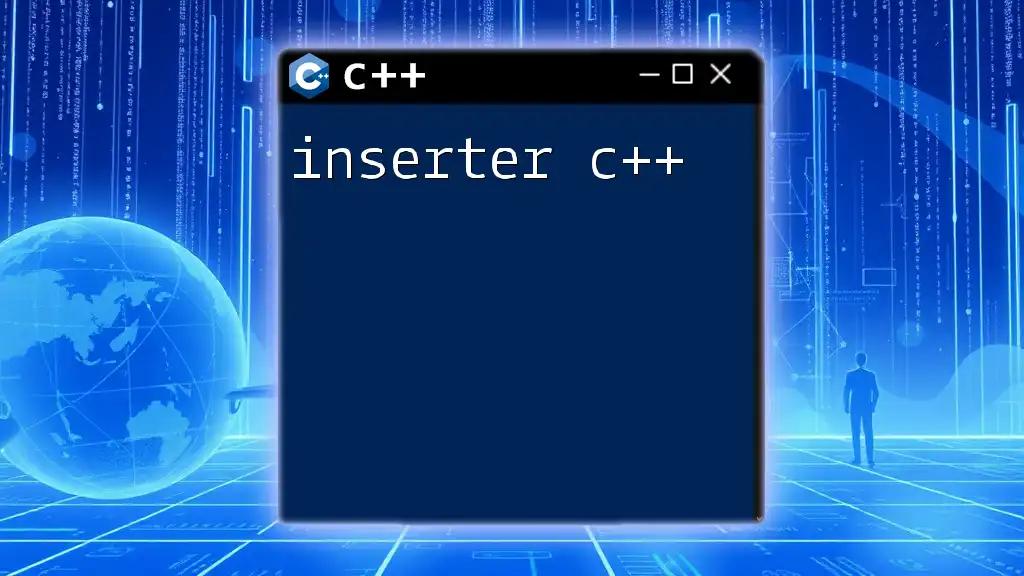
How to Use the Extern Keyword in C++
Declaring Extern Variables in C++
To declare an `extern` variable, you simply prefix its declaration with the `extern` keyword. This indicates that the variable's actual storage is defined in another file.
Syntax:
extern type variableName;
Example:
// file1.cpp
int myVariable = 5; // Definition of the variable
// file2.cpp
extern int myVariable; // Declaration of the variable
In this example, `myVariable` is defined in `file1.cpp` and declared in `file2.cpp`. When `file2.cpp` is compiled, it can refer to `myVariable` without allocating additional storage. This is especially useful when you need to share variables across multiple files.
Using Extern Functions in C++
Similarly, functions can also be declared as `extern`. This is useful when you want to call a function defined in another source file.
Syntax:
extern return_type functionName(parameters);
Example:
// file1.cpp
void myFunction() {
// Function code here
}
// file2.cpp
extern void myFunction(); // Declaration of the function
int main() {
myFunction(); // Call to the external function
}
In this example, `myFunction` is defined in `file1.cpp`, and it can be invoked in `file2.cpp` thanks to the `extern` declaration. Having functions declared as `extern` helps you organize code cleanly and keep implementations separate from interfaces.

Benefits of Using the Extern Keyword in C++
Modular Programming
One of the fundamental benefits of using the `extern` keyword is its ability to facilitate modular programming. By declaring variables and functions as `extern`, you can split your code into manageable modules or files, each responsible for a specific functionality. This makes the overall codebase easier to navigate, maintain, and debug.
Moreover, developing a program in modules encourages the reusability of code. You can easily transfer modules across different projects or applications without redundancy.
Avoiding Multiple Definitions
Using the `extern` keyword also prevents multiple variable definitions. In C++, defining a variable in multiple files can result in linker errors (specifically, the "multiple definition" error). By using `extern`, you define the variable in one file and declare it in others, ensuring that there’s only one definition while still enabling access.
Example:
// file1.cpp
int globalVariable = 10; // Definition
// file2.cpp
extern int globalVariable; // Declaration
// file3.cpp
extern int globalVariable; // Declaration
In this setup, `globalVariable` is defined only once in `file1.cpp`, and both `file2.cpp` and `file3.cpp` declare it as `extern`. If you were to define `globalVariable` in both `file2.cpp` and `file3.cpp`, you would encounter linker errors.

Common Misconceptions about Extern in C++
Extern vs Static in C++
A common misconception is confusing `extern` with `static`. While both keywords deal with linkage and visibility, they serve different purposes. The `extern` keyword allows variables and functions to be visible outside their translation unit, making them accessible from other files. In contrast, the `static` keyword restricts visibility to the file it is declared in, preventing external access entirely.
Example:
// file1.cpp
static int localVariable = 100; // Not visible outside this file
// file2.cpp
extern int localVariable; // This will cause an error
This distinction is crucial when considering how and where to access data and functions in larger applications.
Extern Variables and Scope
Another misconception is regarding the scope of `extern` variables. While `extern` allows you to access variables defined in other files, this does not change the variable's scope. The variable remains in the file where it is defined, and `extern` allows reference from other files without changing its original context.
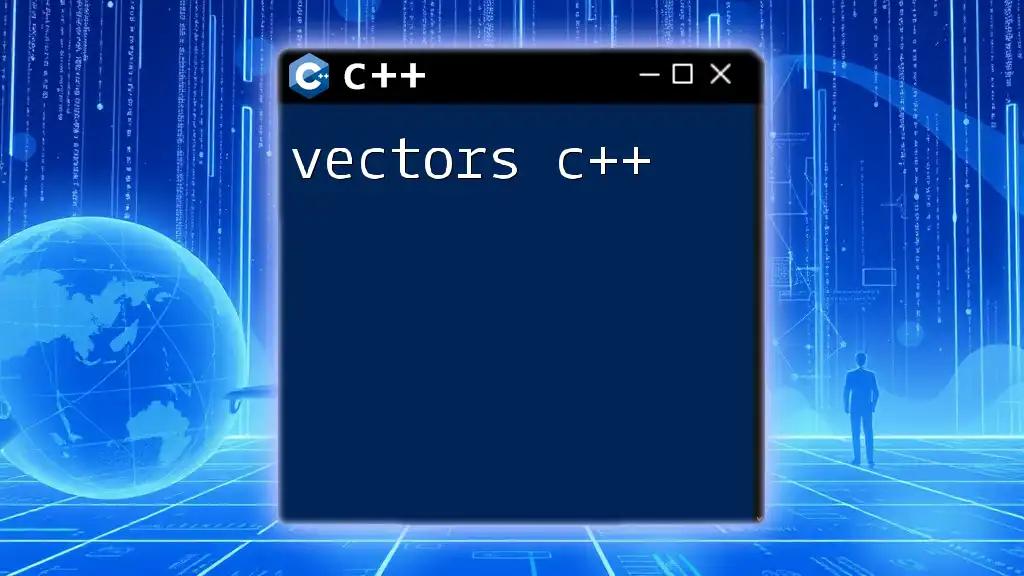
Practical Examples of Extern in C++
Example 1: Multi-file Program
Let's look at a simple multi-file program that utilizes the `extern` keyword. This example illustrates how you can utilize `extern` to share a variable across different source files.
Code Example:
// main.cpp
#include <iostream>
extern int count; // Declaration
int main() {
std::cout << "Count: " << count << std::endl; // Use extern variable
return 0;
}
// count.cpp
int count = 10; // Definition of the variable
In this example, `main.cpp` uses the `extern` declaration to use the `count` variable defined in `count.cpp`. This illustrates how `extern` facilitates communication between separate files in a C++ project.
Example 2: Extern Functions in Libraries
In C++, the `extern` keyword is especially useful when creating function prototypes for libraries. This allows for a clean separation of the function’s interface and its implementation, promoting better coding practices.
Example:
// library.cpp
#include <iostream>
void printMessage() {
std::cout << "Hello from the library!" << std::endl;
}
// main.cpp
#include <iostream>
extern void printMessage(); // Declaration
int main() {
printMessage(); // Call to the external function
return 0;
}
Here, `library.cpp` defines the `printMessage` function, while `main.cpp` declares it using `extern`. This allows the `main` function to call `printMessage` seamlessly, demonstrating how `extern` can be used to build modular applications.

Best Practices When Using Extern in C++
Naming Conventions
When you are working with `extern`, following proper naming conventions is crucial to avoid conflicts. A good practice is to use unique prefixes for global variables or to use namespaces, which can help minimize the possibility of conflict.
Documenting External Declarations
Documentation plays a critical role in code maintainability. Always ensure to comment on `extern` declarations to enhance code readability. This helps other developers (and future you) understand where the variable or function is defined and the intended usage, which is particularly helpful in larger teams or more extensive applications.

Conclusion
In summary, the `extern` keyword in C++ is an essential tool for managing variable and function visibility across multiple files. Understanding how to leverage it for modular programming, avoiding multiple definitions, and maintaining clarity in your code is vital for any C++ developer. Practice with `extern` will enhance your coding skills and help you build more robust and maintainable applications.
To dive deeper into the world of C++, explore more about the intricacies of its features and continuously improve your coding proficiency through hands-on practice!