"Modern C++ books focus on teaching the latest C++ standards (C++11, C++14, C++17, C++20) through clear examples and efficient coding practices."
Here’s a simple example of a C++ lambda function, which is a feature introduced in C++11:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using a lambda to calculate the sum of the numbers
int sum = 0;
std::for_each(numbers.begin(), numbers.end(), [&](int n) { sum += n; });
std::cout << "Sum: " << sum << std::endl; // Output: Sum: 15
return 0;
}
Understanding Modern C++ Features
C++11: A Turning Point
The introduction of C++11 marked a significant evolution of the language, introducing features that improved code readability and developer productivity.
- Key Features Introduced
-
The auto keyword allows for type inference, which means the compiler automatically deduces the variable type. This leads to cleaner and more maintainable code. For example:
auto x = 5; // x is automatically deduced as int
-
Smart Pointers such as `std::unique_ptr` and `std::shared_ptr` help in effective resource management. They prevent common pitfalls like memory leaks. An example of creating a unique pointer is:
std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>();
-
The introduction of a range-based for loop simplifies the iteration over collections, reducing boilerplate code. Consider the following example:
std::vector<int> vec = {1, 2, 3, 4}; for (auto i : vec) { std::cout << i << " "; }
-
C++14 and C++17 Enhancements
C++14 and C++17 enriched the C++ language with additional functionality that further supports modern programming practices.
-
A pivotal feature is generic lambdas in C++14, allowing lambda expressions to take parameters of any type. This increases their versatility, as shown in the example below:
auto lambda = [](auto x) { return x + 10; }; std::cout << lambda(5); // Outputs 15
-
The enhancement of control structures such as if and switch with initializers provides a more streamlined and efficient way to declare and initialize variables within conditional statements.
C++20: The Latest Features
With the arrival of C++20, several groundbreaking changes have solidified modern C++ as a powerful programming language.
-
One of the most exciting advancements is modules, a new way to structure C++ programs that improves compilation speed and enhances code visibility.
-
Another notable introduction is concepts, which allows developers to specify template parameter requirements. This leads to enhanced type safety and clearer error messages at compile-time. Here's a code example:
template<typename T> concept Addable = requires(T a, T b) { { a + b } -> std::same_as<T>; };
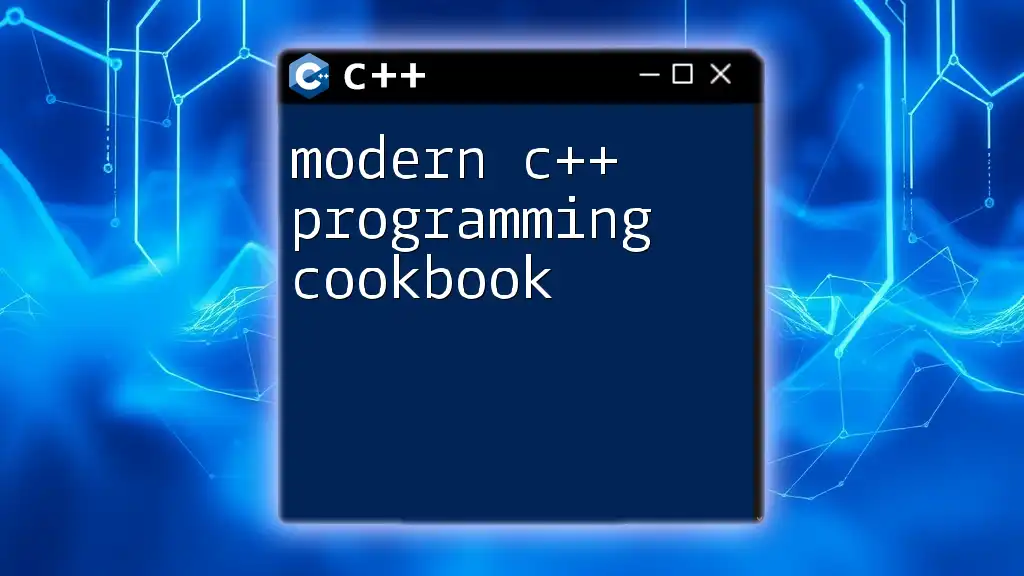
Choosing the Right Modern C++ Book
Finding the right modern C++ book is crucial for anyone looking to deepen their understanding of the language.
Key Features to Look For
When selecting a book, consider the clarity of explanation. A good book should provide clear examples and logical organization to facilitate the learning process. Additionally, ensure the content is relevant, covering features up to C++20 or later to keep you updated with the latest practices.
Recommended Books
Some standout titles include:
-
"The C++ Programming Language" by Bjarne Stroustrup: Authored by the creator of C++, this book offers an in-depth perspective on the language and its intricacies. It's perfect for gaining a comprehensive understanding.
-
"Effective Modern C++" by Scott Meyers: This book provides practical advice and best practices specifically tailored for modern C++ programming, aiding developers in writing more effective code.
Online Resources
In addition to books, tapping into official C++ documentation can provide further insights and guidance. Online forums and community resources can also be invaluable for solving specific queries.
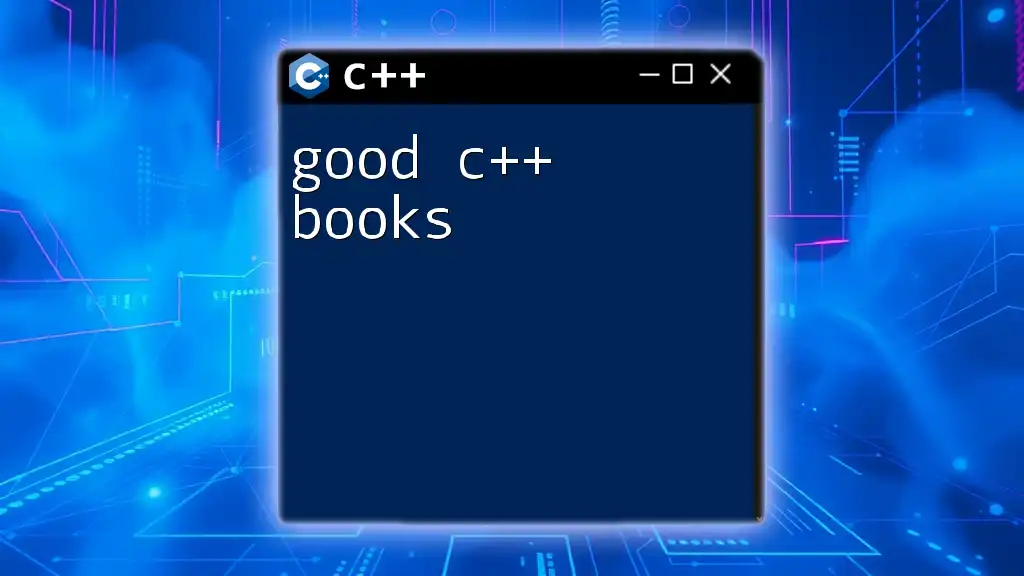
Practical Exercises
Putting theory into practice is essential for mastering modern C++. Here are practical exercises to reinforce your learning:
-
Implementing a Smart Pointer: Create a unique pointer that manages a resource within your application. This exercise will help you grasp the utility of smart pointers and highlight their role in modern C++.
-
Writing a Generic Lambda Function: Challenge yourself to create a lambda that can accept parameters of different types. This task not only tests your understanding of lambdas but also encourages you to use templates effectively.
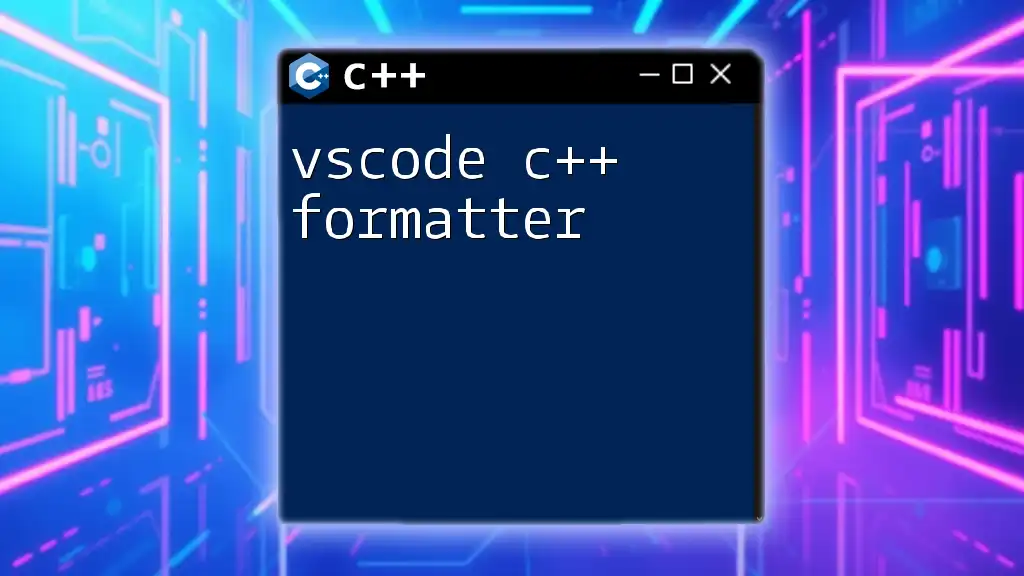
Conclusion
In conclusion, the landscape of modern C++ is continually evolving, and it’s essential to engage in continuous learning. The recommended books and resources will be invaluable as you immerse yourself in the latest features and best practices of C++. Keeping yourself informed with up-to-date materials can greatly enhance your programming skills.
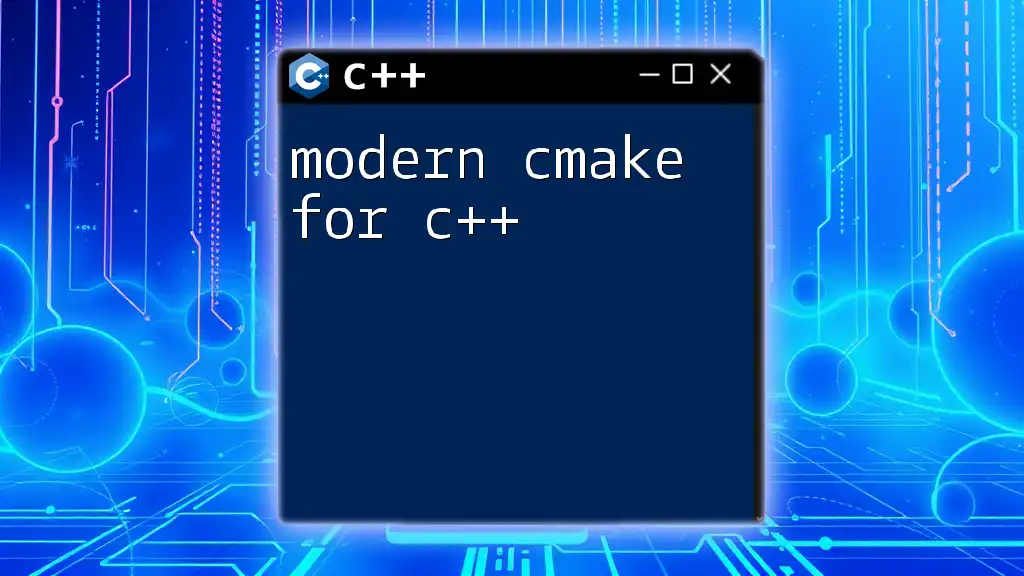
Call to Action
Join our community of aspiring C++ developers and enhance your learning journey! Share your feedback, suggestions, and any other modern C++ books you’ve found useful in the comments. Together, we can deepen our understanding of this powerful programming language!