The "Modern C++ Programming Cookbook" is a practical guide that provides concise examples of contemporary C++ features and best practices for efficient programming.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1, 2};
std::sort(numbers.begin(), numbers.end());
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
Setting Up Your Development Environment
Choosing the Right Compiler
Selecting the correct compiler is vital for a smooth C++ development experience. Three popular compilers stand out:
-
GCC (GNU Compiler Collection): A widely used open-source compiler that supports a variety of programming languages, including C++. It is extremely versatile and available on different platforms.
-
Clang: Known for its high performance and user-friendly error messages, Clang is an attractive choice, especially for developers working on macOS or Linux.
-
MSVC (Microsoft Visual C++): This is integrated into the Visual Studio IDE and provides robust support for Windows applications.
Installation instructions will depend on your operating system. For example, on Ubuntu, you can easily install GCC via terminal:
sudo apt install build-essential
Integrated Development Environments (IDEs)
Choosing a suitable IDE can enhance productivity and ease of coding. Here’s a brief look at some popular options:
-
Visual Studio: This powerful IDE offers excellent debugging tools, integrated Git support, and a vast ecosystem of plugins. It's tailored for developing Windows applications.
-
CLion: This JetBrains IDE is feature-rich, offering intelligent coding assistance, and supports CMake, which allows easy project configuration.
-
Code::Blocks: A lightweight, open-source IDE that is customizable with plugins. It is ideal for beginners who want an uncomplicated environment.
-
Visual Studio Code: A robust code editor with extensions allowing C++ development. Lightweight and highly customizable, making it ideal for cross-platform development.
Configuring your IDE optimally can make coding less error-prone and more efficient.
Setting Up Build Systems
CMake Basics
CMake is an essential cross-platform build system generator, widely used in modern C++ workflows. A basic CMake setup involves creating a `CMakeLists.txt` file in your project directory. Here’s an example of a simple CMake project configuration:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 20)
add_executable(MyExecutable main.cpp)
In this configuration:
- `cmake_minimum_required(VERSION 3.10)` sets the minimum required version for CMake.
- `project(MyProject)` specifies the project name.
- `set(CMAKE_CXX_STANDARD 20)` sets the C++ standard to C++20.
- `add_executable(MyExecutable main.cpp)` tells CMake to compile `main.cpp` into an executable named `MyExecutable`.
Using CMake not only simplifies building your projects but also maintains portability across different operating systems.
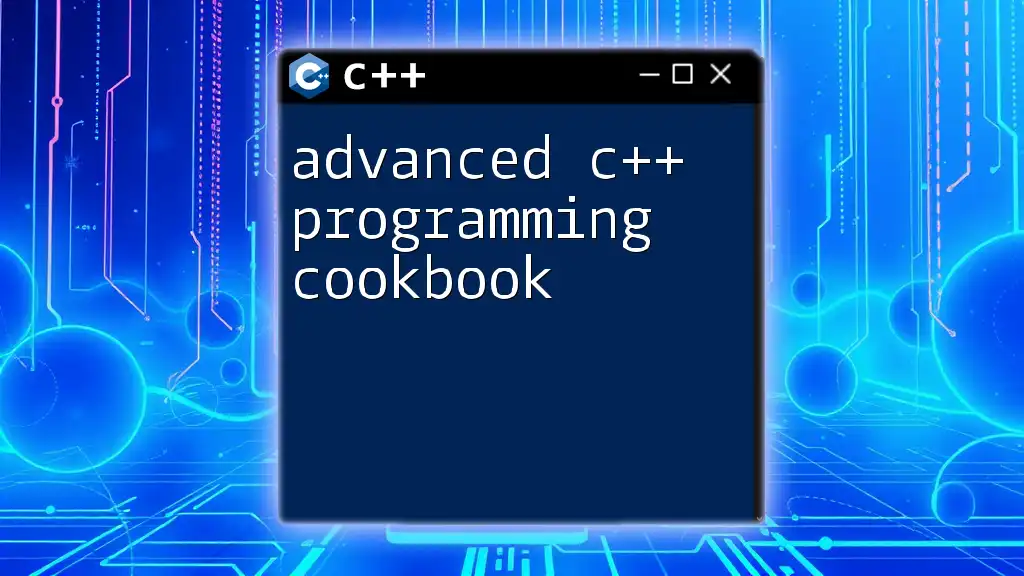
Core Concepts of Modern C++
Smart Pointers
Memory management is a crucial aspect of C++. Smart pointers are modern tools that help manage resource lifetimes automatically, reducing memory leaks and errors.
Understanding Ownership
With smart pointers, ownership can be clearly defined, allowing the program to automatically reclaim memory. Unlike raw pointers, which require explicit deletion, smart pointers handle this automatically.
Types of Smart Pointers
-
`std::unique_ptr`:
- This smart pointer exclusively holds ownership of an object. When the `std::unique_ptr` goes out of scope, the object is automatically deleted.
Example:
#include <memory> void example() { std::unique_ptr<int> ptr = std::make_unique<int>(42); // Automatically deleted when ptr goes out of scope }
-
`std::shared_ptr`:
- This smart pointer allows multiple pointers to share ownership of an object. The object is deleted once all `std::shared_ptr`s pointing to it are out of scope.
Example:
#include <memory> void example() { std::shared_ptr<int> ptr1 = std::make_shared<int>(42); std::shared_ptr<int> ptr2 = ptr1; // Both now share ownership }
-
`std::weak_ptr`:
- This pointer references an object without taking ownership, preventing reference cycles in `std::shared_ptr`.
Example:
#include <memory> void example() { std::shared_ptr<int> sp = std::make_shared<int>(42); std::weak_ptr<int> wp = sp; // Weakly references the shared pointer }
Lambda Expressions
What are Lambdas?
Lambda expressions provide a concise way to define anonymous functions directly in code. They feature the following syntax:
[captures](parameters) -> return_type { body }
Common Use Cases
Lambdas are particularly powerful when used with STL algorithms. For example:
#include <vector>
#include <algorithm>
void example() {
std::vector<int> vec{1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), [](int &n) { n *= 2; });
}
In this snippet, each number in the vector is doubled using a lambda function, demonstrating the elegance of using lambdas alongside STL.
Range-based For Loops
This feature simplifies the iteration over containers, making the code cleaner and less error-prone compared to traditional for loops.
#include <vector>
#include <iostream>
void example() {
std::vector<int> vec{1, 2, 3, 4, 5};
for (const auto& num : vec) {
std::cout << num << " ";
}
}
In this code block, `vec` is traversed directly, enabling easier reading and maintenance.
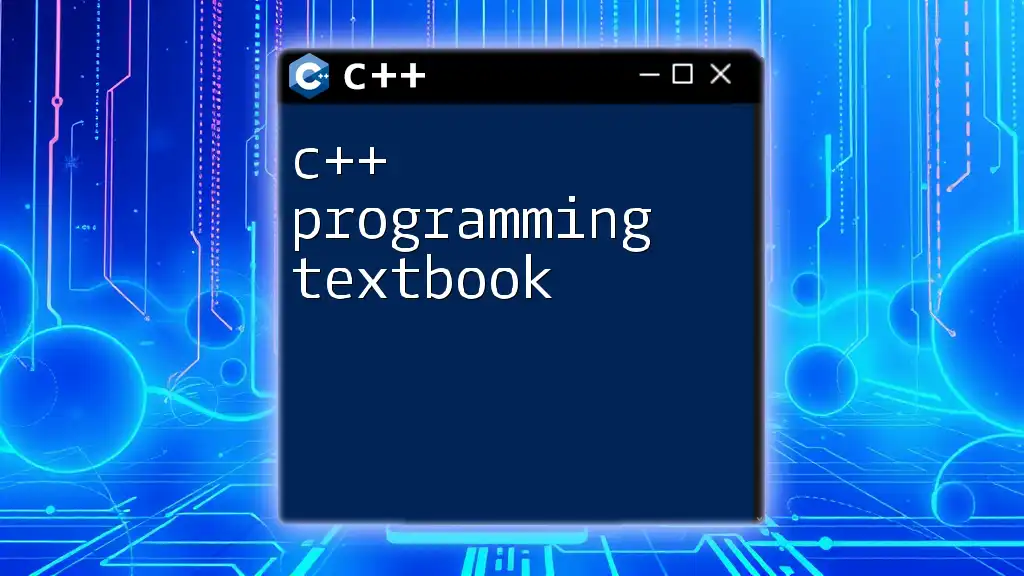
Advanced C++ Features
Variadic Templates
Introduction to Variadic Templates
Variadic templates allow for functions and classes to take an arbitrary number of template parameters. This is especially useful for generic programming.
Practical Examples
A common use for variadic templates is implementing logging functions. Here’s how it can look:
#include <iostream>
#include <string>
template<typename... Args>
void log(Args... args) {
((std::cout << args << " "), ...); // Fold expression in C++17
std::cout << std::endl;
}
void example() {
log("Hello", 42, 3.14); // Output: Hello 42 3.14
}
This demonstration renders logging versatile, allowing it to handle different data types seamlessly.
The "Rule of Five"
Understanding Resource Management
The "Rule of Five" states that if you define one of the following, you should probably define all five: destructor, copy constructor, copy assignment operator, move constructor, and move assignment operator.
Implementation Examples
class Resource {
public:
Resource() { /* Allocate resources */ }
~Resource() { /* Release resources */ }
// Copy Constructor
Resource(const Resource& other) { /* Deep copy */ }
// Copy Assignment
Resource& operator=(const Resource& other) { /* Deep copy */ return *this; }
// Move Constructor
Resource(Resource&& other) noexcept { /* Steal resources */ }
// Move Assignment
Resource& operator=(Resource&& other) noexcept { /* Steal resources */ return *this; }
};
This example encapsulates how to manage resources efficiently, adhering to best practices in modern C++.
Concurrency in C++
Thread Management
C++11 introduced support for multi-threading with `std::thread`, allowing the creation and management of threads quite simply:
#include <thread>
#include <iostream>
void sayHello() {
std::cout << "Hello from thread!" << std::endl;
}
void example() {
std::thread t(sayHello);
t.join(); // Wait for the thread to finish
}
This example demonstrates how to create and manage threads directly within your C++ application.
Using Mutex for Synchronization
To prevent data races in multi-threaded programs, synchronization mechanisms such as `std::mutex` should be employed:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void print(int i) {
mtx.lock();
std::cout << "Thread " << i << std::endl;
mtx.unlock();
}
void example() {
std::thread threads[10];
for (int i = 0; i < 10; ++i) {
threads[i] = std::thread(print, i);
}
for (auto& th : threads) th.join(); // Ensure all threads complete
}
In this case, the `std::mutex` prevents race conditions by ensuring that only one thread prints to the console at a time.
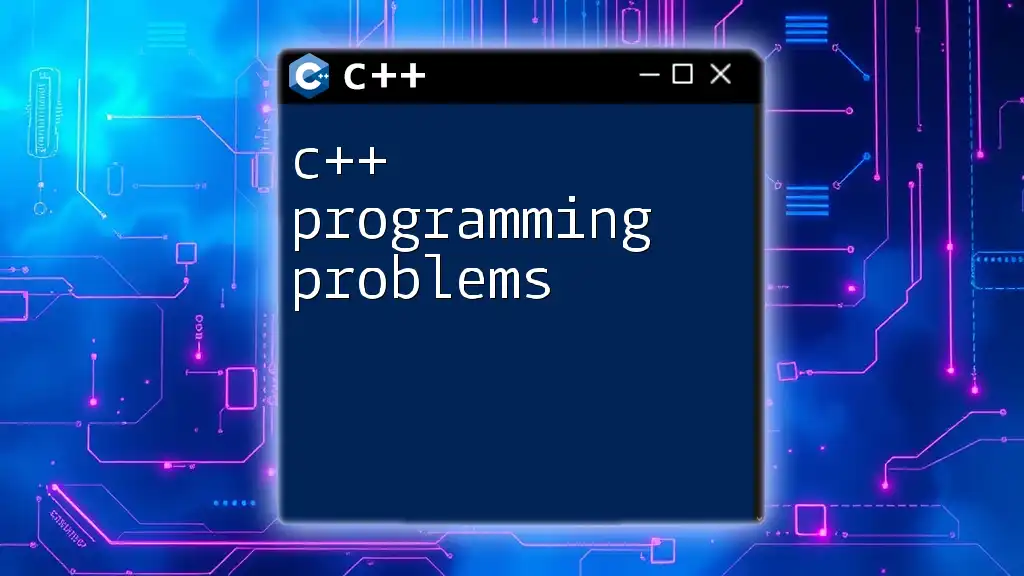
The STL and Its Containers
Overview of STL
The Standard Template Library provides a powerful set of template classes and algorithms that are crucial for effective C++ programming. Utilizing STL can significantly reduce coding time and enhance performance.
Commonly Used Containers
Several containers form the backbone of STL:
- `std::vector`: A dynamic array, providing fast access and automatic resizing.
#include <vector>
void example() {
std::vector<int> vec{1, 2, 3, 4};
vec.push_back(5); // Resizes automatically
}
- `std::list`: A doubly-linked list, allowing efficient insertions and deletions.
- `std::map`: A sorted associative container, storing elements in key-value pairs.
Choosing the right container significantly impacts performance based on use cases.
Iterators
Understanding Iterators
Iterators abstract the concept of pointer-like entities that allow traversal through the elements of a container. They come in various categories, such as input, output, forward, random access, etc.
Custom Iterators Example
Developing custom iterators can enable unique iteration semantics. Here’s a simple iterator implementation:
template<typename T>
class SimpleIterator {
T* ptr;
public:
SimpleIterator(T* p) : ptr(p) {}
// Overloading dereferencing operator
T& operator*() { return *ptr; }
// Overloading increment operator
SimpleIterator& operator++() {
ptr++;
return *this;
}
bool operator!=(const SimpleIterator& other) const { return ptr != other.ptr; }
};
void example() {
int arr[] = {1, 2, 3};
SimpleIterator<int> begin(arr);
SimpleIterator<int> end(arr + 3);
for (auto it = begin; it != end; ++it) {
std::cout << *it << " ";
}
}
This custom iterator showcases how to create custom traversal capabilities, expanding the flexibility of container usage.
Algorithms
Using Standard Algorithms
The STL contains numerous algorithms that operate on containers, including sorting, searching, and transforming.
Common Algorithms
For example, using `std::sort` and `std::find` simplifies tasks:
#include <vector>
#include <algorithm>
#include <iostream>
void example() {
std::vector<int> vec{5, 3, 1, 4, 2};
std::sort(vec.begin(), vec.end()); // Sorts the vector
if (std::find(vec.begin(), vec.end(), 3) != vec.end()) {
std::cout << "Found 3!" << std::endl;
}
}
In this snippet, the vector is sorted using `std::sort` followed by a search for the number 3 using `std::find`, demonstrating the power of STL algorithms.
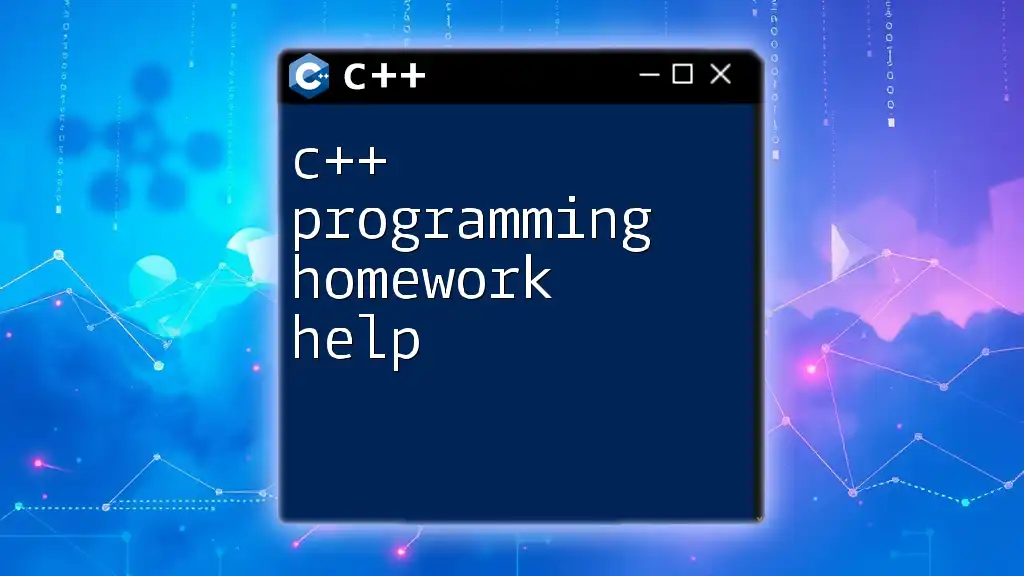
Error Handling and Exceptions
Best Practices for Error Handling
Effective error handling is crucial in robust applications. C++ exceptions provide a means to signal errors during execution instead of handling them at every function return.
Understanding Exceptions
Exceptions provide a structured way to manage errors. The key difference lies in how exceptions let you separate error handling code from regular business logic.
Custom Exception Classes
Creating custom exceptions enhances clarity in error reporting. Here’s an example:
#include <exception>
#include <string>
class MyException : public std::exception {
public:
MyException(const std::string& message) : msg(message) {}
const char* what() const noexcept override { return msg.c_str(); }
private:
std::string msg;
};
void example() {
throw MyException("An error occurred!");
}
In this custom exception example, clarity in error messaging enhances code maintainability.
Using `try`, `catch`, and `throw`
C++ provides structured error handling through `try`, `catch`, and `throw`. Here's a glance at how exceptions work:
void mightGoWrong() {
throw MyException("Oops!");
}
void example() {
try {
mightGoWrong();
} catch (const MyException& e) {
std::cout << e.what() << std::endl; // Output: Oops!
}
}
In this example, the error is thrown and subsequently caught, allowing you to take corrective actions without crashing the program.
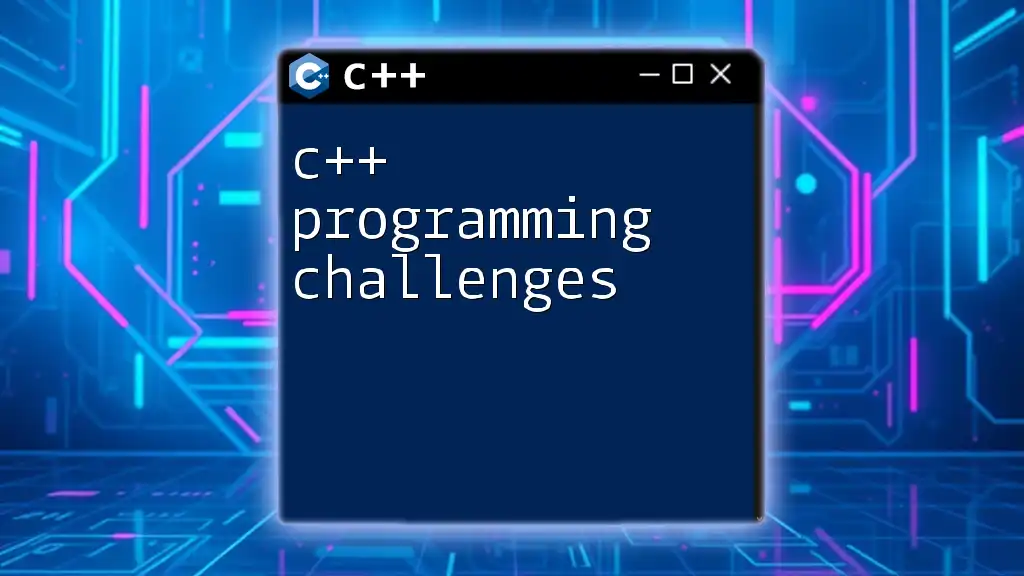
Conclusion
The journey through modern C++ programming showcases its evolution, advanced features, and tools that enhance development productivity. By adopting best practices such as smart pointers, variadic templates, and effective use of STL, programmers can write cleaner and more efficient code.
The Future of C++ Programming
As C++ continues to evolve, keeping abreast of new features, library updates, and improvements remains crucial for any serious programmer.
Encouraging Continuous Learning
With a wealth of resources available, ranging from books to online courses, every programmer can benefit from continual learning and adaptation in this ever-changing landscape.
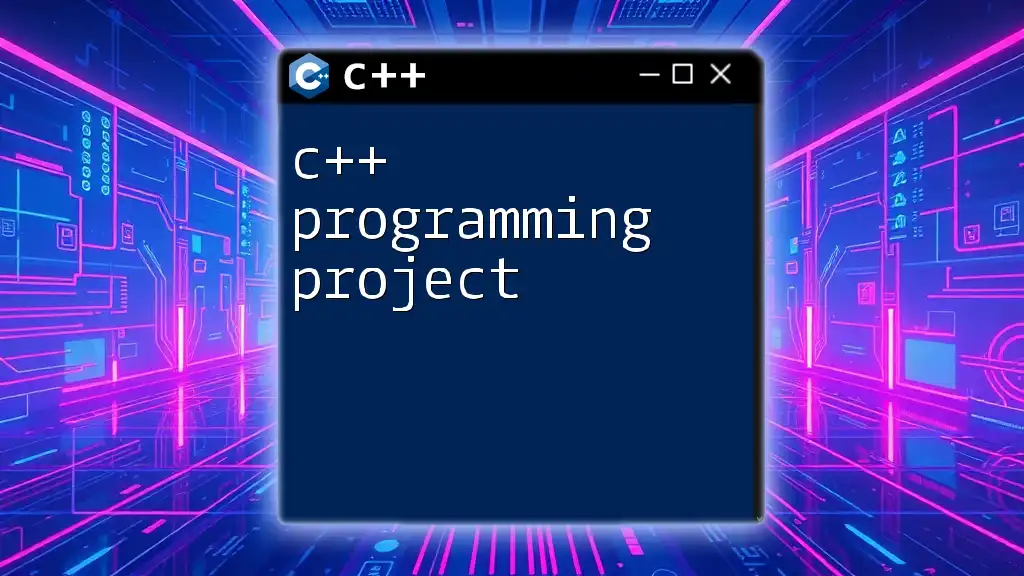
Additional Resources
For further exploration of modern C++ programming techniques, consider diving into recommended books, participating in online communities, and exploring libraries and frameworks that extend C++ capabilities.
This article serves as a comprehensive guide to the modern C++ programming cookbook, perfect for aspiring and seasoned developers alike.