C and C++ are powerful, high-performance programming languages commonly used for system programming and application development, with C being a procedural language and C++ adding object-oriented features.
Here's a simple example of C++ syntax that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Basics of C Programming Language
What is C?
C is a powerful general-purpose programming language that serves as a foundation for many modern languages. Developed in the early 1970s, it is known for its efficiency, simplicity, and low-level access to memory. Due to these features, C has influenced many programming languages, making it an essential language to learn.
Setting Up a C Development Environment
To start programming in C, you need the right tools. Here’s what you will require:
- Compilers: The most popular choices are GCC (GNU Compiler Collection) and Clang. These compilers convert your C code into machine code that the operating system can execute.
- Integrated Development Environments (IDEs): IDEs like Code::Blocks, Dev-C++, and Visual Studio provide a user-friendly interface for writing and managing your C projects.
Installation Steps
To install GCC on a Windows system, follow these steps:
- Download the MinGW-w64 package which includes GCC.
- Run the installer and ensure that you include the path to the `bin` directory in your system’s environment variable.
- Use the command prompt to verify the installation with `gcc --version`.
Basic Syntax
The structure of a C program is relatively straightforward, typically comprising preprocessor directives, the `main` function, and statements. For instance:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
In this example, `#include <stdio.h>` includes the standard input-output library, and `main` is the entry point of the program.
Data Types and Variables
C supports several built-in data types, including `int`, `float`, `char`, and `double`. Understanding these types is crucial for declaring variables correctly.
Declaring Variables:
int age = 30;
float salary = 50000.50;
Here, `age` is an integer variable, while `salary` is a floating-point variable.
Control Structures
Control structures are essential in programming as they dictate the flow of execution.
Conditional Statements
C provides several conditional statements. The `if`, `else if`, and `else` constructs allow you to execute code based on conditions:
if (age < 18) {
printf("Minor");
} else {
printf("Adult");
}
This code checks the variable `age` and prints whether a person is a minor or an adult.
Loops in C
Loops enable you to execute a block of code multiple times, which is vital for tasks that require repetition.
for Loop
The `for` loop is commonly used for counting iterations. Here’s a simple example:
for (int i = 0; i < 5; i++) {
printf("%d ", i);
}
This loop will execute the print statement five times, displaying numbers 0 through 4.
while Loop
Another loop structure in C is the `while` loop, which continues until a specified condition is false:
int i = 0;
while (i < 5) {
printf("%d ", i);
i++;
}
In this example, as long as `i` is less than 5, the loop will print `i` and increment it.
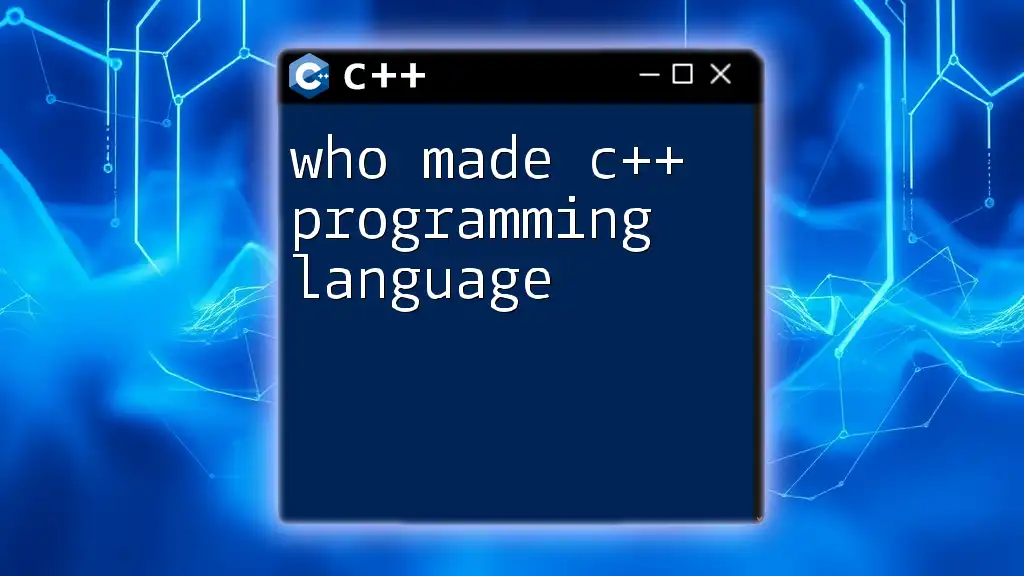
The Basics of C++ Programming Language
What is C++?
C++ is an extension of the C language, incorporating object-oriented programming (OOP) principles. Developed in the early 1980s, C++ enables developers to use classes and objects, enhancing code modularity and reusability.
Setting Up a C++ Development Environment
Just like for C, setting up a C++ development environment requires specific tools.
- Compilers: G++ is a popular compiler for C++ that converts C++ code into machine code.
- IDEs: Eclipse and Visual Studio are widely used IDEs for C++ development.
Installation Steps
To set up G++ on your system, follow these steps:
- Download the MinGW-w64 package that includes G++.
- Run the installer and add the `bin` directory to your system environment variable.
- Check the installation by executing `g++ --version` in your command prompt.
Basic Syntax
C++ syntax is similar to C, but it introduces several new constructs. Here’s an example of a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
Here, the `#include <iostream>` statement imports the input-output stream library, which is essential for output operations in C++.
Object-Oriented Programming Concepts
OOP is a fundamental aspect of C++, making it necessary to understand key concepts like classes and objects.
Classes and Objects
Creating classes allows you to define your own data types. Here's how to define a basic class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!";
}
};
In this example, the `Dog` class has a method called `bark`. To use this class, you create an object:
Dog dog;
dog.bark();
This will output "Woof!"
Inheritance
Inheritance allows a class to inherit characteristics from another class, promoting code reusability. Here is an example:
class Animal {
public:
void eat() {
std::cout << "Eating...";
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!";
}
};
In this case, the `Dog` class inherits the method `eat` from the `Animal` class, allowing a dog to eat and bark.
Important Features Additions in C++
C++ comes with the Standard Template Library (STL), which provides generic classes and functions. This is instrumental for developing complex data structures and algorithms efficiently.
Using Vectors
C++ provides `vector`, a dynamic array that can grow in size. Here’s a brief example:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
This initializes a vector containing integers, offering more flexibility than standard arrays.
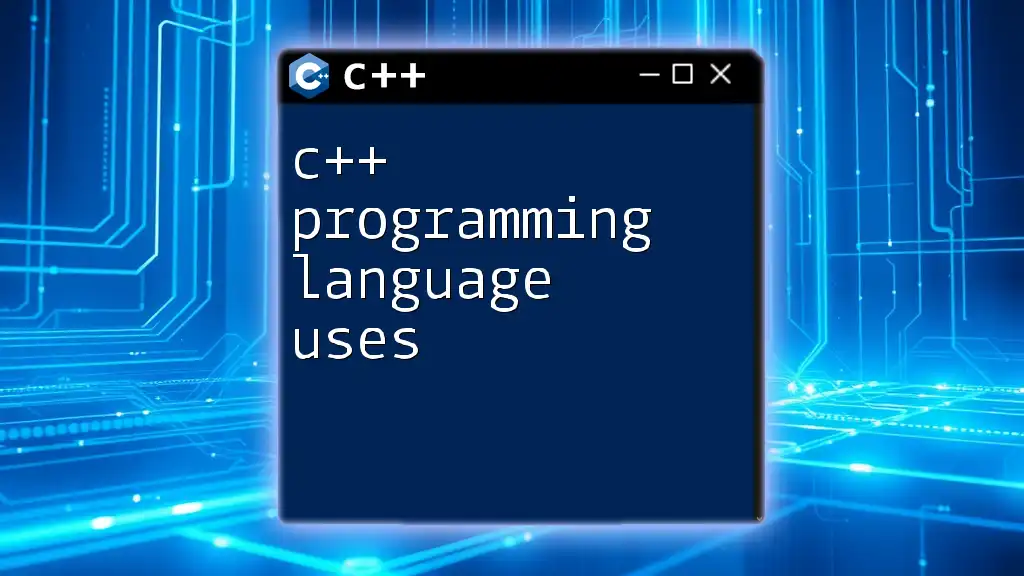
Key Differences Between C and C++
Understanding the differences between C and C++ can help you make better choices while learning.
-
Language Paradigms: C is primarily a procedural language, emphasizing sequential execution, whereas C++ supports both procedural and object-oriented paradigms.
-
Memory Management: C requires manual memory management using functions like `malloc` and `free`, while C++ introduces constructors and destructors for automatic memory handling.
-
Standard Libraries: C has a limited standard library compared to the extensive features provided by C++ through its STL.
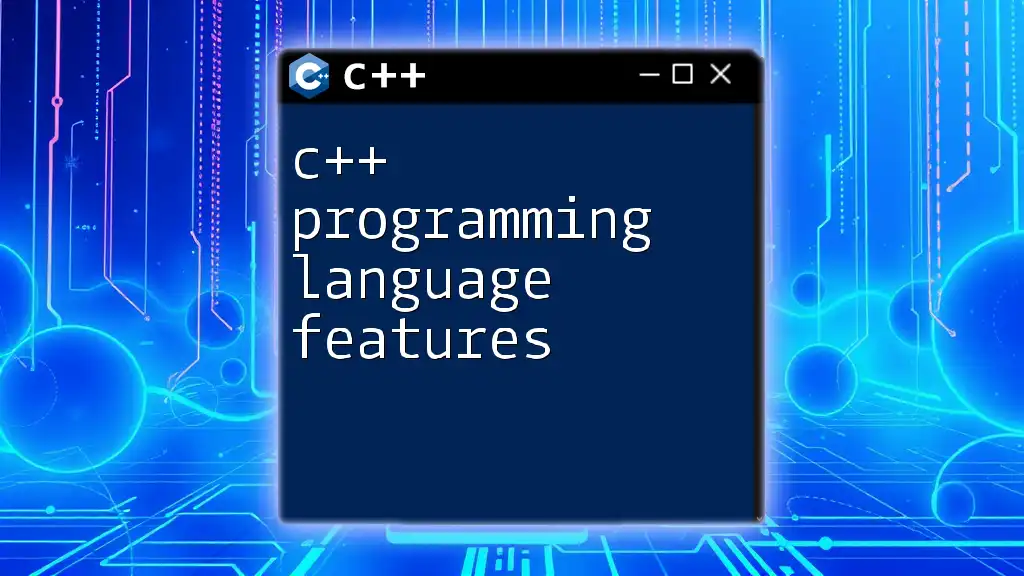
Conclusion
Learning C and C++ programming languages offers a host of opportunities, from software development to systems programming. Both languages provide a strong foundation in computer science concepts and data structures, paving the way for more advanced programming languages.
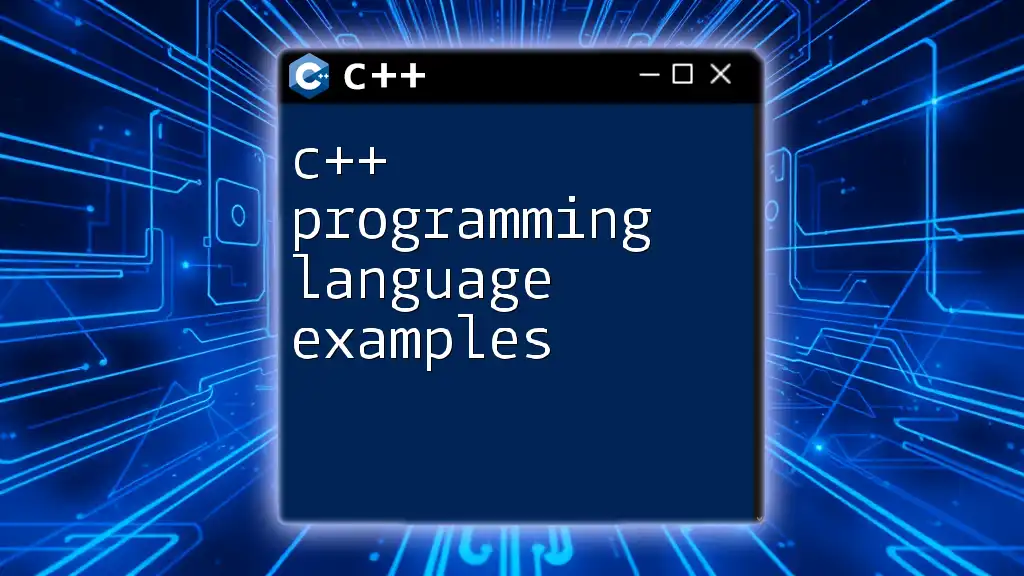
Additional Resources
For anyone looking to expand their knowledge, various books, online courses, and communities are available. Platforms like LeetCode and HackerRank offer practical applications for honing your skills.
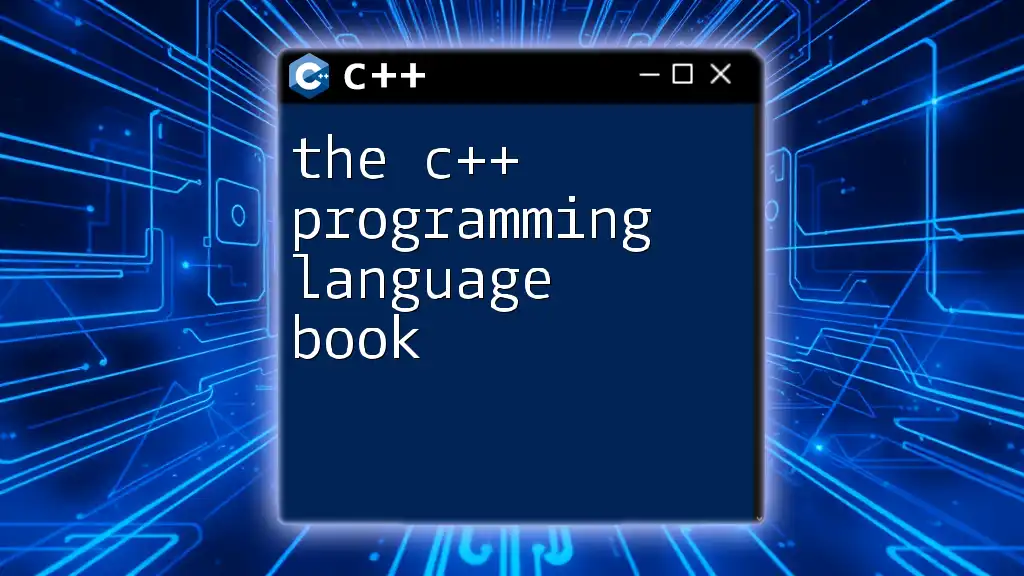
FAQs
-
Is C++ harder than C? C++ has a steeper learning curve due to its additional features, especially relating to OOP.
-
What are practical applications of C and C++? Both languages are widely used in software development, game development, systems programming, and performance-critical applications.