C++ programming problems often challenge developers to solve tasks efficiently using core concepts of the language, such as loops and conditionals.
Here's a simple example of a C++ program that checks if a number is even or odd:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
if (num % 2 == 0)
cout << num << " is even." << endl;
else
cout << num << " is odd." << endl;
return 0;
}
C++ Programming Problems for Beginners
Getting Started with C++ Problems
C++ programming problems are essential for honing your coding skills. Before diving into problem-solving, it's crucial to familiarize yourself with the basic structure of C++ programs. C++ syntax, like any other programming language, follows a specific set of rules, and understanding these can significantly improve your coding efficiency.
When you engage in solving problems, you naturally enhance your algorithmic thinking and sharpen your problem-solving skills. It’s not just about finding the right answer; it’s about developing a method to arrive at that answer systematically.
Essential Topics to Cover for Beginners
Variables and Data Types
Understanding variables and data types is fundamental in C++. Different types of data require different storage sizes and handling methods, impacting how you design your solutions.
Control Structures
Control structures, such as `if`, `for`, and `while`, form the foundation for decision-making and looping in C++. Mastering these allows you to create logic paths in your programs.
Functions and Scope
Functions in C++ enable you to compartmentalize your code for reuse. Understanding scope is key as it defines the visibility and lifespan of the variables you declare.
Arrays and Strings
Arrays are essential for handling collections of data, while strings are crucial for text processing. Both require different handling methods and techniques — knowing how to manipulate them is vital for any C++ developer.
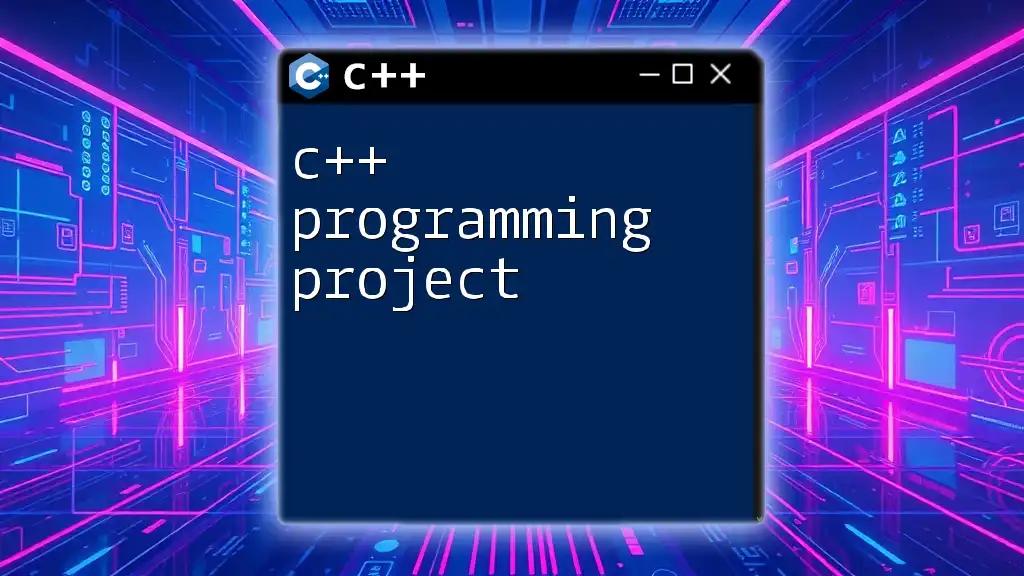
C++ Coding Problems for Practice
Simple Coding Problems
Example: Sum of Two Numbers
To begin with a straightforward problem, let’s calculate the sum of two integers input by the user.
#include <iostream>
using namespace std;
int main() {
int num1, num2, sum;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
sum = num1 + num2;
cout << "The sum is: " << sum << endl;
return 0;
}
This code utilizes input-output operations in C++, showcasing how to take user input and produce an output.
Example: Factorial Calculation
The factorial of a number illustrates how to use loops or recursion in C++. Here's a simple iterative solution:
#include <iostream>
using namespace std;
int main() {
int n;
unsigned long long factorial = 1;
cout << "Enter a positive integer: ";
cin >> n;
for(int i = 1; i <= n; ++i) {
factorial *= i; // Multiply each number
}
cout << "Factorial of " << n << " = " << factorial << endl;
return 0;
}
This example demonstrates iteration and the use of the `for` loop effectively.
Intermediate C++ Problems
Example: Reverse a String
Reversing a string is a common problem that can be solved using various methods. Here’s one straightforward approach using built-in functions:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
reverse(str.begin(), str.end()); // Using STL reverse function
cout << "Reversed string: " << str << endl;
return 0;
}
This example utilizes the Standard Template Library (STL) to perform operations efficiently.
Example: Finding Prime Numbers
Finding all prime numbers up to a certain limit is a classic algorithmic exercise:
#include <iostream>
using namespace std;
bool isPrime(int n) {
if (n <= 1) return false;
for (int i = 2; i <= n / 2; i++) {
if (n % i == 0)
return false;
}
return true;
}
int main() {
int limit;
cout << "Enter the upper limit: ";
cin >> limit;
cout << "Prime numbers up to " << limit << ": ";
for (int i = 2; i <= limit; i++) {
if (isPrime(i)) {
cout << i << " ";
}
}
return 0;
}
Through this example, you gain insight into function creation and conditional checking.
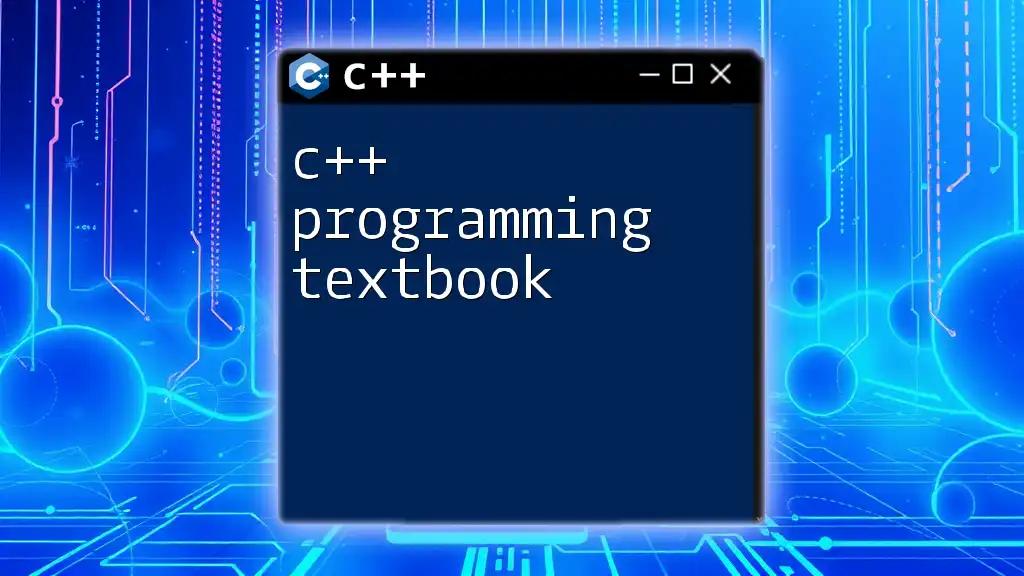
Common C++ Problems and Their Solutions
Top C++ Problems for Beginners
Problem: Fibonacci Series
Generating the Fibonacci series is a classic problem that reinforces recursion or iteration concepts.
#include <iostream>
using namespace std;
void fibonacci(int n) {
int t1 = 0, t2 = 1, nextTerm;
cout << "Fibonacci Series: " << t1 << ", " << t2 << ", ";
for (int i = 3; i <= n; ++i) {
nextTerm = t1 + t2;
cout << nextTerm << ", ";
t1 = t2;
t2 = nextTerm;
}
}
int main() {
int n;
cout << "Enter the number of terms: ";
cin >> n;
fibonacci(n);
return 0;
}
This practical exercise demonstrates how to manage sequences and understand the cumulative essence of the Fibonacci concept.
Problem: Check for Palindrome
A palindrome check is another engaging exercise that explores string handling capabilities.
#include <iostream>
#include <string>
using namespace std;
bool isPalindrome(string str) {
string reversedStr = string(str.rbegin(), str.rend());
return str == reversedStr;
}
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
if (isPalindrome(str)) {
cout << str << " is a palindrome." << endl;
} else {
cout << str << " is not a palindrome." << endl;
}
return 0;
}
This example allows you to practice string manipulation skills and logical programming.
Real-World Application Examples
Example: Basic Banking System
A basic banking system involves real-world practical applications that enhance your understanding of classes, user input, and storage.
#include <iostream>
#include <string>
using namespace std;
class BankAccount {
private:
string accountHolder;
double balance;
public:
BankAccount(string name, double initialBalance) {
accountHolder = name;
balance = initialBalance;
}
void deposit(double amount) {
balance += amount;
cout << "Deposited: " << amount << endl;
}
void displayBalance() {
cout << "Balance: " << balance << endl;
}
};
int main() {
BankAccount account("John Doe", 1000.0);
account.deposit(500);
account.displayBalance();
return 0;
}
This C++ snippet introduces you to classes and objects, reflecting a typical use of OOP principles.
Example: Tic-Tac-Toe Game
Creating a Tic-Tac-Toe game combines numerous C++ programming concepts:
#include <iostream>
using namespace std;
char board[3][3] = {{'1', '2', '3'},{'4', '5', '6'},{'7', '8', '9'}};
char currentPlayer = 'X';
void displayBoard() {
cout << "Current board:\n";
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
bool placeMark(int slot) {
int row = (slot - 1) / 3;
int col = (slot - 1) % 3;
if (board[row][col] != 'X' && board[row][col] != 'O') {
board[row][col] = currentPlayer;
return true;
}
return false;
}
bool checkWin() {
// Check rows, columns, and diagonals for win
for (int i = 0; i < 3; i++)
if ((board[i][0] == currentPlayer && board[i][1] == currentPlayer && board[i][2] == currentPlayer) ||
(board[0][i] == currentPlayer && board[1][i] == currentPlayer && board[2][i] == currentPlayer))
return true;
return (board[0][0] == currentPlayer && board[1][1] == currentPlayer && board[2][2] == currentPlayer) ||
(board[0][2] == currentPlayer && board[1][1] == currentPlayer && board[2][0] == currentPlayer);
}
int main() {
int playerMove;
int moves = 0;
while (moves < 9) {
displayBoard();
cout << "Player " << currentPlayer << ", enter your move (1-9): ";
cin >> playerMove;
if (placeMark(playerMove)) {
moves++;
if (checkWin()) {
displayBoard();
cout << "Player " << currentPlayer << " wins!" << endl;
return 0;
}
currentPlayer = (currentPlayer == 'X') ? 'O' : 'X'; // Switch player
} else {
cout << "Invalid move, try again." << endl;
}
}
displayBoard();
cout << "It's a draw!" << endl;
return 0;
}
This code allows you to build a complete application using multiple programming concepts, from loops and conditionals to functions and arrays.
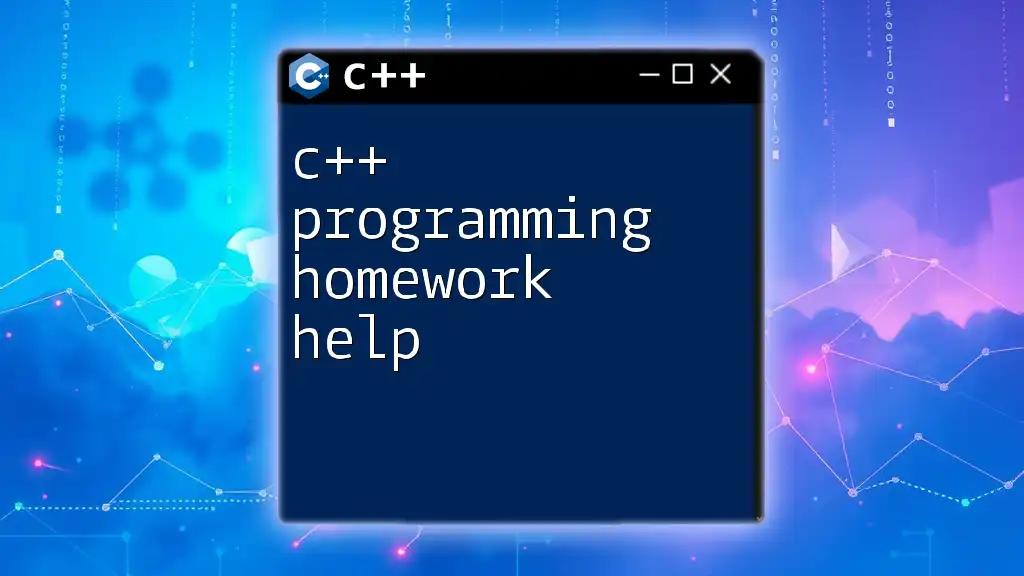
C++ Beginner Practice Problems
Resources for C++ Practice Problems
If you're looking for more challenges, consider visiting online coding platforms like LeetCode, HackerRank, and CodeSignal. These platforms offer a variety of problems that cater to different skill levels, ensuring that you can continue improving your programming proficiency.
Additionally, several recommended books provide a deeper understanding of C++. Incorporating these materials into your study routine can be invaluable for beginners.
Tips for Mastering C++ Through Practice
Establishing a consistent practice schedule can lead to noticeable improvements over time. Focus on understanding not just how to implement a solution, but also why it works. Reviewing various problem-solving approaches and discussing them within a community can further enhance your learning experience.
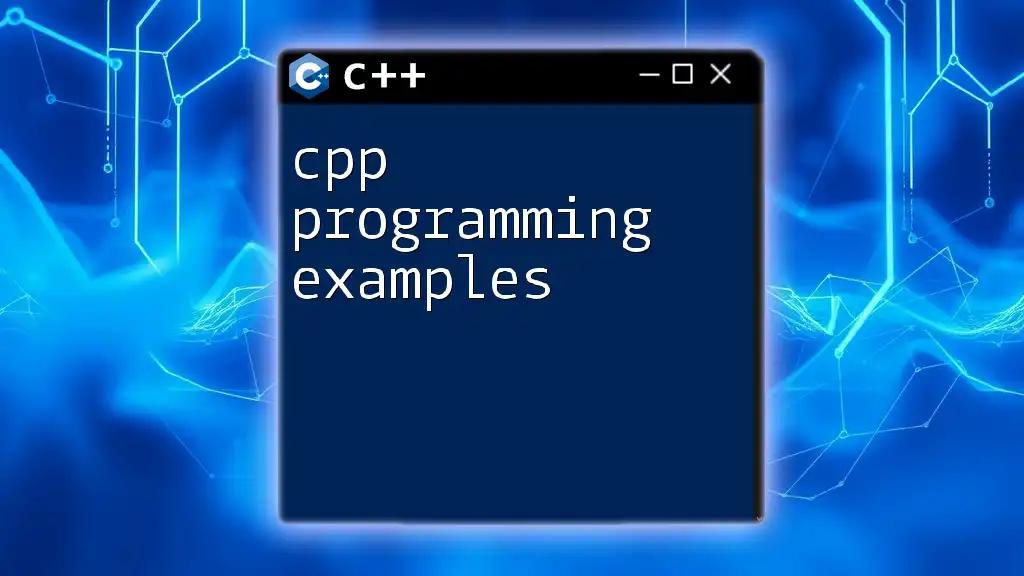
Frequently Asked Questions (FAQs)
What Should I Practice First in C++?
Starting with fundamental topics—such as basic syntax, data types, and control structures—will lay the groundwork for tackling more complex problems in the future. Mastery of the basics is crucial before moving on to more intricate algorithms and structures.
How Can I Track My Progress in C++?
Tracking your progress can be accomplished through various methods, such as keeping a journal of problems solved, maintaining a portfolio of projects, or utilizing platforms that provide performance analytics. Regular reflections on what you've learned can significantly aid in your development.
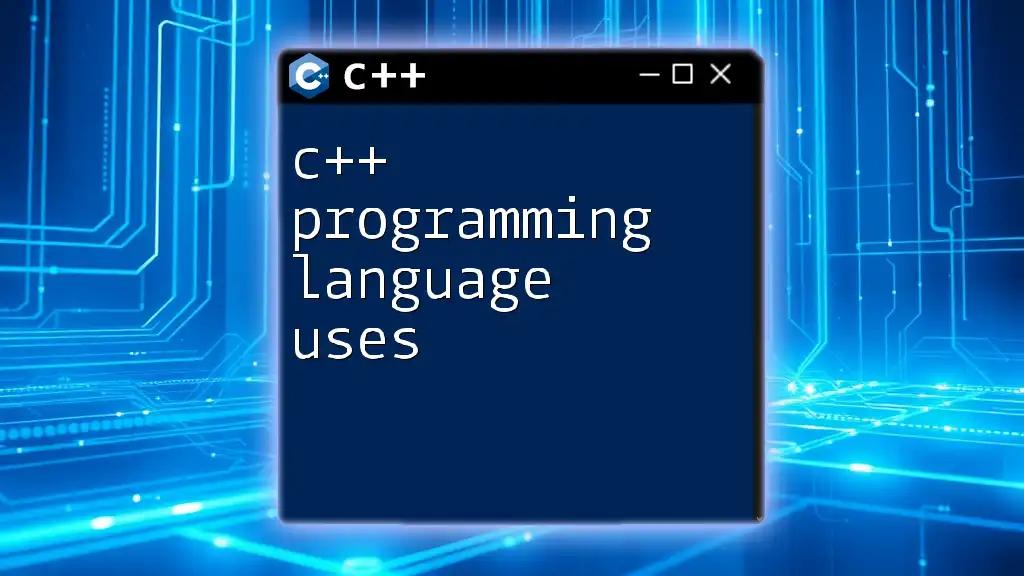
Conclusion
Engaging with C++ programming problems offers an essential pathway to becoming a proficient programmer. By consistently practicing various problems, particularly tailored for beginners, you can solidify your understanding of fundamental concepts while preparing yourself for more advanced challenges. Remember, the key to improvement lies in persistence and a willingness to learn. Embrace challenges and keep coding!
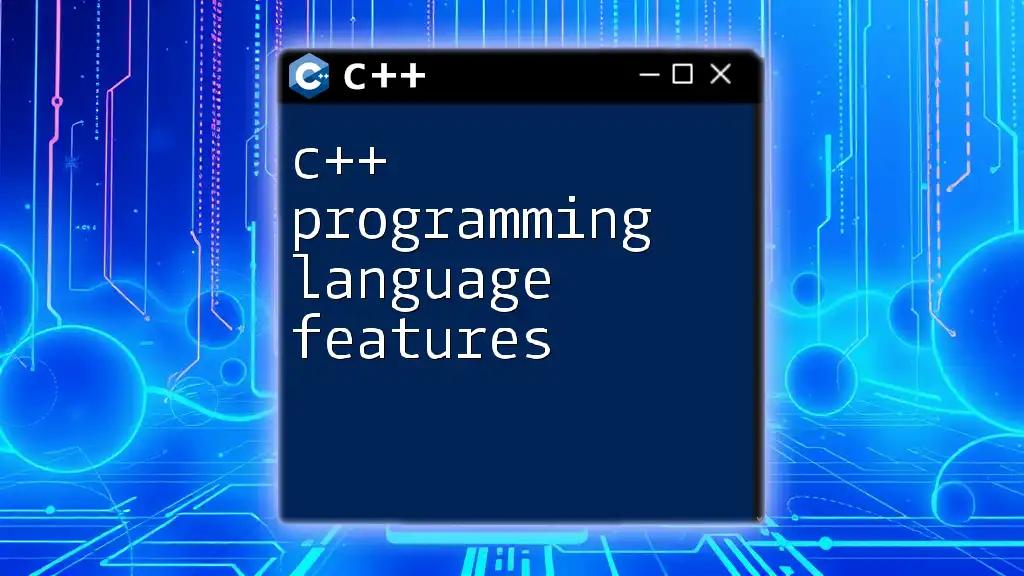
Additional Resources
Books on C++ Programming
For deeper insights, consider reading "C++ Primer" by Stanley Lippman or "Effective C++" by Scott Meyers. These books are widely recognized as excellent resources for both beginners and advanced programmers.
Websites and Forums for C++ Learning
Take advantage of online forums such as Stack Overflow or Reddit communities focused on C++. Engaging with fellow learners provides opportunities to solve problems collaboratively and exchange knowledge.
This article aims to provide a comprehensive understanding of C++ programming problems, ensuring that beginners have all the tools they need to succeed. Happy coding!