A C++ programmer is someone who writes code in the C++ programming language, utilizing its features to create efficient and scalable applications.
Here's a simple code snippet demonstrating a basic C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-performance programming language that supports both procedural and object-oriented programming paradigms. Originally developed by Bjarne Stroustrup in the early 1980s, C++ has evolved over the years, integrating new features and enhancements that make it one of the most widely used programming languages today. Its ability to provide low-level memory manipulation while maintaining high-level abstractions makes it unique among its peers.
Why Learn C++?
Learning C++ opens doors to various opportunities across multiple domains. Its applicability ranges from game development and system software to high-performance applications in finance and real-time systems.
Performance is one of the key reasons why C++ remains popular. Programs written in C++ tend to be faster and more efficient than those written in languages like Python or Java, partly due to its close-to-hardware nature. Additionally, C++ is often the language of choice for building performance-critical applications, making it indispensable for a C++ programmer's toolkit.
Career prospects for C++ programmers are promising, with numerous companies seeking skilled developers. According to various industry reports, C++ programmers can earn competitive salaries, particularly those working in specialized fields like game development, embedded systems, or real-time computing.
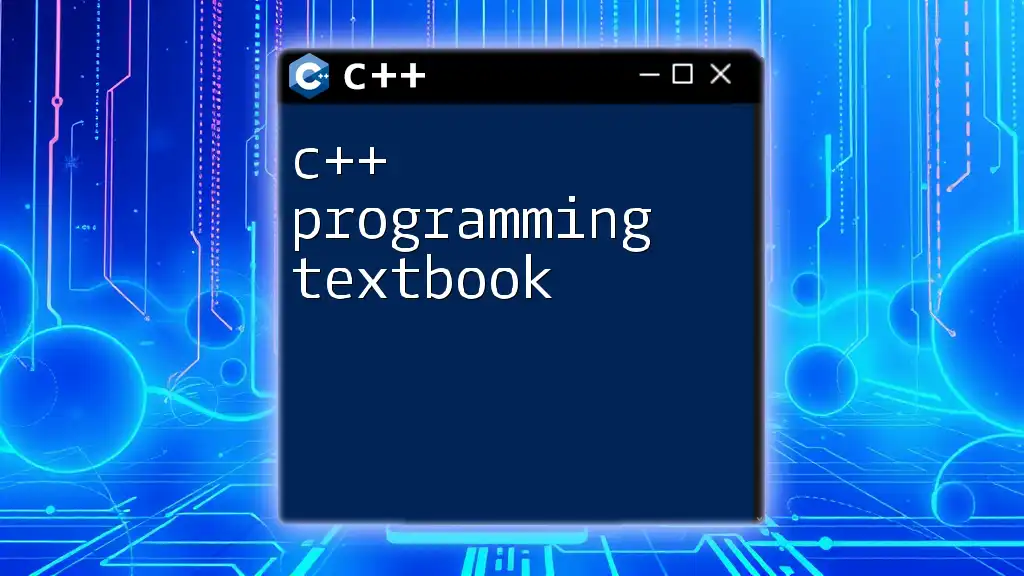
Getting Started with C++
Setting Up Your Development Environment
The first step in your journey as a C++ programmer is to set up your development environment. You will need a suitable Integrated Development Environment (IDE) or text editor. Some popular choices include:
- Visual Studio: A feature-rich environment favored by many C++ developers.
- Code::Blocks: A simple open-source IDE suitable for beginners.
- CLion: A cross-platform IDE from JetBrains, known for its smart coding assistance.
Next, you must install a C++ compiler. Some of the most commonly used compilers are:
- GCC: The GNU Compiler Collection, widely used in various environments.
- Clang: Known for its fast compilation and extensive support for modern C++ features.
Once you’ve installed an IDE and compiler, you can create your first C++ project and start coding.
Your First C++ Program: Hello World
Creating a simple C++ program is the best way to familiarize yourself with the language's syntax. Below is the classic "Hello, World!" program.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Breakdown of the code:
- `#include <iostream>`: This line includes the Input/Output stream library, which allows us to use `cout`.
- `using namespace std;`: This line enables us to avoid prefixing standard functions with `std::`.
- `int main() {...}`: The main function serves as the entry point of the program.
- `cout << "Hello, World!" << endl;`: This line outputs the text to the console.
- `return 0;`: This statement indicates that the program finished successfully.
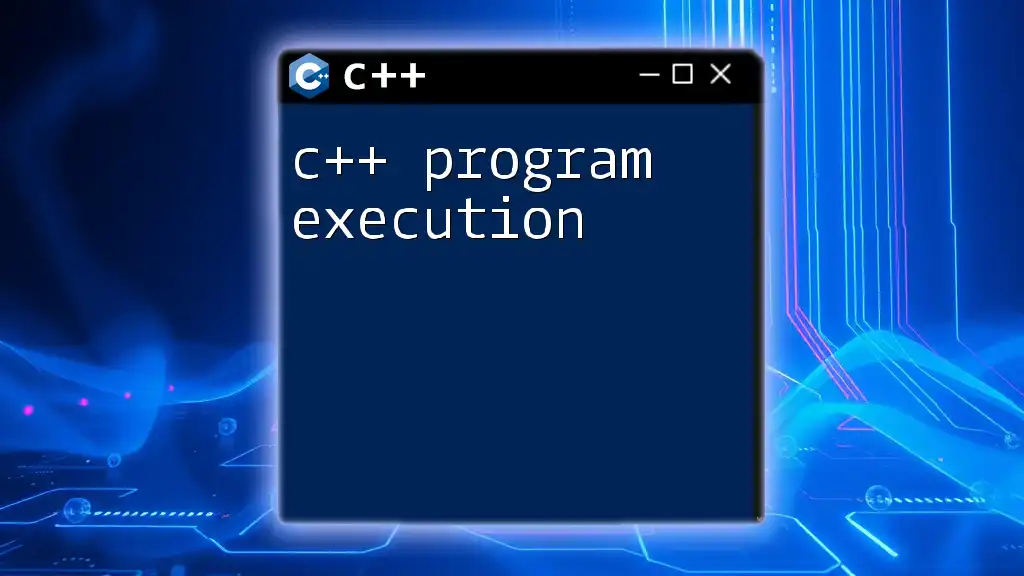
Core Concepts of C++
Basic Syntax and Data Types
Understanding the basic syntax and data types in C++ is crucial for any aspiring C++ programmer. C++ has a set of rules that define how code should be structured and written.
Primitive Data Types:
- int: Represents integer values.
- float: Represents floating-point numbers.
- char: Represents single characters.
- bool: Represents Boolean values (true or false).
Here’s a code example illustrating the declaration of different data types:
int number = 10;
char letter = 'A';
float pi = 3.14f;
Control Structures
Conditional Statements
Conditional statements allow you to execute specific blocks of code based on whether conditions evaluate to true or false. `if`, `else if`, and `else` statements are commonly used for branching logic.
Here’s a basic example of a conditional statement:
if (number > 0) {
cout << "Positive Number";
} else {
cout << "Negative Number";
}
In this scenario, it checks if `number` is greater than zero and prints an appropriate message accordingly.
Loops
Loops are essential for performing repeated tasks. C++ provides various loop constructs including `for`, `while`, and `do-while` loops.
Here’s an example of a `for` loop:
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i << endl;
}
This loop will execute five times, printing the iteration number on each pass.
Functions
Functions are fundamental to structuring your C++ program. They enable code reusability and logical separation of functionalities.
In C++, you define a function with its return type, name, and parameters. Here’s a simple function that adds two numbers:
int add(int a, int b) {
return a + b;
}
cout << add(5, 3); // Outputs: 8
Advanced C++ Concepts
Object-Oriented Programming (OOP)
OOP is a programming paradigm that fosters code organization and reusability. It revolves around the concepts of classes and objects.
Understanding Classes and Objects: A class is a blueprint for creating objects. An object is an instance of a class. The following code snippet illustrates how to define a class and create an object:
class Dog {
public:
string name;
void bark() {
cout << name << " says Woof!" << endl;
}
};
Dog myDog;
myDog.name = "Buddy";
myDog.bark(); // Outputs: Buddy says Woof!
Inheritance and Polymorphism
Inheritance allows a class to acquire properties and behaviors from another. Polymorphism enables a single interface to represent different underlying forms (data types).
Here’s an example demonstrating inheritance:
class Animal {
public:
virtual void sound() { cout << "Animal sound"; }
};
class Cat : public Animal {
public:
void sound() override { cout << "Meow"; }
};
In this example, `Cat` inherits from `Animal` and overrides the `sound()` method.
Memory Management
Pointers and References
Memory management is crucial in C++. Pointers store the memory address of another variable. Here’s how pointers work:
int a = 10;
int* ptr = &a;
cout << *ptr; // Outputs: 10
In this example, `ptr` holds the address of `a`, and `*ptr` dereferences that pointer to access the value.
Dynamic Memory Management
C++ allows dynamic memory management through the use of `new` and `delete`. For instance:
int* arr = new int[10]; // Allocates an array of 10 integers
delete[] arr; // Frees the dynamically allocated memory
This example demonstrates how to allocate and deallocate memory manually.
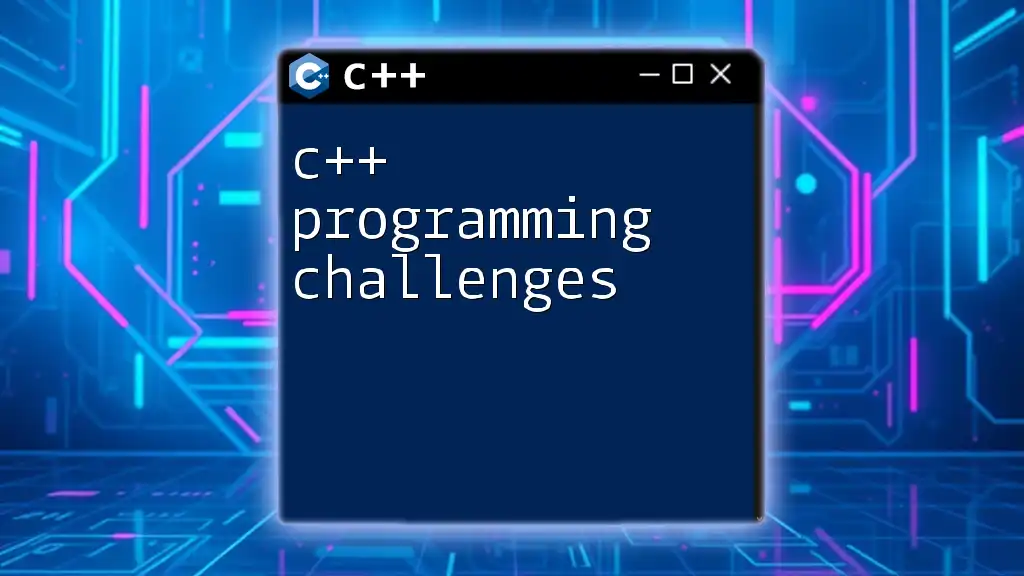
Best Practices for C++ Programmers
Writing Clean and Efficient Code
For any C++ programmer, writing clean and efficient code is paramount. Consider structuring your code for readability, making use of functions to handle repetitive tasks and ensuring proper indentation. Always remember the importance of commenting; well-commented code is easier to maintain and understand.
Utilizing modern C++ features, such as smart pointers and the Standard Template Library (STL), can greatly enhance your coding efficiency and reduce potential memory leaks or bugs.
Debugging and Testing
Debugging is an integral part of programming. Familiarizing yourself with common debugging techniques—such as using breakpoints, examining variable values, and stepping through code—will significantly improve your debugging skills.
Unit testing is another crucial practice. It verifies that individual components of the software work as intended. Libraries like Google Test provide a powerful framework for writing tests that help ensure your code's reliability.
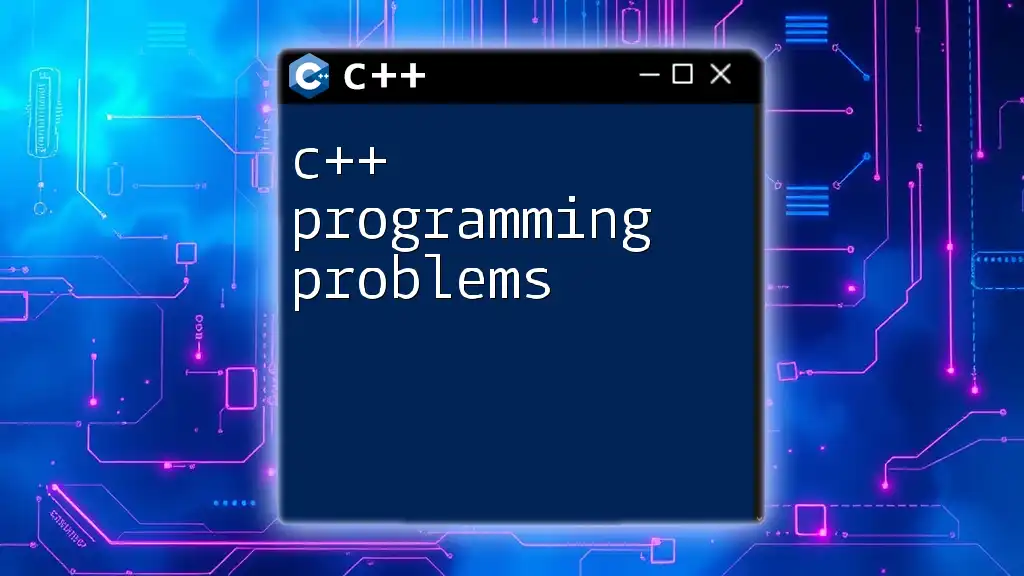
Resources for Continuous Learning
Recommended Books and Tutorials
To deepen your knowledge, consider reading some of the most lauded books on C++, such as:
- "The C++ Programming Language" by Bjarne Stroustrup
- "Effective C++" by Scott Meyers
- "C++ Primer" by Stanley B. Lippman
Additionally, online platforms like Coursera, Udacity, and freeCodeCamp offer comprehensive C++ tutorials that can further aid your learning.
Community and Forums
Engagement with the programming community can significantly enhance your skills. Websites like Stack Overflow and the C++ subreddit provide platforms for asking questions, sharing knowledge, and networking with other developers.
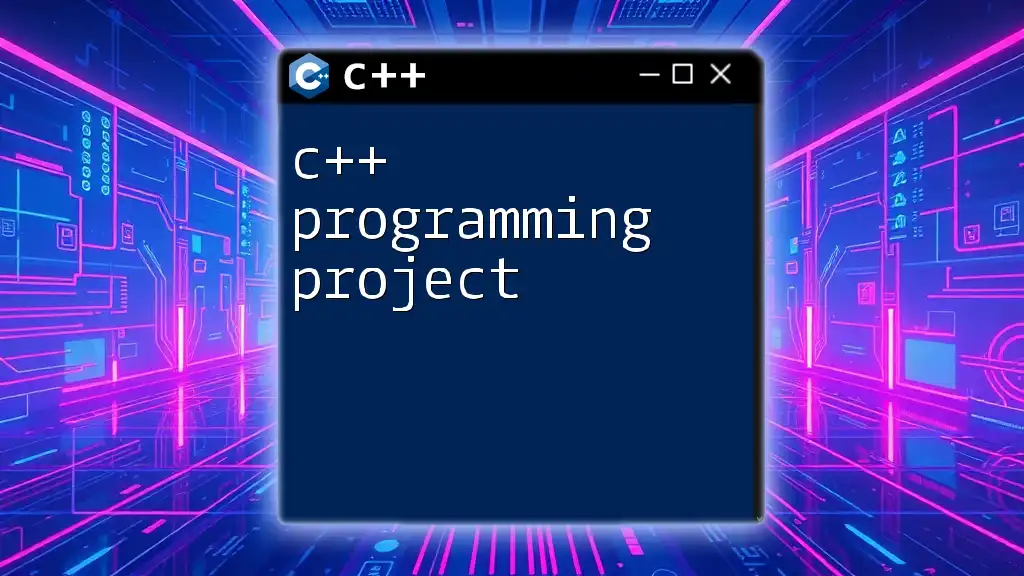
Conclusion
Becoming a proficient C++ programmer is a rewarding journey filled with continuous learning and practical application. The language's relevance in many domains ensures that you will always find opportunities to apply your skills.
The key takeaway is to engage regularly with coding practices, join communities, and embrace continuous learning. By doing so, you’ll be well on your way to mastering C++ and unlocking your potential in the programming world.
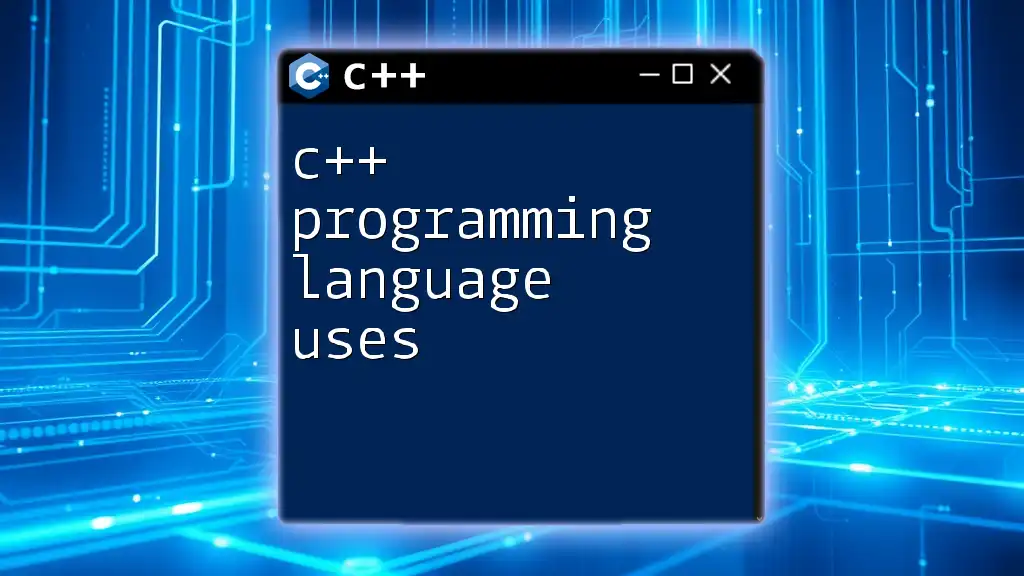
Call to Action
Join our courses to master C++ quickly and efficiently. Whether you’re starting your coding journey or looking to enhance your existing skills, we’re here to support you every step of the way. Ready to dive in? Sign up today!