C++ for programmers is an essential skill that empowers developers to create efficient software through its powerful object-oriented features and rich standard library, enabling quick problem-solving and performance optimization.
Here’s a simple code snippet demonstrating a basic C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful general-purpose programming language that extends the C programming language with object-oriented features. It has gained immense popularity due to its efficiency and flexibility. As a multi-paradigm language, it supports both procedural and object-oriented programming, making it versatile for various applications, ranging from system software to high-performance games and real-time simulations.
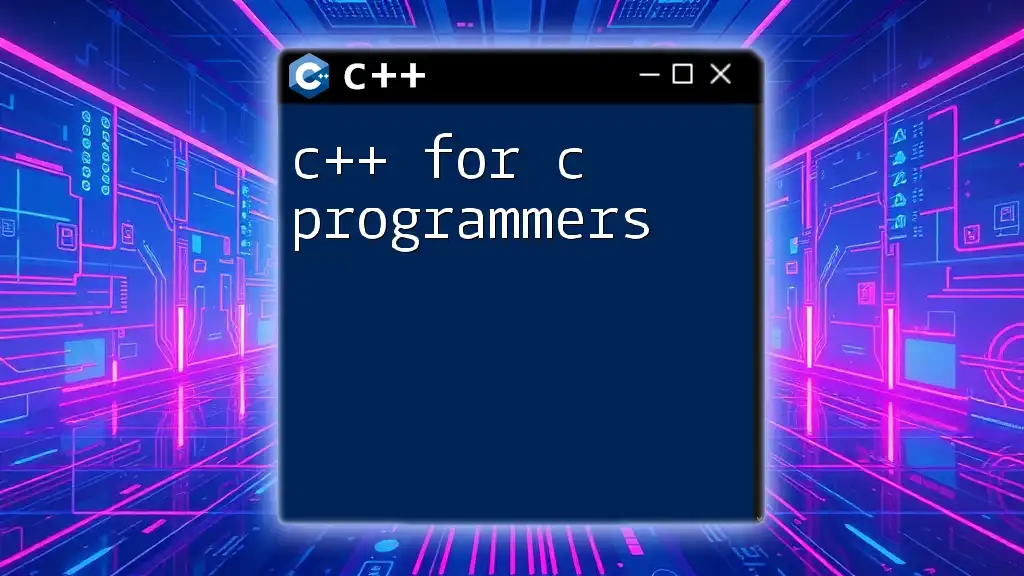
Key Features of C++
Object-Oriented Programming (OOP)
One of the defining features of C++ is its support for Object-Oriented Programming (OOP). OOP is a programming paradigm that uses "objects" to represent data and methods. The primary concepts include:
- Classes and Objects: Classes serve as blueprints for creating objects. An object is an instance of a class.
Example: A simple class definition in C++ might look like this:
class Animal {
public:
virtual void sound() {
std::cout << "Some sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark" << std::endl;
}
};
In this example, `Animal` is a base class, and `Dog` is a derived class that extends its functionality by overriding the `sound()` method.
-
Inheritance: Inheritance allows a class to derive properties and behaviors from another class. This helps to promote code reusability.
-
Polymorphism: It lets you use a single interface to represent different underlying data types. With polymorphism, you can call methods of derived classes through base class pointers.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides a collection of template classes and functions for managing data structures and algorithms. STL includes several generic classes like vectors, lists, sets, maps, etc.
Example: Here’s how you can use a vector in C++:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int n : numbers) {
std::cout << n << " ";
}
}
In this example, a vector stores integers, and we can easily iterate through the elements.
Memory Management
C++ provides extensive support for memory management, which is crucial when you want to handle dynamic memory. This includes the use of pointers, which allow for the direct manipulation of memory locations.
You can allocate memory using `new` and free it using `delete`. Proper memory management is vital to avoid memory leaks.
Example: Here’s how to dynamically allocate and free memory:
int* ptr = new int(10); // Dynamic memory allocation
std::cout << *ptr; // Output: 10
delete ptr; // Freeing the allocated memory
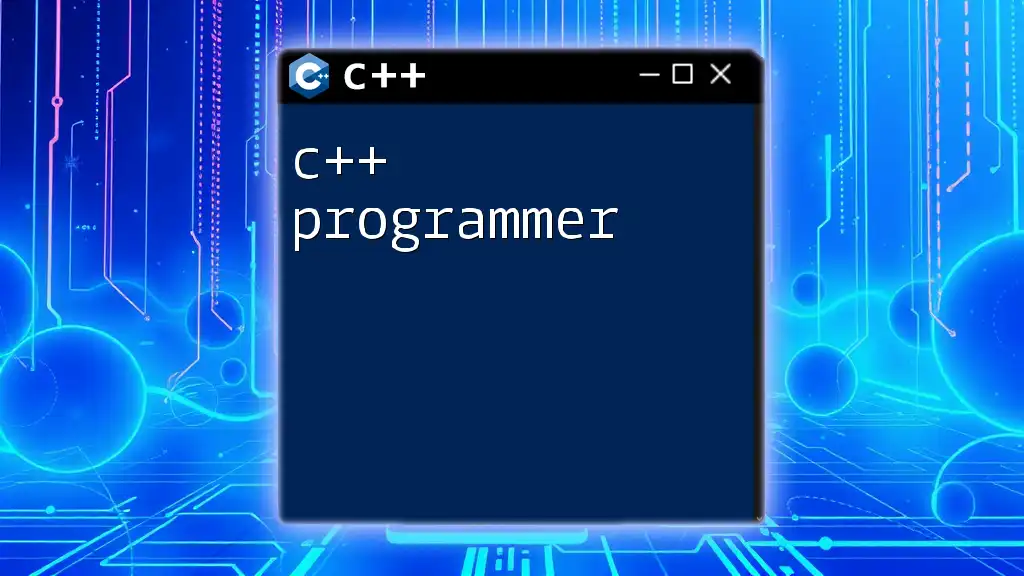
Setting Up the C++ Environment
Before diving into C++ programming, you need to set up your environment. This typically involves installing a compiler and an Integrated Development Environment (IDE).
Installing Compilers and IDEs
Popular options include:
-
GCC: The GNU Compiler Collection is widely used for compiling C++ programs, especially on Linux systems.
-
Clang: Known for its fast compilation and excellent diagnostics.
-
Visual Studio: A powerful IDE popular among Windows users, offering extensive debugging and project management features.
-
Code::Blocks: A free and open-source IDE that supports multiple compilers and is easy to use for beginners.
Each option will come with installation guides tailored to different operating systems, allowing for a streamlined setup.
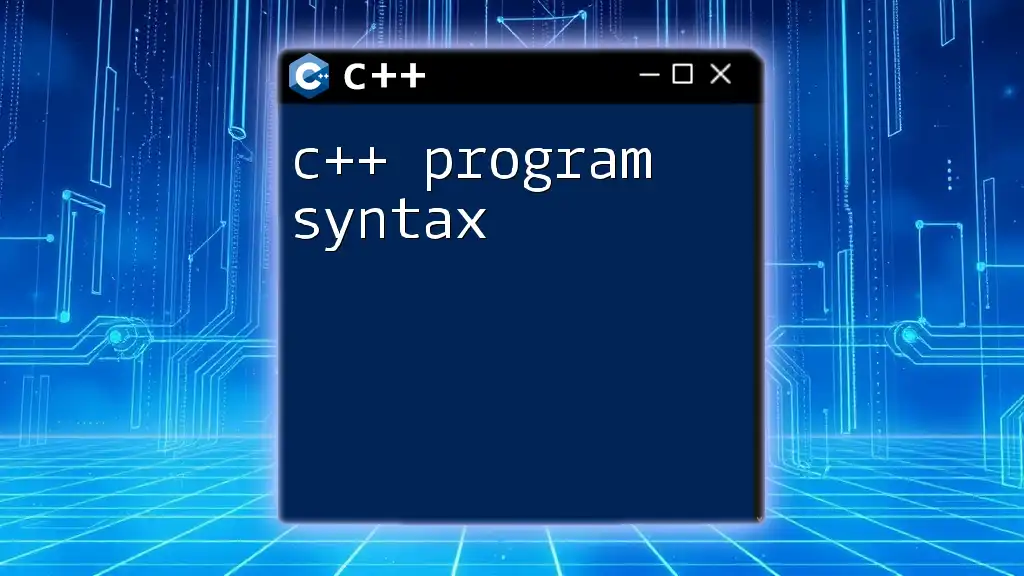
Basic Syntax and Structure
Understanding the basic syntax of C++ is essential for effective programming. C++ relies heavily on clarity and structure.
Comments and Documentation
Comments are vital for documenting code, making it easier for others (or yourself at a later time) to understand what the code does.
Example: Demonstrating both single-line and multi-line comments:
// This is a single line comment
/* This is a
multi-line comment */
Data Types and Variables
C++ supports various data types. Understanding these will help you define variables effectively.
Common data types include:
- `int`: Represents integer values.
- `float`: Represents floating-point numbers.
- `char`: Represents single characters.
- `std::string`: Represent sequences of characters (strings).
Example: Declaring and initializing variables in C++:
int age = 25;
float salary = 50000.50;
char grade = 'A';
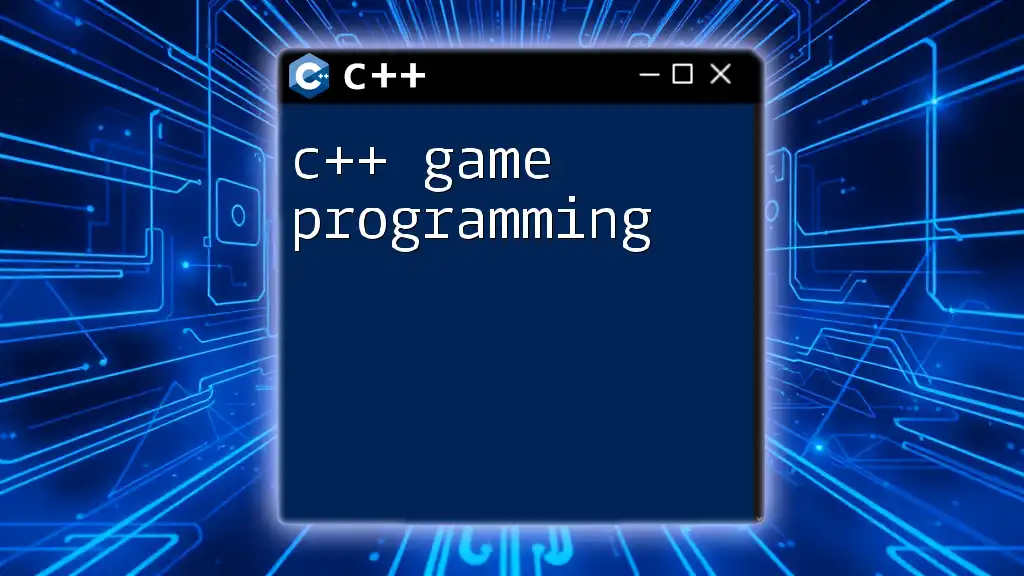
Control Structures
C++ allows you to control the flow of your program using various constructs.
Conditional Statements
Conditional statements help you execute specific blocks of code based on certain conditions. The primary forms are `if`, `else if`, `else`, and `switch` statements.
Example: Control flow using an `if` statement:
int x = 10;
if (x > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Non-positive number" << std::endl;
}
Loops
Loops facilitate the repetition of code blocks. C++ provides different looping constructs, including `for`, `while`, and `do-while`.
Example: Using a `for` loop to iterate over a range of numbers:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
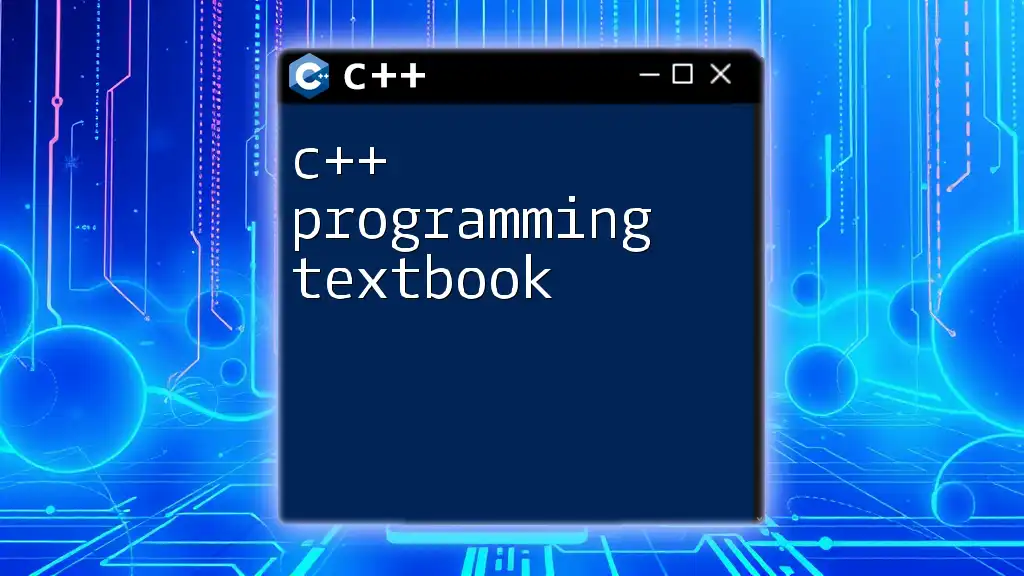
Functions and Overloading
Functions encapsulate code blocks that perform specific tasks, making your code modular and re-usable.
Function Declaration and Definition
You define a function once and invoke it multiple times. The declaration includes the function’s name, return type, and parameters.
Example: A simple function that prints a greeting:
void greet() {
std::cout << "Hello, C++" << std::endl;
}
Function Overloading
C++ allows you to have multiple functions with the same name but different parameter lists, known as function overloading. This feature enhances code readability and usability.
Example: Overloading a function to handle different data types:
void print(int n) {
std::cout << "Integer: " << n << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
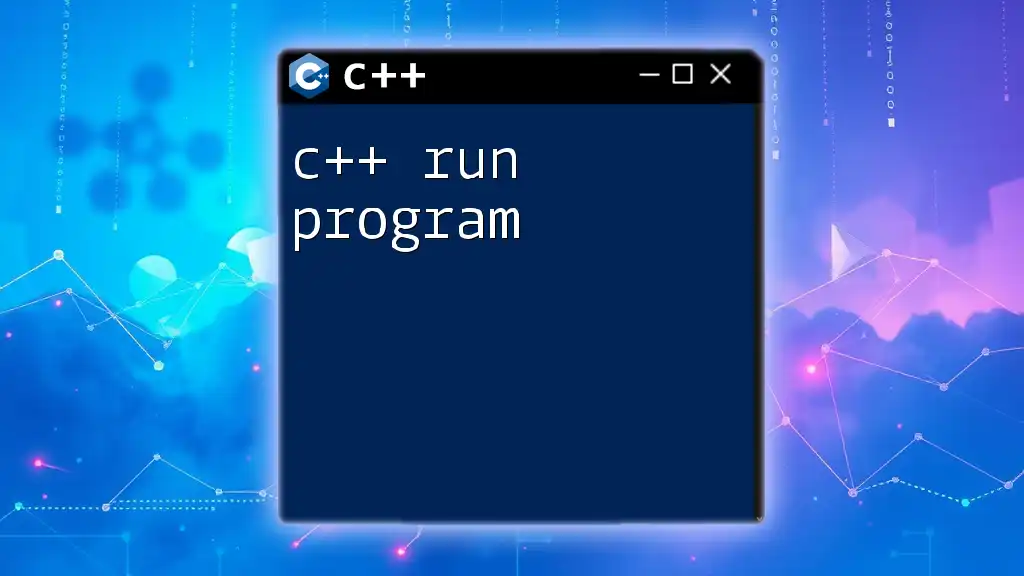
Advanced Topics
As you become proficient with C++, you may want to explore advanced concepts that deepen your understanding and capability as a programmer.
Templates and Generic Programming
Templates enable the creation of functions and classes that work with any data type. This leads to code reusability and flexibility.
Example: A simple function template:
template <typename T>
void display(T value) {
std::cout << value << std::endl;
}
Here, `display()` can accept any data type, making your functions more generic.
Exception Handling
Error management is crucial in programming, and C++ provides mechanisms for handling exceptions. The key components are `try`, `catch`, and `throw`.
Example: Basic use of exception handling:
try {
throw std::runtime_error("An error occurred");
} catch (std::exception &e) {
std::cout << e.what() << std::endl;
}
This allows you to gracefully handle errors without crashing your program.
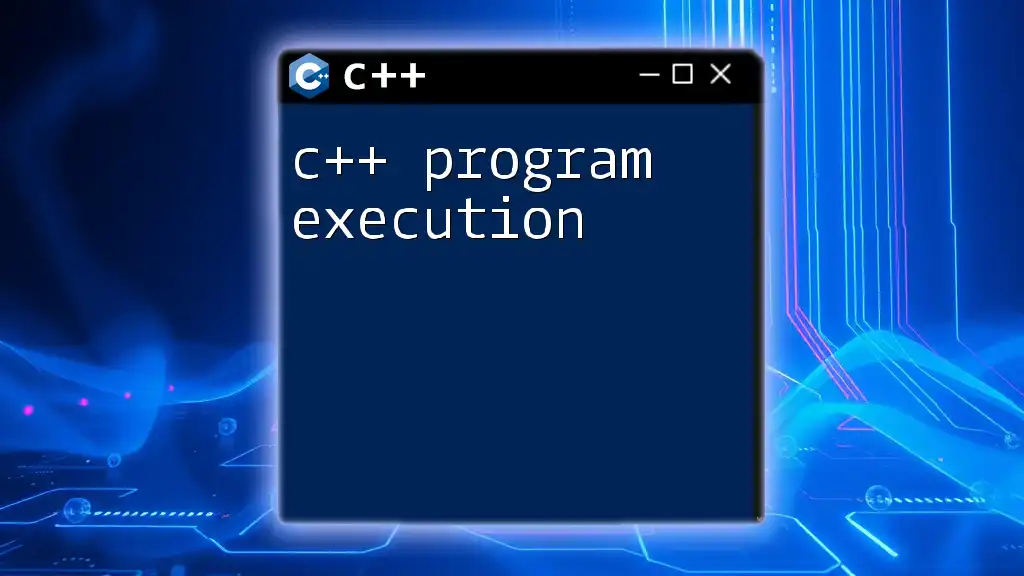
Conclusion
C++ is a powerful language that combines performance with complex functionalities, making it a preferred choice among experienced programmers. Understanding its core principles—such as OOP, STL, memory management, and advanced features—will significantly enhance your ability to develop robust applications.
By mastering C++ for programmers, you open doors to various fields, including game development, system programming, and robotics. Practice regularly, explore advanced resources, and continually challenge yourself to grow as a C++ programmer.