C++ offers Python programmers powerful features like manual memory management and performance optimization, enabling them to leverage lower-level programming capabilities for more efficient applications.
Here’s a simple example demonstrating the difference in syntax for a class definition and function implementation between Python and C++:
#include <iostream>
class Greeting {
public:
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
Greeting greet;
greet.sayHello();
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-performance programming language that was developed as an extension of the C programming language. It incorporates features such as object-oriented programming, which enables developers to create programs in a more organized and reusable manner. C++ offers enhanced control over system resources and memory management, making it an ideal choice for performance-critical applications like game development, system/software development, and real-time simulations.
C++ Syntax vs. Python Syntax
When transitioning from Python to C++, programmers will notice several syntactical differences. For example, while Python is dynamically typed, C++ is statically typed, meaning that you must declare the types of your variables.
Basic Syntax Differences
- In C++, every statement ends with a semicolon. This is not the case in Python, where newlines often signify the end of a statement.
Example of a simple variable declaration in both languages:
int a = 10; // C++
a = 10 # Python
Understanding these distinctions is crucial for making the transition smoother.
Data Types in C++
C++ has a rich set of built-in data types, which include both primitive types and user-defined types.
Primitive Data Types
The basic data types in C++ are:
- int: for integers
- char: for single characters
- float: for floating-point numbers
- double: for double-precision floating-point numbers
Example declarations:
int age = 25; // Integer
char initial = 'A'; // Character
float height = 5.9f; // Float
double pi = 3.14159; // Double
User-defined Data Types
In addition to primitive types, C++ allows developers to define their own data types using structs and classes, enabling a high degree of customization.
Example of a struct:
struct Person {
std::string name;
int age;
};
This powerful feature allows C++ programmers to model real-world entities more effectively.
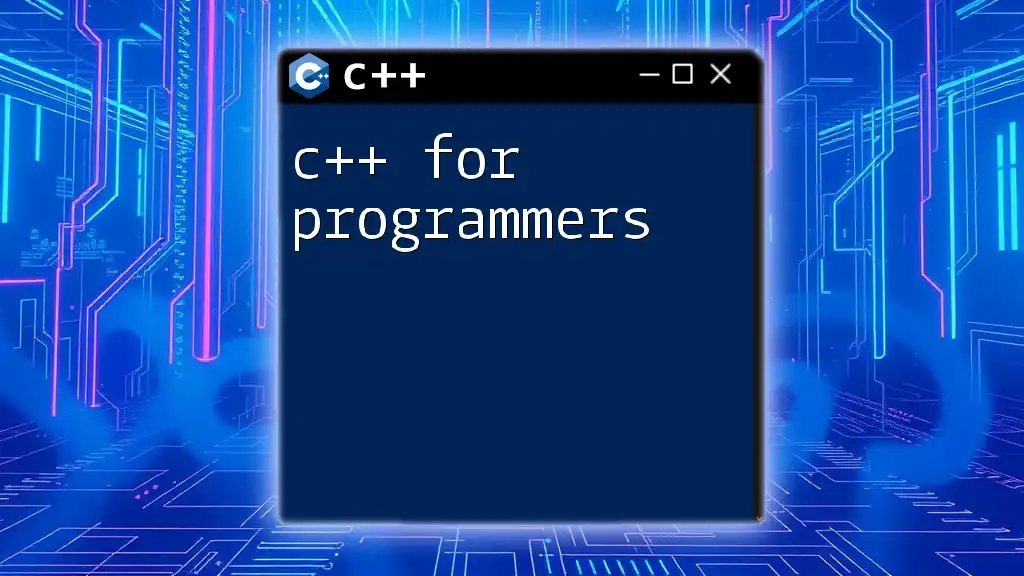
Control Structures
Conditionals and Loops
Control structures are vital for decision-making in programming. C++ provides several ways to implement conditional logic.
If-Else Statements in C++
These statements allow the execution of different blocks of code based on a condition.
Example:
if (age < 18) {
std::cout << "Minor";
} else {
std::cout << "Adult";
}
For Loop vs. While Loop
C++ supports both for loops and while loops, offering greater flexibility compared to Python.
Example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i;
}
int j = 0;
while (j < 5) {
std::cout << j++;
}
Understanding these structures in C++ allows Python programmers to translate their logic directly into this language.
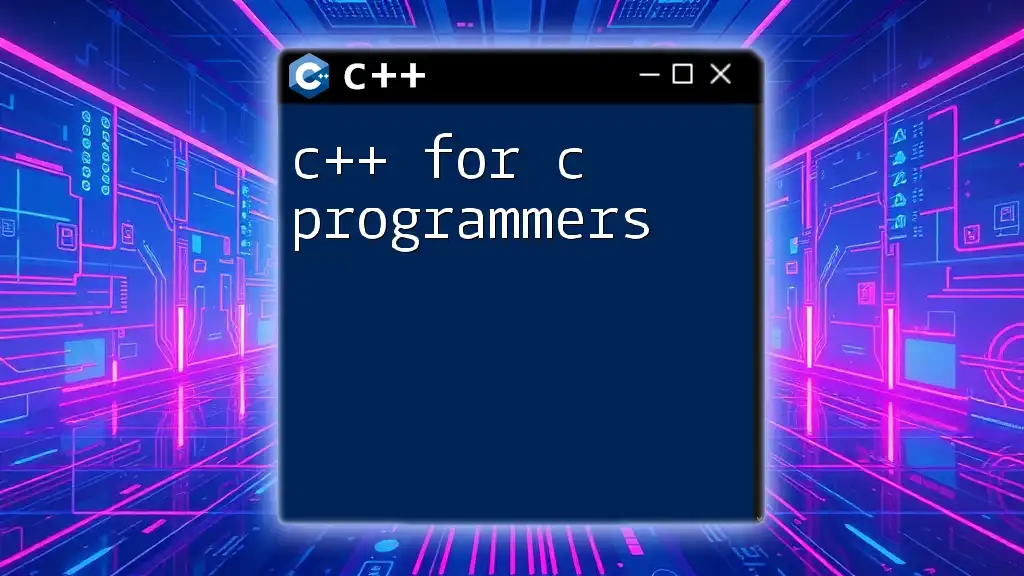
Functions in C++
Function Declaration and Definition
Functions are essential for code organization and reuse. While Python allows for easy declarations, C++ requires a bit more structure.
Example of function definition in C++:
int add(int a, int b) {
return a + b;
}
Function Overloading
One of the unique features of C++ is function overloading, which allows multiple functions to have the same name but differ in parameters.
Example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This feature can simplify function names and enhance code readability.
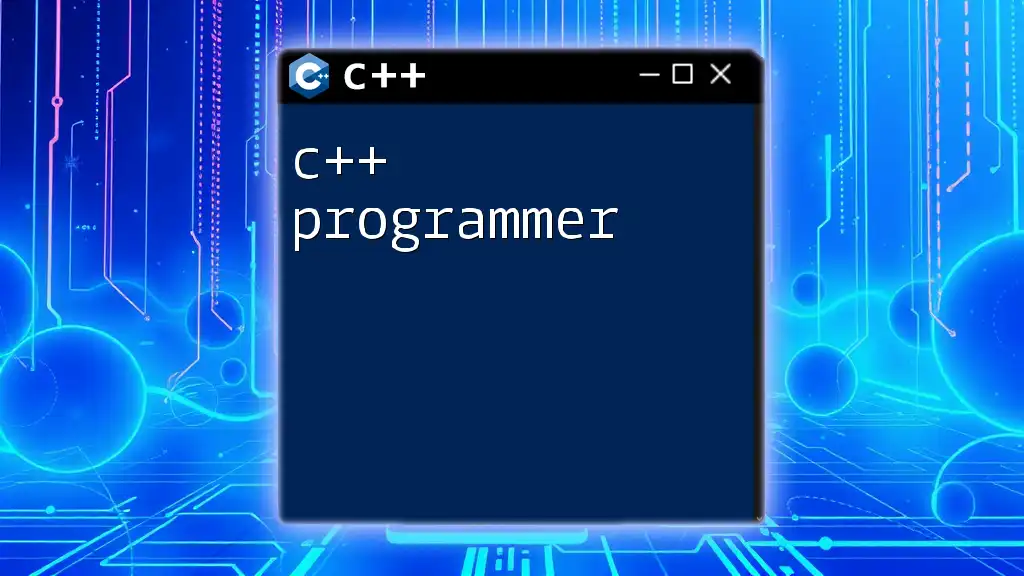
Object-Oriented Programming in C++
Classes and Objects
C++ is fundamentally an object-oriented programming (OOP) language. Learning how to create and manipulate classes and objects is essential.
Example of a basic class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!";
}
};
Here, `Dog` is a class with a method `bark`.
Inheritance
Inheritance allows a new class to adopt properties and behaviors from an existing class, promoting code reusability.
Example of inheritance:
class Animal {
public:
void eat() {}
};
class Dog : public Animal {
public:
void bark() {}
};
This feature emphasizes the polymorphic nature of C++, allowing programmers to create flexible and extensible code structures.
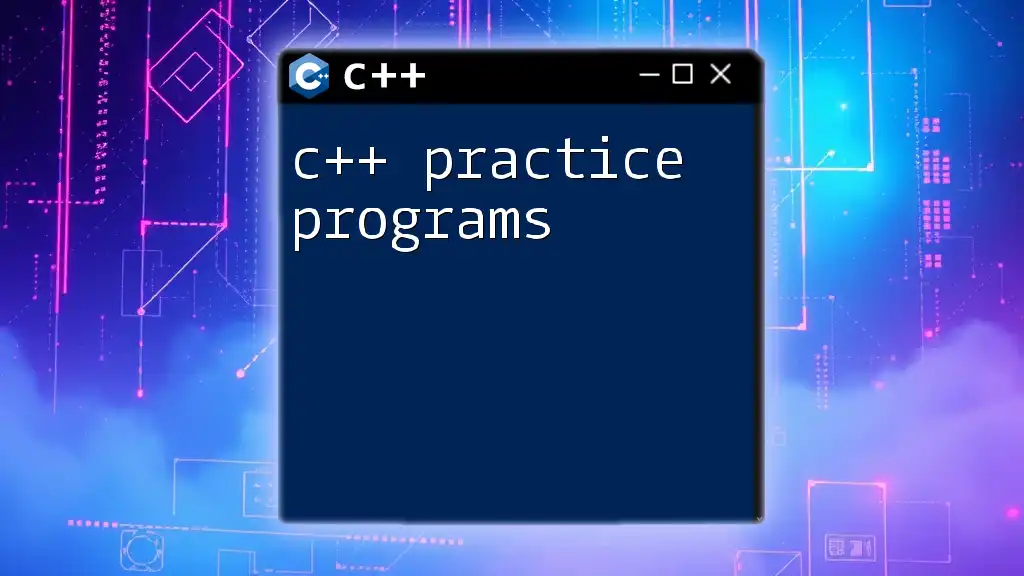
C++ Libraries and Frameworks
Standard Template Library (STL)
C++ boasts the Standard Template Library (STL), which provides a collection of useful classes and functions, particularly for data structures like vectors, lists, and maps.
Example of using vectors:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
STL facilitates efficient algorithm implementation and data management within C++ programs.
Comparison to Python Libraries
While Python has numerous libraries like NumPy, pandas, and Django, C++ primarily relies on STL for collection types and algorithms. Understanding STL will give Python developers a solid foundation for translating their skills to C++ effectively.
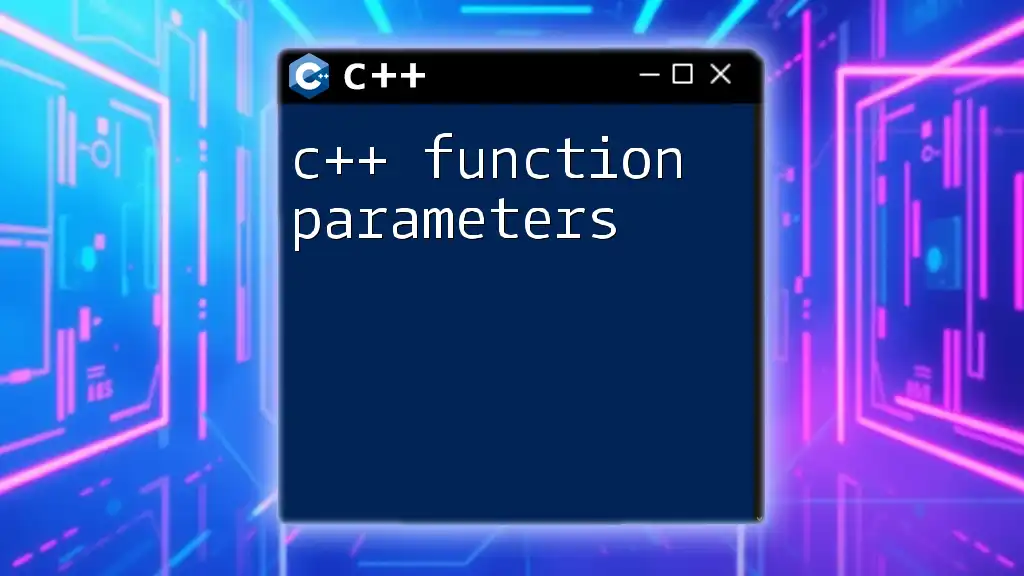
Memory Management in C++
Pointers and References
C++ provides low-level memory management features through the use of pointers and references. This differs significantly from Python's automatic memory management.
Example of pointers:
int age = 25;
int *p = &age; // Pointer to an integer
Learning to manipulate pointers is crucial for performance optimization in C++.
Dynamic Memory Allocation
Dynamic memory allocation allows programmers to request memory during runtime. C++ uses `new` and `delete` for this purpose.
Example:
int* arr = new int[5]; // Creating an array dynamically
delete[] arr; // Deleting allocated memory
Understanding these concepts is essential for effective memory management in C++ programs.
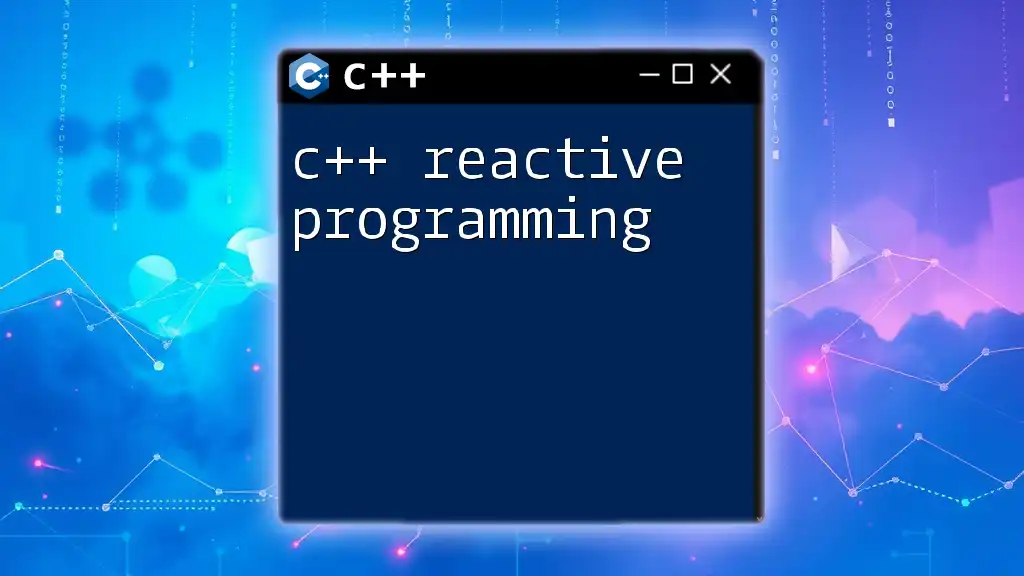
Error Handling in C++
Exception Handling
C++ offers robust exception handling to manage errors gracefully. Using `try`, `catch`, and `throw`, programmers can handle unforeseen issues efficiently.
Example:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
std::cout << e.what();
}
Understanding exception handling is paramount to ensuring that your C++ applications remain stable and user-friendly.
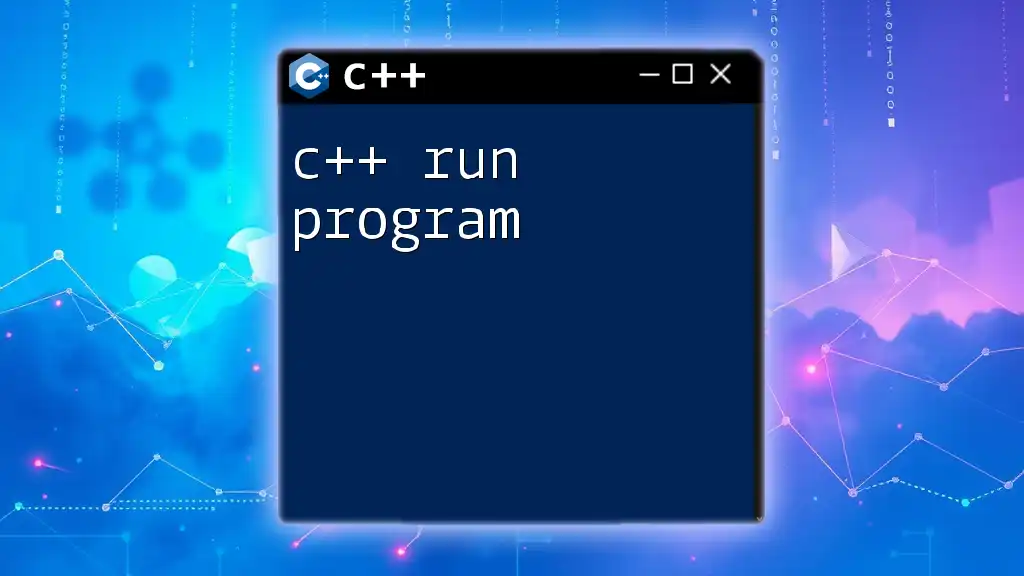
Conclusion
In conclusion, mastering C++ for Python programmers provides a unique opportunity to enhance your programming skills and expand your career horizons. The differences in syntax, data types, memory management, and error handling are critical to understanding C++. With this knowledge, you can harness the power of C++ in your projects and benefit from its performance capabilities.
Resources for Further Study
To deepen your understanding, consider exploring recommended books, online courses, and programming communities that focus on C++. Engaging with these resources will solidify your knowledge and prepare you for a successful transition from Python to C++.
By embracing the challenges and strengths of C++, you can elevate your programming prowess to new heights.