C++ network programming involves using C++ libraries and sockets to enable communication between devices over a network, allowing for the development of client-server applications.
Here's a simple example of a TCP client that connects to a server using C++:
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <unistd.h>
int main() {
int sock;
struct sockaddr_in server;
sock = socket(AF_INET, SOCK_STREAM, 0);
server.sin_family = AF_INET;
server.sin_port = htons(8888);
inet_pton(AF_INET, "127.0.0.1", &server.sin_addr);
if (connect(sock, (struct sockaddr *)&server, sizeof(server)) < 0) {
std::cerr << "Connection error" << std::endl;
return 1;
}
std::cout << "Connected to the server!" << std::endl;
close(sock);
return 0;
}
Understanding Networking Basics
What is Networking?
Networking refers to the practice of connecting computers and devices to share resources and communicate. There are various types of networks:
- Local Area Network (LAN): Connects computers within a limited area, like a home or office.
- Wide Area Network (WAN): Spans a larger geographical area, such as cities or countries.
Protocols are standardized rules that define how data is transmitted over networks. Two key protocols are:
- TCP/IP (Transmission Control Protocol/Internet Protocol): Ensures reliable communication by establishing a connection before data is sent.
- UDP (User Datagram Protocol): A faster but less reliable protocol that sends data without establishing a connection.
Key Networking Concepts
IP Addressing: An IP address is a unique identifier for each device connected to a network. There are two versions:
- IPv4: Utilizes a 32-bit address space, e.g., `192.168.1.1`.
- IPv6: Uses a 128-bit address space to accommodate the growing number of devices.
Ports and Sockets: Ports allow multiple applications on the same device to communicate. A socket is an endpoint for sending or receiving data across a network. It is defined by an IP address and a port number.
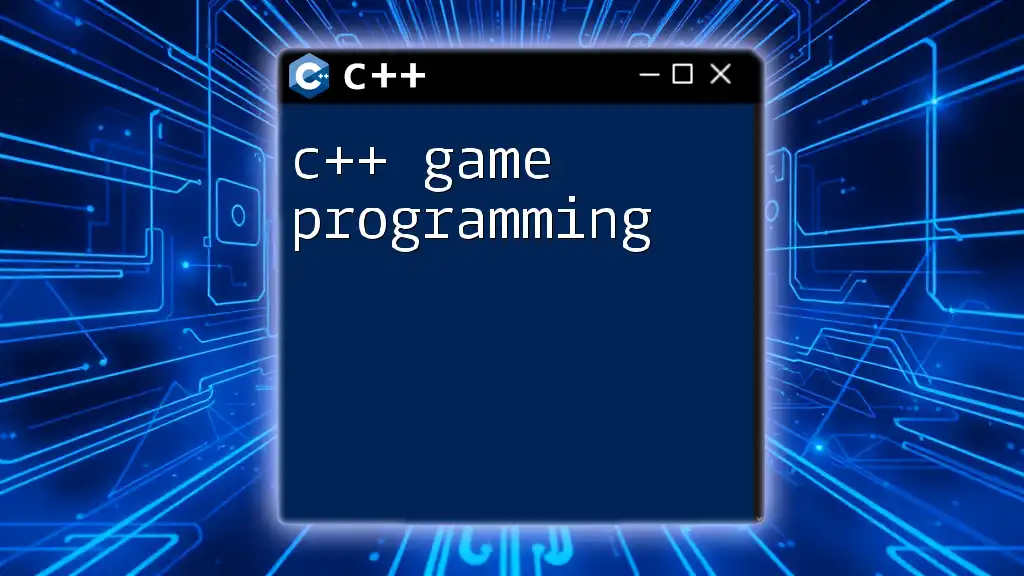
Setting Up Your C++ Development Environment
Required Libraries
Boost Asio is one of the most widely-used libraries for C++ network programming. It offers functionalities for asynchronous input and output, enabling the development of scalable applications. To install Boost Asio, follow the instructions for your development environment.
Additionally, some alternatives like POCO and Qt Network provide robust networking capabilities, allowing developers to choose based on project requirements.
Development Tools and IDEs
Recommended IDEs for C++ development include:
- Visual Studio: Excellent for Windows applications with extensive debugging tools.
- Code::Blocks: A lightweight and cross-platform environment.
To compile and run network applications, open the command line and use commands such as:
g++ -o my_app my_app.cpp -lboost_system -lpthread
./my_app
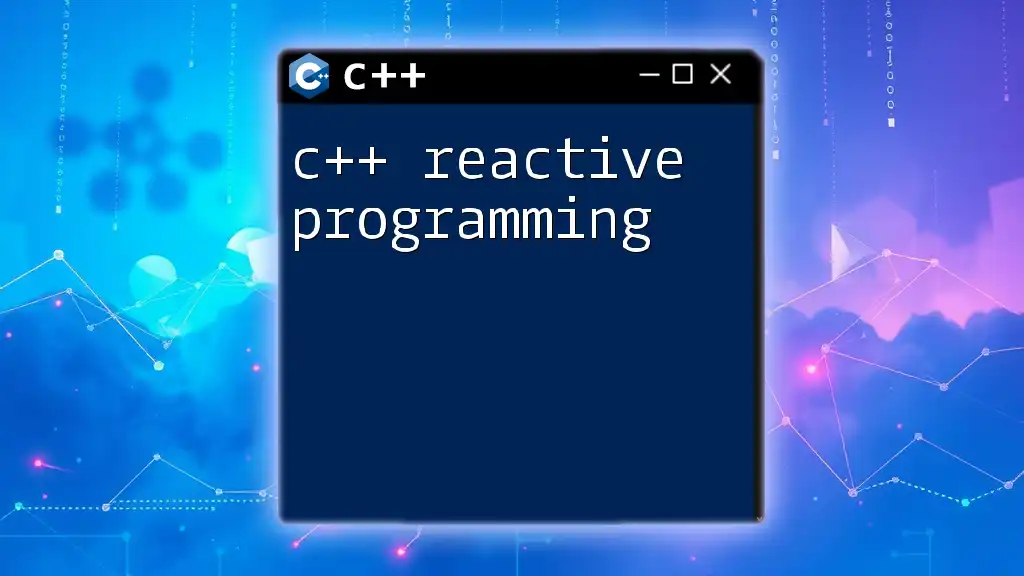
Writing a Basic C++ Network Application
Creating a Simple TCP Client
A TCP client connects to a server and sends data. This follows the client-server model, where the server waits for incoming connections.
Here’s a simple example of a TCP client using Boost Asio:
#include <iostream>
#include <boost/asio.hpp>
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::socket socket(io_context);
// Connect to server
socket.connect({boost::asio::ip::make_address("127.0.0.1"), 8080});
std::cout << "Connected to server!" << std::endl;
return 0;
}
In this code, `boost::asio::io_context` handles I/O operations, and `boost::asio::ip::tcp::socket` creates a socket for communication. The `connect` method establishes a connection to the server at the specified IP address and port.
Creating a Simple TCP Server
The TCP server listens for incoming connections. Here’s an example of a TCP server using Boost Asio:
#include <iostream>
#include <boost/asio.hpp>
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::acceptor acceptor(io_context, {boost::asio::ip::tcp::v4(), 8080});
boost::asio::ip::tcp::socket socket(io_context);
// Accept a connection
acceptor.accept(socket);
std::cout << "Client connected!" << std::endl;
return 0;
}
The server is set to accept connections on port 8080. `boost::asio::ip::tcp::acceptor` listens for incoming client requests, while the `accept` method establishes a connection when a client attempts to connect.
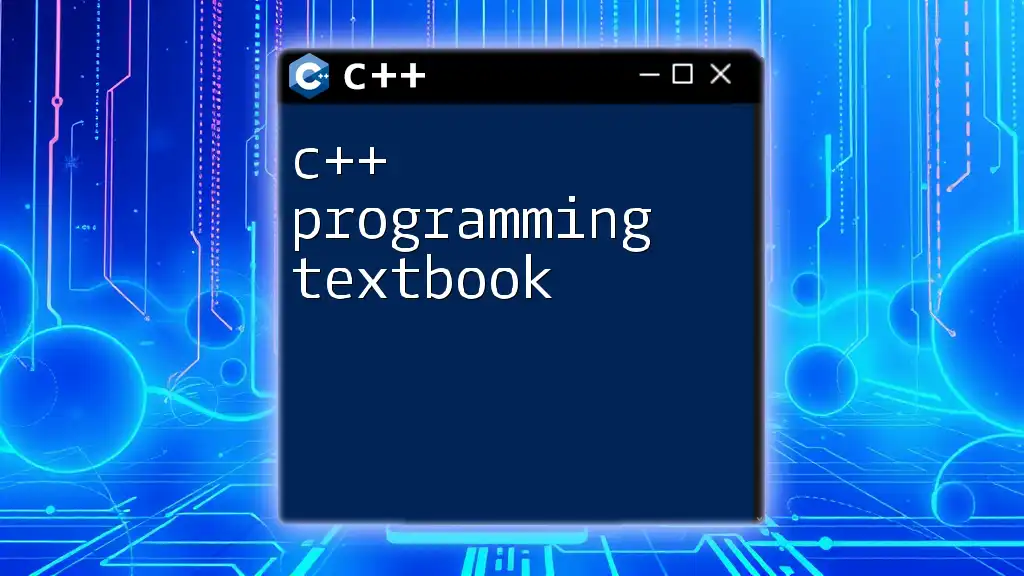
Advanced C++ Network Programming Concepts
Asynchronous Networking
Asynchronous networking allows your application to handle multiple tasks at once, improving performance and responsiveness. Rather than blocking execution until a task is complete, asynchronous operations enable the program to continue running while waiting for data.
Here’s an example of using asynchronous operations with Boost Asio:
void on_accept(boost::asio::ip::tcp::socket socket) {
// Handle client connection
}
In this case, `on_accept` can be invoked as a callback when a client connects, preventing the server from freezing while it waits for connections.
Handling Multiple Connections
To manage multiple client connections, you can employ multi-threading. Each connection can be handled in a separate thread, allowing concurrent processing. Here’s a code snippet for a multi-threaded TCP server:
#include <iostream>
#include <thread>
#include <boost/asio.hpp>
void handle_client(boost::asio::ip::tcp::socket socket) {
// Code to interact with the client
}
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::acceptor acceptor(io_context, {boost::asio::ip::tcp::v4(), 8080});
while (true) {
boost::asio::ip::tcp::socket socket(io_context);
acceptor.accept(socket);
std::thread(handle_client, std::move(socket)).detach();
}
return 0;
}
In this example, the main loop continuously accepts incoming connections, creating a new thread for each client to handle interactions concurrently.
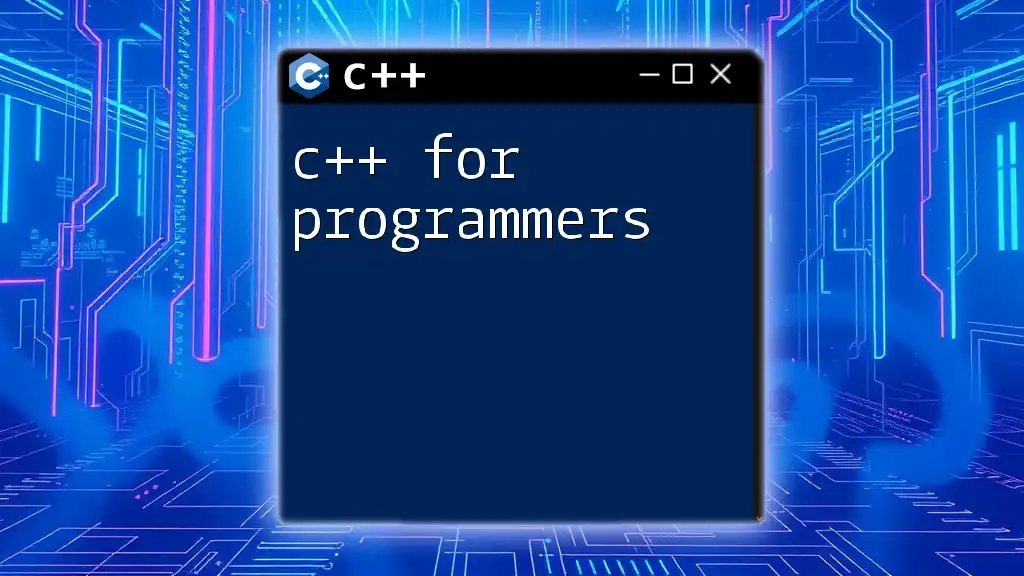
Debugging and Error Handling
Common Networking Issues
Networking applications often face challenges such as connection timeouts and data transmission failures. These can stem from various sources like network congestion or incorrect configurations.
Handling Network Errors in C++
Error handling is crucial for network applications. Utilize exception handling to manage errors gracefully. Here's a simple error handling example for a socket connection:
try {
socket.connect({boost::asio::ip::make_address("127.0.0.1"), 8080});
} catch (const boost::system::system_error& e) {
std::cerr << "Error connecting to server: " << e.what() << std::endl;
}
Debugging Tips
For effective debugging, utilize tools like Wireshark to monitor network traffic and identify issues. Logging messages in your application can also help trace problems.
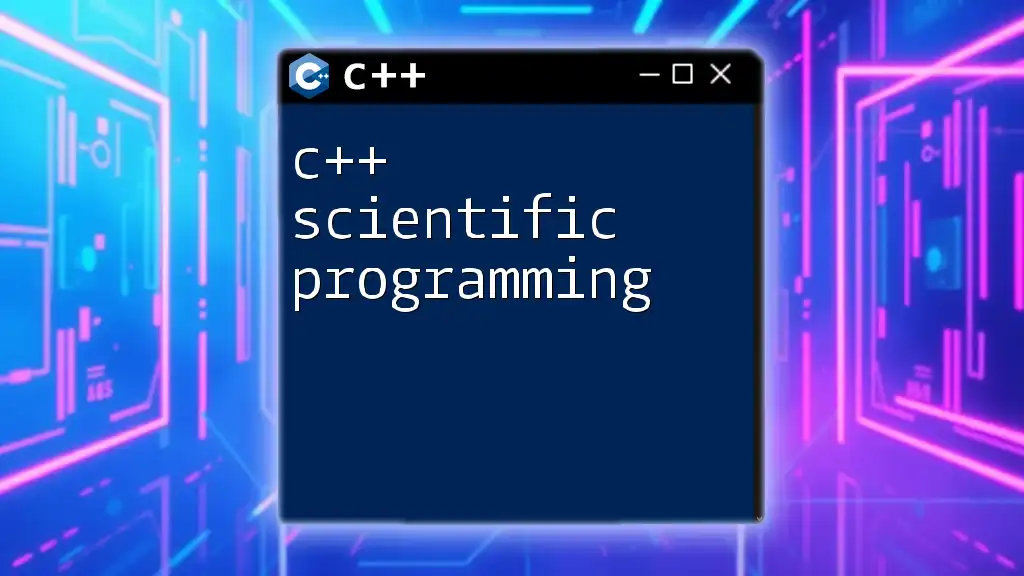
Security Considerations in C++ Networking
Importance of Security
Network security is vital, especially for applications handling sensitive information. Common threats include DDoS attacks and data interception.
Implementing Basic Security Measures
To protect communications, data encryption is essential. Implementing SSL/TLS ensures secure data transmission. Here’s a basic overview of using SSL in a C++ application:
// Example of initializing SSL might involve including the openssl header files
This requires integrating a library like OpenSSL, which provides functions to secure socket connections.
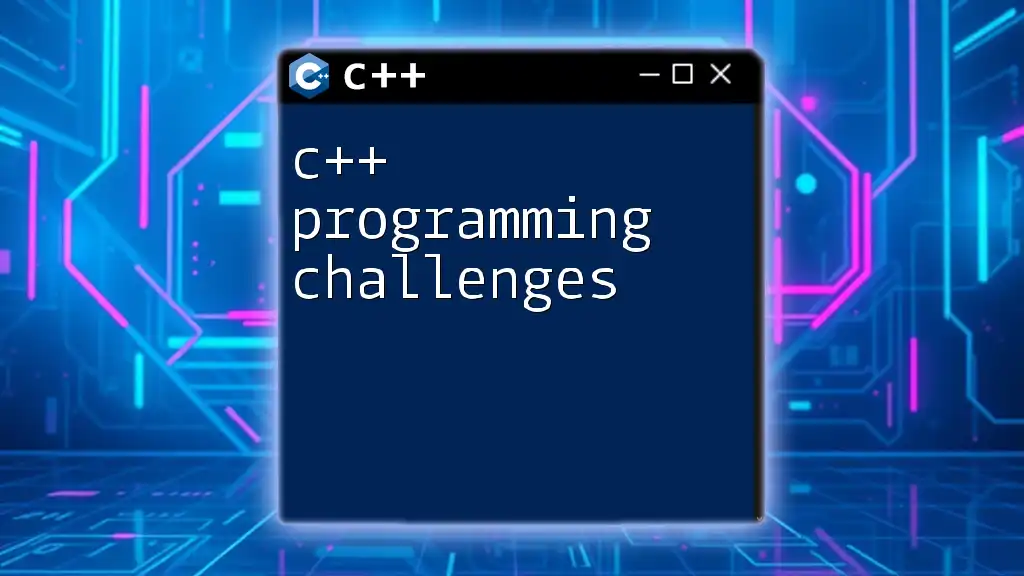
Conclusion
In this guide, you've learned the fundamentals of C++ network programming, including the setup of a development environment, client-server architecture, asynchronous operations, handling multiple connections, debugging techniques, and essential security measures.
By practicing these concepts and building your projects, you will deepen your understanding and capability in C++ network programming. Consider exploring additional resources such as books, online courses, and the documentation for libraries like Boost Asio to further enhance your skills.