C++ reactive programming is a programming paradigm that focuses on asynchronous data streams and the propagation of change, enabling developers to respond to events and data as they occur.
Here's a simple code snippet demonstrating a basic reactive programming concept using the RxCPP library:
#include <rxcpp/rx.hpp>
#include <iostream>
int main() {
auto values = rxcpp::observable<>::range(1, 5)
.map([](int v) { return v * v; })
.subscribe([](int v) { std::cout << "Value: " << v << std::endl; });
return 0;
}
What is Reactive Programming?
Reactive programming is a programming paradigm oriented around data flows and the propagation of change. In simpler terms, it's a way of building systems that respond to changes, such as new data or events. This adaptability is especially important in today's fast-paced software landscapes, where applications need to handle asynchronous data and events efficiently.
The key characteristics of reactive systems include responsiveness, resilience, elasticity, and message-driven architectures. These attributes are crucial for creating applications that are not only reliable but also scalable.
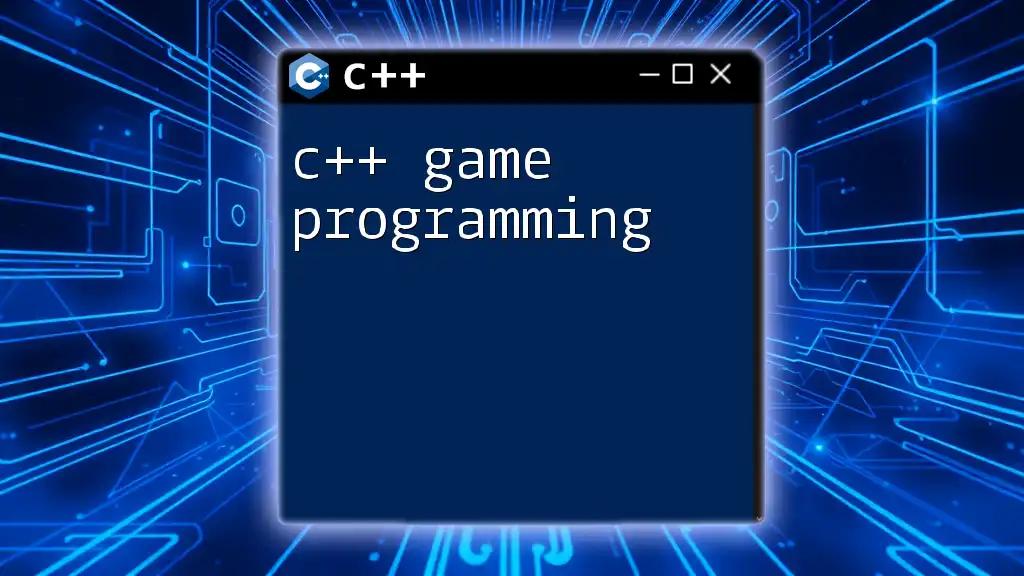
The Benefits of Reactive Programming
Responsiveness
Reactive programming allows systems to remain responsive under varying load conditions. When changes occur, such as a user input or an incoming network packet, the system reacts immediately, ensuring smooth user experiences.
Resilience
Due to its emphasis on asynchronous operations and distributed architectures, reactive programming enhances system resilience. If one component fails, other parts of the system can still function correctly.
Scalability
Reactive applications are designed to scale naturally. As demand grows, new resources can be added without rewriting large sections of code, allowing systems to handle more load efficiently.
Flexibility
Reactive programming supports a declarative style of coding, allowing developers to write more concise, readable code that is easier to maintain and extend.
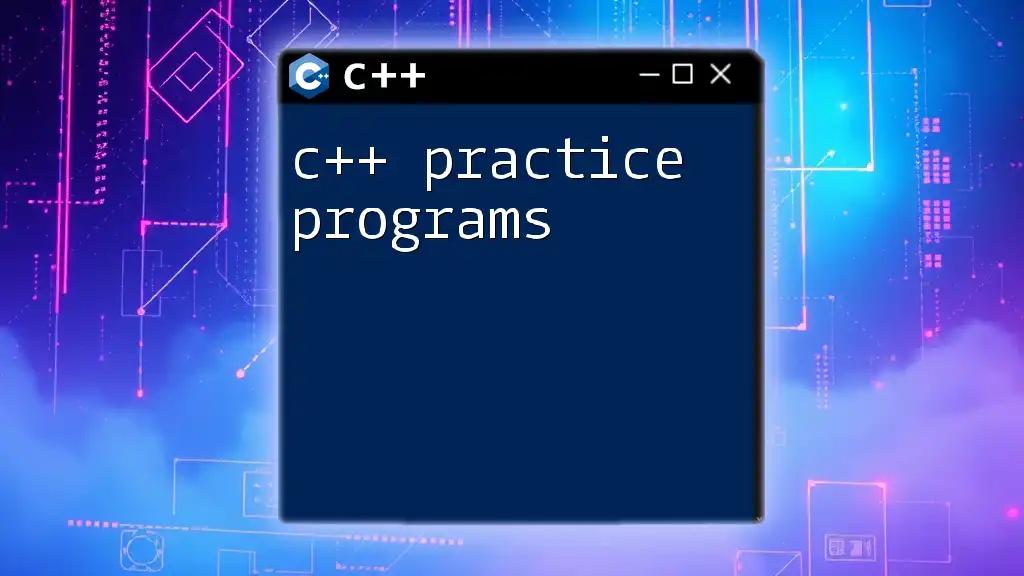
Use Cases of Reactive Programming in C++
Reactive programming shines in several scenarios:
- Real-time Data Processing: Ideal for applications that manage streams of data, like sensor data in IoT.
- User Interfaces: Enhances responsiveness in UI frameworks by reacting to user actions immediately.
- Event-Driven Systems: Facilitates responsiveness in systems that react to events, such as messaging apps.
- Networked Applications: Optimizes server-client communications by handling multiple sessions concurrently.
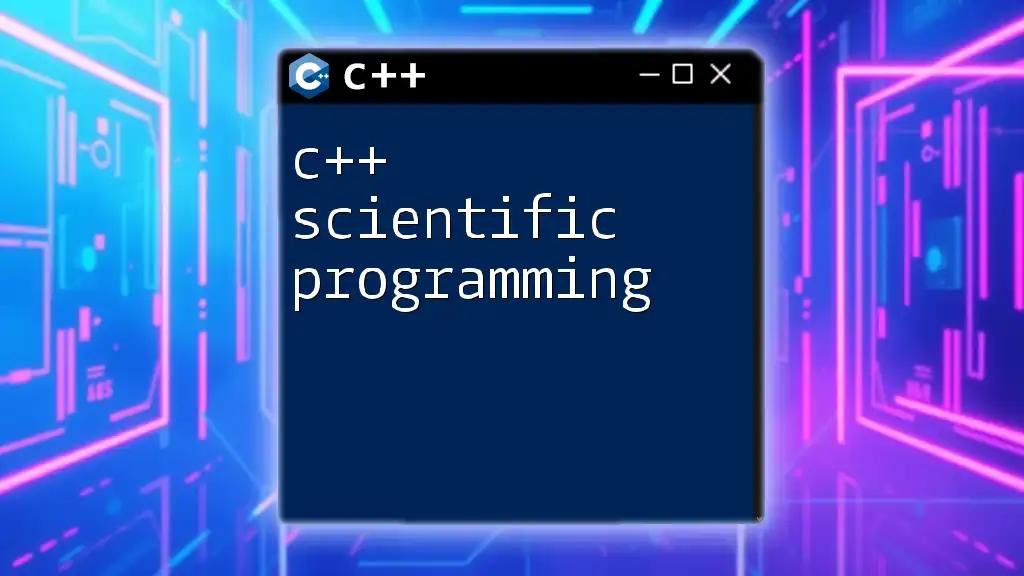
Core Concepts of Reactive Programming
Reactive Streams
Reactive streams provide a standard for asynchronous stream processing with non-blocking backpressure. Backpressure is critical as it prevents the system from being overwhelmed by excessive data.
Here's a simple example of implementing a reactive stream in C++:
#include <rxcpp/rx.hpp>
int main() {
auto rx_stream = rxcpp::observable<>::range(1, 10);
rx_stream.subscribe([](int value) {
std::cout << "Received: " << value << std::endl;
});
return 0;
}
Observables and Subscribers
In reactive programming, Observables are the data producers that emit values over time, while Subscribers react to these values. The interaction between observables and subscribers is central to understanding reactive programming.
For example, here’s how to create an observable in C++:
#include <rxcpp/rx.hpp>
int main() {
auto observable = rxcpp::observable<>::just(10, 20, 30);
observable.subscribe([](int value) {
std::cout << "Value: " << value << std::endl;
});
return 0;
}
Operators in Reactive Programming
Reactive programming includes various operators that transform data streams. Common operators are `map`, `filter`, and `merge`. These allow developers to manipulate data flows effectively.
For example, using the `map` operator:
auto observable = rxcpp::observable<>::range(1, 5);
observable
.map([](int value) { return value * value; })
.subscribe([](int value) {
std::cout << "Squared: " << value << std::endl;
});
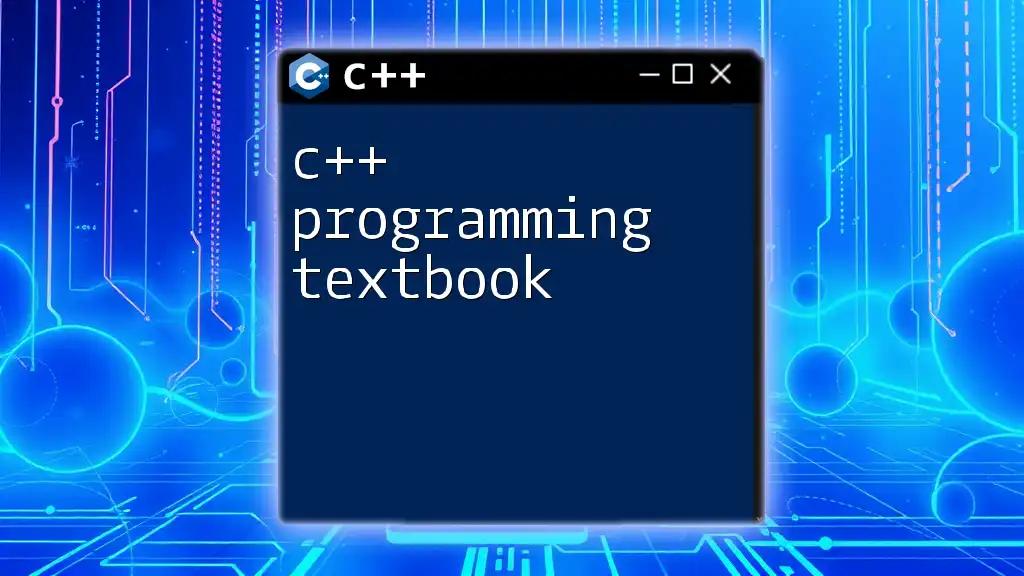
Libraries for Reactive Programming in C++
Overview of Popular Libraries
When working with C++ reactive programming, several libraries can assist developers. RxCPP is the most widely used, providing an extensive suite of functionalities.
Getting Started with RxCPP
To get started with RxCPP, you'll need to install it, often using package managers like vcpkg or building from source.
Here’s a basic example that showcases the core of reactive programming in action:
#include <rxcpp/rx.hpp>
int main() {
auto source = rxcpp::observable<>::interval(std::chrono::milliseconds(100));
source.subscribe([](auto i) {
std::cout << "Tick: " << i << std::endl;
});
std::this_thread::sleep_for(std::chrono::seconds(1)); // Keep the program running
return 0;
}
Advanced Usage of RxCPP
Composing Observables
With RxCPP, it's beneficial to compose multiple observables to forge complex data flows. Here's how to merge two streams:
auto source1 = rxcpp::observable<>::interval(std::chrono::milliseconds(100)).map([](int i) { return "Source1: " + std::to_string(i); });
auto source2 = rxcpp::observable<>::interval(std::chrono::milliseconds(150)).map([](int i) { return "Source2: " + std::to_string(i); });
auto merged = source1.merge(source2);
merged.subscribe([](const std::string& value) {
std::cout << value << std::endl;
});
Error Handling
Error management is crucial in reactive programming. RxCPP allows developers to manage errors gracefully and continue processing streams.
auto failing_observable = rxcpp::observable<>::create<int>([](rxcpp::subscriber<int> s) {
s.on_next(1);
s.on_error(std::runtime_error("An error occurred!"));
});
failing_observable.subscribe(
[](int value) { std::cout << "Received: " << value << std::endl; },
[](std::exception_ptr eptr) { std::cout << "Error caught!" << std::endl; }
);
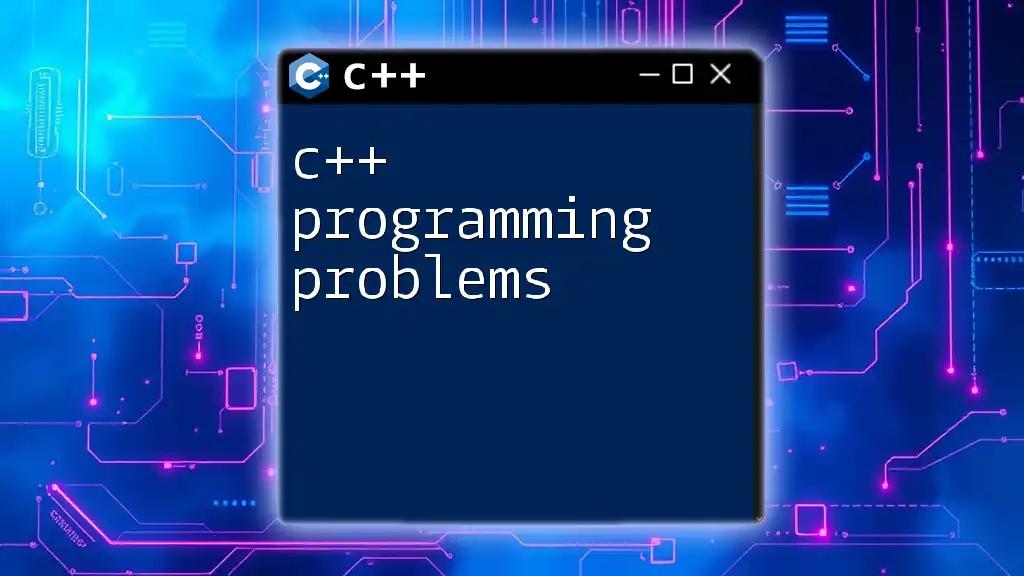
Designing Reactive Applications in C++
Applying Reactive Principles
When designing reactive applications, it's essential to apply core reactive principles. Focus on resilience, event-driven communication, and separation of concerns to craft effective systems.
Event-Driven Architecture
In reactive programming, the architecture focuses on handling events efficiently. For instance, a basic event-driven application could react to user inputs or sensor notifications.
Testing Reactive Applications
Testing can be challenging in reactive programming due to its asynchronous nature. Best practices include using mocking libraries and writing unit tests that simulate different data flows.
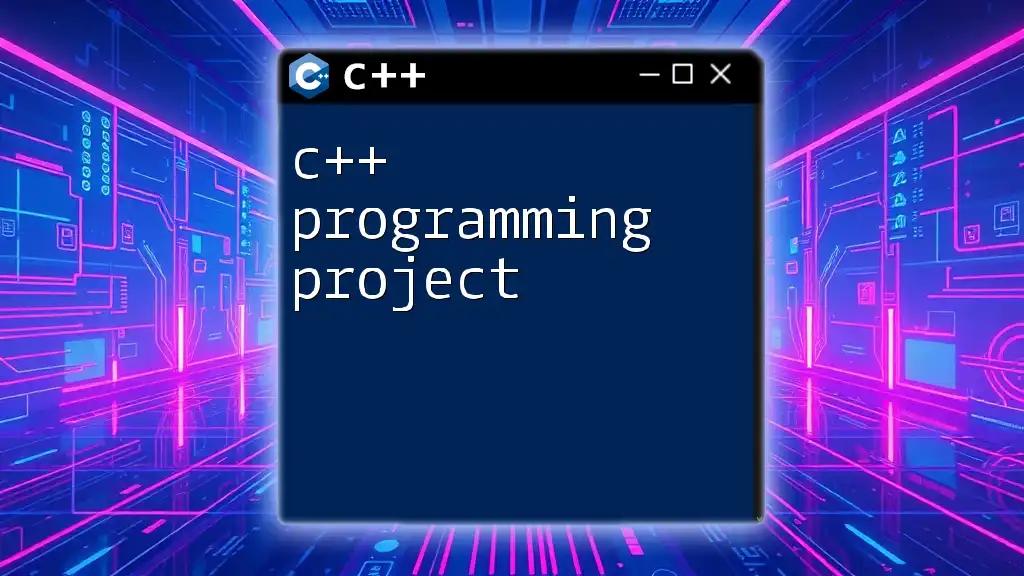
Common Pitfalls in Reactive Programming
Overcomplication
One of the most significant risks in adopting reactive programming is overcomplicating the design with convoluted streams. Focus on simplicity and clarity to maintain readability.
Resource Management
Proper resource management is crucial to prevent memory leaks. Always ensure streams complete properly and subscribers unsubscribe when no longer needed.
Debugging Reactive Code
Debugging in reactive programming might be tricky due to the asynchronous flow. Utilize logging mechanisms and debuggers that can handle asynchronous contexts for effective investigation of issues.
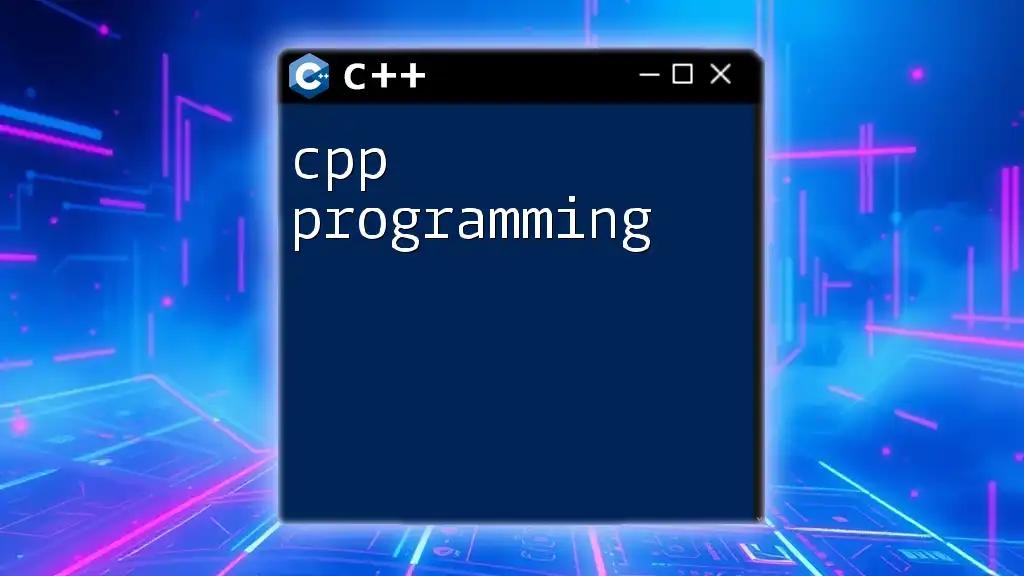
Conclusion
As reactive programming evolves, it will increasingly shape how we build C++ applications. The flexibility it provides will allow developers to create seamless, responsive experiences for users. Embracing the reactive paradigm can enhance how software systems manage complexity and scale.
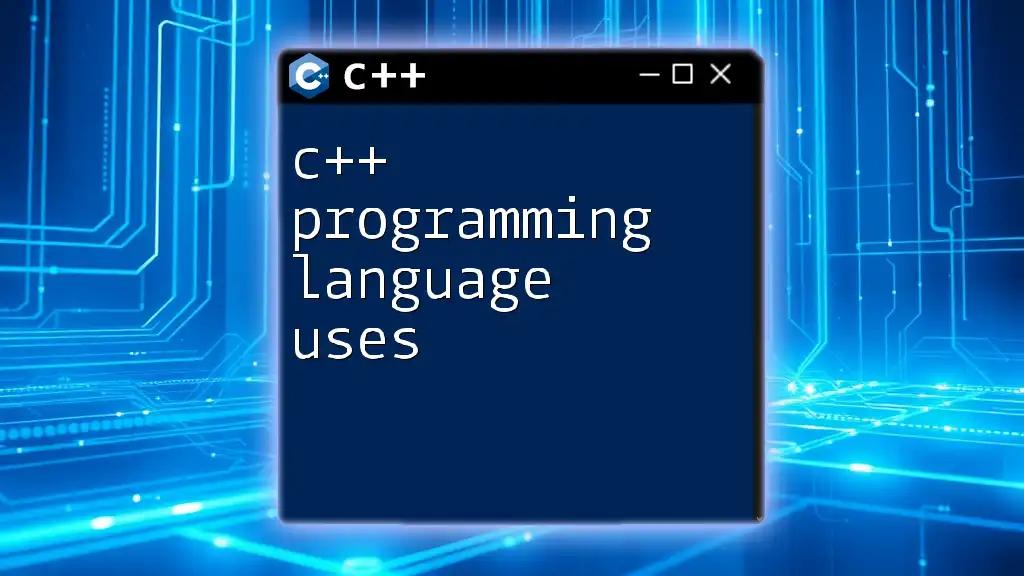
Resources for Further Learning
To deepen your understanding of C++ reactive programming, consider exploring the following resources: books specifically on RxCPP, online courses platform, extensive documentation, and community forums. Engaging with these resources will enrich your learning journey.
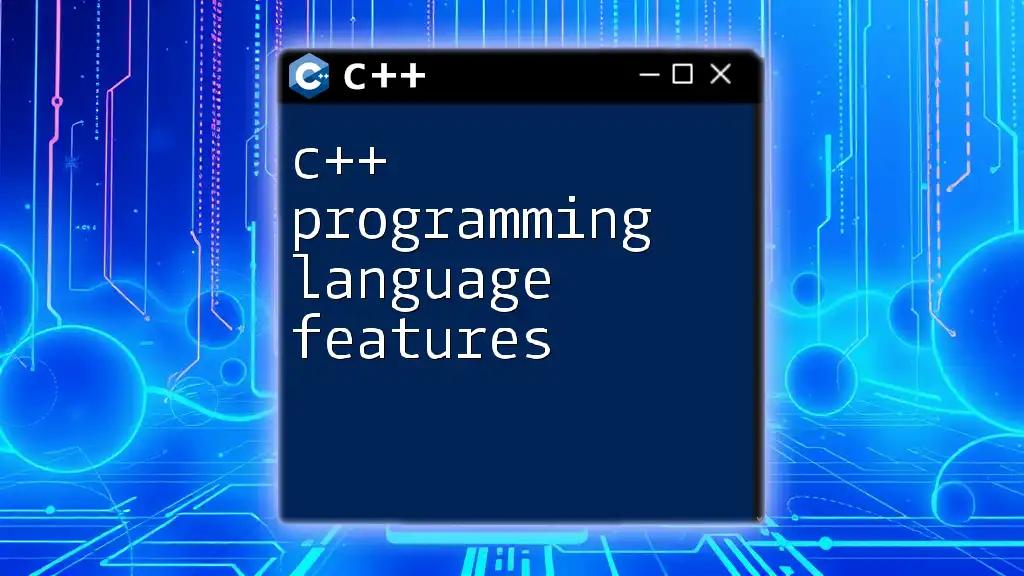
Call to Action
I encourage you to dive into C++ reactive programming by experimenting with the examples shared in this article. Experiment, build, and share your insights and projects in learning communities to foster a deeper understanding of this exciting paradigm!