C++ scientific programming involves using the C++ language to perform complex mathematical computations and simulations, enabling efficient data analysis and the development of algorithms for scientific research.
Here's a simple example of solving a quadratic equation using C++:
#include <iostream>
#include <cmath>
int main() {
double a, b, c, discriminant, root1, root2;
std::cout << "Enter coefficients a, b and c: ";
std::cin >> a >> b >> c;
discriminant = b*b - 4*a*c;
if (discriminant > 0) {
root1 = (-b + sqrt(discriminant)) / (2*a);
root2 = (-b - sqrt(discriminant)) / (2*a);
std::cout << "Roots are real and different: " << root1 << " and " << root2 << std::endl;
} else if (discriminant == 0) {
root1 = root2 = -b / (2*a);
std::cout << "Roots are real and the same: " << root1 << std::endl;
} else {
std::cout << "Roots are complex and different." << std::endl;
}
return 0;
}
Introduction to C++ Scientific Programming
What is Scientific Programming?
Scientific programming is a specialized branch of programming focused on the development of algorithms and software that facilitate solving complex scientific problems. It plays a crucial role in conducting simulations, processing data, and creating models in various fields, such as physics, biology, engineering, and economics. C++ stands out as a powerful tool for scientific computing due to its high performance and rich feature set, enabling scientists and engineers to perform calculations and simulations efficiently.
Why Choose C++ for Scientific Computing?
C++ is a compelling choice for scientific computing for several reasons:
- Performance: C++ offers high execution speed and low-level memory control, making it ideal for computationally intensive tasks.
- Object-Oriented Programming: C++ supports OOP principles, facilitating easier organization and management of complex code.
- Rich Standard Library: The C++ Standard Template Library (STL) provides a collection of useful algorithms and data structures that simplify programming tasks.
- Cross-Platform Compatibility: C++ applications can run on various platforms with minimal adjustments.
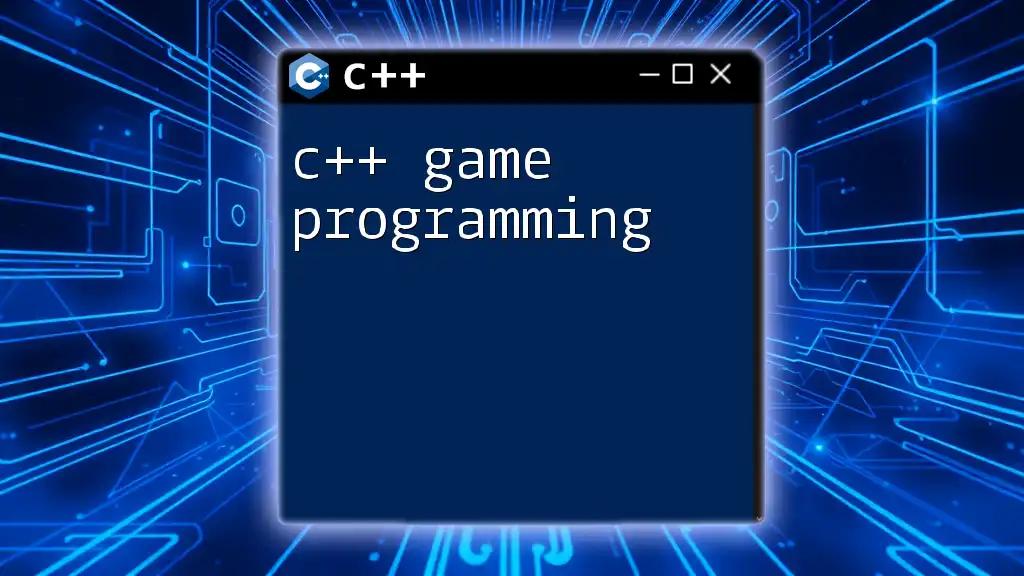
Setting Up Your C++ Environment for Scientific Computing
Selecting a Compiler
Choosing the right compiler is the first step in setting up your C++ environment. Popular options include:
- GCC (GNU Compiler Collection): Widely used, especially on Linux platforms.
- Clang: Known for its fast compilation and helpful error messages.
- MSVC (Microsoft Visual C++): The default development environment for Windows users.
Integrated Development Environments (IDEs)
An IDE helps manage and write code efficiently. Some popular IDEs for C++ development include:
- Visual Studio: Offers a comprehensive set of tools, great for Windows users.
- Code::Blocks: A versatile, open-source IDE suitable for various platforms.
- CLion: A powerful IDE from JetBrains with excellent CMake support.
Installing Necessary Libraries
For scientific computing, leveraging additional libraries can significantly enhance productivity. Some essential libraries to consider are:
- Eigen: A C++ template library for linear algebra.
- Armadillo: A high-quality linear algebra library that is easy to use.
- Boost: A collection of libraries for various tasks, including numerical computations and data structures.
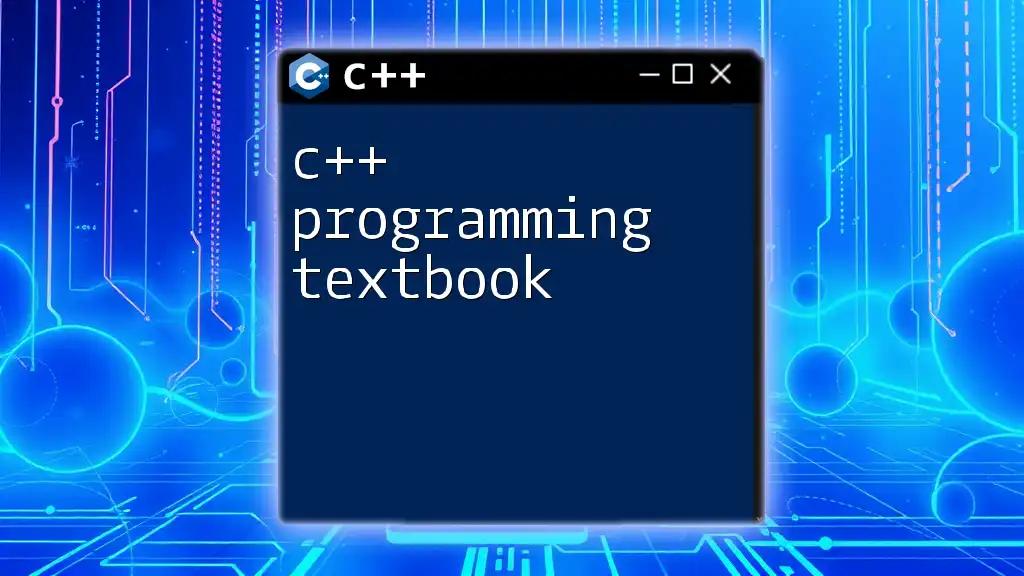
Fundamental Concepts in C++ for Scientific Computing
C++ Data Types and Structures
Understanding data types and structures is crucial for efficient programming. C++ supports various primitive types (e.g., `int`, `float`, `double`) and complex data structures using classes and structs.
For instance, we can define a simple class for a 3D point:
class Point3D {
public:
double x, y, z;
Point3D(double x, double y, double z) : x(x), y(y), z(z) {}
};
Here, we define a `Point3D` class with three coordinates. You can use this class to represent points in three-dimensional space, which is essential for many scientific computations.
Control Structures and Algorithms
C++ offers a variety of control structures, such as conditionals and loops, which are fundamental for implementing algorithms. Understanding these is key to writing efficient scientific computations.
As an example, consider Euler's method, a simple numerical technique used to solve ordinary differential equations:
double euler(double (*f)(double, double), double y0, double x0, double h, int n) {
double y = y0;
for (int i = 0; i < n; i++) {
y += h * f(x0 + i*h, y);
}
return y;
}
In this function, `f` represents the derivative function, and we iteratively calculate the value of `y` based on its previous value.
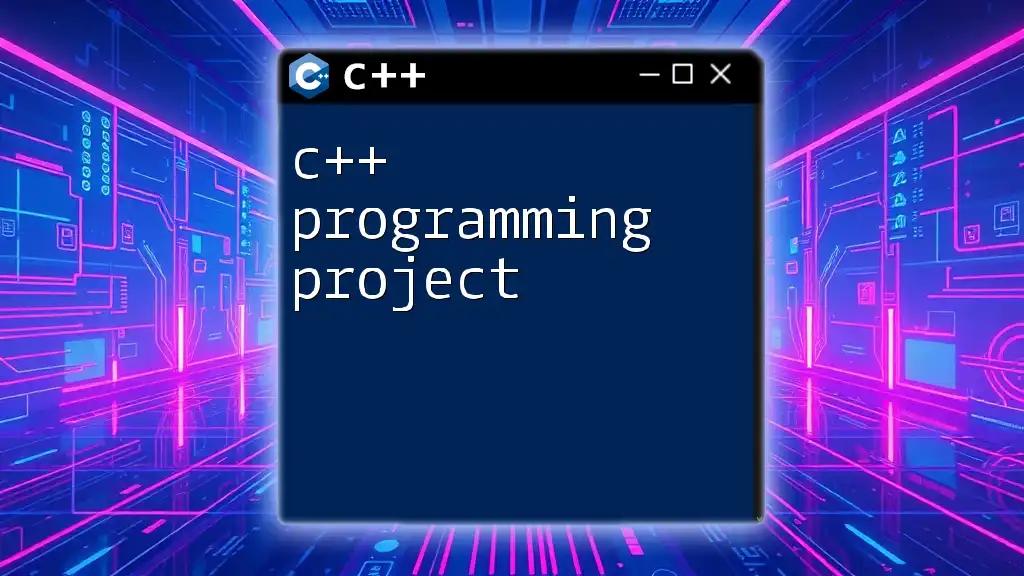
Employing C++ Libraries for Scientific Computing
Overview of Essential C++ Libraries
Utilizing libraries is a cornerstone of effective scientific programming. They provide pre-built functions and classes, saving time and ensuring reliability.
Eigen Library for Linear Algebra
Eigen is an excellent library for performing linear algebra operations, such as matrix multiplication and solving linear systems. With its intuitive syntax, you can quickly implement complex mathematical computations. Here’s an example of matrix multiplication using Eigen:
#include <Eigen/Dense>
using namespace Eigen;
MatrixXd A(2, 2);
A << 1, 2,
3, 4;
MatrixXd B(2, 2);
B << 5, 6,
7, 8;
MatrixXd C = A * B; // Perform matrix multiplication
In this snippet, we define two 2x2 matrices and perform matrix multiplication, illustrating how easy it is to handle mathematical operations with Eigen.
Armadillo for High-Performance Linear Algebra
Armadillo provides a straightforward interface similar to MATLAB. It is designed for linear algebra applications and offers robust performance, making it suitable for large datasets and complex calculations.
Boost Libraries for Extended Functionality
The Boost library collection encompasses numerous functionalities, from numeric libraries to smart pointers. Utilizing Boost can enhance your code's capability, making scientific programming in C++ more efficient.
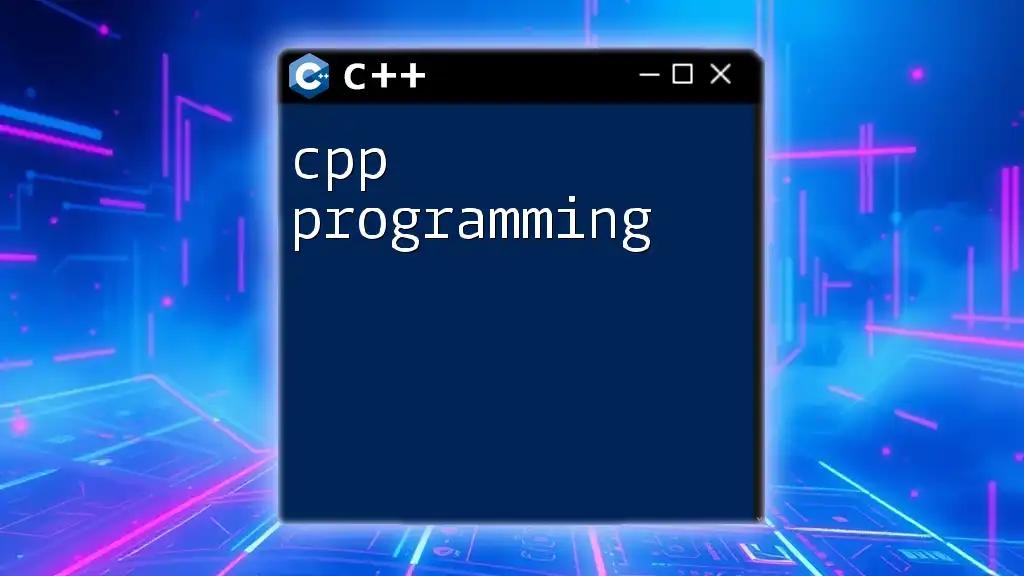
Practical Applications of Scientific Computing with C++
Simulations in Natural Sciences
C++ is often used for simulating physical systems, such as fluid dynamics or particle simulations. These simulations require complex mathematical models and high-performance computations, which C++ can deliver. By leveraging C++'s capabilities, scientists can model realities accurately and efficiently.
Data Analysis and Visualization
While C++ excels in computing, integration with data visualization tools is essential for analysis. Although C++ does not have built-in visualization libraries like Python's Matplotlib, you can use libraries such as OpenGL or even link C++ with Python to utilize its graphical capabilities.
For example, you could write data processing in C++ and then visualize it with Python:
// Data generation in C++
#include <iostream>
#include <vector>
// Assume data generation and storing in a vector of doubles
std::vector<double> simulate_data() {
// Simulate some data
return {1.0, 2.5, 3.3, 4.8};
}
Then, transfer that data to Python for visualization.
Solve Ordinary Differential Equations (ODEs)
Solving ODEs is a critical application in scientific computing. In addition to Euler's method, scientists often use more sophisticated techniques like Runge-Kutta methods. Here’s a snippet to demonstrate a simple implementation of Heun's method:
void heun(double (*f)(double, double), double y0, double x0, double h, int n) {
double y = y0;
double x = x0;
for (int i = 0; i < n; ++i) {
double y_predict = y + h * f(x, y);
y += h * 0.5 * (f(x, y) + f(x + h, y_predict));
x += h;
}
}
This code calculates the next value of `y` based on the derivative function `f`, providing a more accurate numerical approximation than simpler methods.
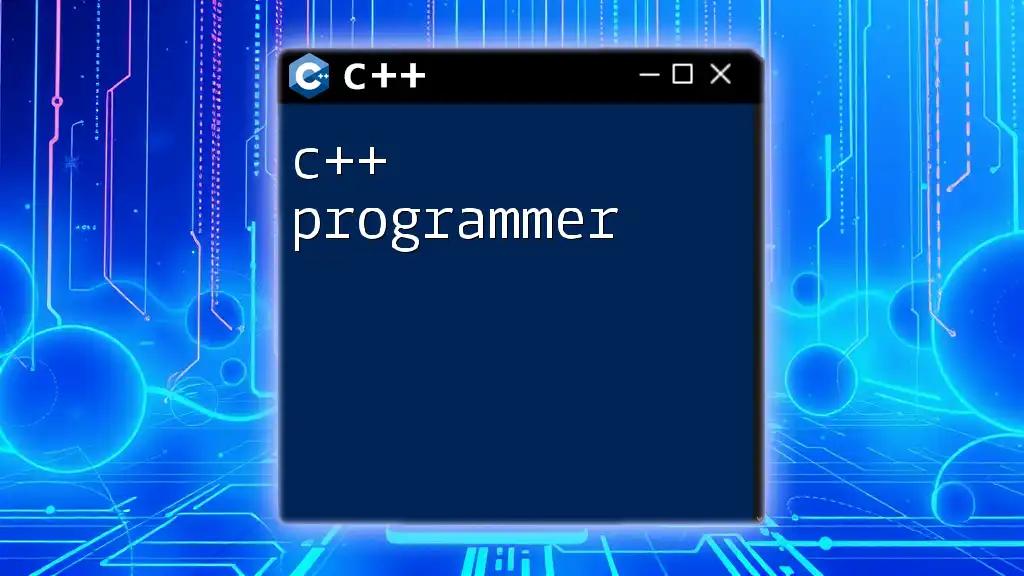
Best Practices for Scientific Programming in C++
Code Efficiency and Profiling
Optimizing code for efficiency is crucial in scientific computing, where calculations can be time-intensive. Profiling your code identifies bottlenecks, enabling targeted optimization strategies.
Memory Management
C++ provides powerful, low-level memory management capabilities. Understanding dynamic vs. static memory allocation can significantly affect your program's performance and resource utilization. Utilizing smart pointers from the STL can help manage memory automatically while avoiding common pitfalls, like memory leaks.
Testing and Validation
Rigorous testing is essential in scientific computing to ensure the accuracy of results. Adopting unit tests using frameworks like Google Test can help validate your functions against expected outcomes, maintaining trust in your computational models.
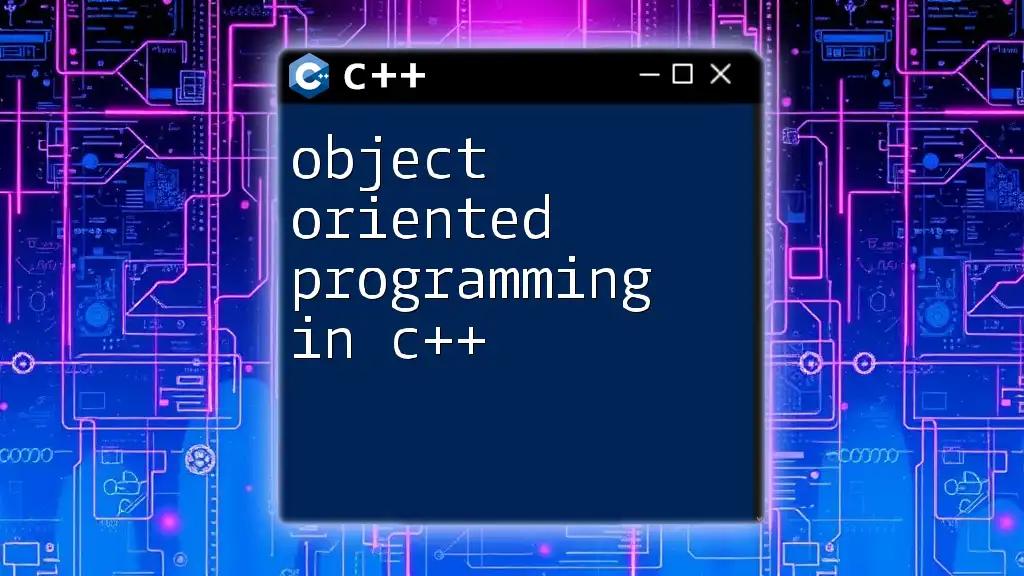
Conclusion
C++ scientific programming offers a robust framework for solving complex mathematical and scientific problems. Its performance, language features, and extensive libraries make it an ideal choice for researchers and engineers alike. By mastering the fundamental concepts and leveraging powerful libraries, you can unlock the potential of scientific computing with C++.
As you delve deeper into C++ for scientific applications, consider exploring various resources such as books, courses, and online communities to expand your knowledge and enhance your programming skills.