C++ practice programs are essential for honing programming skills by providing hands-on experience with various C++ concepts and commands.
Here's a simple example of a C++ program that demonstrates a basic "Hello, World!" output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Basics
Fundamental Concepts
When diving into C++ practice programs, it's essential to grasp the fundamental concepts of the language. Variables are key components, acting as containers for data. Familiarity with the most commonly used data types such as `int`, `float`, and `char` is crucial.
Control structures—including loops and conditional statements—are vital for controlling the flow of a program. Loops let you repeat actions, while conditional statements allow your code to make decisions based on certain conditions.
Essential Syntax
Understanding the syntax of C++ is the foundation upon which all programs are built. Pay attention to rules like:
- Every statement ends with a semicolon.
- Use of braces `{}` to denote blocks of code.
- Each variable must be declared with its associated type before use.
For instance:
int age = 25; // An example of variable declaration
This snippet sets the variable `age` with an integer value of 25, showcasing a standard practice in C++ syntax.
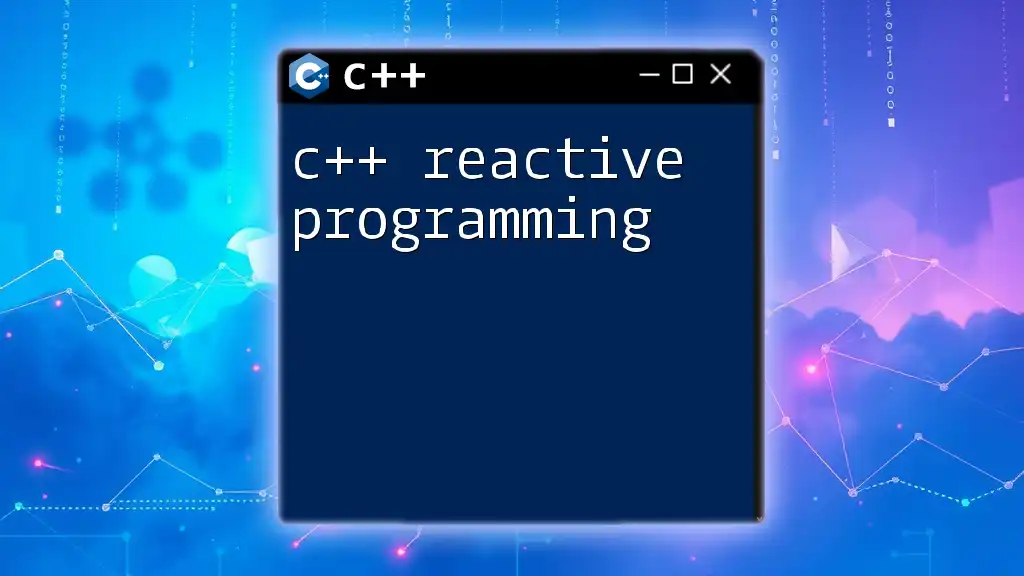
Why Practice is Important
Practicing C++ programming is not just about writing code; it's about sharpening your problem-solving skills, enhancing your understanding of algorithms, and debugging effectively. When you engage in coding practice, you solidify theoretical knowledge, making it easier to recall and apply when necessary. This repeating cycle of practicing, failing, and improving fosters a strong understanding of programming principles.
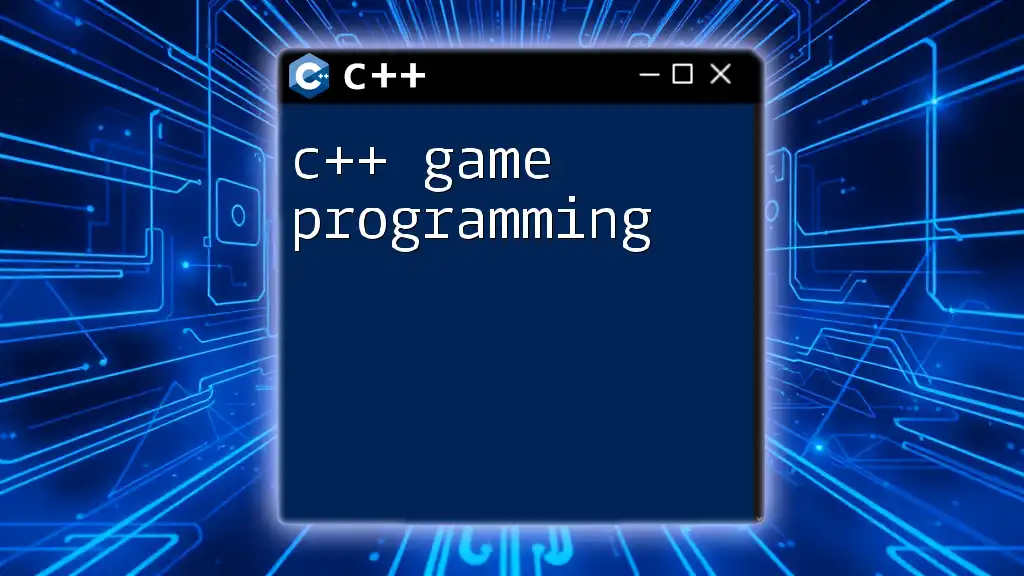
C++ Programs for Practice
Simple Programs
Hello World Program
Every programming journey begins with the classic Hello World program. It's an excellent introduction to the syntax and structure of a C++ program.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple program demonstrates the use of header files and the core function, `main()`, which serves as the starting point of execution. It outputs a greeting to the console, showcasing how to use the `cout` stream to display text.
Basic Calculator
Moving on to a slightly more complex example, a basic calculator can help you understand user input and perform operations:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
cout << "Enter operator (+, -, *, /): ";
cin >> operation;
switch (operation) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
cout << num1 / num2;
break;
default:
cout << "Invalid operator!";
}
return 0;
}
In this example, user input via `cin`, paired with the `switch` statement, allows users to perform basic arithmetic operations, illustrating the effectiveness of decision-making structures in programming.
Intermediate Programs
Guess the Number Game
Creating a guess the number game is an engaging way to practice loops and random number generation skills:
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
srand(static_cast<unsigned int>(time(0)));
int number = rand() % 100 + 1; // random number between 1 and 100
int guess;
do {
cout << "Enter your guess (1-100): ";
cin >> guess;
if (guess > number) {
cout << "Too high! Try again." << endl;
} else if (guess < number) {
cout << "Too low! Try again." << endl;
} else {
cout << "Congratulations! You guessed the number." << endl;
}
} while (guess != number);
return 0;
}
This program uses loops to allow multiple attempts at guessing the randomly generated number. Understanding this flow is essential as it encourages user interaction and reinforces control structures.
Simple Bank Management System
A simple bank management system can introduce you to object-oriented programming in C++. Here's a brief example:
#include <iostream>
#include <string>
using namespace std;
class BankAccount {
private:
string name;
double balance;
public:
BankAccount(string accName, double initialBalance) {
name = accName;
balance = initialBalance;
}
void deposit(double amount) {
balance += amount;
cout << "Deposited: " << amount << ", New Balance: " << balance << endl;
}
void withdraw(double amount) {
if (amount > balance) {
cout << "Insufficient balance!" << endl;
} else {
balance -= amount;
cout << "Withdrawn: " << amount << ", New Balance: " << balance << endl;
}
}
};
int main() {
BankAccount account("John Doe", 1000.0);
account.deposit(500.0);
account.withdraw(200.0);
account.withdraw(1500.0);
return 0;
}
This snippet demonstrates encapsulation with a class that manages a bank account's name and balance. You'll see how methods like `deposit` and `withdraw` manipulate the balance, showcasing the power of object-oriented principles.
Advanced Programs
Tic-Tac-Toe Game
As you advance, creating a Tic-Tac-Toe game can be a rewarding experience combining various skills:
#include <iostream>
using namespace std;
char board[3][3] = { {'1','2','3'},{'4','5','6'},{'7','8','9'} };
void drawBoard() {
cout << "Current Board: " << endl;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
bool checkWin() {
// Check rows, columns, and diagonals for a win condition
// Logic to be implemented
return false; // Placeholder
}
int main() {
// Game loop and logic to be implemented
return 0;
}
In this code, the structure is set up for the game, but logic for checking winning conditions and user input can be implemented. This project will engage your coding skills deeply, requiring both design thinking and technical knowledge.
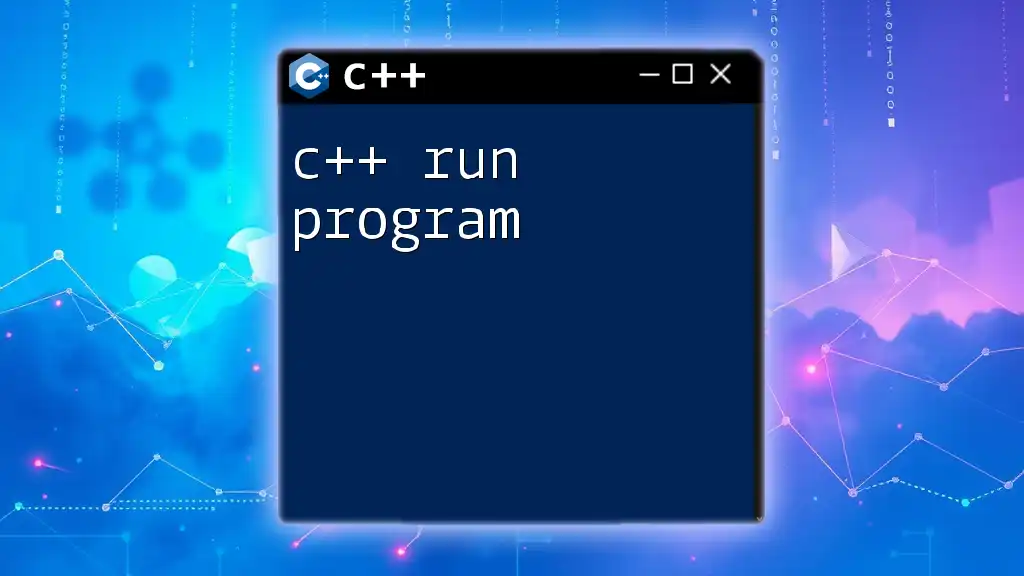
Additional Resources
To further enhance your learning, consider exploring coding platforms like LeetCode and HackerRank. These websites offer countless challenges that can help reinforce your C++ skills. Additionally, books focused on C++ programming and various online courses can provide structured learning pathways.
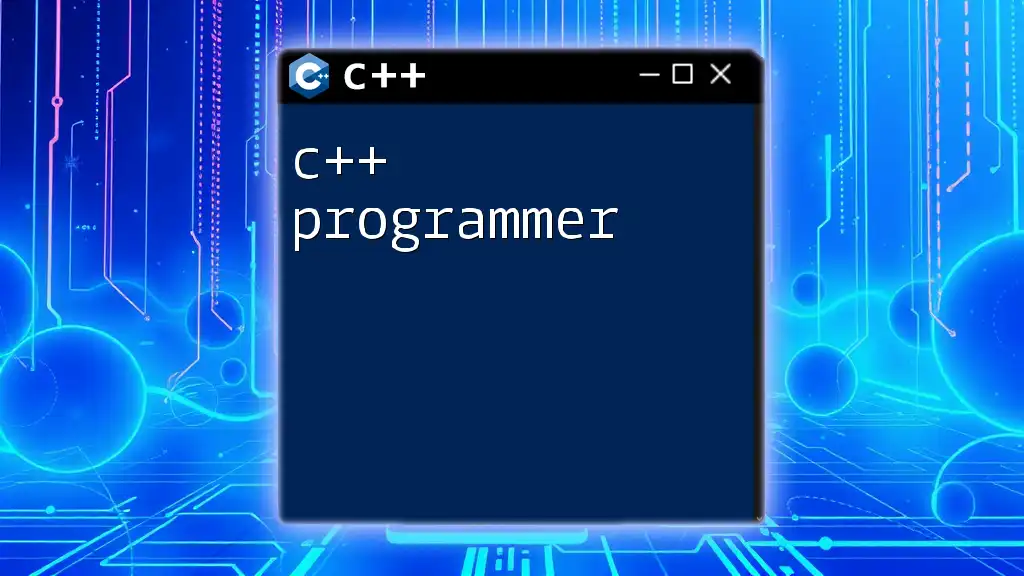
Conclusion
In summary, engaging in C++ practice programs is invaluable for anyone looking to improve their programming skills. These exercises help solidify your understanding and provide a platform for experimentation. By consistently practicing, you'll find yourself becoming proficient in C++ and ready to tackle more complex programming challenges.
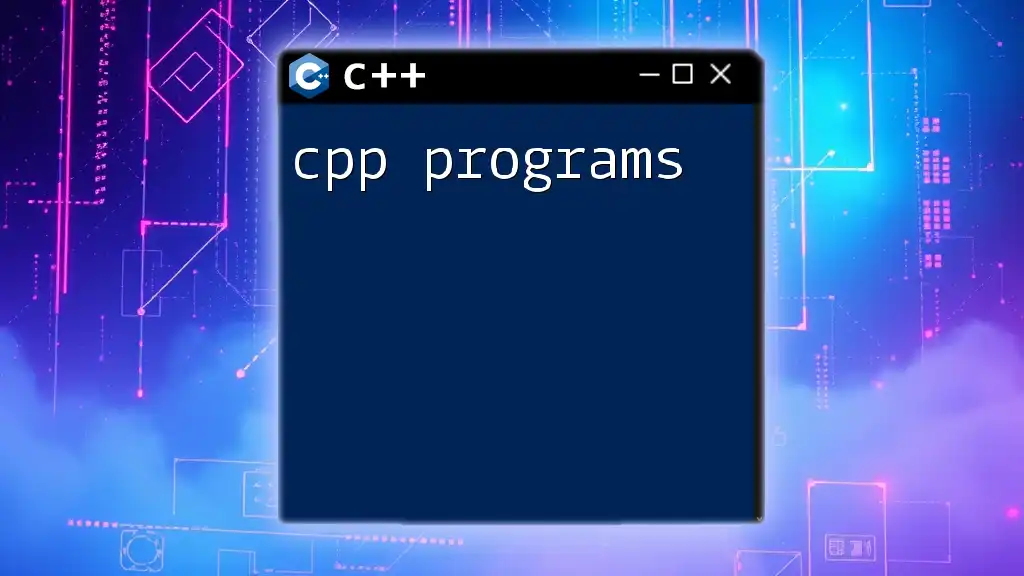
Call to Action
Don’t forget to share your experiences with C++ practice programs and seek out challenges that push your limits. Subscribe for more tips and coding exercises that will guide you on your journey to mastering C++!