Learn how to effectively utilize C++ commands through simple and concise program examples, demonstrated below with a basic "Hello, World!" program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What are C++ Programs?
C++ programs are collections of instructions written in the C++ programming language that tells a computer what to do. When executed, these instructions are compiled into machine code, which the computer can understand. Understanding how to write and execute C++ programs is crucial for anyone looking to become proficient in C++.
Compiling and running a C++ program involves using a compiler, which translates the source code into executable code. Common compilers include GCC and Visual C++. After writing the code in a file with a `.cpp` extension, you can compile it using a command in the terminal and run the resulting executable to see your program in action.
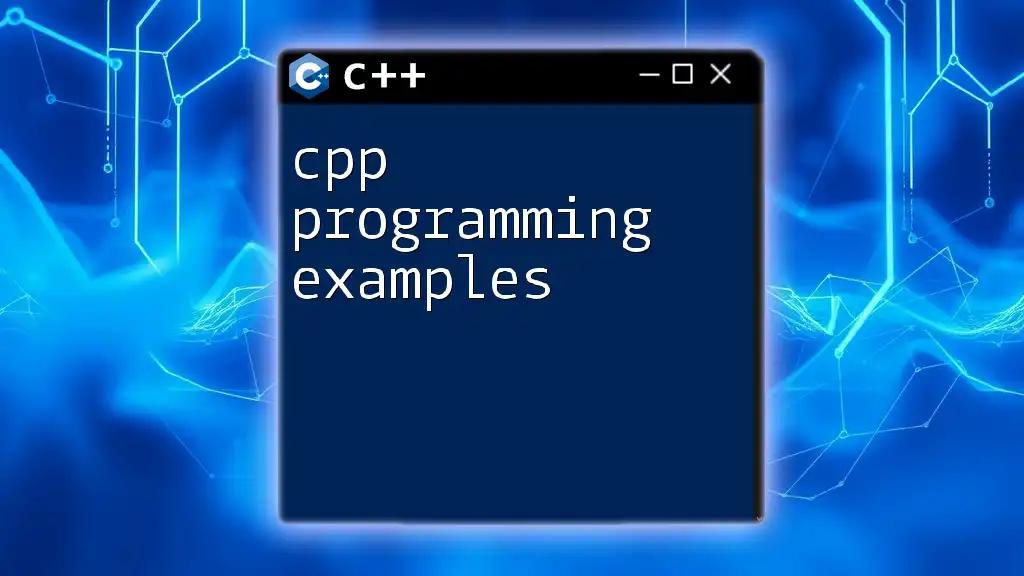
Basic Structure of a C++ Program
Every C++ program starts with a `main` function, which serves as the entry point of the program. The basic structure of a C++ program includes:
#include <iostream> // Including necessary header
using namespace std; // Using the standard namespace
int main() { // Main function
// Code goes here
return 0; // Return statement
}
Importance of Header Files
Header files, such as `<iostream>`, are essential in C++ as they contain declarations of functions and classes used in the program. For instance, `iostream` allows you to perform input and output operations.
Input and Output (I/O) in C++
C++ primarily uses `cin` for input and `cout` for output. By incorporating these functions, you can interact with the user.

Simple C++ Example Programs
Sample C++ Program: Hello World
The Hello World program is the simplest example that illustrates the basic syntax of C++.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Output Hello World
return 0; // End of program
}
In this code, `cout` is used to display text, while the `endl` manipulator is used to end the line. Running this program will prompt the output: Hello, World!.
C++ Program Example: Basic Arithmetic
To perform arithmetic operations, you can write a program that takes two numbers as input and displays their sum.
#include <iostream>
using namespace std;
int main() {
int a, b; // Declare variables
cout << "Enter two numbers: "; // Prompt user input
cin >> a >> b; // Read numbers
cout << "Sum: " << (a + b) << endl; // Output the sum
return 0; // End of program
}
This program takes input from the user and calculates the sum of two integers. It demonstrates both input and output operations effectively.
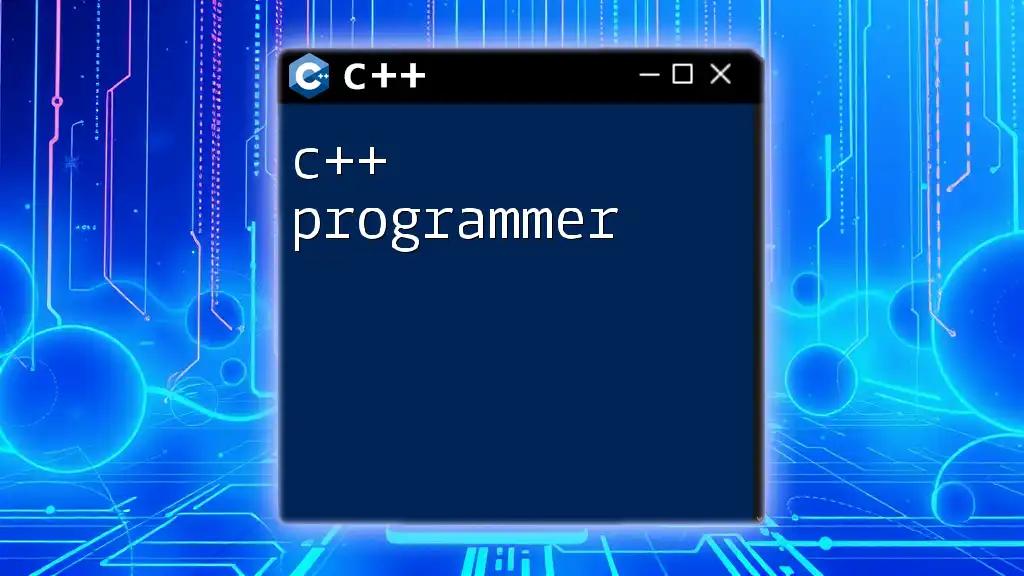
Intermediate C++ Example Programs
C++ Programming Examples: Loops
Loops are essential control structures that allow you to execute code repeatedly.
Example: For Loop
Using a for loop, you can perform a repetitive task, such as printing numbers.
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) { // Loop from 1 to 5
cout << "Number: " << i << endl; // Print the number
}
return 0; // End of program
}
In this example, the loop iterates from 1 through 5, printing each number consecutively. This illustrates the power of loops in handling repetitive tasks efficiently.
Example: While Loop
A while loop is another way to repeat actions based on a condition.
#include <iostream>
using namespace std;
int main() {
int n; // Declare variable
cout << "Enter a number (0 to exit): "; // Prompt user input
cin >> n; // Read the number
while (n != 0) { // Loop until 0 is entered
cout << "You entered: " << n << endl; // Output entered number
cout << "Enter another number (0 to exit): "; // Prompt user again
cin >> n; // Read the next number
}
return 0; // End of program
}
This program demonstrates how to continuously accept user inputs until a specified exit condition is met (in this case, entering 0).
Sample C++ Program: Functions
Functions help organize code into reusable blocks. Here’s a simple example demonstrating this.
#include <iostream>
using namespace std;
void greet() { // Function definition
cout << "Welcome to C++ Programming!" << endl; // Greeting message
}
int main() {
greet(); // Function call
return 0; // End of program
}
In this example, the `greet` function encapsulates the greeting message. This modular approach not only organizes code but also enhances its readability and maintainability.
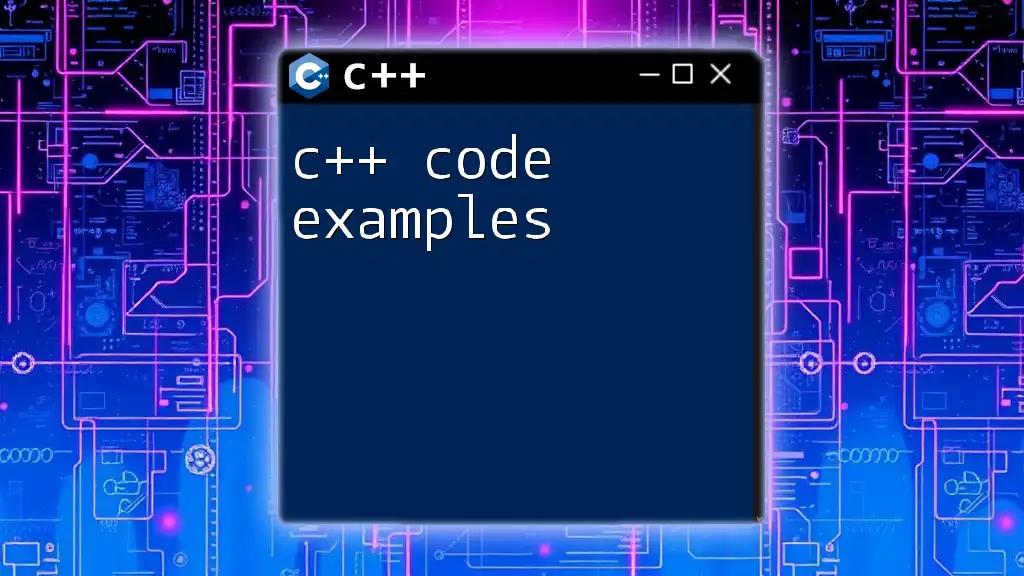
Advanced C++ Example Programs
C++ Example Programs: Object-Oriented Programming (OOP)
Object-Oriented Programming is a foundational paradigm in C++. Here’s how you can define and use a class.
Example: Creating a Class
Classes are blueprints for creating objects. In this example, we define a class named `Car`.
#include <iostream>
using namespace std;
class Car { // Class declaration
public:
void display() { // Method of class
cout << "This is a Car." << endl; // Output message
}
};
int main() {
Car myCar; // Create object of Car class
myCar.display(); // Call method
return 0; // End of program
}
This program creates a `Car` class with a public method `display`. The `main` function creates an object of `Car` and calls its method. This highlights the concept of encapsulation in OOP.
C++ Programming Examples: Data Structures
Data structures help to organize data systematically. Here's a simple example demonstrating the use of arrays.
Example: Arrays
Arrays are collections of similar types of elements. Here’s how you can use them in C++.
#include <iostream>
using namespace std;
int main() {
int myArray[] = {1, 2, 3, 4, 5}; // Initialize array
for (int i = 0; i < 5; i++) { // Iterate through array elements
cout << myArray[i] << " "; // Print each element
}
cout << endl; // End the line
return 0; // End of program
}
This program initializes an array of integers and iterates through it using a for loop, demonstrating how to access and display array elements.

Conclusion
Through these C++ programs examples, we have explored the foundational concepts of C++ programming including the basic structure, loops, functions, classes, and arrays. Each example serves to illustrate key concepts and provides a practical application to solidify understanding.
Building a solid foundation in these programming examples is crucial for tackling more advanced topics in C++. Embrace consistent practice with these concepts to enhance your programming skill set.
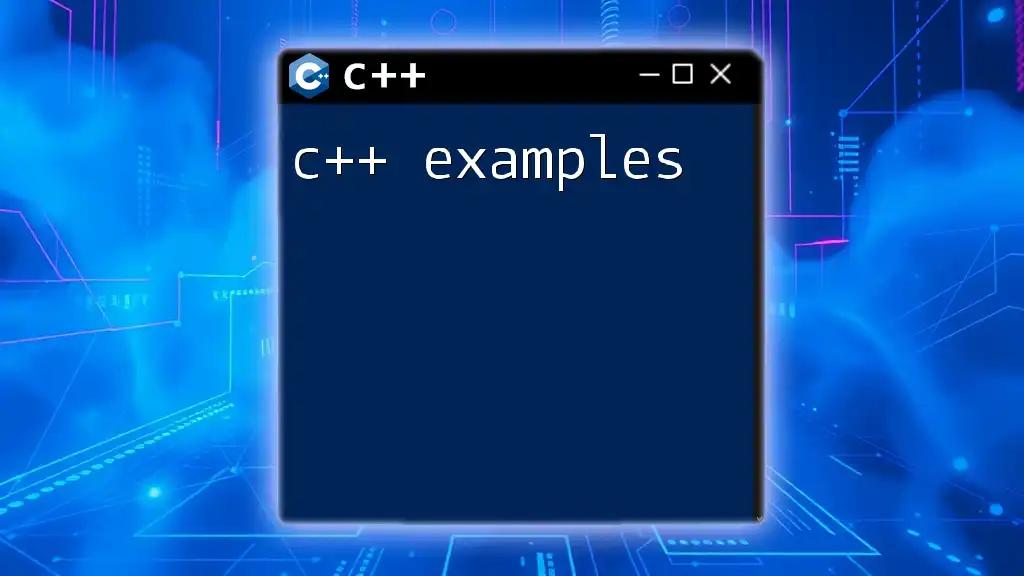
Additional Resources
To further your learning, consider exploring online compilers like Repl.it or GeeksforGeeks' C++ resources. Recommended textbooks and online courses can provide structured learning paths. Engaging in community forums or local coding groups can also enrich your learning experience by offering support and real-world application discussions.