In this post, we provide a simple example of a C++ program that prints "Hello, World!" to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful and versatile programming language that has undergone significant evolution since its inception in the early 1980s. Originally developed by Bjarne Stroustrup, it extends the C programming language, adding features like classes and object-oriented programming. C++ remains crucial in a wide range of domains, from systems programming to game development, making it one of the most widely used languages today.
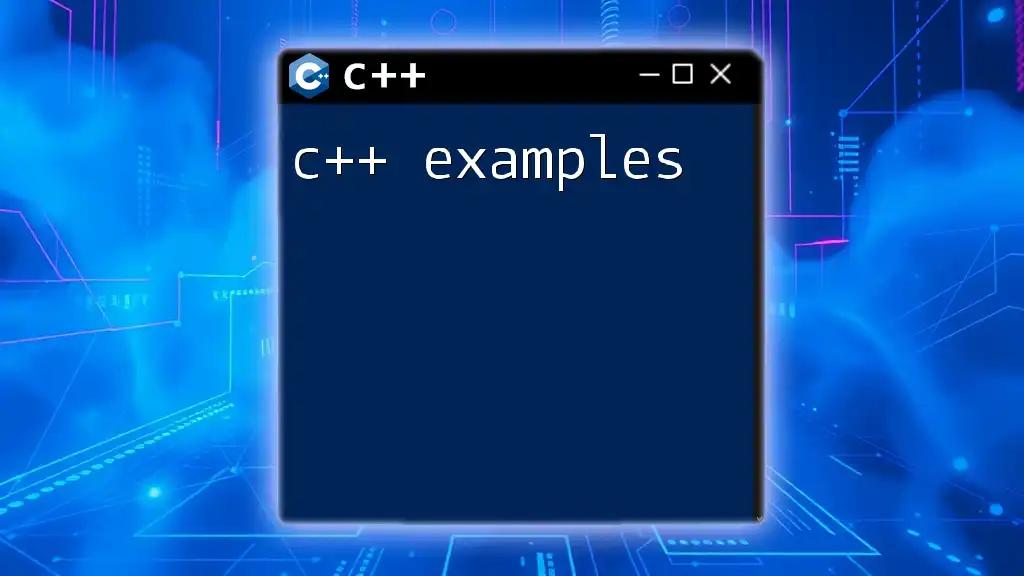
Why Learn C++?
Understanding C++ opens the door to a myriad of opportunities. Its performance efficiency makes it suitable for resource-constrained applications. Many large-scale systems, including operating systems and game engines, rely on C++ due to its speed and performance. Furthermore, learning C++ gives you valuable insight into how low-level operations work, enhancing your programming acumen.
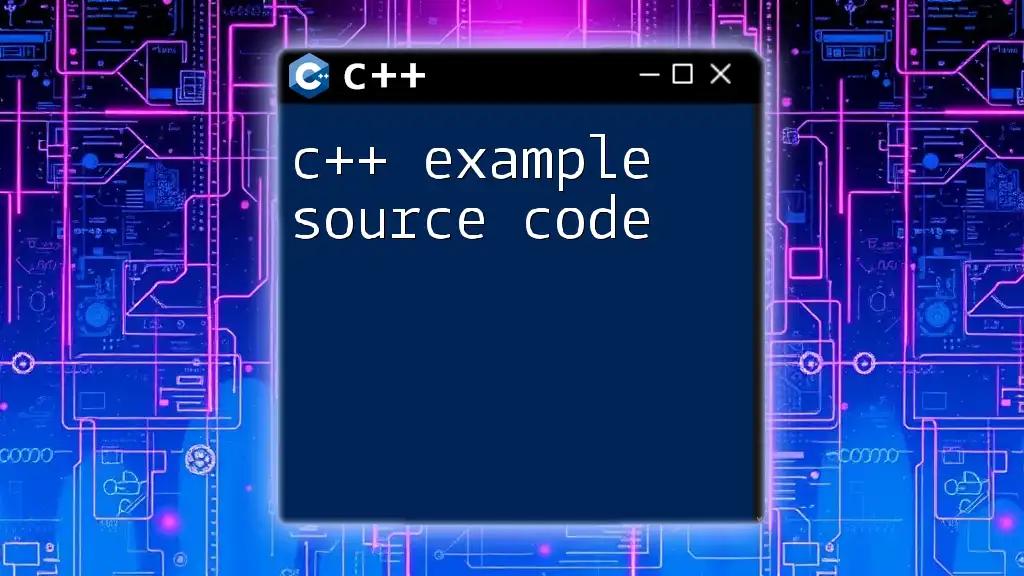
Basic Structure of a C++ Program
A typical C++ program follows a straightforward structure. At its core are preprocessor directives like `#include`, the `main()` function, and additional function definitions.
Here’s a simple example illustrating this structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` lets your program use the standard input/output stream library. The `main()` function is where the program starts its execution. Finally, `std::cout` prints the message to the console, followed by `std::endl` to insert a newline.
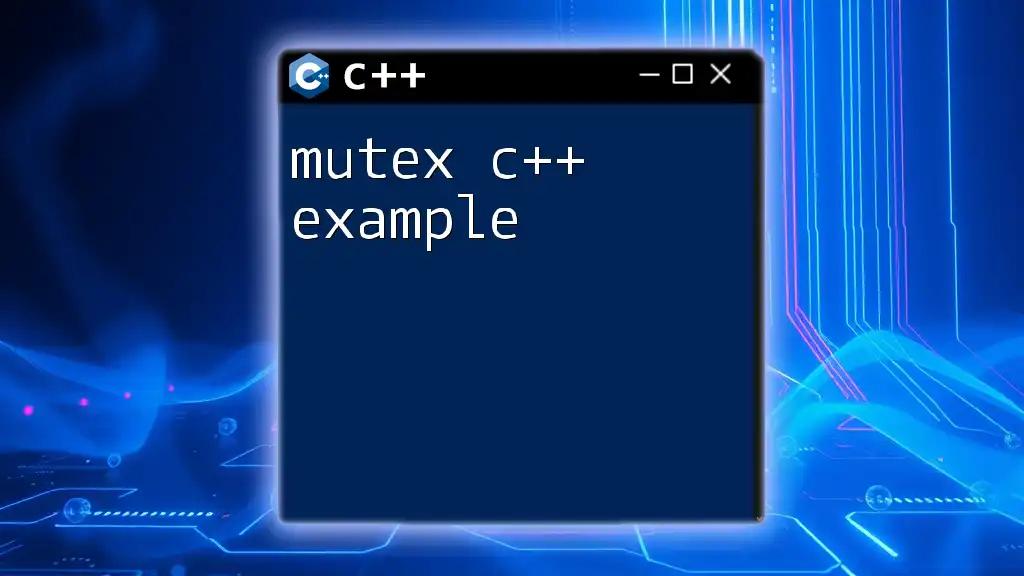
Data Types in C++
C++ provides several built-in data types which can be classified into different categories. These include:
- Primitive Data Types: `int`, `float`, `double`, `char`, and `bool`.
- User-Defined Data Types: These include classes, structures, unions, and enumerations.
Here's how you can declare variables of various data types:
int age = 30;
double height = 5.9;
char initial = 'A';
Choosing the correct data type is critical because it affects memory usage and performance.
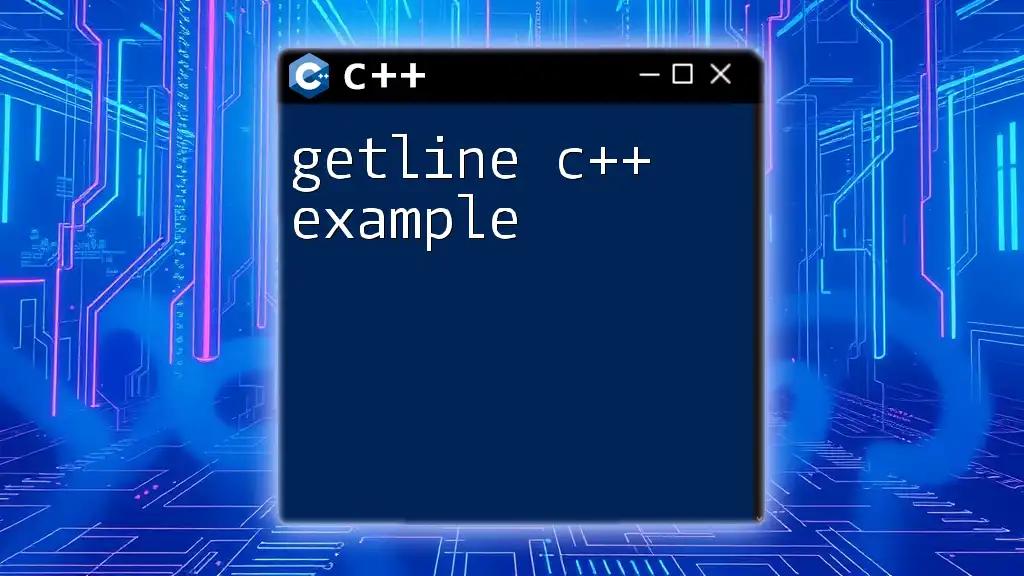
Control Structures
Conditional Statements
Control structures allow your program to make decisions based on conditions. While the `if` statement is standard, C++ also provides `else if` and `else`. Consider the example below:
int number = 10;
if (number > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Negative number or zero" << std::endl;
}
In this code snippet, the program checks if `number` is greater than zero. The relevant message is printed according to the condition met.
Loops in C++
Loops are essential for repetitive tasks. C++ supports several types of loops, including `for`, `while`, and `do-while`.
For Loop
The `for` loop is often used when you know how many times you want to iterate.
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
In this example, the program outputs the numbers 0 to 4.
While Loop
The `while` loop continues running as long as the specified condition is true.
int j = 0;
while (j < 5) {
std::cout << j << std::endl;
j++;
}
This snippet will print numbers 0 through 4, similar to the `for` loop above.
Do-While Loop
The `do-while` loop guarantees at least one iteration, as the condition is checked after the execution of the loop body.
int k = 0;
do {
std::cout << k << std::endl;
k++;
} while (k < 5);
In this case, the output will also show numbers from 0 to 4, maintaining its versatility.
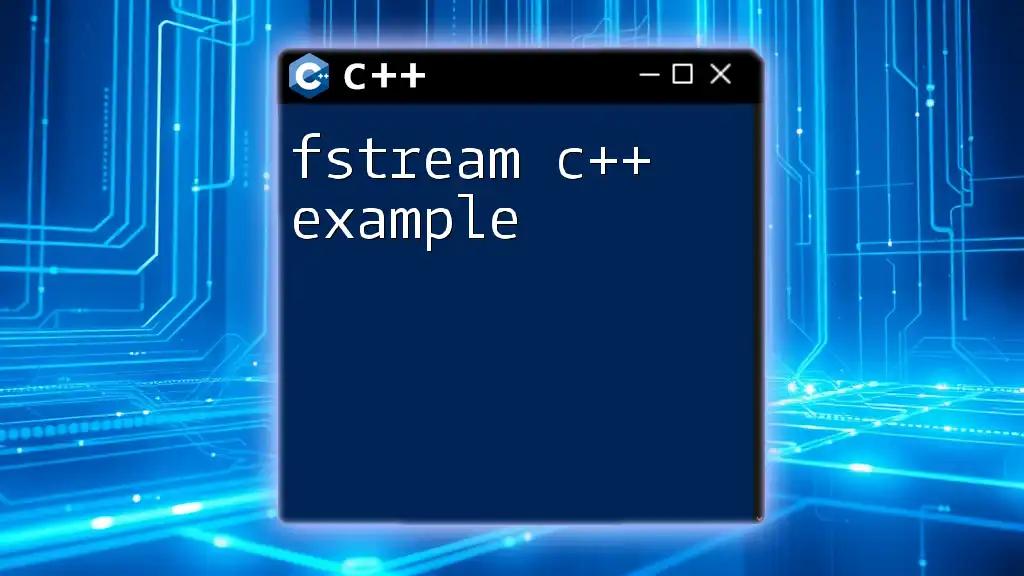
Functions in C++
Functions are blocks of code designed to perform a particular task. They increase the modularity and reusability of your code.
Defining and Calling Functions
To define a function, specify its return type, name, and possibly parameters. Below is an example:
void greet() {
std::cout << "Hello, User!" << std::endl;
}
int main() {
greet();
return 0;
}
In this example, the `greet` function is called within the `main()` function, which means "Hello, User!" will be printed to the console.
Function Parameters and Return Types
C++ allows you to pass parameters to functions and return values. Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
int main() {
int sum = add(5, 3);
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Here, `add` takes two integers as input and returns their sum.
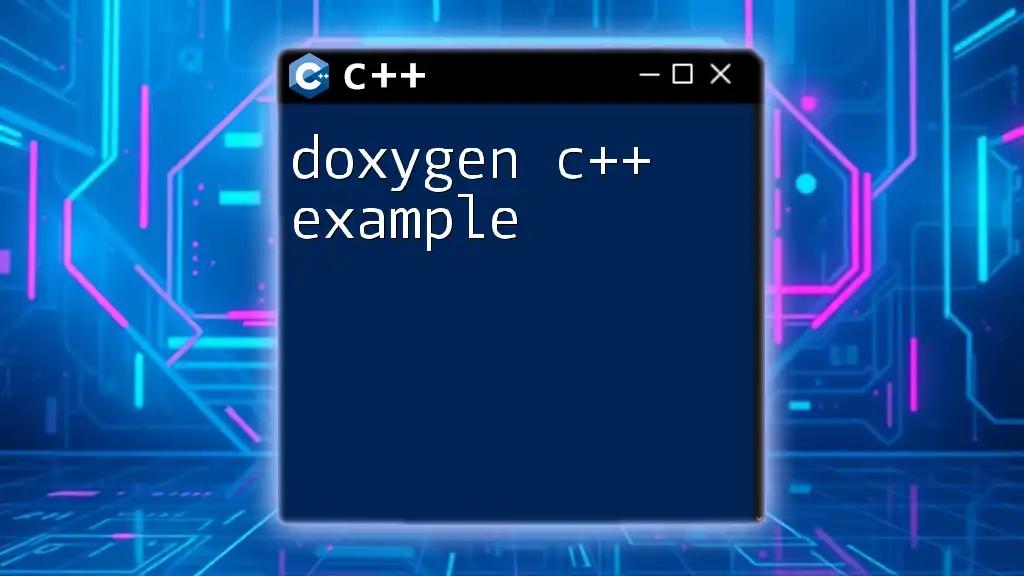
Object-Oriented Programming in C++
C++ supports object-oriented programming (OOP), which uses objects and classes to encapsulate data and behavior.
Understanding Classes and Objects
Classes define blueprints for creating objects. Objects represent instances of these classes.
Here’s a basic example showcasing a class and its method:
class Car {
public:
void drive() {
std::cout << "Car is driving" << std::endl;
}
};
int main() {
Car myCar;
myCar.drive();
return 0;
}
In this example, the `Car` class has a method called `drive`, which produces an output when invoked.
Inheritance and Polymorphism
Inheritance allows one class to inherit properties from another, promoting code reuse. Polymorphism lets you use a single interface to represent different data types. These concepts can be explored further as you deepen your understanding of C++.
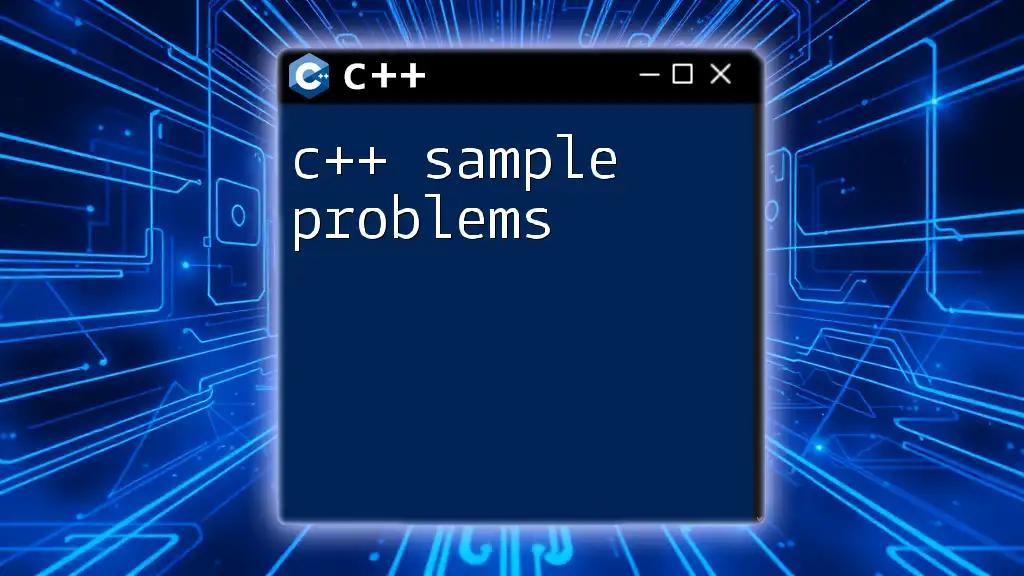
Standard Template Library (STL)
The Standard Template Library (STL) provides ready-to-use classes and functions for managing collections of data.
Introduction to STL
STL greatly enhances the efficiency of C++ programming. It includes various components such as algorithms, iterators, and containers.
Common Containers
Some of the most commonly used containers in STL are:
- Vector: A dynamic array that can grow in size.
- Map: A key-value store that allows for fast lookup.
- List: A doubly linked list providing efficient insertion and deletion.
Let’s see an example of using a `vector`:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
for (int num : numbers) {
std::cout << num << std::endl;
}
This code snippet initializes a vector and iterates through it, printing each number.
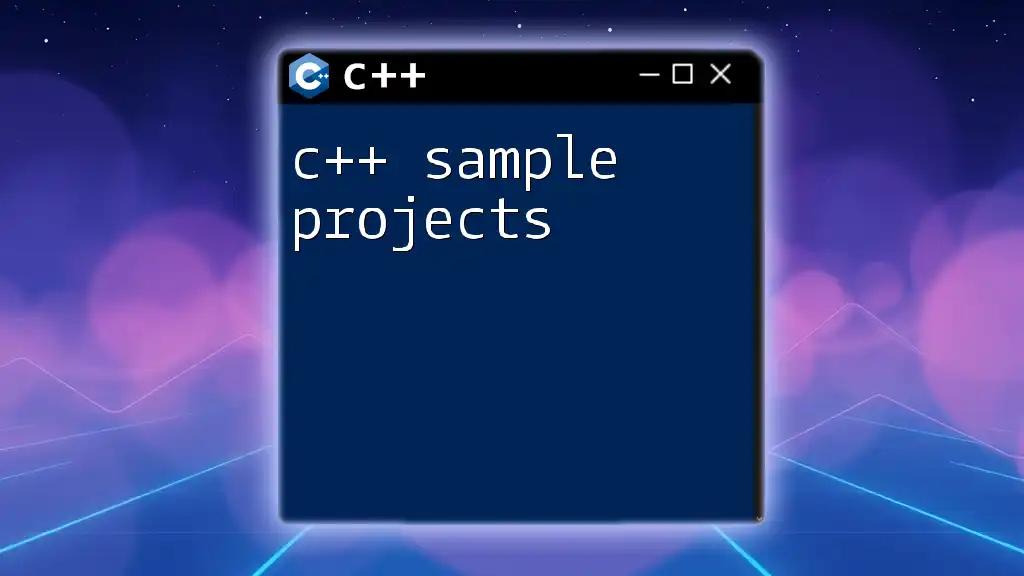
Error Handling in C++
Effective error handling is essential to build robust applications. C++ uses exceptions for error management.
Understanding Exceptions
Exceptions provide a way to react to exceptional circumstances, such as runtime errors. It is crucial to handle these scenarios without crashing the program.
Using Try-Catch Blocks
To manage exceptions, you can use try-catch blocks:
try {
int x = 5;
if (x < 10) throw "Value is less than 10!";
} catch (const char* msg) {
std::cerr << msg << std::endl;
}
In this snippet, if `x` is less than 10, an exception is thrown and caught, preventing potential program failure.
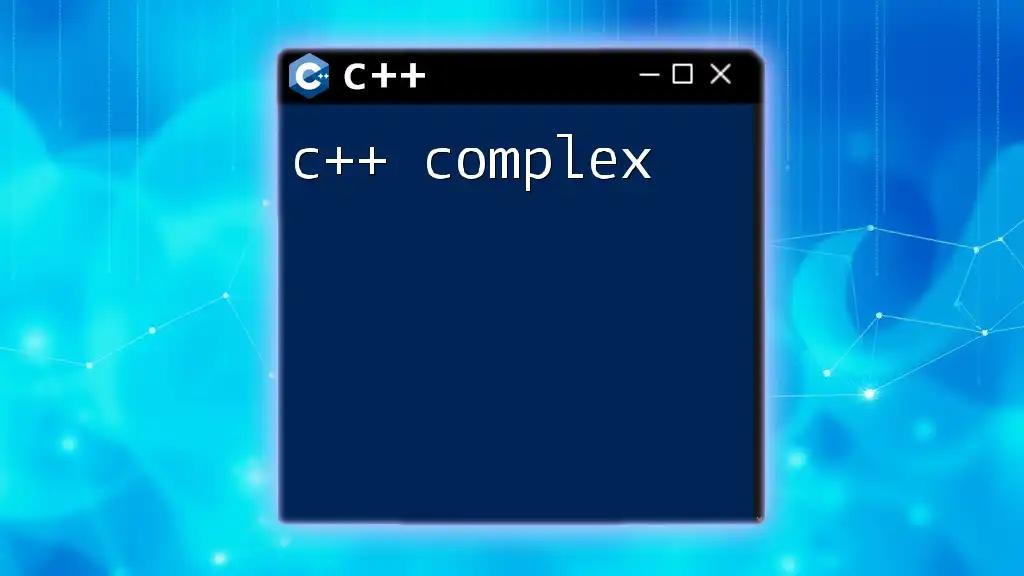
Best Practices for Writing C++ Code
Code Readability and Comments
Writing readable code is just as important as functional code. Use comments to elaborate on complex logic and ensure that your code can be easily followed by others.
Efficient Memory Management
Dynamic memory management is another vital aspect of C++. Pointers allow you to allocate and deallocate memory manually. Here's an example:
int* ptr = new int;
*ptr = 42;
delete ptr; // Remember to free allocated memory
This code allocates an integer dynamically and then frees the memory, ensuring there are no memory leaks.
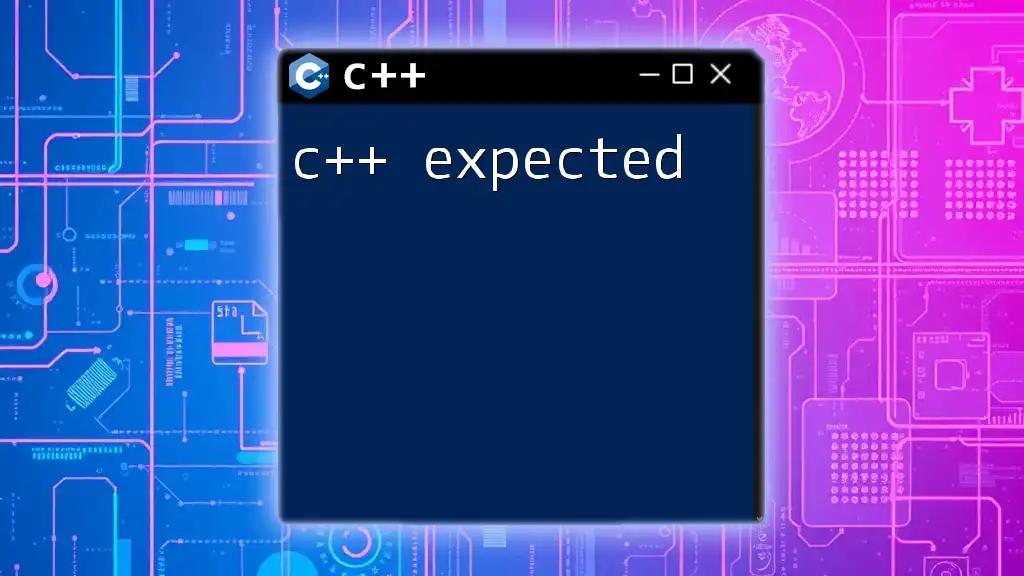
Conclusion
In this guide, we've explored various C++ examples that demonstrate essential programming concepts, including syntax, control structures, functions, object-oriented principles, and error handling. These foundational skills are pivotal for any aspiring C++ developer.
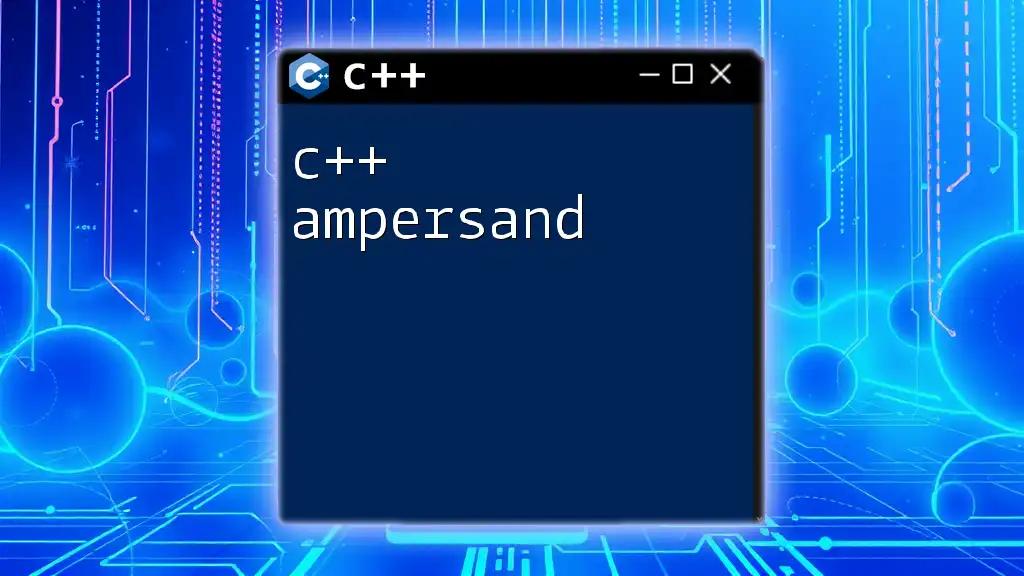
Call to Action
If you're eager to delve deeper into the world of C++, we invite you to join our learning journey! Explore our courses, subscribe to our newsletter for the latest updates, or participate in our workshops to enhance your skills and connect with fellow enthusiasts. Happy coding!