In C++, a vector is a dynamic array that can grow in size, and here's a simple example demonstrating how to create a vector, add elements to it, and access them:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Create a vector of integers
numbers.push_back(10); // Add elements
numbers.push_back(20);
numbers.push_back(30);
// Access and print elements
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a Vector?
A vector in C++ is a dynamic array that can automatically resize itself when elements are added or removed. Unlike traditional arrays, vectors do not have a fixed size; they can grow and shrink as needed. This flexibility makes them an integral component of modern C++ programming.
Features of C++ Vectors
-
Dynamic Sizing: Unlike standard arrays, which are static, vectors can expand or contract at runtime. This makes them particularly useful in scenarios where the number of elements is not known in advance.
-
Automatic Memory Management: The C++ Standard Library takes care of memory allocation and deallocation, helping to prevent memory leaks and other memory-related issues.
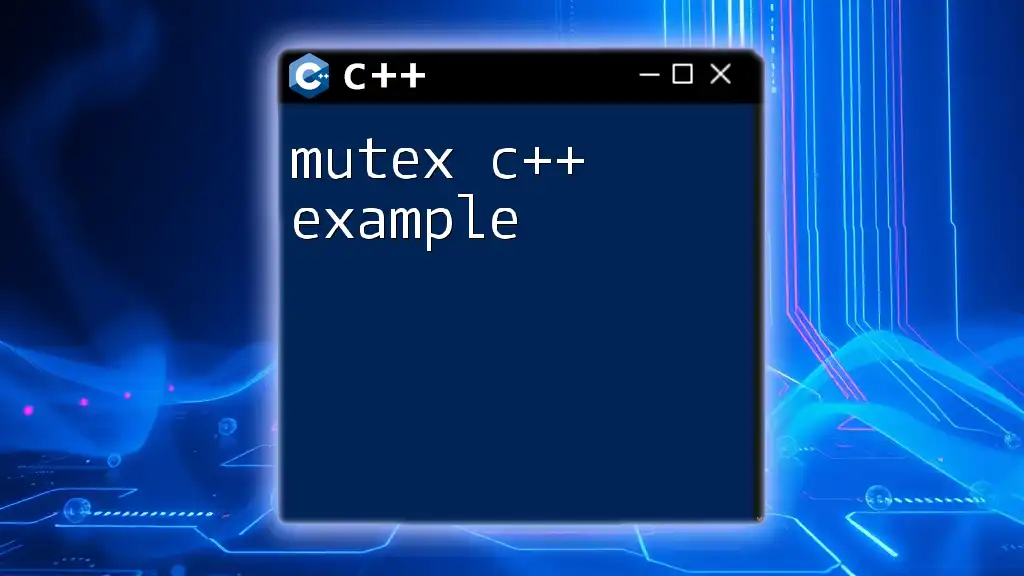
Understanding C++ STL Vector
What is STL?
The Standard Template Library (STL) is a powerful set of C++ template classes to provide general-purpose classes and functions with template-based design. STL provides ready-to-use data structures and algorithms, enhancing productivity and enabling developers to focus on problem-solving rather than building common structures from scratch.
Vectors in STL
Vectors are one of the most commonly used containers in the STL due to their ease of use and rich functionality. By utilizing vectors, developers can take advantage of built-in methods for insertion, deletion, and memory management, making them a preferred choice over raw arrays.
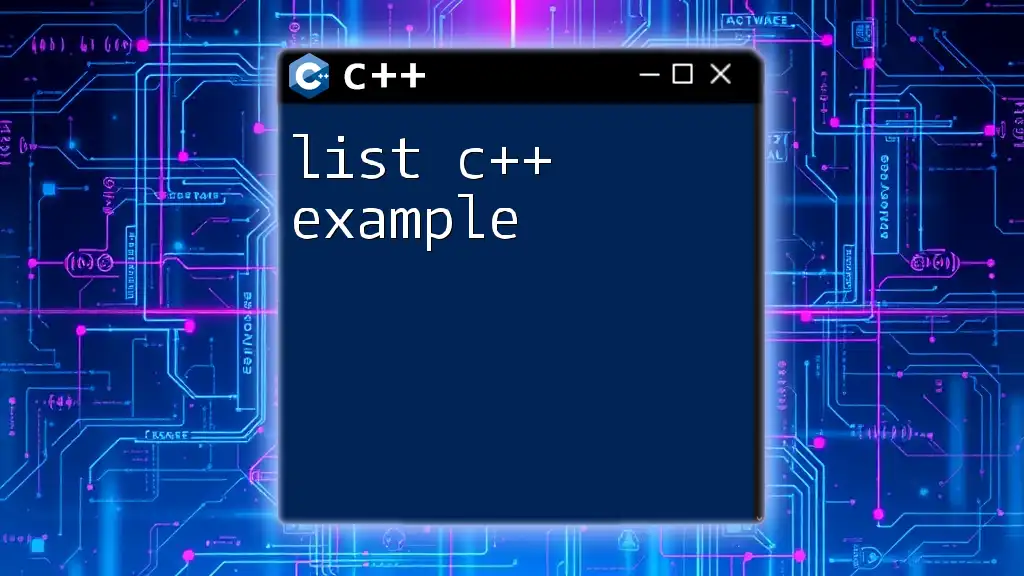
Basic Operations on Vectors
Declaring and Initializing Vectors
A vector is declared using the `std::vector` syntax. Here’s the basic format for declaration:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers; // Declare an empty vector
std::vector<int> initializedNumbers = {1, 2, 3, 4, 5}; // Initialize with elements
return 0;
}
Different ways to initialize vectors include using a list of elements, assigning size at the time of declaration, or using other vectors.
Accessing Vector Elements
You can access vector elements using either the `at()` method or the array subscript operator (`[]`). The `at()` method performs bounds checking and throws an exception if the index is out of range, while the subscript operator does not.
Example of element access:
std::cout << "First element: " << initializedNumbers[0] << std::endl; // Outputs: 1
std::cout << "Second element using at(): " << initializedNumbers.at(1) << std::endl; // Outputs: 2
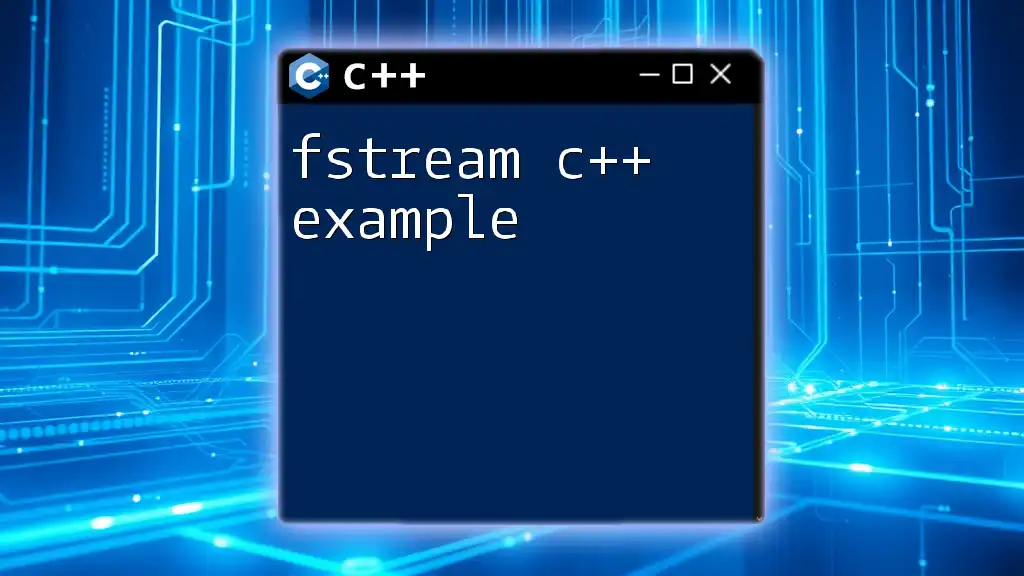
Modifying Vectors
Adding Elements
Vectors allow you to add elements using the `push_back()` or `emplace_back()` methods.
initializedNumbers.push_back(6); // Adds 6 to the end of the vector
Removing Elements
To remove elements, you can use `pop_back()` to remove the last element or `erase()` to remove specific elements.
initializedNumbers.pop_back(); // Removes the last element (6, in this case)
initializedNumbers.erase(initializedNumbers.begin() + 1); // Removes the second element (value 2)
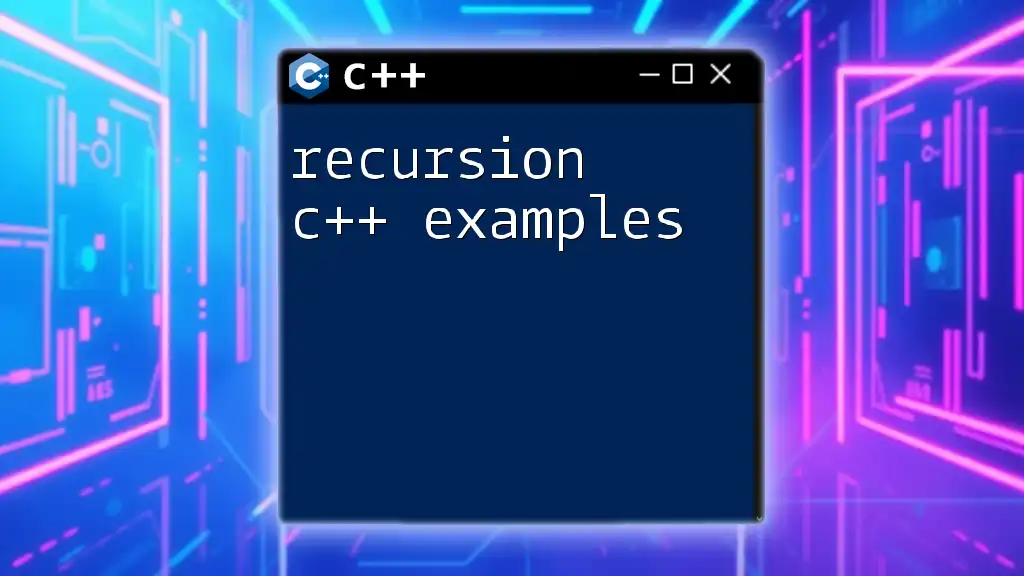
Vector Iteration
Using Iterators
C++ provides iterators that allow you to traverse vectors seamlessly. Iterators can point to any element in the vector.
for (auto it = initializedNumbers.begin(); it != initializedNumbers.end(); ++it) {
std::cout << *it << " "; // Outputs each element
}
Range-based For Loop
C++11 introduced range-based for loops, making it easier to iterate through a vector.
for (int number : initializedNumbers) {
std::cout << number << " "; // Outputs each element
}
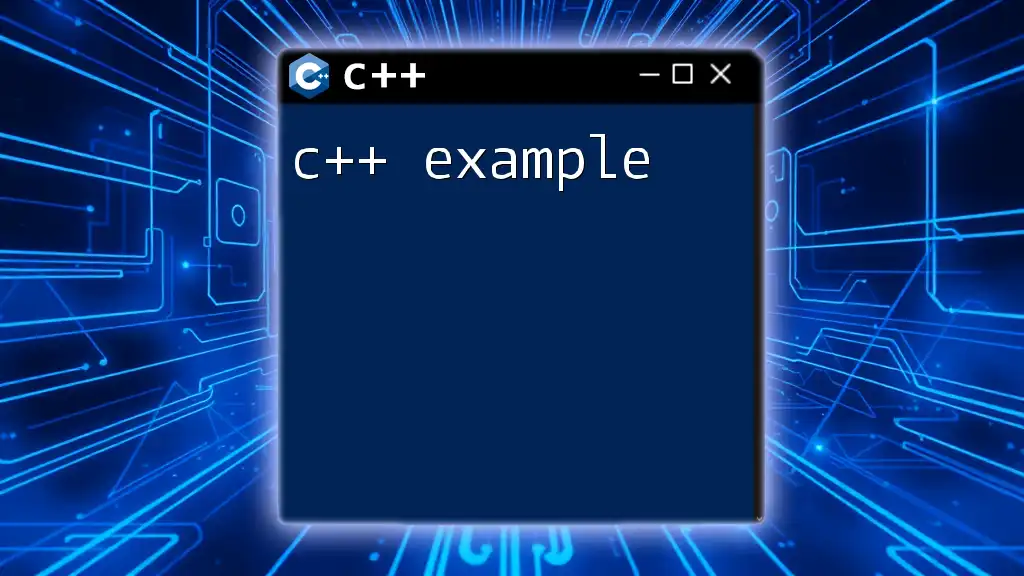
Advanced Vector Operations
Sorting and Searching
Vectors can be sorted using the `std::sort()` algorithm that makes it easy to order elements.
#include <algorithm>
std::sort(initializedNumbers.begin(), initializedNumbers.end()); // Sorts the vector
Searching for an element can be accomplished using `std::find()`.
#include <algorithm>
auto it = std::find(initializedNumbers.begin(), initializedNumbers.end(), 3);
if (it != initializedNumbers.end()) {
std::cout << "Element found: " << *it << std::endl; // Element found
} else {
std::cout << "Element not found" << std::endl;
}
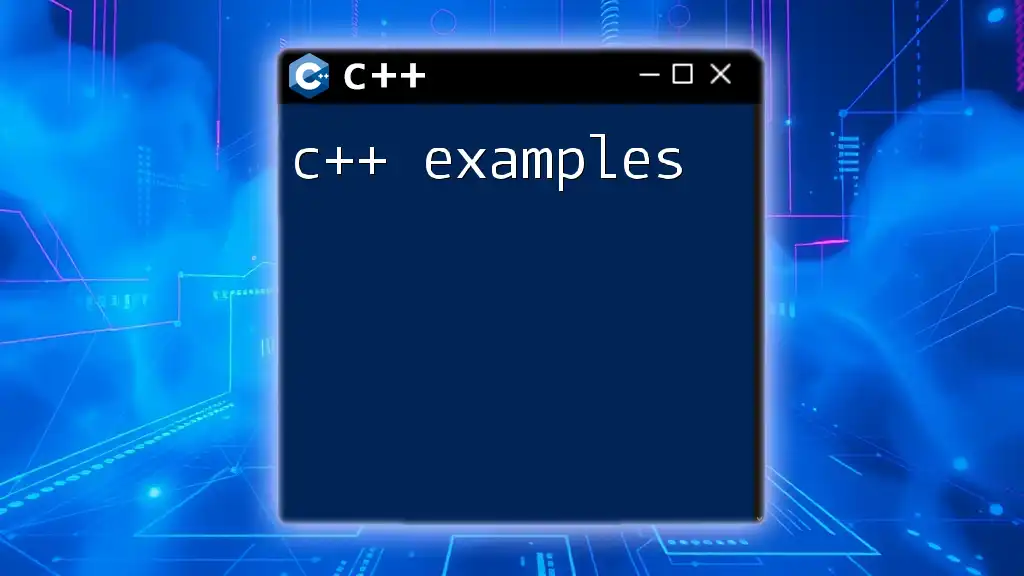
Best Practices When Using Vectors
Choosing the Right Initial Capacity
When declaring a vector, if you know an approximate number of elements beforehand, using `reserve()` to allocate space can improve performance by reducing the number of reallocations needed as elements are added.
Understanding Copy vs Move Semantics
With the introduction of C++11, move semantics minimize unnecessary copying of vectors, which can be beneficial for performance. Use `std::move()` when you are done with an object and want to transfer ownership efficiently.
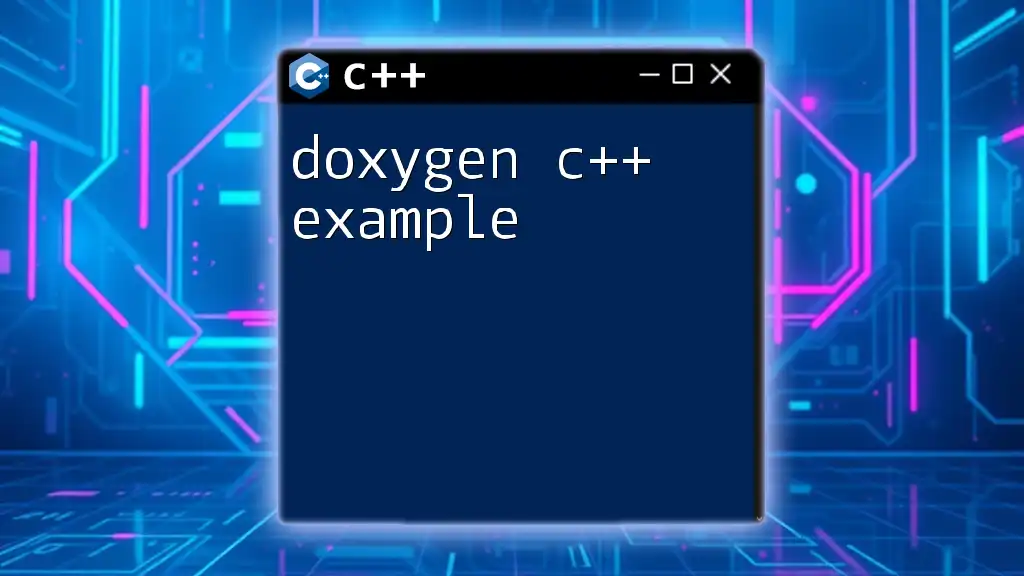
Common Mistakes to Avoid with Vectors
Out-of-Bounds Access
Accessing an out-of-bounds index is a common mistake that can lead to undefined behavior. Always use the `at()` method for added safety, as it checks bounds and throws an exception.
Inefficient Memory Usage
Vectors automatically reallocate memory when they grow beyond their capacity, which can be inefficient. Be mindful of how frequently you modify the vector size, and consider reserving an appropriate amount of space in advance.
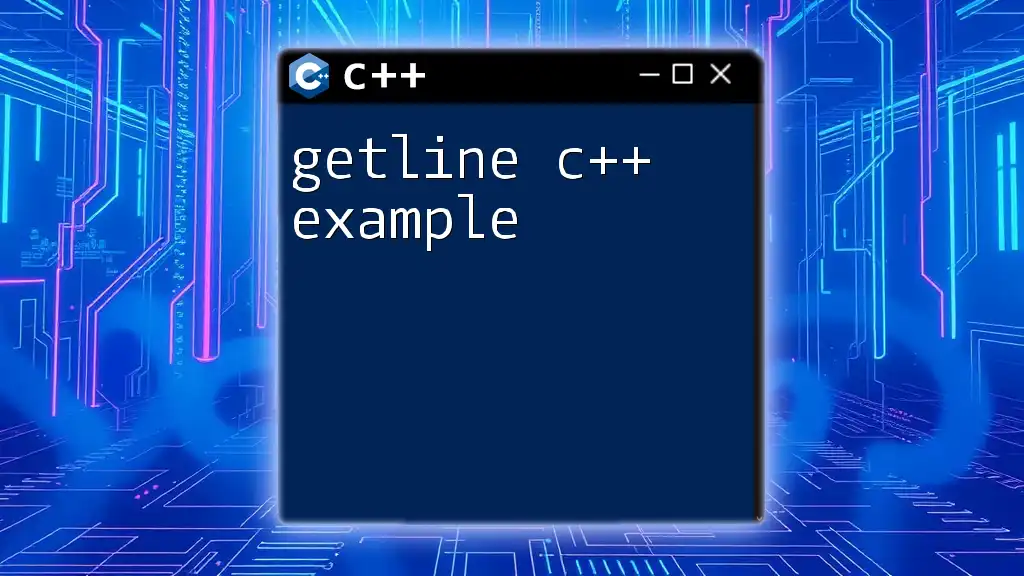
Conclusion
In conclusion, understanding the nuances of utilizing vectors in C++ can greatly enhance your programming efficiency. They are versatile, powerful, and easy to use for a package of features that streamline common operations. By mastering vectors, you can create highly dynamic and flexible applications that handle collections of data effectively.
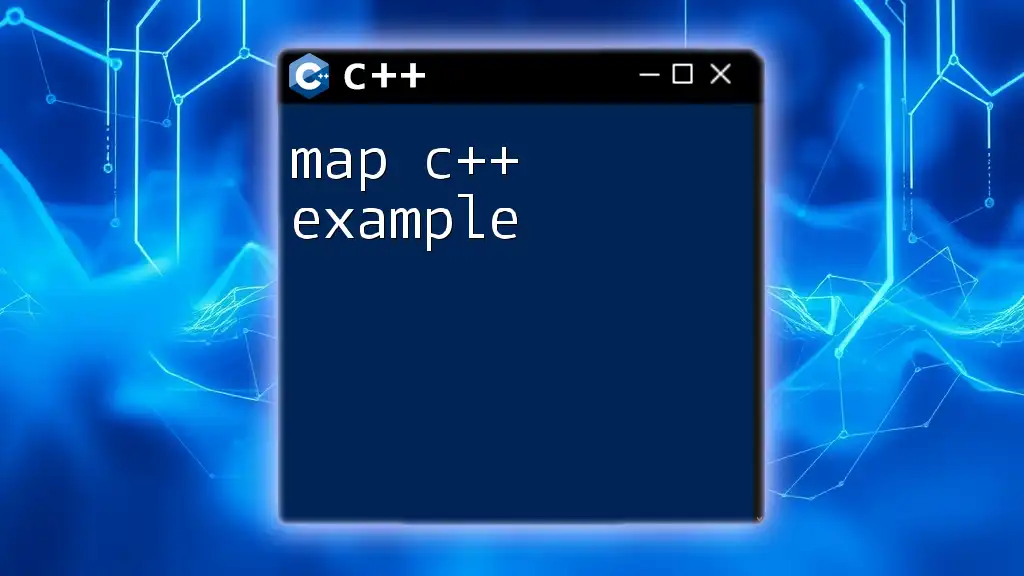
FAQs
What are the differences between arrays and vectors in C++?
While both arrays and vectors can hold collections of data, arrays have a fixed size and require manual memory management. In contrast, vectors are dynamic, automatically resize, and provide a member function interface for easier manipulation.
When should I use a vector instead of a list?
Use vectors when you need random access and frequent insertions/removals at the back. If you require efficient insertions and deletions at arbitrary positions, a list might be more suitable due to its node-based structure.