A constructor in C++ is a special member function that is automatically called when an object of a class is created, initializing the object's properties.
Here's a simple example:
#include <iostream>
using namespace std;
class Car {
public:
string brand;
int year;
// Constructor
Car(string b, int y) {
brand = b;
year = y;
}
void display() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
int main() {
Car myCar("Toyota", 2020);
myCar.display(); // Output: Brand: Toyota, Year: 2020
return 0;
}
What is a Constructor?
A constructor is a special class member function that is automatically called when an object of the class is created. Its primary role is to initialize objects and allocate memory as necessary. Constructors play an essential part in the object-oriented programming paradigm of C++, as they ensure that the objects start their life in a correctly defined state.
Types of Constructors
There are three main types of constructors in C++:
- Default Constructor: This constructor takes no arguments. If no constructor is defined in a class, C++ automatically provides a default constructor.
- Parameterized Constructor: This constructor takes arguments, allowing for the initialization of an object with specific values.
- Copy Constructor: This constructor initializes an object using another object of the same class, facilitating deep copies necessary for dynamic memory allocation.
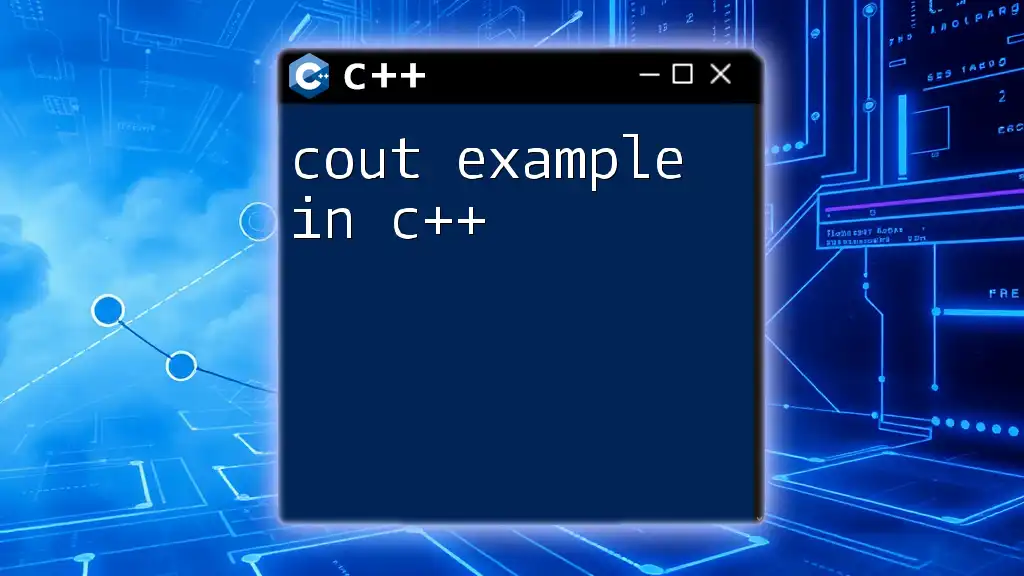
Understanding Default Constructors
What is a Default Constructor?
A default constructor is a constructor that does not take any parameters. It can initialize member variables with default values. When a new object is created without passing any parameters to the constructor, this constructor is invoked automatically.
Example of Default Constructor in C++
Here’s a concise example demonstrating a default constructor:
class Box {
public:
Box() {
// Default constructor
length = 0;
width = 0;
}
int length;
int width;
};
In this code snippet, the `Box` class has a default constructor that initializes both `length` and `width` to `0`. When an object of `Box` is created without parameters, it automatically takes these default values, ensuring that it always starts with a known state.
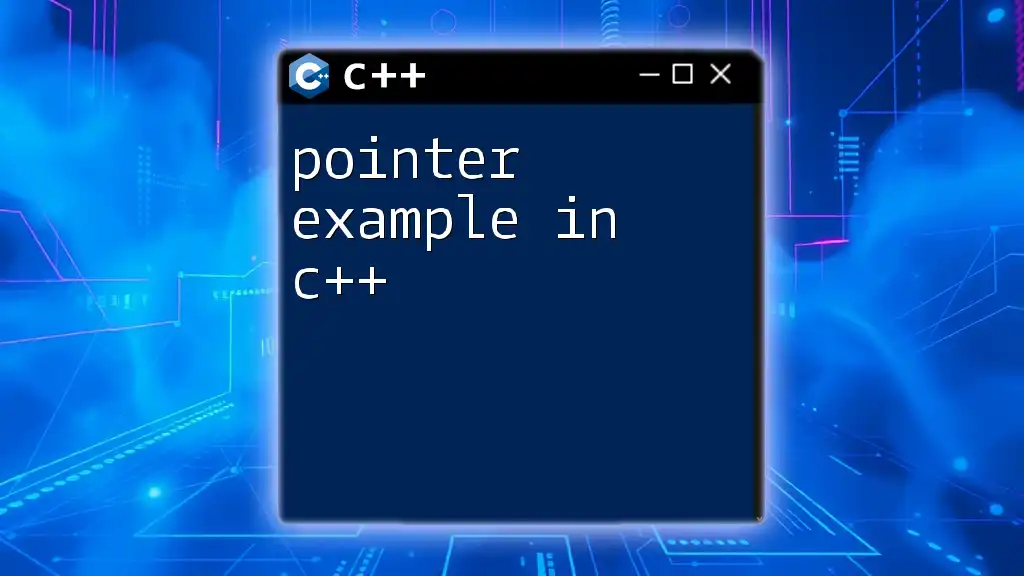
Parameterized Constructors
Definition and Purpose
A parameterized constructor allows you to create objects with specific initial values. By taking arguments, it provides flexibility in initializing an object upon creation, enabling the programmer to set values based on user input or other conditions.
C++ Constructor Example
Consider the following code that demonstrates a parameterized constructor:
class Box {
public:
Box(int l, int w) {
length = l;
width = w;
}
int length;
int width;
};
In this example, the `Box` class includes a parameterized constructor that accepts two parameters: `l` and `w`. When an object is instantiated using this constructor, you can specify unique dimensions for that object.
Usage Example
To use the parameterized constructor, we can create an instance of `Box` like this:
Box box1(10, 5); // Creating a box with length 10 and width 5
Here, `box1` is created with a length of `10` and a width of `5`, showcasing the flexibility and power of parameterized constructors in object initialization.
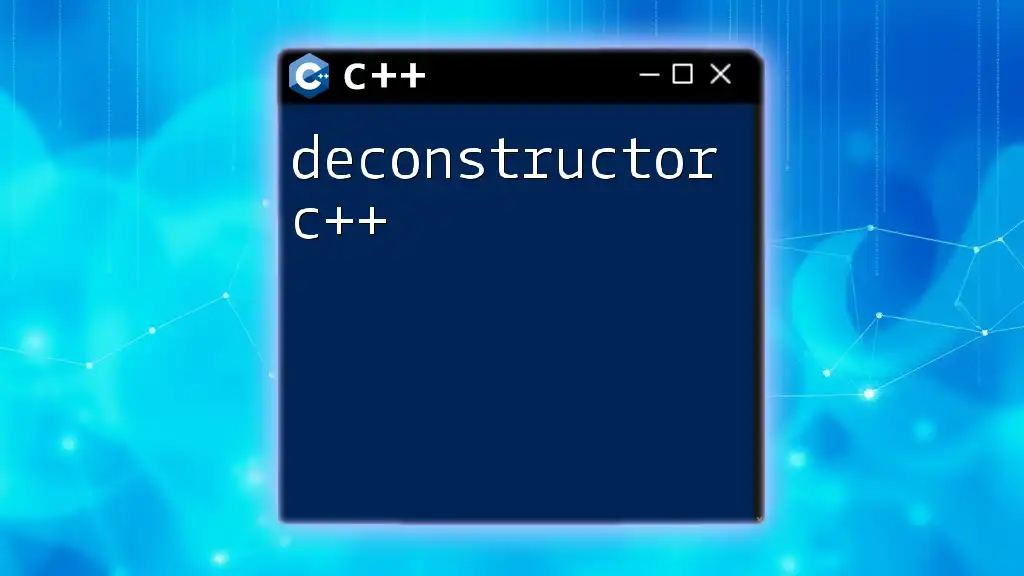
Copy Constructors
What is a Copy Constructor?
A copy constructor is a special type of constructor that initializes an object using another object of the same class. This constructor is crucial for managing dynamic memory. If there are pointers in a class, a default copy constructor would merely copy the pointer address rather than the actual data, leading to issues such as double deletion when both objects go out of scope.
C++ Copy Constructor Example
Here’s an example of a copy constructor:
class Box {
public:
Box(int l, int w) {
length = l;
width = w;
}
// Copy constructor
Box(const Box &b) {
length = b.length;
width = b.width;
}
int length;
int width;
};
In this code snippet, the `Box` class not only includes a parameterized constructor but also defines a copy constructor that takes a reference to another `Box` object. This ensures that when an object is copied, both `length` and `width` are replicated properly, facilitating deep copies.
Use Case of Copy Constructor
You can create a new object using the copy constructor as follows:
Box box2 = box1; // Copying box1 to box2 using the copy constructor
Here, `box2` is created as a copy of `box1`. Both objects will have identical lengths and widths, demonstrating that the copy constructor has successfully copied the data.
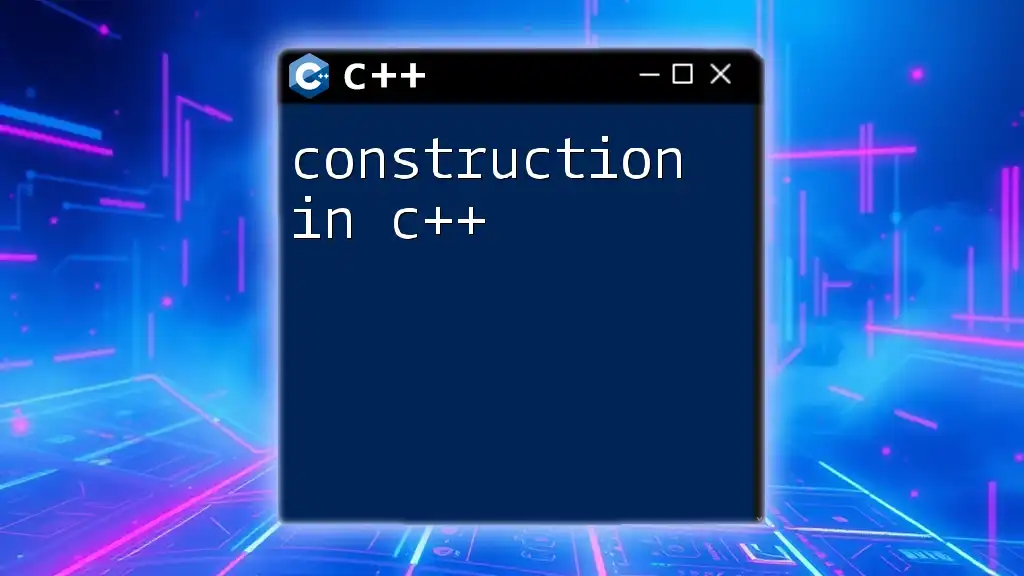
Best Practices for Using Constructors
When defining constructors, it is important to adhere to certain best practices:
-
Initialize All Member Variables: Always initialize all member variables in the constructor to avoid undefined behavior.
-
Use Member Initializer Lists: When performance is crucial, consider using member initializer lists instead of assignment in the constructor body.
Box(int l, int w) : length(l), width(w) {}
This approach enhances performance by initializing variables directly rather than assigning them values post-construction.
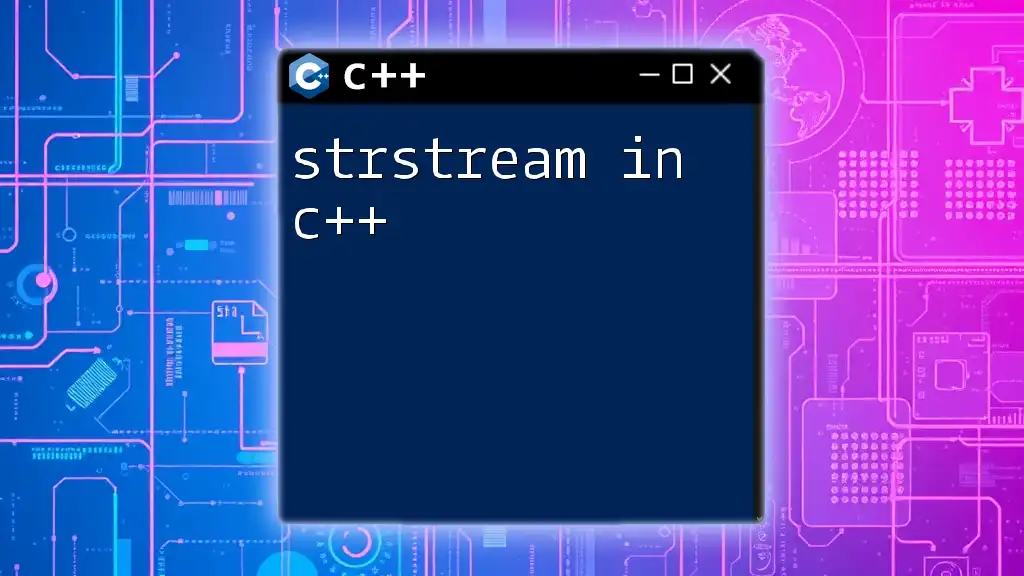
Common Pitfalls with Constructors
While constructors are critical for effective C++ programming, developers can encounter pitfalls:
- Forgetting to Define a Constructor: If no constructor is defined, the compiler provides a default constructor. Be cautious with this implicit behavior as it might not be what you expect.
- Issues with Implicit Constructors: If both a default and parameterized constructor exist, care must be taken to avoid ambiguous calls when creating objects.
Solutions to Avoid These Mistakes
- Always define at least one constructor in your classes.
- Use `explicit` keyword for parameterized constructors if you want to avoid unintended implicit conversions.
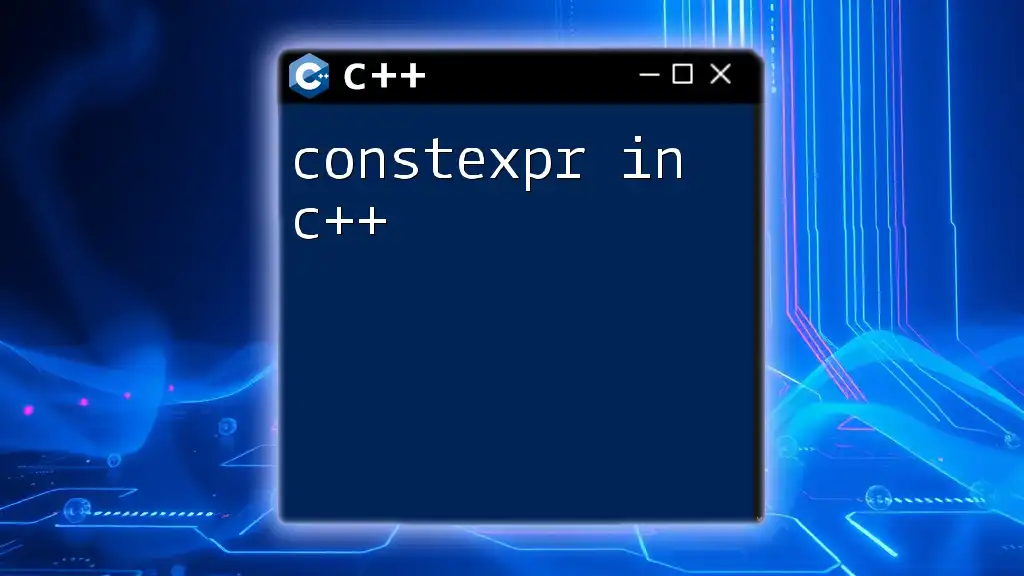
Conclusion
Constructors are foundational to object-oriented programming in C++. A well-defined constructor ensures that objects are initialized in a predictable state and that dynamic memory is managed effectively. As you continue to explore C++, practicing with constructors through various examples will deepen your understanding and enhance your programming skills.
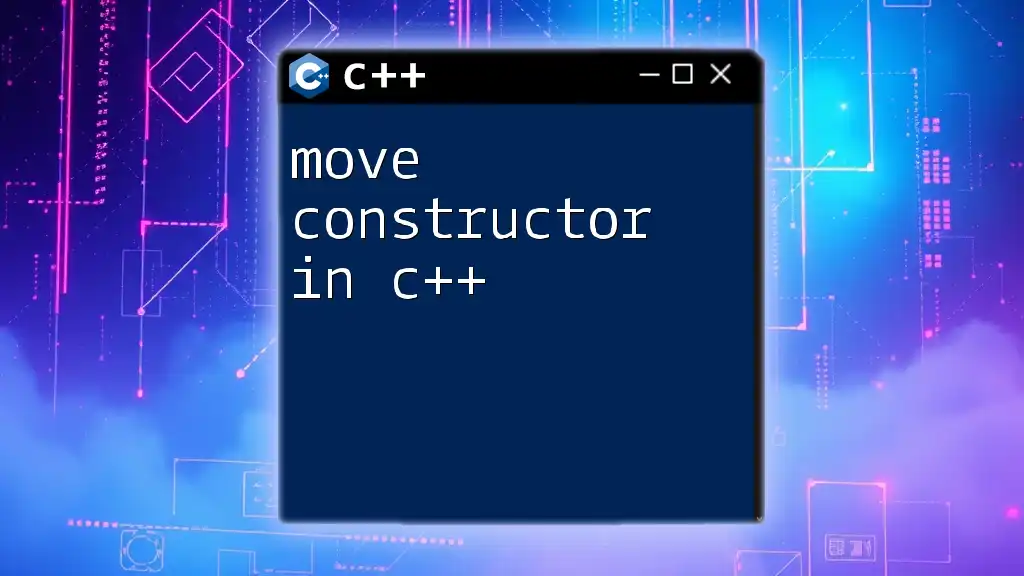
Call to Action
For those eager to expand their knowledge of C++ and constructors further, we invite you to sign up for our tutorials and subscribe to our newsletter for updates on the latest programming insights!