Data structures in C++ are essential constructs that enable efficient organization, management, and storage of data, allowing developers to manipulate it effectively.
Here's a simple example of a C++ struct as a data structure:
struct Student {
int id;
std::string name;
};
Understanding Data Structures
What are Data Structures?
Data structures are fundamental constructs in programming that allow us to organize, manage, and store data efficiently. They provide a systematic way to handle data so that it can be accessed and modified effectively. Each data structure offers various ways to manage data depending on the specific needs of an application, such as the speed of access and manipulation operations.
Importance of Data Structures in C++
The significance of using data structures in C++ cannot be overstated. They play a critical role in optimizing the performance of software applications. Choosing the right data structure can lead to greater efficiency in terms of both speed and memory consumption. For instance, using a linked list instead of an array may result in better performance for certain dynamic data operations, such as insertions and deletions.
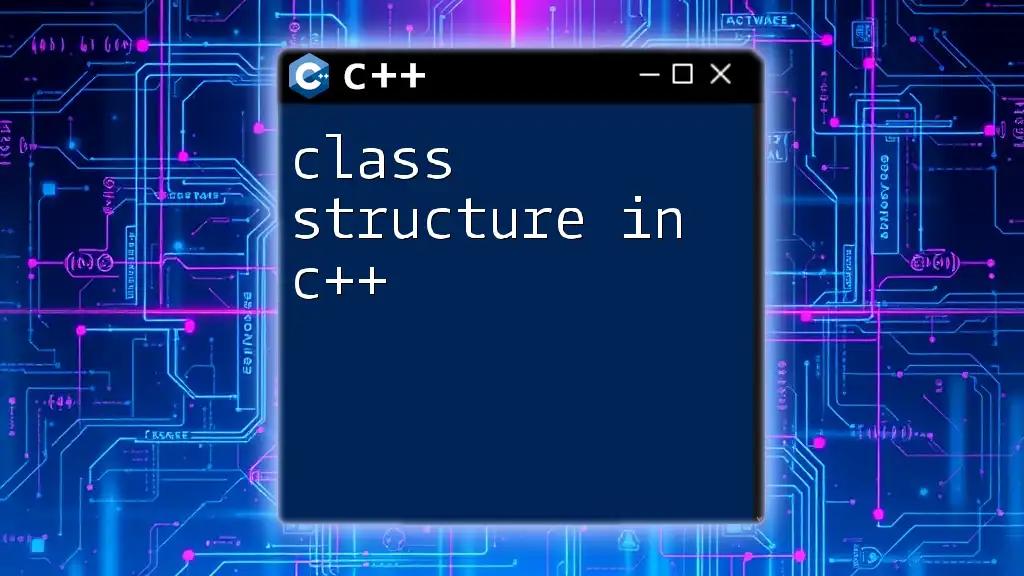
Basic Data Structures in C++
Arrays
Arrays are one of the simplest data structures in C++. They consist of a fixed-size sequence of elements of the same type, stored in contiguous memory locations. Their primary advantage lies in the ease of accessing elements using indices.
Example Code Snippet:
int arr[5] = {10, 20, 30, 40, 50}; // Declaration and initialization of an array
With arrays, accessing an element is performed in constant time, i.e., O(1), making them incredibly efficient for read operations. However, they have limitations, such as a fixed size and costly insertions and deletions.
Structures
Structures in C++ allow us to create custom data types that group related variables. This capability helps in modeling complex real-world entities effectively.
Example Code Snippet:
struct Student {
int rollNo;
std::string name;
};
Using structures, you can encapsulate multiple related attributes into a single data type, making your code cleaner and more understandable. For example, a `Student` struct can hold both roll number and name as a cohesive unit.
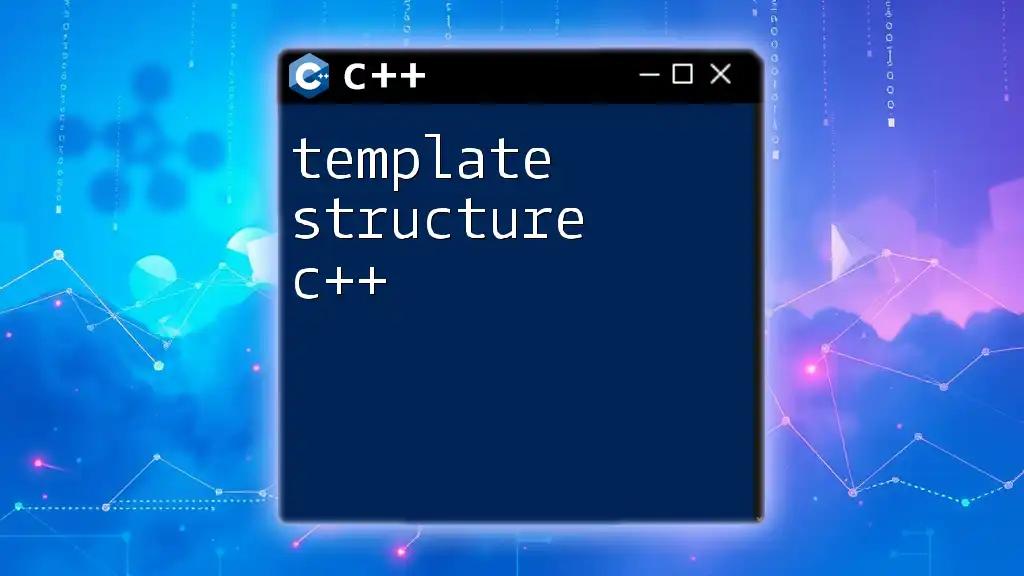
Advanced Data Structures
Linked Lists
A linked list is a linear data structure where elements, called nodes, are stored in separate memory locations. Each node points to the next node, which allows for efficient insertion and deletion operations at the cost of accessing elements via traversal.
Example Code Snippet:
struct Node {
int data;
Node* next; // Pointer to the next node
};
In a linked list, you can easily add new elements without needing to reallocate or resize as with arrays. This flexibility makes linked lists advantageous for applications where the size of the data is unknown in advance.
Stacks
Stacks are a crucial data structure that follow the Last In, First Out (LIFO) principle. They are often used in algorithms that require backtracking, such as depth-first search.
Example Code Snippet:
#include <stack>
std::stack<int> s;
s.push(1); // Pushes 1 onto the stack
s.pop(); // Removes the top element
Stacks are particularly useful for function calls, as they help manage the sequence of operations and maintain the order of execution.
Queues
In contrast to stacks, queues operate on a First In, First Out (FIFO) basis. They are particularly effective in scenarios where processing order is critical, such as task scheduling.
Example Code Snippet:
#include <queue>
std::queue<int> q;
q.push(1); // Adds 1 to the back of the queue
q.pop(); // Removes the front element
Queues are commonly used in breadth-first search algorithms and resource management in concurrent systems.
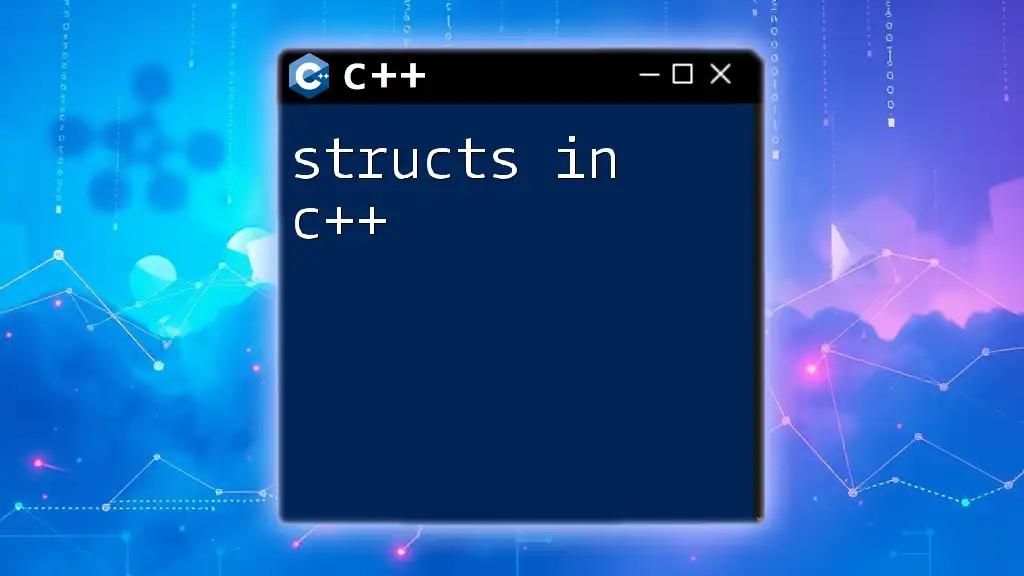
Specialized Data Structures
Trees
Trees are hierarchical data structures consisting of nodes connected by edges. The topmost node is known as the root, and every node can have zero or more children.
Types of Trees
Binary Trees
In a binary tree, each node has at most two children, referred to as the left and right children. Binary trees form the basis of many more advanced data structures.
Example Code Snippet:
struct TreeNode {
int value;
TreeNode* left;
TreeNode* right;
};
Binary trees are highly versatile and can be used in various applications, such as parsing expressions and representing hierarchical data.
Binary Search Trees
Binary Search Trees (BSTs) are a specialized form of binary trees that maintain an organized structure by ensuring that the left subtree contains only nodes with values less than the parent node, and the right subtree contains only nodes with values greater.
Example Code Snippet:
void insert(TreeNode*& root, int key) {
if (!root) {
root = new TreeNode{key, nullptr, nullptr}; // Creating a new node
} else if (key < root->value) {
insert(root->left, key); // Recursively insert in left subtree
} else {
insert(root->right, key); // Recursively insert in right subtree
}
}
This structured organization allows for efficient searching, insertion, and deletion operations, typically performed in O(log n) time.
Heaps
Heaps are tree-based data structures that satisfy the heap property, where a parent node's value must be greater than or equal to the values of its children (max-heap) or less than or equal (min-heap).
Example Code Snippet:
#include <queue>
std::priority_queue<int> maxHeap; // A Max-Heap
Heaps are essential for implementing efficient priority queues and algorithms like heapsort.
Graphs
Graphs consist of nodes (or vertices) connected by edges. They can represent various relationships and are invaluable in numerous applications such as social networks, routing algorithms, and finding the shortest path.
Graphs can be represented in two primary formats: adjacency lists and adjacency matrices. Each representation has its own benefits and drawbacks depending on the application and data density.

Conclusion
In summary, data structures in C++ provide a foundational framework for handling data efficiently. By choosing the right data structure, developers can optimize performance, enhance code readability, and simplify complex problems. As you explore these structures, practice implementing them in your projects to solidify your understanding and navigate the intricacies of data handling in C++.

Additional Resources
To further your understanding of data structures in C++, consider exploring online platforms such as Codecademy, Coursera, or reading notable texts like “Data Structures and Algorithms in C++” by Adam Drozdek. Engaging with these resources will deepen your knowledge and enhance your programming skills.