Data structures and algorithms in C++ provide efficient ways to organize and manipulate data, enhancing performance and enabling the development of sophisticated software solutions.
Here's a simple example demonstrating the use of a vector data structure and a basic algorithm to sort it:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 1, 3};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Data Structures in C++
What are Data Structures?
Data structures are systematic ways of organizing and storing data in a computer so that it can be accessed and modified efficiently. They enable programmers to manage large amounts of data, offering a way to organize, retrieve, and manipulate that data effectively. In C++, understanding data structures is vital since they form the foundation of efficient algorithm implementation and overall program performance.
Common Data Structures Overview
Arrays
An array is a collection of elements identified by index or key. They are fixed in size, allowing quick access to elements using their indices. For example, declaring and initializing an array in C++ can be done as follows:
int arr[5] = {1, 2, 3, 4, 5};
This line creates an array named `arr` that contains five integers. Arrays are suitable for storing data where the size is known beforehand and accessing elements by index is frequent.
Linked Lists
A linked list is a linear data structure where elements, known as nodes, are connected using pointers. Each node contains data and a reference to the next node, allowing for efficient insertion and deletion operations. Here’s a simple definition for a singly linked list:
struct Node {
int data;
Node* next;
};
In comparison to arrays, linked lists are more flexible because they can grow and shrink in size. However, accessing elements takes more time since it requires traversing the list.
Stacks
A stack is a collection of elements that follows the Last In First Out (LIFO) principle. Think of it like a stack of plates; the last plate placed on top is the first one to be removed. Here’s a basic implementation of a stack using an array:
class Stack {
private:
int arr[100];
int top;
public:
Stack() { top = -1; }
void push(int x) { arr[++top] = x; }
int pop() { return arr[top--]; }
};
Stacks are extensively used in scenarios like function call management, expression evaluation, and backtracking algorithms. They provide fast operations due to their simplicity.
Queues
A queue is a collection of elements that follows the First In First Out (FIFO) principle. Unlike stacks, queues allow the first element added to be the first one to be removed. A simple implementation using a linked list may resemble this:
class Queue {
Node* front;
Node* rear;
public:
Queue() {
front = rear = nullptr;
}
void enqueue(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->next = nullptr;
if (rear == nullptr) {
front = rear = newNode;
return;
}
rear->next = newNode;
rear = newNode;
}
int dequeue() {
if (front == nullptr) return -1; // Queue is empty
Node* temp = front;
front = front->next;
if (front == nullptr) rear = nullptr;
int data = temp->data;
delete temp;
return data;
}
};
Queues are particularly useful in scenarios like job scheduling, handling requests in a printer queue, and breadth-first search algorithms.
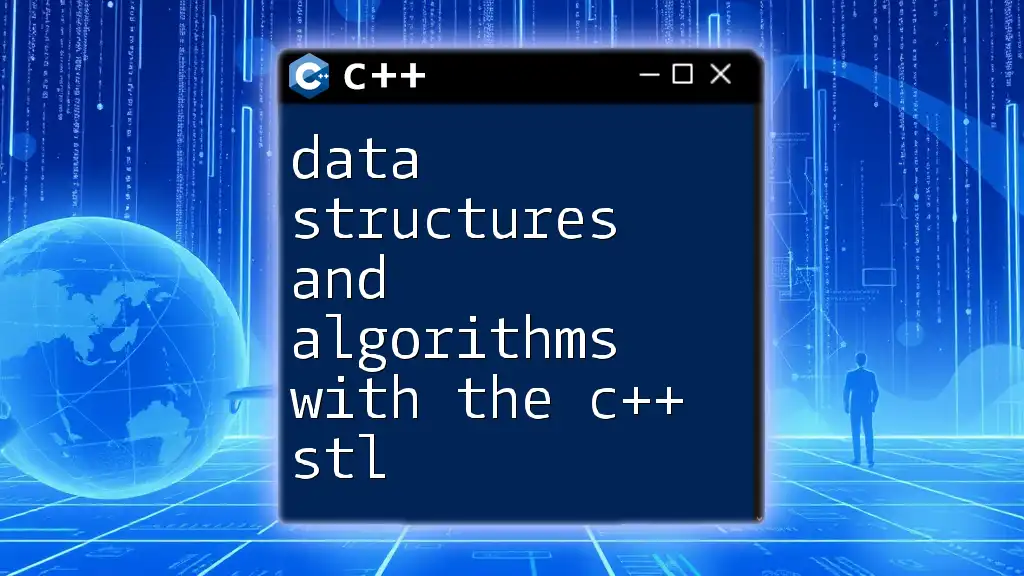
Introduction to Algorithms
What are Algorithms?
An algorithm is a step-by-step procedure or formula for solving a problem. In programming, algorithms define the sequence of operations needed to accomplish a task, such as searching, sorting, or processing data. The design of an efficient algorithm is crucial for improving performance in coding; therefore, understanding different algorithms is an integral part of learning data structures and algorithms with C++.
Common Algorithm Types
Sorting Algorithms
Sorting algorithms organize data in a specific order (ascending or descending). These algorithms play a pivotal role in optimizing data processing and retrieval. For example, the insertion sort algorithm sorts an array by repeatedly picking the next element and inserting it into the correct position:
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
Insertion sort is simple and efficient for small datasets but less suitable for larger lists compared to more complex sorting algorithms, like quicksort.
Searching Algorithms
Searching algorithms locate a specific element within a data structure. Two fundamental types are linear search and binary search. Binary search is efficient on sorted arrays, reducing the average search time significantly. Here’s a basic implementation of binary search in C++:
int binarySearch(int arr[], int l, int r, int x) {
while (l <= r) {
int mid = l + (r - l) / 2;
if (arr[mid] == x) return mid;
if (arr[mid] < x) l = mid + 1;
else r = mid - 1;
}
return -1; // Element not found
}
This algorithm efficiently halves the search space with each iteration, making it much faster than linear search for large datasets.
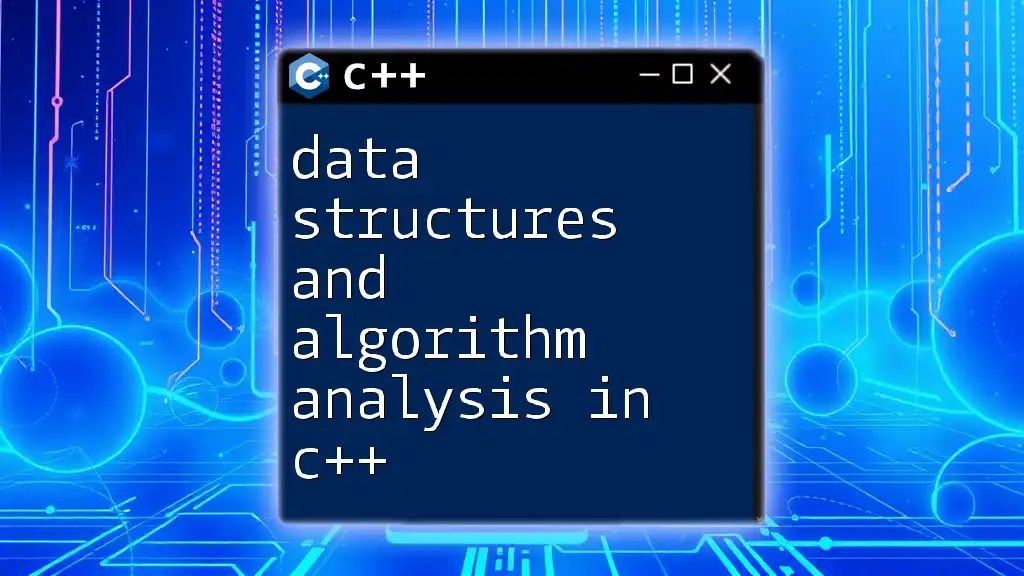
Integrating Data Structures with Algorithms
How Data Structures Improve Algorithm Efficiency
The efficiency of algorithms is profoundly impacted by the choice of data structures. Understanding time complexity and space complexity is crucial in evaluating the performance of an algorithm. For instance, searching for an element in an unsorted array requires O(n) time complexity, while the same operation in a balanced binary search tree can be done in O(log n) time.
Real-World Applications
Data structures and algorithms with C++ find numerous applications in the real world. For example, databases leverage efficient data structures for storage and retrieval operations. In web development, understanding queues can optimize the handling of requests, while gaming often involves stacks to manage game states. By mastering these concepts, developers can create scalable and efficient applications.
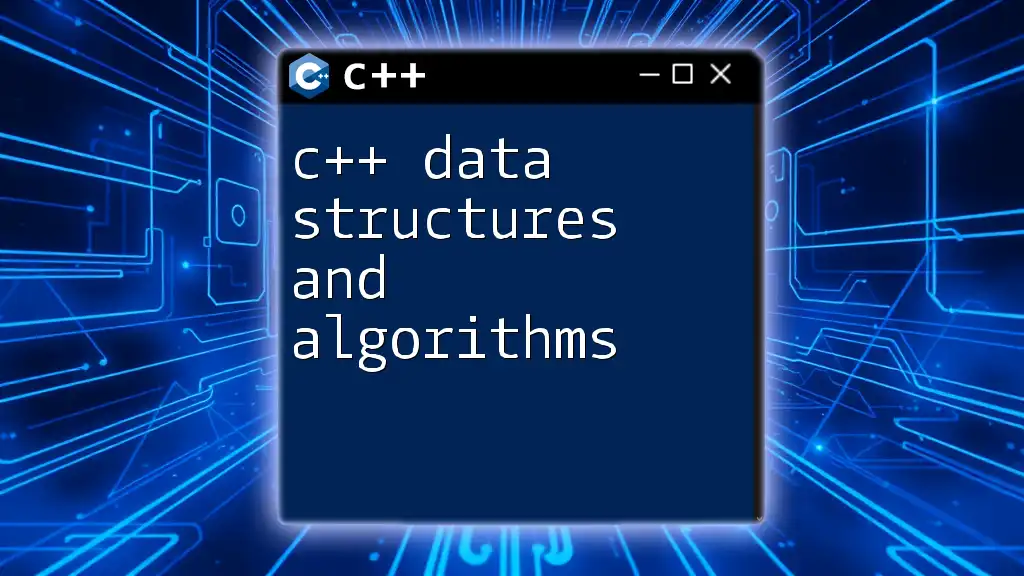
Practical Tips for Mastering Data Structures and Algorithms in C++
Regular Practice
Consistent practice is key to mastering data structures and algorithms. Solving various problems on competitive programming platforms helps solidify your understanding and exposes you to different scenarios where these structures and algorithms come into play.
Recommended Resources
To enhance your understanding further, consider exploring resources such as:
- Books on algorithms like "Introduction to Algorithms" by Cormen et al.
- Online courses on platforms like Coursera or edX focusing on data structures and algorithms with C++.
- Code practice sites such as LeetCode, HackerRank, or CodeSignal.
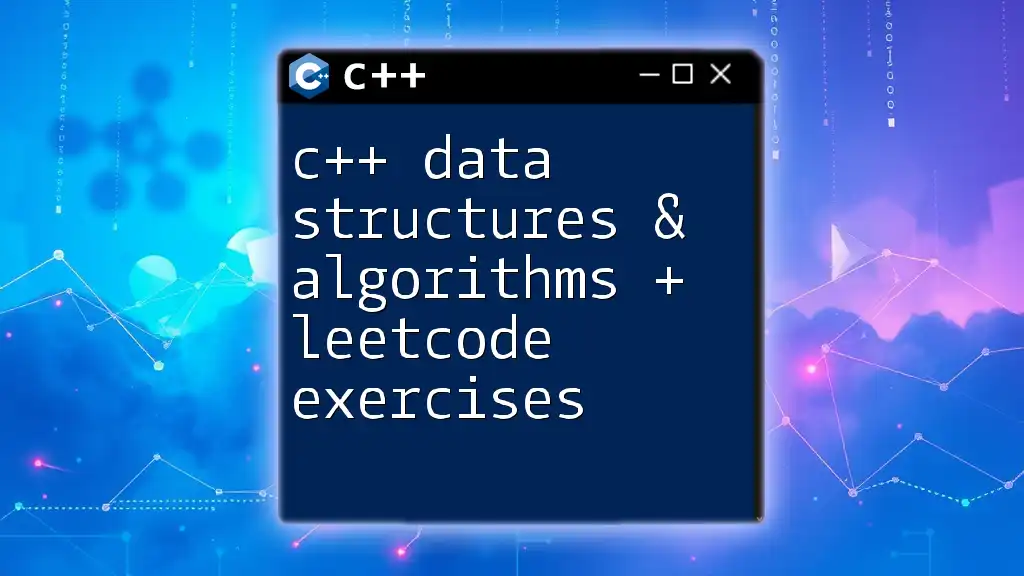
Conclusion
Understanding data structures and algorithms with C++ is fundamental to enhancing your programming skills. These concepts enable you to write efficient, high-performing code applicable across varied domains. By mastering them, you position yourself favorably in the ever-evolving tech landscape.
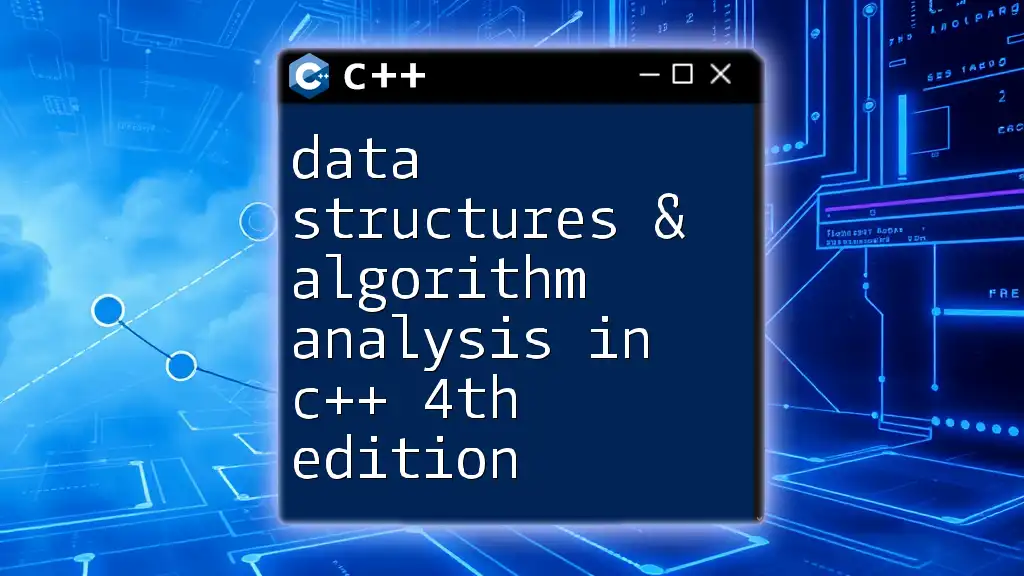
Call to Action
Stay tuned for more tips and tricks on mastering C++ commands and programming concepts. Subscribe to our updates to ensure you’re on the cutting edge of your learning journey!