Dijkstra's algorithm is a popular graph search algorithm used to find the shortest paths from a source node to all other nodes in a weighted graph, implemented in C++ as follows:
#include <iostream>
#include <vector>
#include <set>
#include <climits>
using namespace std;
void dijkstra(int src, const vector<vector<pair<int, int>>>& graph) {
vector<int> dist(graph.size(), INT_MAX);
dist[src] = 0;
set<pair<int, int>> activeVertices;
activeVertices.insert({0, src});
while (!activeVertices.empty()) {
int u = activeVertices.begin()->second;
activeVertices.erase(activeVertices.begin());
for (const auto& edge : graph[u]) {
int v = edge.first;
int weight = edge.second;
if (dist[u] + weight < dist[v]) {
activeVertices.erase({dist[v], v});
dist[v] = dist[u] + weight;
activeVertices.insert({dist[v], v});
}
}
}
for (int i = 0; i < dist.size(); ++i) {
cout << "Distance from node " << src << " to node " << i << " is " << dist[i] << endl;
}
}
Introduction to Dijkstra's Algorithm
Dijkstra's algorithm is a popular and influential algorithm used in computer science for solving the single-source shortest path problem in graphs with non-negative edge weights. The algorithm was developed by Edsger W. Dijkstra in 1956 and has since found applications in various real-world scenarios such as GPS navigation systems and network routing protocols.
Understanding Dijkstra's algorithm is crucial for anyone delving into graph theory and provides a strong base for exploring more complex algorithms.
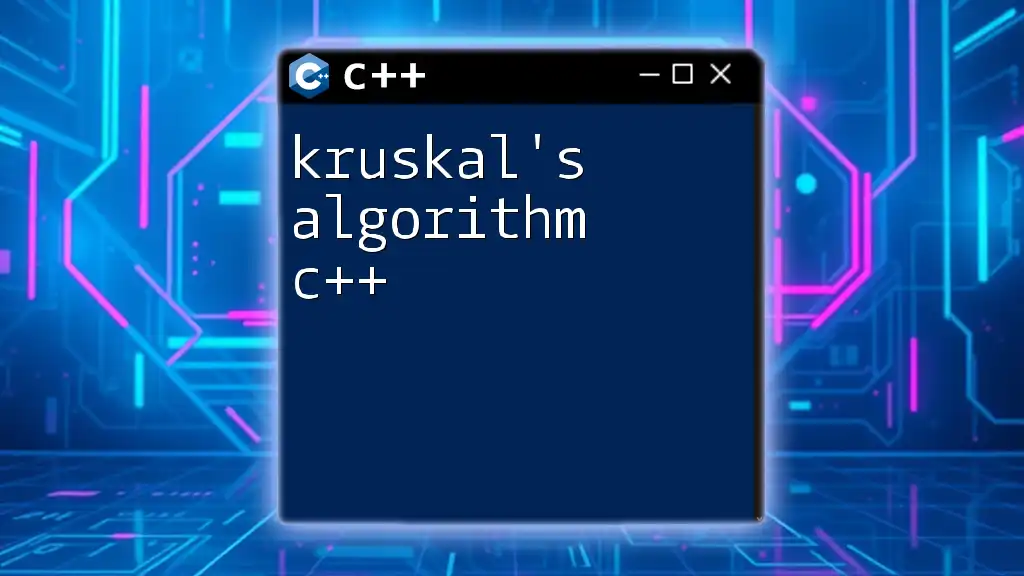
Understanding Graphs
To comprehend Dijkstra’s algorithm, one must first understand graphs. A graph is a collection of nodes, known as vertices, connected by edges. In graph theory, we encounter two main types:
- Directed graphs: Where edges have a direction, indicating the path goes from vertex A to vertex B.
- Undirected graphs: Where edges are bidirectional, implying a mutual connection between vertices A and B.
Representation of Graphs in C++
In C++, there are two common ways to represent graphs:
- Adjacency List: This is a more space-efficient way of representing sparse graphs. Each vertex has a list of connected vertices along with the corresponding edge weights.
- Adjacency Matrix: A 2D array where the rows and columns represent vertices, and the matrix values (0 or the weight) represent the edges between them.
Here’s an example of a simple representation of a graph using an adjacency list:
vector<vector<pair<int, int>>> graph = {
{{1, 2}, {2, 4}}, // edges from vertex 0
{{0, 2}, {2, 1}, {3, 7}}, // edges from vertex 1
{{0, 4}, {1, 1}, {3, 3}}, // edges from vertex 2
{{1, 7}, {2, 3}} // edges from vertex 3
};
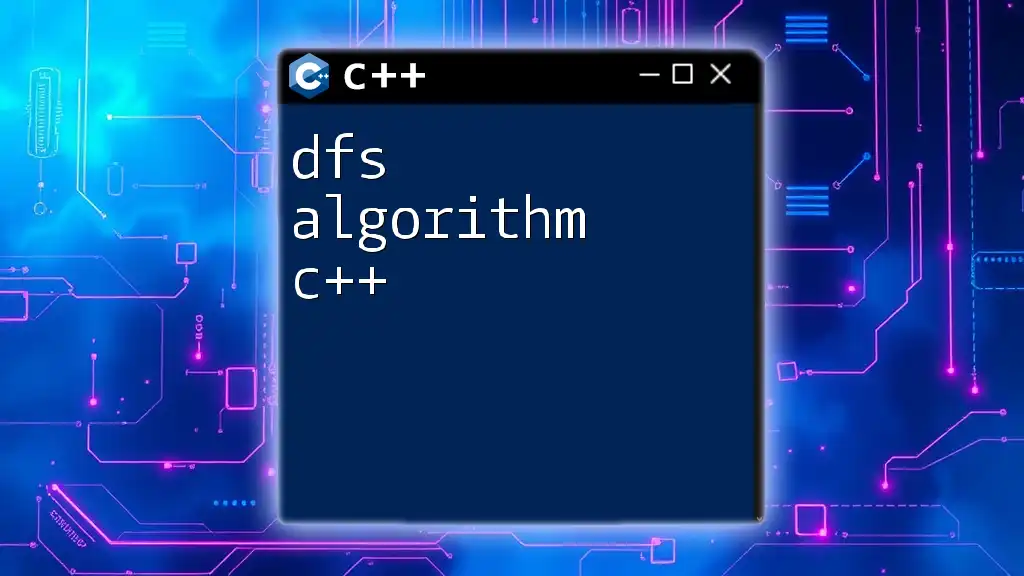
The Concept of Dijkstra's Algorithm
Dijkstra's algorithm operates on the principle of greedy optimization, which means it makes the best choice in each step with the hope of finding a global optimum.
How Dijkstra’s Algorithm Works
The algorithm maintains a distance array that stores the shortest known distance from the source vertex to each other vertex in the graph. The main steps of the algorithm include:
- Initialization: Set distances to infinity for all vertices except the source, which is set to zero. A priority queue (min-heap) is used to efficiently extract the vertex with the smallest distance.
- Relaxation: For each adjacent vertex of the current vertex, update the distance if a shorter path through the current vertex is found.
Pseudocode for Dijkstra's Algorithm
The pseudocode for Dijkstra's algorithm can be visualized as follows:
function Dijkstra(graph, source):
initialize distance[]
set distance[source] = 0
while priority queue is not empty:
u = extract minimum from priority queue
for each neighbor v of u:
if distance[u] + weight(u, v) < distance[v]:
distance[v] = distance[u] + weight(u, v)
update priority queue with new distance[v]
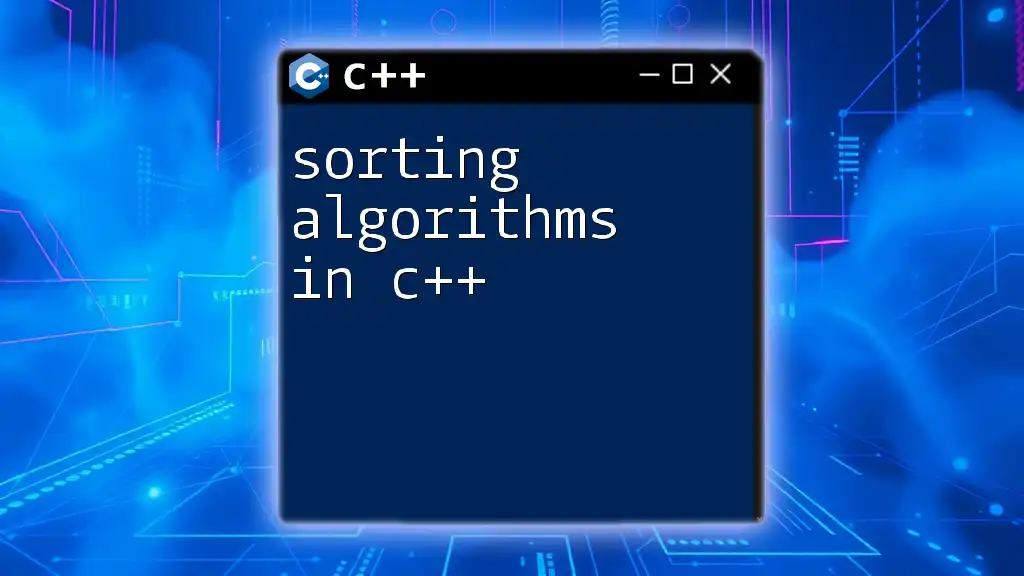
Implementing Dijkstra's Algorithm in C++
Setting Up Your C++ Environment
To implement Dijkstra's algorithm in C++, you'll need to include the following libraries:
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
Writing the C++ Code
The following code illustrates a complete implementation of Dijkstra's algorithm:
using namespace std;
void dijkstra(int source, const vector<vector<pair<int, int>>>& graph) {
int n = graph.size();
vector<int> distance(n, numeric_limits<int>::max());
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
distance[source] = 0;
pq.push({0, source});
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
for (const auto& edge : graph[u]) {
int v = edge.first;
int weight = edge.second;
if (distance[u] + weight < distance[v]) {
distance[v] = distance[u] + weight;
pq.push({distance[v], v});
}
}
}
// Output the shortest distances
for (int i = 0; i < n; i++)
cout << "Distance from source to " << i << ": " << distance[i] << endl;
}
Code Walkthrough
In the provided code:
- We initialize the graph and create a distance array initialized to infinity (`numeric_limits<int>::max()`) for all vertices except the source, which is set to zero.
- A priority queue is employed to retrieve the vertex with the minimum distance efficiently.
- The algorithm iteratively processes each vertex, updating distances based on current known shortest paths and checks if a shorter path to adjacent vertices exists.
Testing the Algorithm
You can test the implementation by creating a sample graph and invoking the `dijkstra` function:
int main() {
vector<vector<pair<int, int>>> graph = {
{{1, 2}, {2, 4}}, // edges from vertex 0
{{0, 2}, {2, 1}, {3, 7}}, // edges from vertex 1
{{0, 4}, {1, 1}, {3, 3}}, // edges from vertex 2
{{1, 7}, {2, 3}} // edges from vertex 3
};
dijkstra(0, graph);
return 0;
}
When this main function is executed, it will calculate the shortest paths from vertex 0 to all other vertices, outputting the distances.
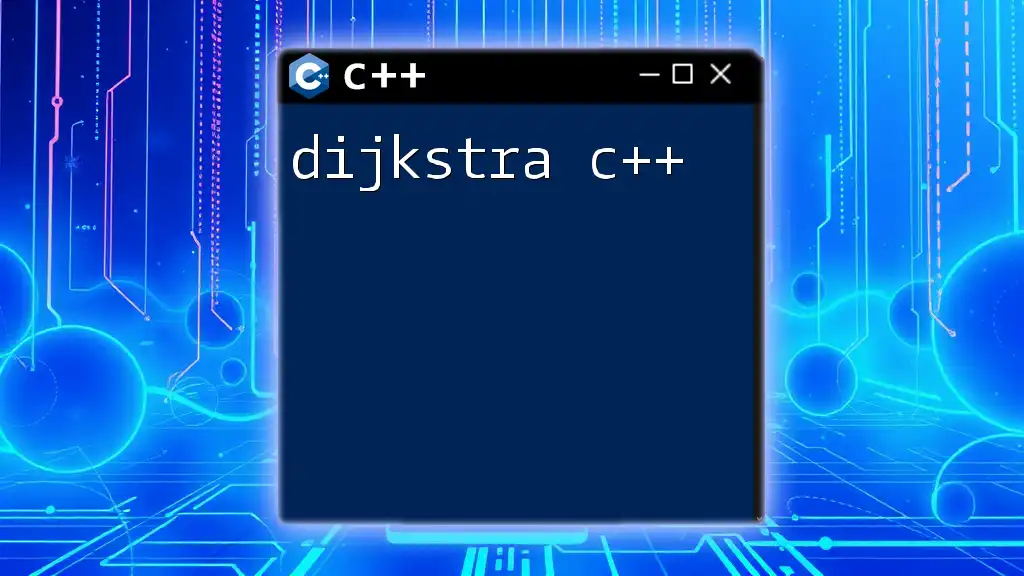
Optimizations and Considerations
Performance Analysis
Dijkstra’s algorithm has a time complexity of O((V + E) log V), where V is the number of vertices and E is the number of edges. This efficiency stems from the use of the priority queue to select the vertex with the minimum distance efficiently.
Limitations of Dijkstra’s Algorithm
While powerful, Dijkstra's algorithm has limitations, notably its inability to handle graphs with negative weight edges. In such cases, other algorithms, such as Bellman-Ford, should be considered.
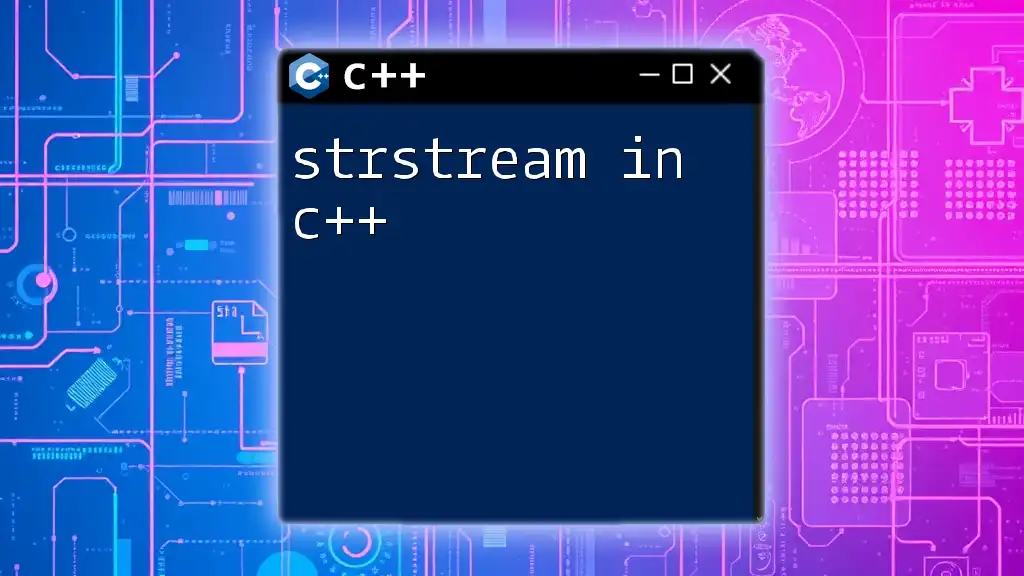
Conclusion
In this article, we explored Dijkstra's algorithm in C++, understanding its significance in solving shortest path problems. We covered everything from graph representation to code implementation and performance considerations. For further learning, consider diving into graph theory resources or related algorithmic studies to expand your knowledge and skills.
With a solid understanding of algorithms like Dijkstra's, you can tackle a wide range of computational and real-world problems with confidence.