Coding standards in C++ are a set of guidelines that help ensure the consistency, readability, and maintainability of code across teams and projects by promoting best practices and code conventions.
Here's a code snippet demonstrating proper naming conventions and comments, which are part of C++ coding standards:
// Function to calculate the factorial of a number
int calculateFactorial(int number) {
int factorial = 1;
for (int i = 1; i <= number; ++i) {
factorial *= i; // Multiply current number to factorial
}
return factorial;
}
What Are Coding Standards?
Definition of Coding Standards
Coding standards are a set of guidelines intended to improve the quality and readability of code. In the context of C++, these guidelines help define conventions regarding style, formatting, and practices to ensure code is consistent, efficient, and understandable.
Benefits of Following Coding Standards
By adhering to coding standards in C++, developers can achieve several significant advantages:
-
Consistency: Maintaining a uniform approach across the codebase not only aids individual developers but also fosters a collaborative atmosphere. When everyone adheres to the same standards, it becomes much easier for team members to read and understand each other's code.
-
Readability: Well-structured code is much easier to read. Clear naming conventions, consistent formatting, and organized structure dramatically improve the readability of the code, reducing the time required to comprehend the logic.
-
Maintainability: Code that adheres to established standards is inherently easier to maintain and modify. When changes are necessary, developers can confidently navigate the code without having to decipher varied structures or styles.
-
Collaboration: In a team setting, coding standards facilitate smoother collaboration. They make it easier to onboard new members, conduct code reviews, and carry out collective maintenance.
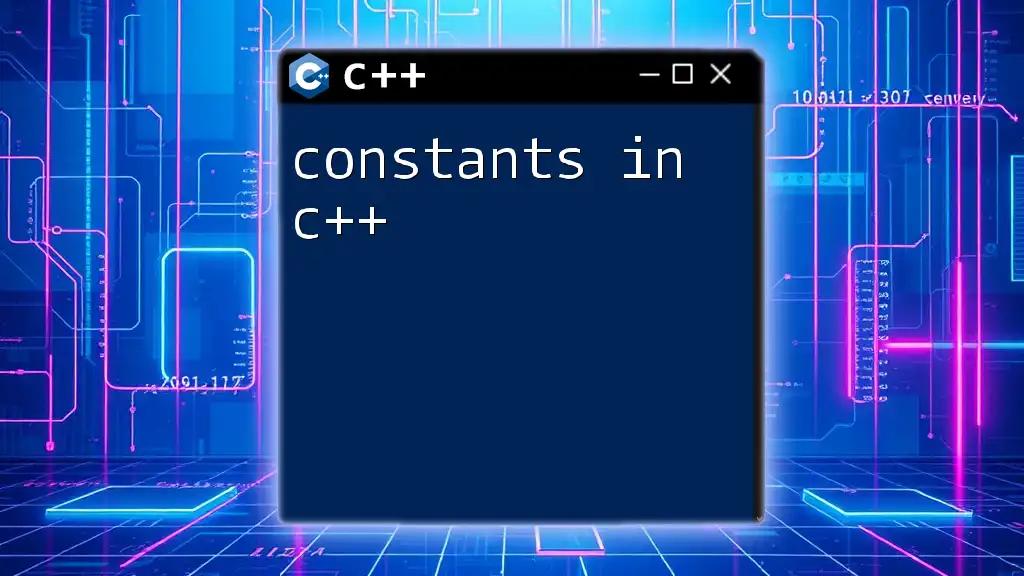
Popular C++ Coding Standards
ISO C++ Guidelines
The ISO C++ standards provide a foundational set of rules and recommendations that apply universally to C++ programming. By strict adherence to these guidelines, code can achieve a high level of safety and performance. Some key principles include:
- Use `nullptr` instead of `NULL` for pointer definitions to enhance type safety.
- Prefer range-based for loops for iterating over containers, improving readability and safety.
Implementing these guidelines can often prevent common pitfalls that may arise during the development process.
Google C++ Style Guide
The Google C++ Style Guide is another widely recognized set of standards designed to enhance consistency and readability across C++ codebases. Some important rules include:
-
Naming conventions: Classes should use CamelCase, while variables and functions typically use lower_case_with_underscores. For example:
class Student { public: void calculate_average(); private: int student_age; };
-
Bracing style: Always place braces on the same line as the declaration. This approach minimizes confusion regarding code blocks.
By following these guidelines, developers can create cohesive and easily navigable code.
CPPCoreGuidelines
CPPCoreGuidelines provide modern C++ best practices with an emphasis on safety and performance. They are authored by Bjarne Stroustrup and a team of experts, advocating for principles that promote robustness. For instance, prefer smart pointers over raw pointers to manage resource lifetimes effectively.
An example:
#include <memory>
void example() {
std::unique_ptr<int> ptr = std::make_unique<int>(10);
// Automatic memory management
}
The guidelines also emphasize clarity and minimizing complexity, aiming to streamline code for better comprehension.

Key Elements of C++ Coding Standards
Naming Conventions
Naming conventions play a pivotal role in coding standards in C++. They set a framework for naming variables, functions, classes, and other elements in your code, which fosters better communication among developers. Recommended rules include:
-
Variables and Functions: Always choose descriptive names that reflect the purpose of the variable or function. For example, `int studentAge;` is more informative than `int age;`.
-
Classes and Structures: Use PascalCase for class names to differentiate them easily from variables and functions. For instance, `class EmployeeRecord {}` follows the convention neatly.
Code Structure
A clear and consistent code structure is essential for maintenance and readability:
- Indentation: Code indentation must be consistent. One common approach is to use four spaces per indentation level. Poor indentation can lead to confusion and misinterpretation of the code structure, as shown below:
if (condition) {
doSomething();
} else {
doSomethingElse();
}
-
Braces Style: Different styles exist for braces placement. The K&R style without line breaks is concise but may reduce readability in larger code blocks.
-
Line Length: Aim to keep line length under 80 characters. If a line exceeds this limit, consider breaking it up for clarity.
Commenting and Documentation
Effective commenting and documentation are crucial for maintaining code clarity:
-
Types of Comments: Different styles of comments serve various purposes. Inline comments can explain specific lines, while block comments can introduce larger sections of code. Always aim for clear, concise commentary that adds value.
-
Documentation Systems: Tools like Doxygen help automate documentation generation. Here's a basic example of how to comment for Doxygen:
/**
* Calculates the average score.
* @param scores A vector of integer scores.
* @return The average score.
*/
double calculateAverage(const std::vector<int>& scores);
Error Handling
Error handling is a vital aspect of coding standards. Clear practices not only increase robustness but also enhance user experience and safety.
- Prefer exceptions over error codes when dealing with exceptional situations. This approach often makes the code cleaner and easier to understand, as illustrated below:
void readFile(const std::string& filePath) {
if (!fileExists(filePath)) {
throw std::runtime_error("File does not exist!");
}
// Further file processing
}
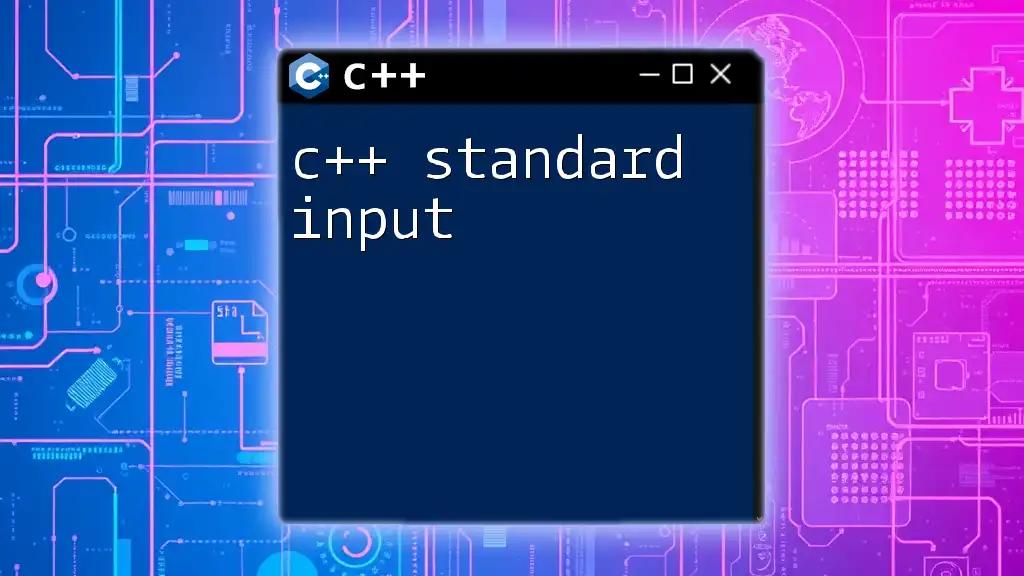
Advanced Topics in C++ Coding Standards
Code Optimization and Performance
When designing code, it’s critical to consider optimization. Efficient code enhances performance and reduces resource consumption. Avoid common pitfalls like unnecessary copies. Instead, utilize references and move semantics effectively:
void processData(const std::vector<int>& data) {
// Pass by reference to prevent copying
}
Additionally, favor algorithmic efficiency. Choose suitable algorithms and data structures for the task at hand, as this can significantly affect performance.
Security Considerations
Incorporating security into coding standards is essential to protect against vulnerabilities.
- Common Vulnerabilities: Familiarize yourself with known vulnerabilities like buffer overflows. Using standard functions with built-in bounds checking, such as `std::string` instead of C-style strings, can mitigate risks.
Example of a secure implementation:
#include <string>
void safeCopy(const std::string& source) {
std::string destination = source; // Safe copy with bounds checking
}

Tools and Resources to Enforce Coding Standards
Static Code Analysis Tools
Static code analysis tools play a critical role in maintaining coding standards. Tools like `cpplint` and `Clang-tidy` systematically review your code against predefined coding standards, identifying issues early in the development process.
Integrating these tools into your development workflow will help enforce adherence to standards and reduce the likelihood of errors.
Code Review Practices
Peer code reviews are essential for enforcing coding standards. They not only help identify potential issues but also serve as a learning opportunity for team members.
A checklist for effective code reviews can include:
- Consistent naming conventions
- Proper use of comments
- Error handling techniques
- Code optimization considerations
Developers should engage in constructive feedback, focusing on improving code quality collectively.
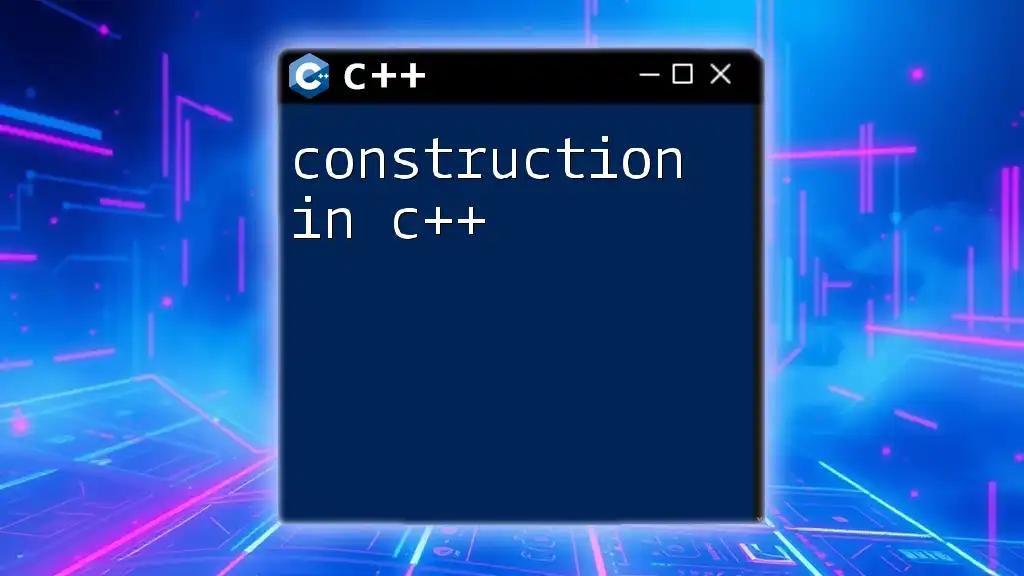
Conclusion
In summary, coding standards in C++ are not just recommendations; they are essential for achieving high-quality, maintainable, and secure code. By embracing these guidelines, developers can foster collaboration, improve readability, and ultimately create a more robust codebase. By acknowledging the importance of these standards and actively implementing them, you can significantly enhance your coding practices.
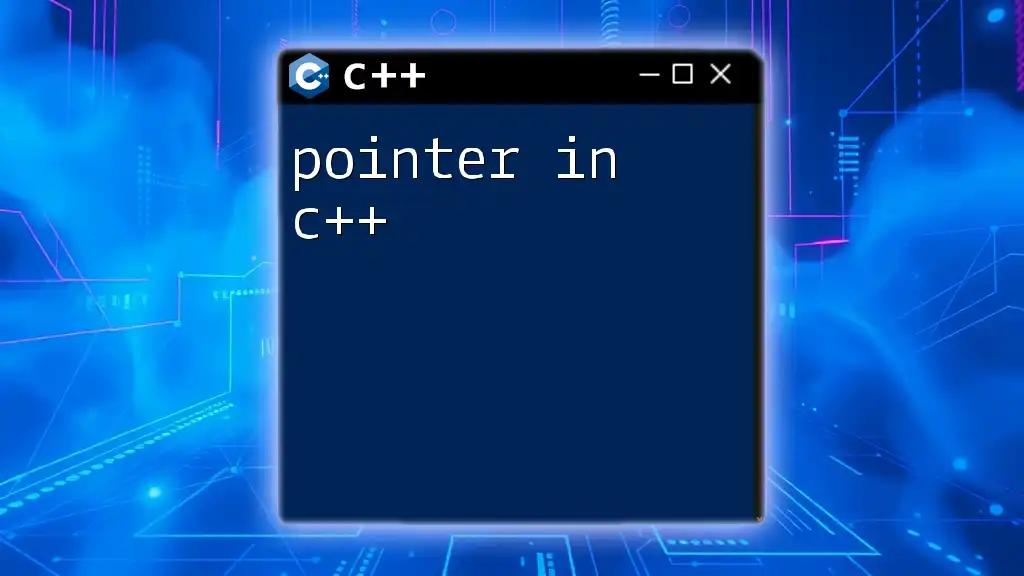
Additional Resources
For further exploration of coding standards, consider diving into recommended books, articles, and online courses. Familiarize yourself with the official guidelines discussed in this article to strengthen your understanding and application of C++ coding standards.