In C++, you can sort an array using the `std::sort` function from the `<algorithm>` header, which sorts the elements in ascending order efficiently.
Here’s a code snippet demonstrating how to sort an array:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
for (int i = 0; i < n; i++)
std::cout << arr[i] << " ";
return 0;
}
Understanding the C++ Array
In C++, an array is a collection of elements of the same data type that are stored in contiguous memory locations. This data structure provides a way to organize and manage a set of related data efficiently. The syntax for declaring and initializing arrays is straightforward:
dataType arrayName[arraySize];
For example, to declare and initialize a one-dimensional array of integers with five elements:
int numbers[5] = {1, 2, 3, 4, 5};
C++ also supports multidimensional arrays. For instance, a two-dimensional array can be declared as follows:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
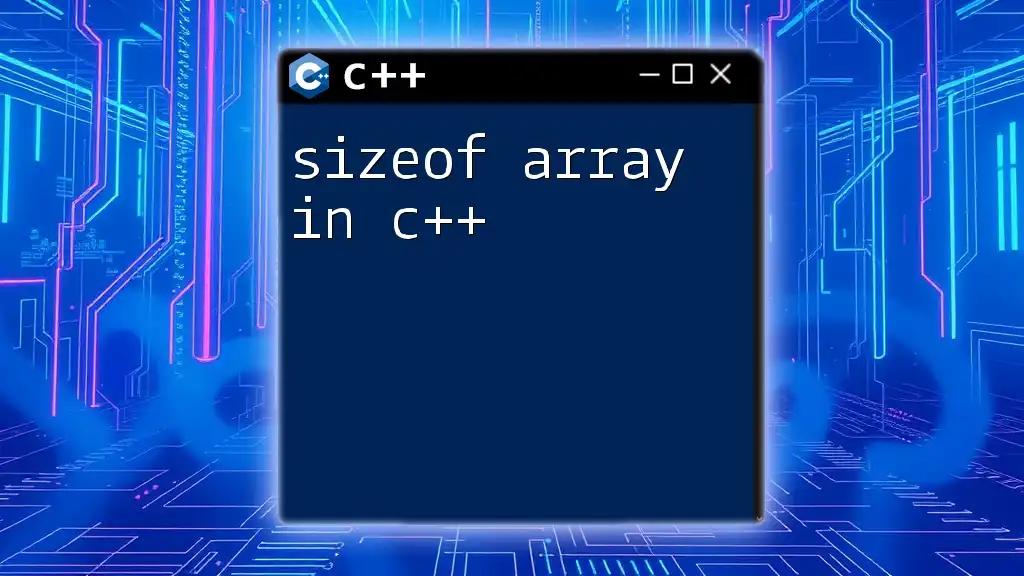
Why Sort an Array?
Sorting an array is crucial for several reasons:
- Search Efficiency: A sorted array allows for faster search operations, especially when using algorithms like binary search.
- Data Organization: Many algorithms and data structures function more effectively with sorted data.
- Statistical Applications: Sorted data makes it easier to compute statistical measures, such as medians and percentiles.
Real-world applications of sorted arrays include database indexing, data analysis, and managing inventory records.

Types of Sorting Algorithms
When it comes to sorting an array in C++, multiple algorithms can be employed. Each algorithm has its own advantages and time complexities. Here’s a brief overview:
-
Bubble Sort: Simple to implement but inefficient for large datasets. It repeatedly steps through the list to be sorted, compares adjacent elements, and swaps them if they are in the wrong order. Its average and worst-case time complexity is O(n²).
-
Selection Sort: This algorithm divides the array into two parts: a sorted and an unsorted section. It repeatedly selects the smallest (or largest) element from the unsorted section and moves it to the end of the sorted section. Its time complexity is also O(n²).
-
Insertion Sort: Efficient for small data sets, this algorithm builds a sorted array one element at a time by picking an element and inserting it into the correct position in the sorted part. It has an average and worst-case time complexity of O(n²), but it performs well on partially sorted data.
-
Merge Sort: A more efficient, divide-and-conquer algorithm that divides the array into halves, sorts each half, and merges them back together. Its time complexity is O(n log n), making it significantly faster for large datasets.
-
Quick Sort: Another divide-and-conquer algorithm that selects a 'pivot' element and partitions the array around it. It has an average-case time complexity of O(n log n) and is generally faster than merge sort.

Utilizing the Standard Template Library (STL) for Sorting Arrays
C++ offers a powerful tool through the Standard Template Library (STL), which simplifies many tasks, including sorting. The `std::sort()` function is a versatile built-in function that can sort arrays efficiently.
Code Snippet — Sorting with `std::sort()`
To sort an array using `std::sort()`, include the `<algorithm>` header:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {5, 2, 8, 1, 4};
std::sort(arr, arr + 5);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
In this example, `std::sort()` sorts the array `arr`. The function takes two arguments: the beginning and the end of the array. This approach is typically efficient and uses the Quick Sort algorithm internally.

Implementing Custom Sorting Functions
In certain cases, you may need to implement a custom sorting function to sort arrays based on specific criteria. This is easily achievable with `std::sort()` by providing a comparator function.
Code Snippet — Custom Sorting with a Comparator
To define a custom sorting criterion, create a function that returns `true` if the first argument should come before the second:
#include <iostream>
#include <algorithm>
bool customCompare(int a, int b) {
return a > b; // Sort in descending order
}
int main() {
int arr[] = {5, 2, 8, 1, 4};
std::sort(arr, arr + 5, customCompare);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
In this example, the `customCompare` function sorts the array in descending order. By passing this function as the third argument to `std::sort()`, you can easily customize the sorting behavior.

Handling Special Cases
Sorting an Empty Array
Sorting an empty array is a straightforward case in C++. When you attempt to sort an empty array, the `std::sort()` function effectively does nothing. This is beneficial because it avoids unnecessary computations and errors.
Sorting a Nearly Sorted Array
When dealing with nearly sorted arrays, insertion sort is often a more efficient choice due to its ability to run in linear time for nearly sorted data. The choice of sorting algorithm can have significant performance implications, and understanding the data you are working with is crucial for optimized performance.
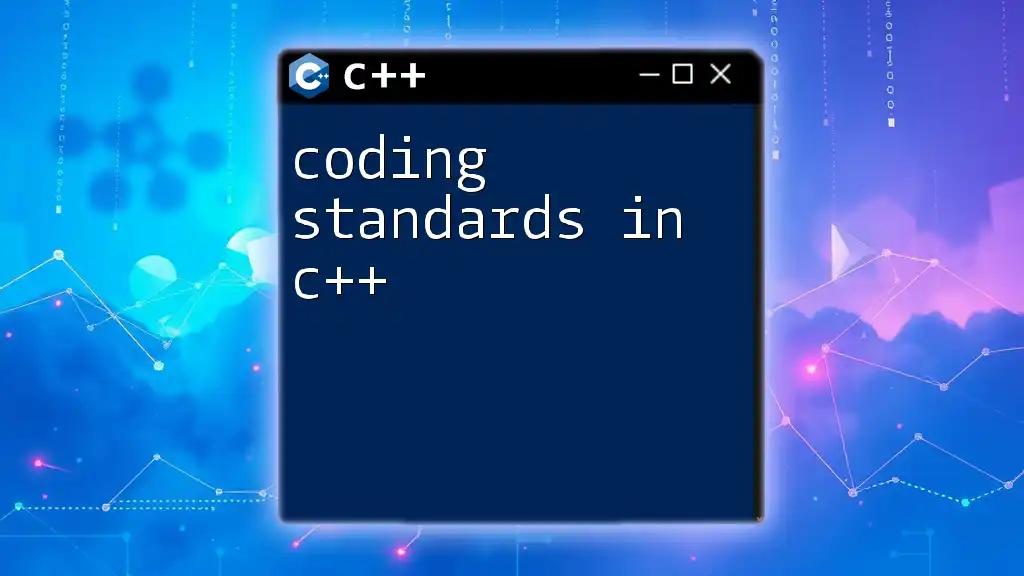
Tips for Optimal Sorting in C++
To ensure efficient sorting operations, keep the following tips in mind:
- Choose the Right Algorithm: Select an algorithm based on the size and nature of the dataset. For small datasets, simpler algorithms may suffice, while larger datasets benefit from more complex ones like merge or quick sort.
- Minimize Memory Usage: Some sorting algorithms require additional space. If memory is limited, consider in-place sorting algorithms.
- Avoid Redundant Sorting: Only sort when necessary. If the data is already sorted or nearly sorted, avoid repeating the sorting process.
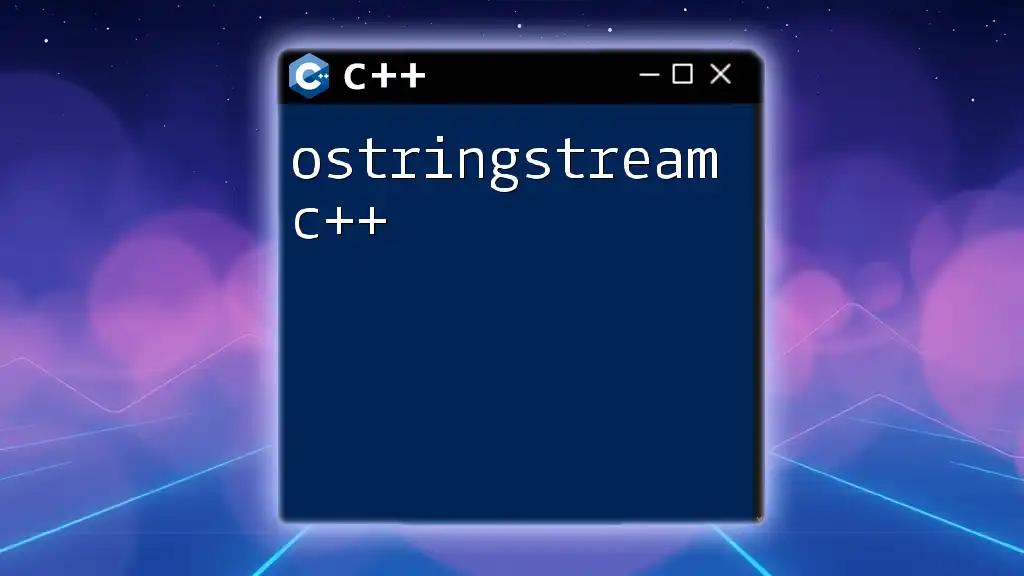
Conclusion
Sorting an array in C++ is fundamental for efficient data processing and retrieval. By understanding the various sorting algorithms, utilizing the STL, and implementing custom sorting functions, you can greatly enhance the performance of your applications. Whether for academic purposes or real-world applications, mastering array sorting in C++ will undoubtedly serve you well. As you continue your journey in C++, remember to practice with various sorting examples and explore the rich resources available for further learning.