A structure array in C++ allows you to create an array of structures, enabling you to group different data types under a single name, making data management easier.
Here's a simple code snippet showcasing a structure array in C++:
#include <iostream>
using namespace std;
struct Student {
string name;
int age;
};
int main() {
Student students[3] = {{"Alice", 20}, {"Bob", 21}, {"Charlie", 22}};
for (int i = 0; i < 3; i++) {
cout << "Name: " << students[i].name << ", Age: " << students[i].age << endl;
}
return 0;
}
What is a Structure in C++?
A structure in C++ is a user-defined data type that groups together different types of variables (called members) under a single name. This allows you to create a composite data type that can hold complex data in a more organized way.
Benefits of Using Structures
Using structures can significantly enhance the organization and readability of your code. For instance, when handling a student record, instead of creating separate variables for the student's ID, name, and marks, a structure allows you to encapsulate all the relevant information within one entity. This not only improves the logical grouping of data but also simplifies data manipulation.
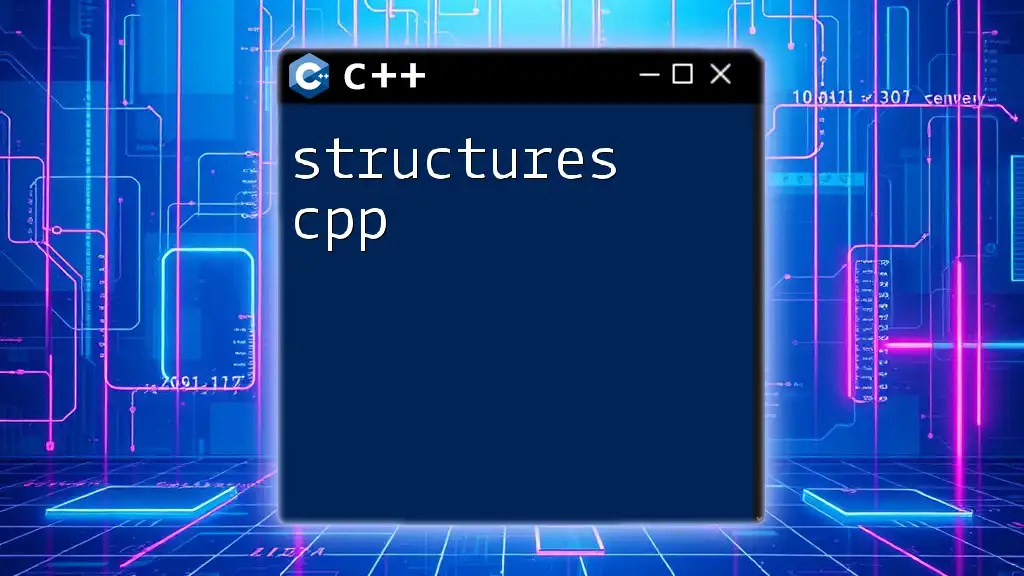
Understanding Arrays in C++
An array is a collection of variables that are accessed with a single name and an index. All elements in an array are of the same data type, which makes arrays a powerful tool for managing multiple related items efficiently.
Characteristics of Arrays
Arrays have several important characteristics:
- Fixed Size: The size of an array is determined at the time of its declaration and cannot be changed during runtime.
- Homogeneous Data Types: All elements in an array must be of the same data type, either primitive ones like integers or complex data types like structures.
Here's a simple example of how to declare an array:
int numbers[5]; // Declaration of an integer array of size 5
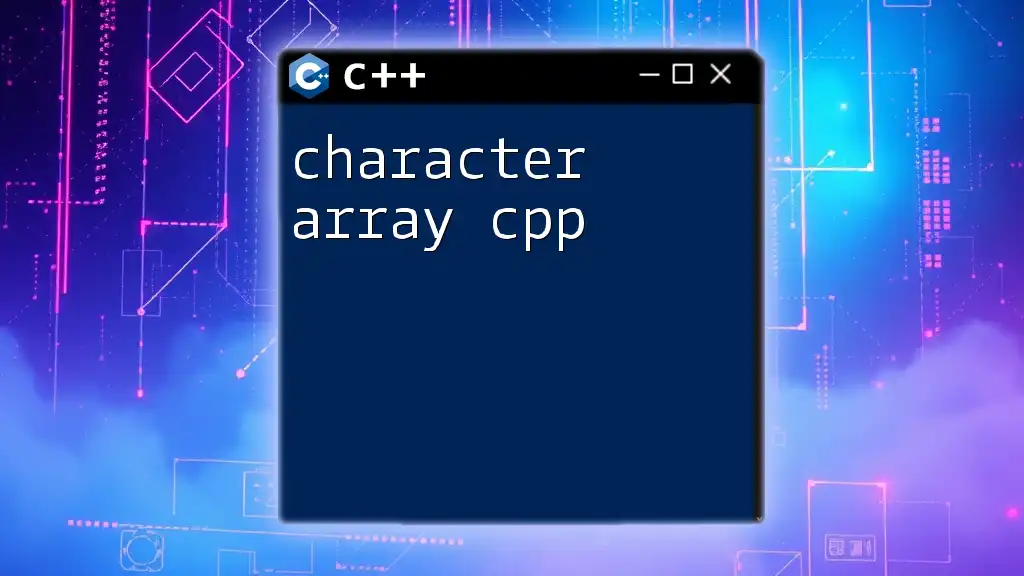
Combining Structures and Arrays
What is a Structure Array?
A structure array is essentially an array where each element is a structure. This allows you to create multiple records of the same type while keeping the associated data together. For instance, if you have a `Student` structure, an array of `Student` structures will enable you to maintain records for an entire class of students.
Syntax of Declaring a Structure Array
Declaring a structure array follows a standard syntax that involves defining the structure first and then creating an array of that structure. Here’s how you can declare an array of structures:
struct Student {
int id;
char name[30];
float marks;
};
Student students[100]; // Declare an array to hold 100 Student records
In this example, we've defined a structure named `Student`, containing three members: an unsigned integer for `id`, an array of characters for `name`, and a float for `marks`. We then create an array `students` that can hold up to 100 such `Student` structures.
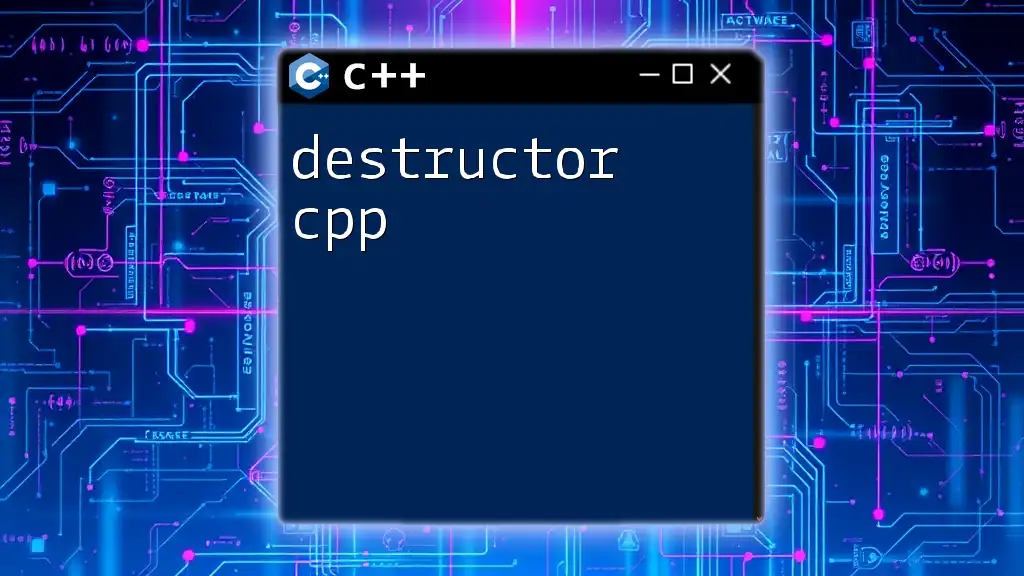
Accessing Structure Members in Arrays
How to Access Members
Once a structure array is created, you can access the members of each structure using the dot operator. For example, you can assign values to the members of the second structure in the array like this:
students[1].id = 102;
strcpy(students[1].name, "Bob");
students[1].marks = 92.0;
In this snippet, we access the `id`, `name`, and `marks` members of the student at index 1. The `strcpy` function is used here to copy the string for the student's name since `name` is an array of characters.
Looping through Structure Arrays
You can also iterate through structure arrays using loops, which is particularly useful when you need to access, output, or update data for multiple records. Here’s an example of how to loop through and display each student’s information:
for (int i = 0; i < 100; i++) {
cout << students[i].id << " " << students[i].name << " " << students[i].marks << endl;
}
This loop will print out the details of all students in the `students` array, demonstrating how to access multiple structure members efficiently.
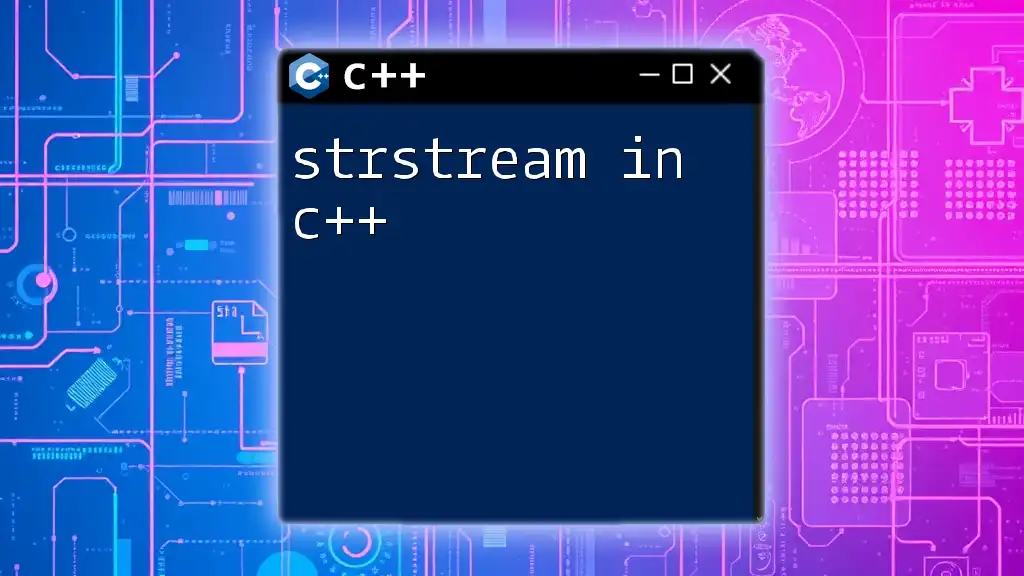
Practical Example: Student Database
Scenario Description
Let's consider a simple student database management application that utilizes a structure array to handle students' records seamlessly.
Defining the Structure
First, we will define a `Student` structure that includes an ID, name, and marks:
struct Student {
int id;
char name[30];
float marks;
};
Creating and Initializing the Array
Next, we can create and initialize an array of structures to represent a few students:
Student students[3] = {
{101, "Alice", 85.5},
{102, "Bob", 92.0},
{103, "Charlie", 76.5}
};
In this example, we have initialized an array with three `Student` records, each containing relevant data.
Function to Display Students
It’s essential to create a function to display the data stored in the structure array. Here’s a simple function to do that:
void displayStudents(Student students[], int count) {
for (int i = 0; i < count; i++) {
cout << students[i].id << " " << students[i].name << " " << students[i].marks << endl;
}
}
You can call this function in your main program to output the details of all students within the `students` array.
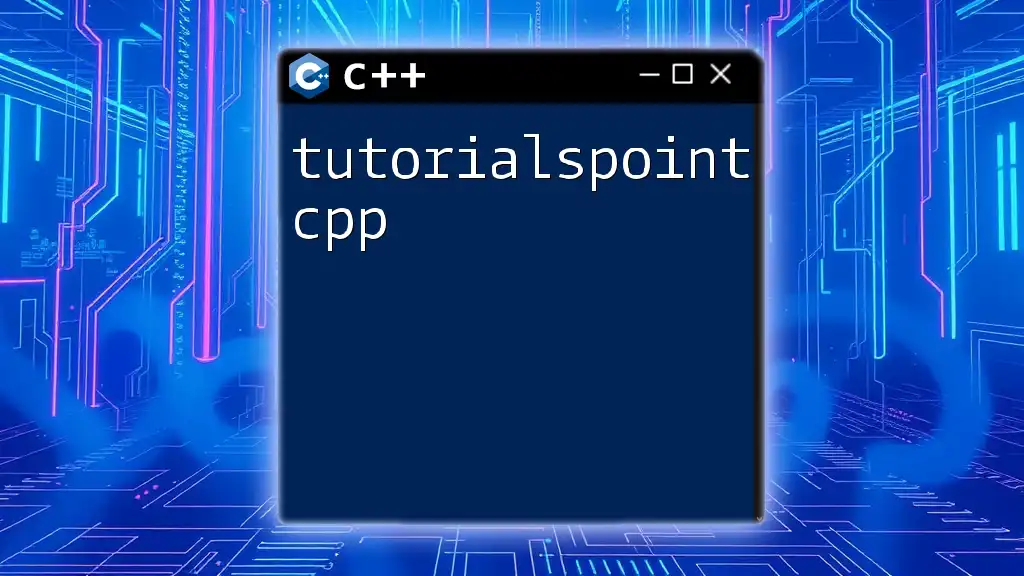
Common Mistakes and Troubleshooting
Type Mismatch
One common error programmers might encounter when dealing with structure arrays is a type mismatch. For instance, trying to assign a string directly to an integer member will lead to a compilation error. Always ensure you are matching data types when working with members.
Memory Management Issues
When accessing unallocated members or exceeding the array bounds, you might face segmentation faults. To avoid this, always perform checks to ensure you are working within the allocated limits of the structure array.
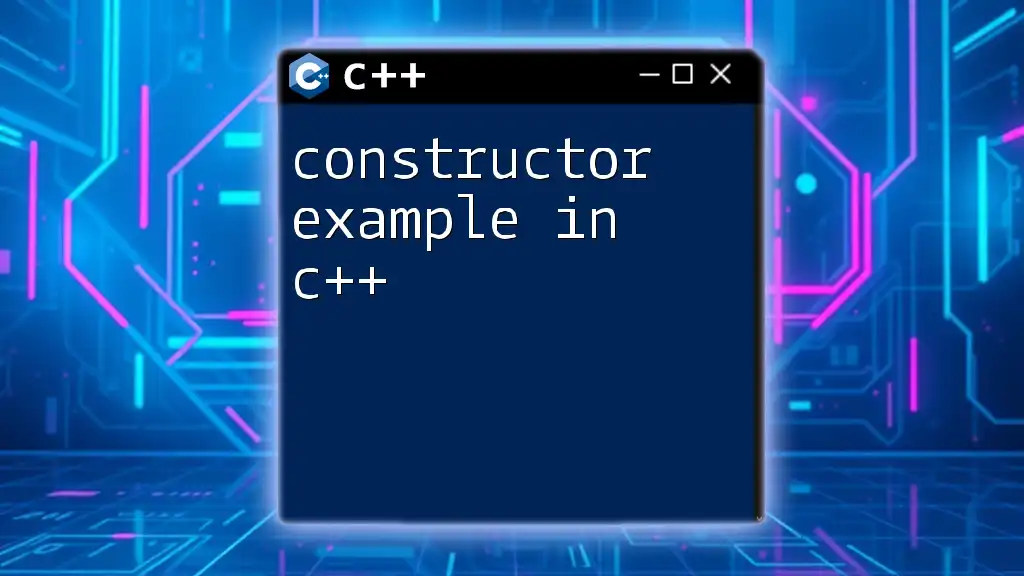
Conclusion
Understanding the structure array in C++ is fundamental for efficient data management, especially when dealing with bulk data like records. Structures allow you to encapsulate related data, while arrays provide a method to hold multiple records of that data type. By practicing the examples and concepts discussed, you'll gain confidence in utilizing structure arrays to create robust C++ applications. Happy coding!
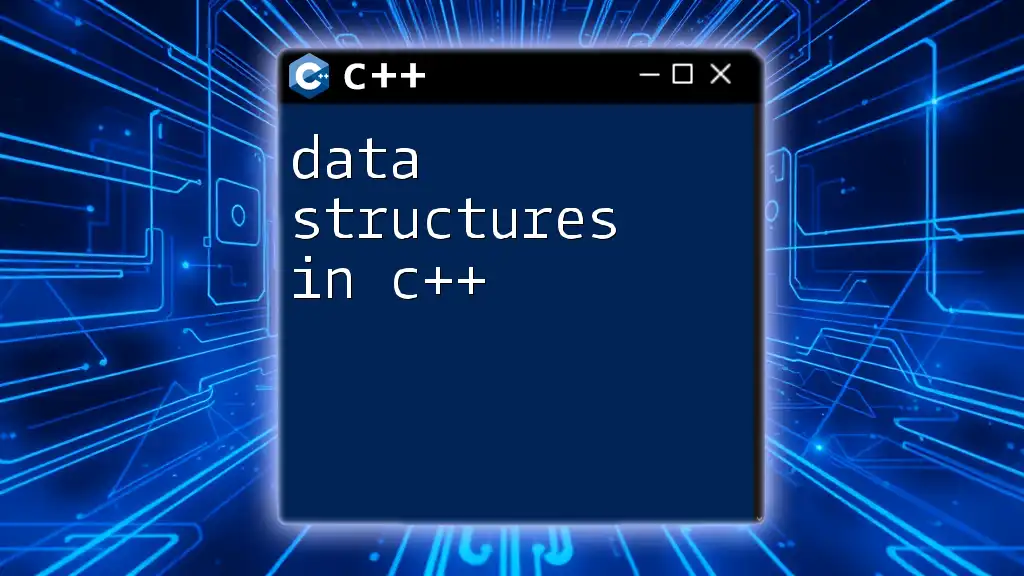
Additional Resources
Recommended Books and References
While your journey in learning may not end here, consider referring to classic C++ programming books and resources available online for deeper insights and examples.
Online Forums and Communities
Engaging with online communities such as Stack Overflow can provide you with additional support and enable discussions on best practices for using structure arrays in C++.