Structures in C++ are user-defined data types that group related variables of different types under a single name, allowing for more organized and manageable code.
struct Person {
std::string name;
int age;
float height;
};
Person john;
john.name = "John Doe";
john.age = 30;
john.height = 5.9;
Understanding the Syntax of Structures in C++
C++ Struct Syntax
In C++, a struct is a user-defined data type that groups different data types together. The syntax for declaring a structure is straightforward. Here’s how you can define a basic struct:
struct StructureName {
dataType member1;
dataType member2;
// ... more members
};
In this definition:
- `StructureName` is the name you give to your struct.
- `member1`, `member2`, etc., are the variables (members) that belong to the structure, each of which can be of different data types.
Example of Structure in C++
To illustrate the concept of structures, consider this simple example:
struct Point {
int x;
int y;
};
In this example, we defined a structure named Point with two integer members, `x` and `y`. This structure can be used to represent a point in a 2D space, where `x` represents the horizontal coordinate and `y` represents the vertical coordinate.
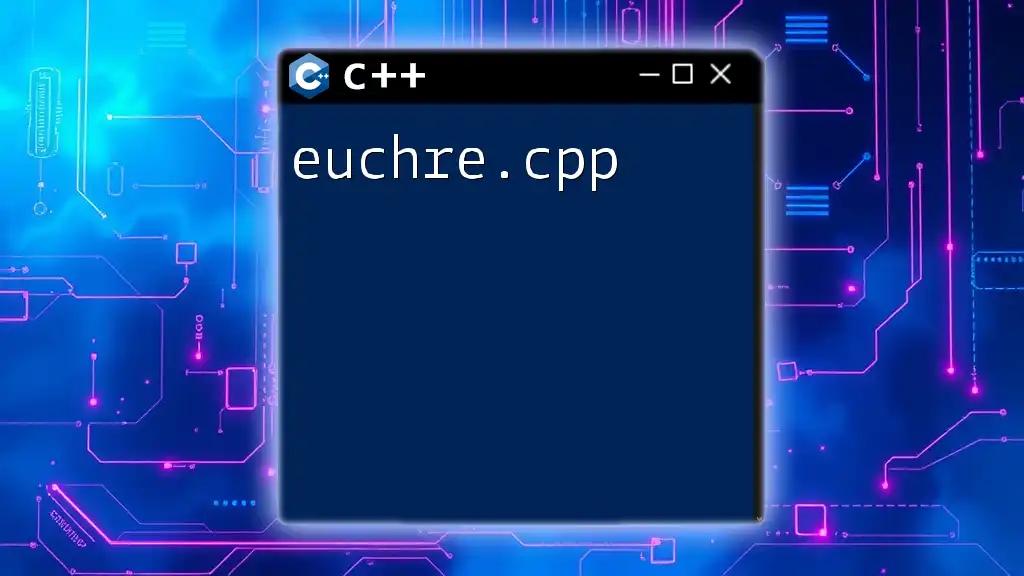
Creating and Using Structures in C++
Declaring a Structure
The declaration of a structure in C++ makes it ready for use. After defining a structure, you can create variables of that structure type.
Accessing Structure Members
Once you have a structure declared, you need to know how to access its members. For instance, if you create an instance of the `Point` structure:
Point p;
You can assign values to its members as follows:
p.x = 10;
p.y = 20;
In this code snippet, we’re using the `.` operator to access the members of `p`. This operator allows us to specify which member of the struct we want to work with.
Initializing a Structure
Structures can be initialized in several ways:
-
Declaration and Initialization
You can initialize a structure at the time of declaration:
Point p = {10, 20};
In this case, `p` is directly initialized with `x` set to 10 and `y` set to 20.
-
Using the Constructor
Another approach is to define a constructor within the structure itself. For example:
struct Rectangle { int length; int breadth; Rectangle(int l, int b) : length(l), breadth(b) {} }; Rectangle rect(10, 5);
Here, we defined a Rectangle structure that has a constructor to initialize the `length` and `breadth` upon instantiation.

Advanced Topics in Structures
Nested Structures
C++ allows structures to contain other structures. This is particularly useful for organizing complex data. For instance:
struct Employee {
int id;
struct Address {
std::string street;
std::string city;
} address;
};
In this example, we have a structure named Employee that contains an `id` and another structure called Address. To access the nested members, you would use the `.` operator twice:
Employee emp;
emp.id = 1;
emp.address.street = "123 Main St";
emp.address.city = "Anytown";
Passing Structures to Functions
Structures can be passed to functions, either by value or by reference. Passing by value means that a copy of the structure is made, while passing by reference allows the function to modify the original structure.
By Value Example:
void printPoint(Point p) {
std::cout << "Point(" << p.x << ", " << p.y << ")" << std::endl;
}
In this function, a copy of `p` is created, so modifications made inside `printPoint` won’t affect the original `p`.
By Reference Example:
void modifyPoint(Point &p) {
p.x += 5;
p.y += 5;
}
Here, the `&` symbol indicates that we are passing `p` by reference, allowing the function to modify the original structure.
Working with Arrays of Structures
You can also create arrays of structures. This is useful when you want to store multiple instances of a struct. Here’s an example:
Point points[3] = {{1, 2}, {3, 4}, {5, 6}};
You can access individual elements and their members just as you would with regular arrays:
std::cout << "Point 1: (" << points[0].x << ", " << points[0].y << ")" << std::endl;
Structure and Memory Alignment
Understanding memory alignment is vital when working with structures. Memory alignment ensures that data is properly organized in memory, which can affect performance. C++ automatically aligns member variables in structures, possibly adding padding between them.
For example:
struct AlignedStruct {
char a; // 1 byte
int b; // 4 bytes
};
Here, depending on the compiler and platform, padding may be added after `a` to align `b` on a 4-byte boundary. This optimization can reduce access times and improve performance.
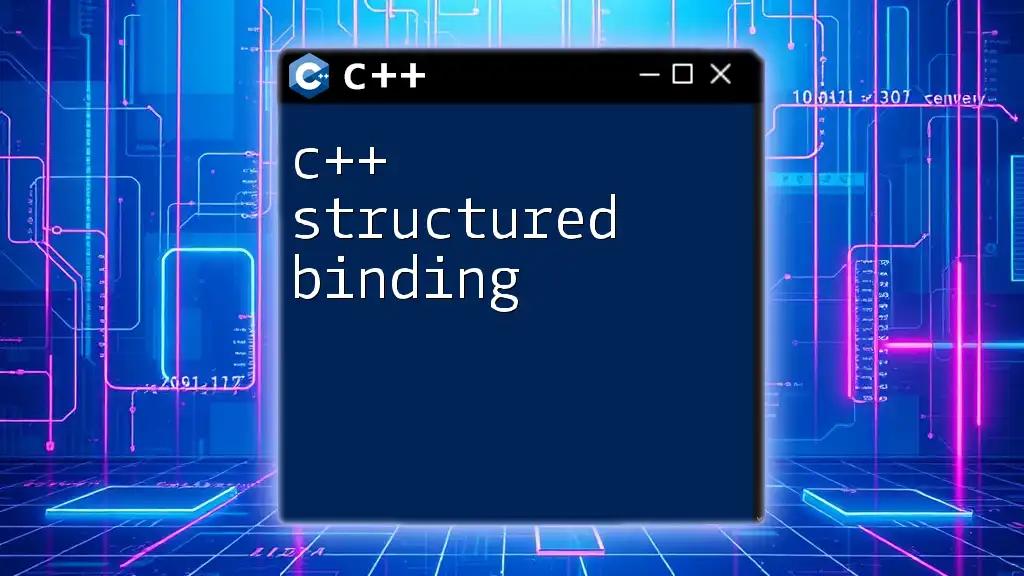
Best Practices for Using Structures in C++
Naming Conventions
When naming structures and their members, clarity and consistency are crucial. Use descriptive names that reflect the purpose of the structure and its members.
When to Use Structures vs. Classes
While both structures and classes are user-defined types in C++, the key difference lies in the default access level: members of structures are `public` by default, while those of classes are `private`.
A good rule of thumb is to use structures for plain data aggregates and classes for more complex behaviors and encapsulation.
Common Mistakes to Avoid
-
Overusing Structures: Structures should ideally be used for grouping related data together. Avoid using them for complex logic, as that is better handled within classes.
-
Not Initializing Members: Always initialize your structure members to avoid undefined behavior. Using constructors is a good practice.
-
Confusing Structures with Classes: Understand the differences in access control and use the appropriate type based on your needs.
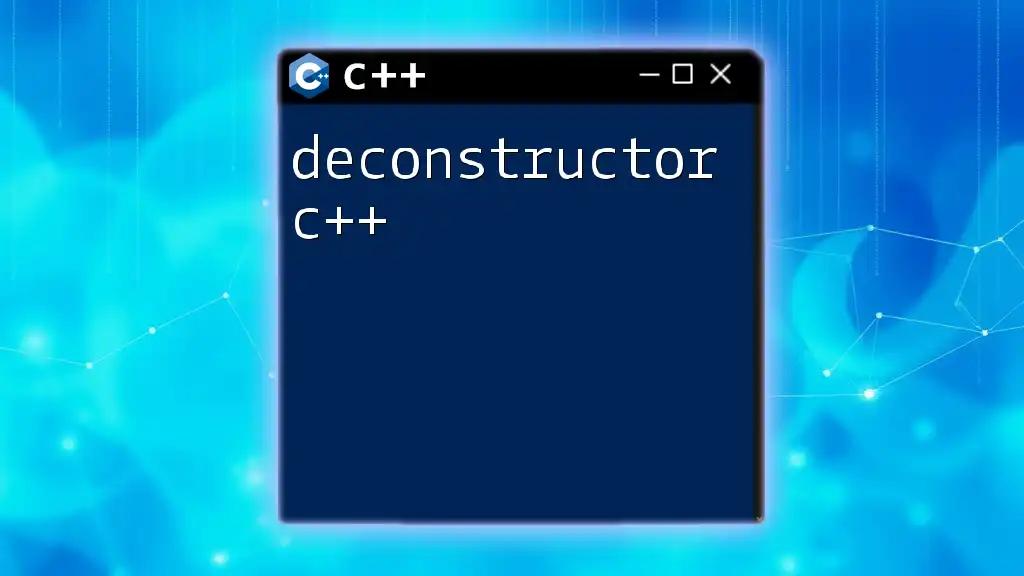
Conclusion
Structures in C++ are an essential feature that allows programmers to create complex data types by grouping multiple variables. By mastering the syntax and usage of structures, you can organize your data more effectively, enhancing the clarity and maintainability of your code. Practice working with structures, and implement them in your C++ projects to gain hands-on experience with this fundamental concept.