A C++ data structures cheat sheet provides a quick reference to the essential data structures in C++, along with their syntax and usage.
// Example of common C++ data structures
#include <iostream>
#include <vector>
#include <map>
#include <string>
int main() {
// Vector
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Map
std::map<std::string, int> age = {{"Alice", 30}, {"Bob", 25}};
// Output
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << "\n";
for (const auto& pair : age) {
std::cout << pair.first << " is " << pair.second << " years old.\n";
}
return 0;
}
Understanding Data Structures
What Are Data Structures?
Data structures are organized formats for storing, managing, and accessing data. In C++, they serve as the backbone for efficient data manipulation, allowing programmers to structure data logically and optimally for various applications. By using the right data structures, the performance of your programs can be dramatically improved.
Why Use Data Structures?
The choice of data structures ensures that your C++ programs run efficiently, both in terms of speed and memory usage. Using the right data structure can lead to:
- Optimized memory usage: Some structures are more memory-efficient than others.
- Faster execution time: Certain operations, like searching, inserting, and deleting, are more efficient with specific data structures.
- Better organization of code: Well-chosen data structures make your code more organized and easier to understand.
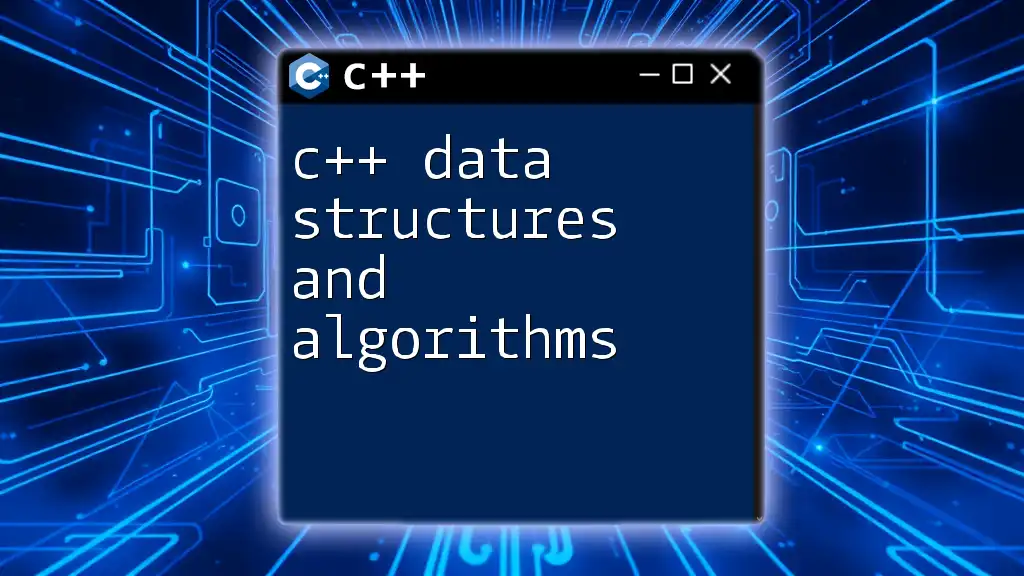
Types of Data Structures
Linear Data Structures
Arrays
An array is a collection of elements of the same type, stored contiguously in memory. They are one of the simplest data structures and are useful for handling lists of data.
Creating and accessing an array in C++ works as follows:
int arr[] = {1, 2, 3, 4, 5};
cout << "First element: " << arr[0] << endl;
Pros: Arrays provide constant time access to their elements and are simple to implement.
Cons: Their fixed size can lead to wasted memory if the array is underused or overflow errors if it’s exceeded.
Linked Lists
A linked list is a collection of nodes, where each node contains data and a pointer to the next node. Unlike arrays, linked lists can grow and shrink dynamically.
A basic singly linked list can be implemented like this:
struct Node {
int data;
Node* next;
};
Advantages: Linked lists allow for efficient insertions and deletions as you don't need to shift elements like in an array.
Stacks
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle, meaning that the most recently added item is the first to be removed.
Here’s how to push and pop items in a stack:
#include <stack>
stack<int> myStack;
myStack.push(10);
myStack.pop();
Stacks are often used in algorithm implementations, like recursive function calls or backtracking algorithms.
Queues
Queues operate on a First-In-First-Out (FIFO) basis. This means that the first element added is the first one to be removed.
Here's a basic implementation for queue operations:
#include <queue>
queue<int> myQueue;
myQueue.push(1);
myQueue.pop();
Queues are commonly used in scenarios where order matters, such as task scheduling or handling requests.
Non-Linear Data Structures
Trees
A tree is a hierarchical data structure with a root node and sub-nodes. Trees can create complex structures that represent hierarchical relationships, such as file systems.
You can declare a binary tree node as follows:
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
Trees can be used for databases, storage systems, and maintaining hierarchical data effectively.
Graphs
Graphs consist of vertices (or nodes) connected by edges. They can be directed (edges have a direction) or undirected (edges do not have a direction).
A simple representation of a graph using an adjacency list can look like this:
#include <vector>
std::vector<std::vector<int>> graph(5);
Graphs are useful for modeling networks, social connections, and paths in navigation systems.
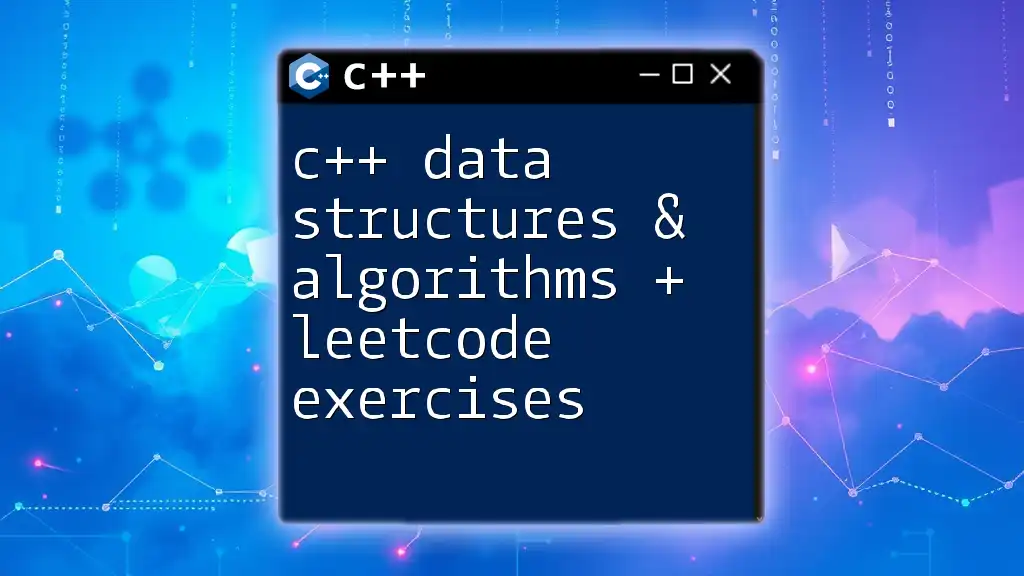
Advanced Data Structures
Hash Tables
Hash tables use a hashing function to map keys to values, enabling fast data retrieval. They are excellent for applications that require constant-time complexity lookups.
Here’s how to use C++ STL's `unordered_map` for a hash table:
#include <unordered_map>
std::unordered_map<int, int> hashTable;
hashTable[10] = 20;
Performance: With a good hash function, lookups in hash tables can be much faster than in other structures.
Sets
Sets are collections that contain unique elements. They are often implemented using hash tables or balanced trees which allow for fast operations.
Using C++ STL `set`:
#include <set>
std::set<int> mySet = {1, 2, 3};
Advantage: Sets automatically handle duplicates, making them ideal for storing unique elements.
Trees (Advanced)
AVL Trees
AVL trees are a type of self-balancing binary search tree. They guarantee O(log n) time complexity for search, insertion, and deletion. By maintaining balance through rotations, they ensure that operations remain efficient even as data is added or removed.
Binary Search Trees
A binary search tree (BST) is a tree structure where each node has at most two children. For each node, values in the left subtree are less and those in the right subtree are greater, facilitating efficient searching.
In-order traversal of a BST can be implemented to retrieve values in sorted order.
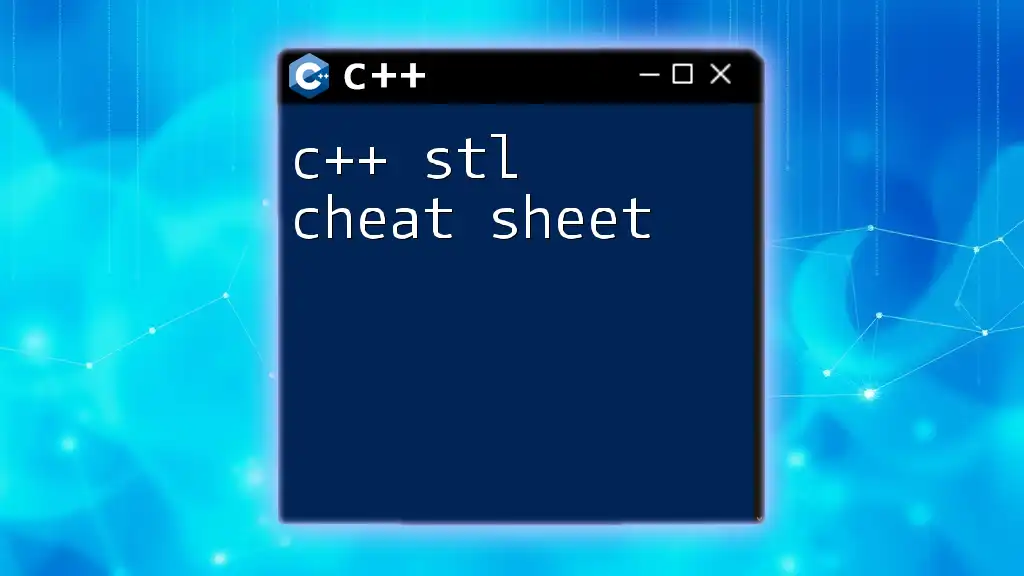
Conclusion
In sum, mastering C++ data structures is crucial for any programmer looking to write efficient, organized, and maintainable code. The C++ data structures cheat sheet outlined above provides a fundamental overview of essential structures, their implementations, and their real-world applications.
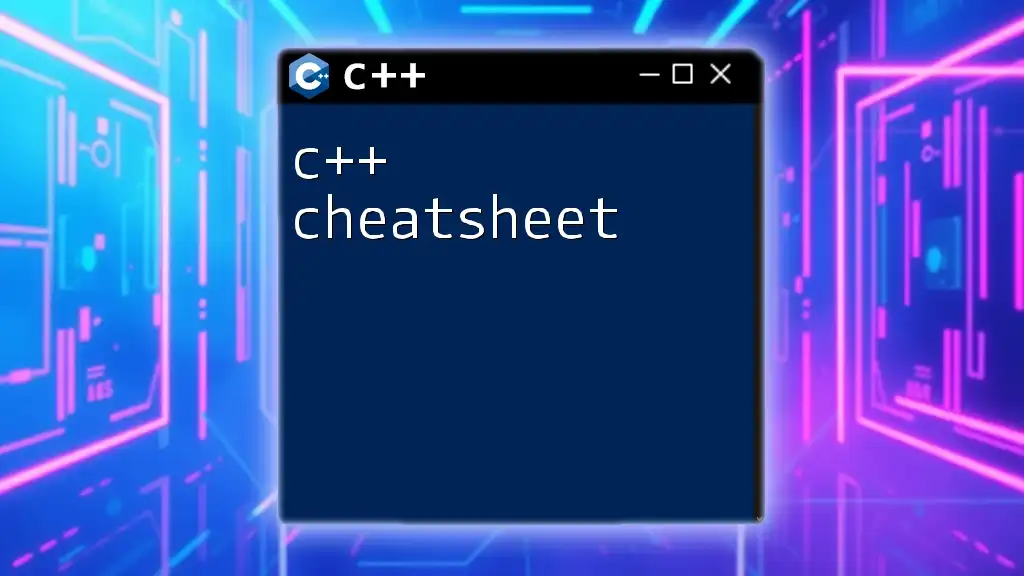
Additional Resources
To deepen your knowledge, consider exploring programming textbooks focused on C++. Online courses and tutorials can enhance your understanding of data structures and algorithms, enabling you to apply these concepts in practical settings. The C++ documentation and community forums are invaluable resources for troubleshooting and learning from experienced programmers.
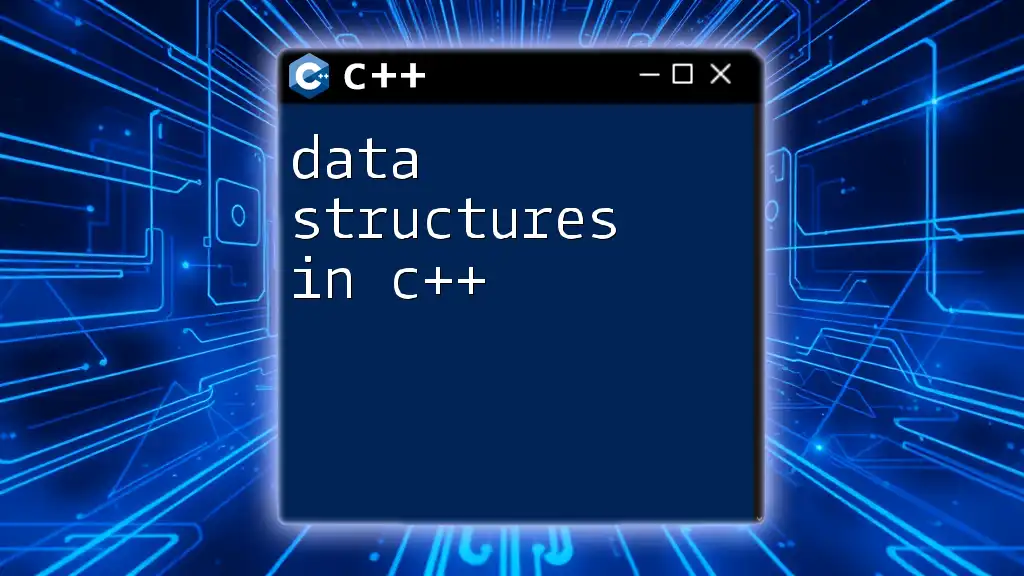
Call to Action
Start applying the knowledge from this cheat sheet by experimenting with different data structures. Practice implementation through small projects, and subscribe for updates to continuously improve your C++ programming skills!