The C++ data stream is a mechanism that allows for the input and output of data through streams, enabling users to read and write from various sources like files or standard input/output.
Here's a simple code snippet demonstrating the use of input and output streams in C++:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt"); // Create and open a text file
outFile << "Hello, World!" << std::endl; // Write to the file
outFile.close(); // Close the file
std::ifstream inFile("example.txt"); // Open the file for reading
std::string line;
while (std::getline(inFile, line)) { // Read the file line by line
std::cout << line << std::endl; // Output each line to the console
}
inFile.close(); // Close the file
return 0;
}
Types of Data Streams
Input Streams
Input streams in C++ are used to read data from various sources, including keyboards, files, and network connections. Understanding input streams is crucial for interacting with users and handling data effectively.
Common scenarios for reading data with input streams include accepting user input and processing information from text files. Input streams utilize the `cin` object from the `<iostream>` library for reading data directly from the keyboard.
Example: Reading Data from Keyboard
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In the example above, we utilize `cin` to read an integer from the user and store it in the variable `number`. The program then outputs the entered value using `cout`.
Output Streams
Output streams serve the purpose of writing data to various destinations such as console displays or files. They provide a way to output messages or results to the user, enhancing user interaction.
Example: Writing Data to Console
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, `cout` is used to display the message "Hello, World!" on the console. By understanding output streams, you can enhance the functionality and usability of your C++ programs.
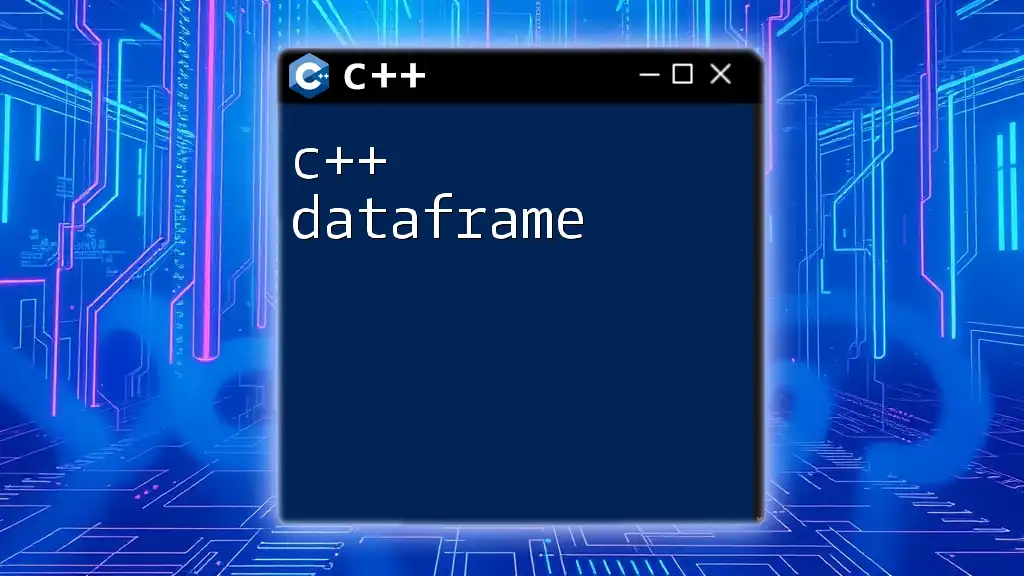
C++ IO Streams Explained
The `<iostream>` Header File
The `<iostream>` header file is fundamental to C++ as it provides the essential tools for input and output operations. It includes two primary objects: `cin` for input and `cout` for output.
Basic Concepts
- `cin`: Used for input operations, it allows the program to retrieve information from the standard input (usually the keyboard).
- `cout`: Responsible for output operations, this object sends data to the standard output (usually the console).
- `cerr`: Used for error messages, it outputs errors to the console.
Example Demonstration: Using `cin`, `cout`, and `cerr`
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cerr << "Your age is: " << age << endl; // Outputting error message
return 0;
}
In the example above, `cout` prompts the user to enter their age, while `cerr` outputs this age prefaced by the "Your age is:" message, highlighting error output usage.
The `<fstream>` Header File
The `<fstream>` library extends C++’s input and output capabilities beyond the console, enabling file input and output. It includes different stream classes for handling file operations, belonging to the same family of functionality as `cin` and `cout`.
Using `ifstream` for Input
The `ifstream` class (input file stream) reads data from files. It is essential for applications that require persistent data storage, like games and databases.
Example: Reading from a File
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ifstream inputFile("data.txt");
if (!inputFile) {
cerr << "Unable to open file data.txt";
return 1; // Exit if file not found
}
string line;
while (getline(inputFile, line)) {
cout << line << endl; // Displaying each line of the file
}
inputFile.close();
return 0;
}
In this example, the program attempts to open a file called `data.txt`. If the file cannot be opened, it outputs an error message to the console. Otherwise, it reads each line and displays it, demonstrating effective file handling using `ifstream`.
The `<sstream>` Header File
The `<sstream>` library provides the ability to create and manipulate string streams, which are useful for parsing string data. This allows for flexibility when dealing with string conversions and formatting, enhancing your program's capabilities.
Example of `stringstream`
#include <sstream>
#include <iostream>
using namespace std;
int main() {
stringstream ss;
ss << "Hello, World!";
string s;
ss >> s;
cout << s << endl; // Outputs: Hello,
return 0;
}
In this example, `stringstream` is utilized to store and manipulate a string. This highlights the power of string streams in parsing and managing text data dynamically.
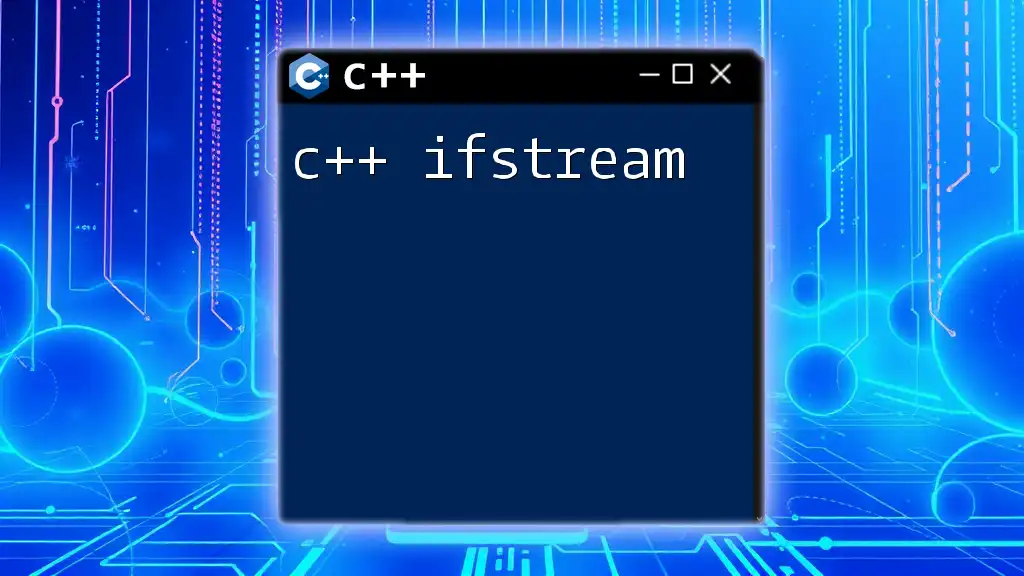
Advanced C++ Stream Concepts
Stream Manipulators
Stream manipulators are special functions in C++ that alter the behavior of I/O operations. They enhance formatting and output handling, allowing developers to create cleaner and more professional-looking outputs.
Common Manipulators Include:
- `std::endl`: Inserts a new line and flushes the output buffer.
- `std::fixed`: Sets the floating-point notation to fixed.
- `std::setprecision`: Controls the precision of floating-point output.
Code Example Using Manipulators
#include <iostream>
#include <iomanip> // for std::setprecision
using namespace std;
int main() {
double pi = 3.14159;
cout << fixed << setprecision(2) << pi << endl; // Output: 3.14
return 0;
}
In this snippet, we format the output of the variable `pi` to display only two decimal places by combining `fixed` with `setprecision`. This enhances the readability and usability of floating-point numbers in your output.
Stream States and Error Handling
Understanding stream states is vital for effective error handling in C++. Streams can represent different states indicating success or failure in operations.
What are Stream States?
- good(): Indicates that the stream is functioning correctly.
- eof(): Signals that the end of the file has been reached.
- fail(): Denotes that an operation has failed due to invalid input or other errors.
Error Handling Example
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (cin.fail()) {
cerr << "Input error: Please enter a valid number." << endl;
}
return 0;
}
In this example, the program checks if the input operation failed using `cin.fail()`. If it did, an error message is displayed, ensuring that users are informed about input issues.
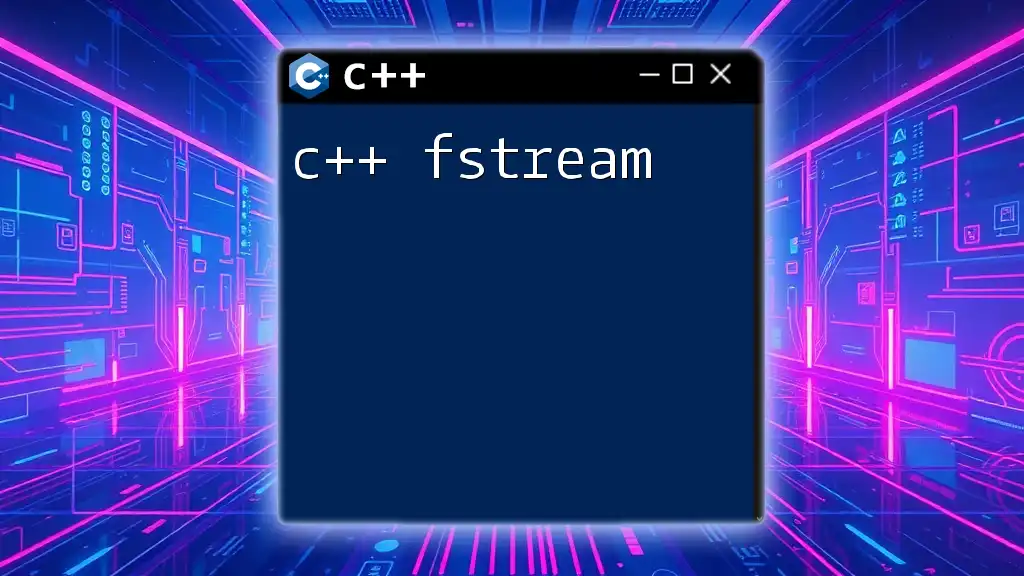
Best Practices for Working with C++ Data Streams
Efficient Use of Streams
To make your programs efficient, it's important to use the appropriate streams for the desired operations. It’s advisable to:
- Minimize the usage of `std::endl` if not necessary, as it flushes the output buffers, which can slow down your program.
- Use buffered output effectively, especially when dealing with large volumes of data.
Resource Management with Streams
Properly managing resources when using streams is crucial for application stability:
- Always close file streams using `close()` to free resources correctly.
- Employ RAII (Resource Acquisition Is Initialization) principles to ensure streams are closed as soon as they go out of scope.
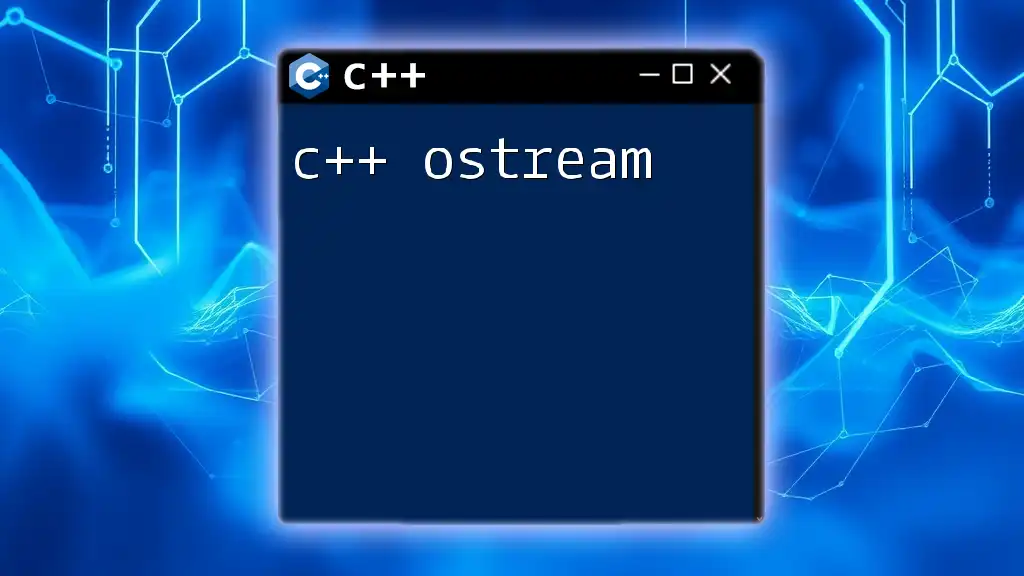
Conclusion
In summary, understanding C++ data streams is vital for effective programming. They enable interaction with users, handle file input and output, and allow for complex data manipulations through string streams. Mastering these components will not only enhance your development skills but also facilitate the creation of efficient and responsive applications.
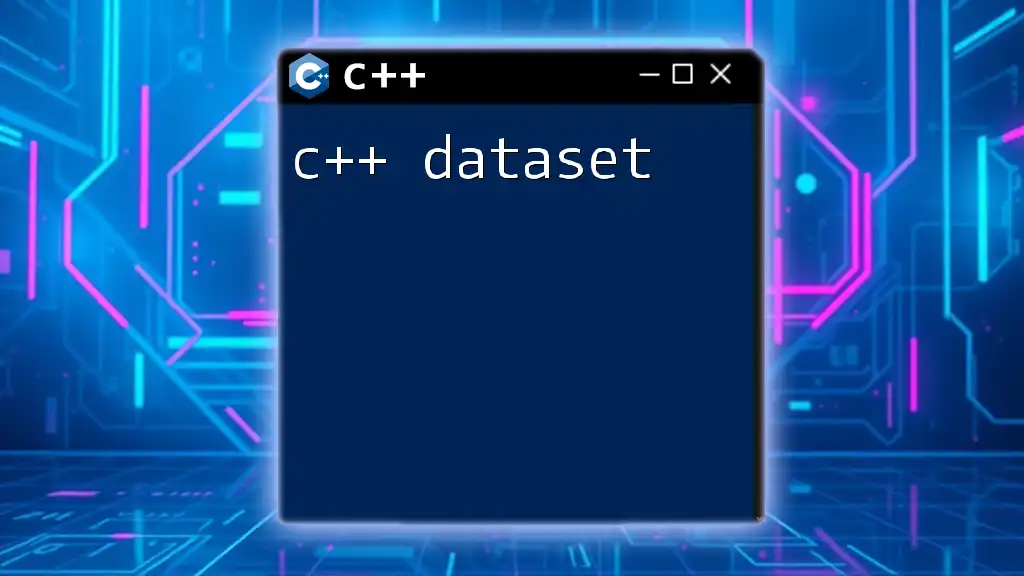
FAQs about C++ Data Streams
-
What are the primary types of data streams in C++? Input and output streams are the primary types, with `cin` for input and `cout` for output.
-
How do I handle errors when using input streams? Utilize stream state functions like `fail()`, `eof()`, and `good()` to check for errors and manage user input effectively.
-
Can I read from more than one file in C++? Yes, by creating multiple `ifstream` objects, you can read from several files within the same program.