C++ fstream is a part of the iostream library used for file handling, allowing you to create, read, and write to files using input/output streams.
Here’s a simple example demonstrating how to use fstream to write text to a file and then read it back:
#include <fstream>
#include <iostream>
#include <string>
int main() {
// Write to a file
std::ofstream outFile("example.txt");
outFile << "Hello, File!" << std::endl;
outFile.close();
// Read from a file
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
Understanding File Streams
In C++, file streams provide a way to interact with files on disk, allowing programs to read from and write to them in an organized manner. They are crucial for applications that require persistent data storage.
File streams are part of the C++ Standard Library and bring several advantages over traditional C-style file handling, notably the principles of Object-Oriented Programming (OOP). Utilizing class-based structures can enhance code readability and maintainability.
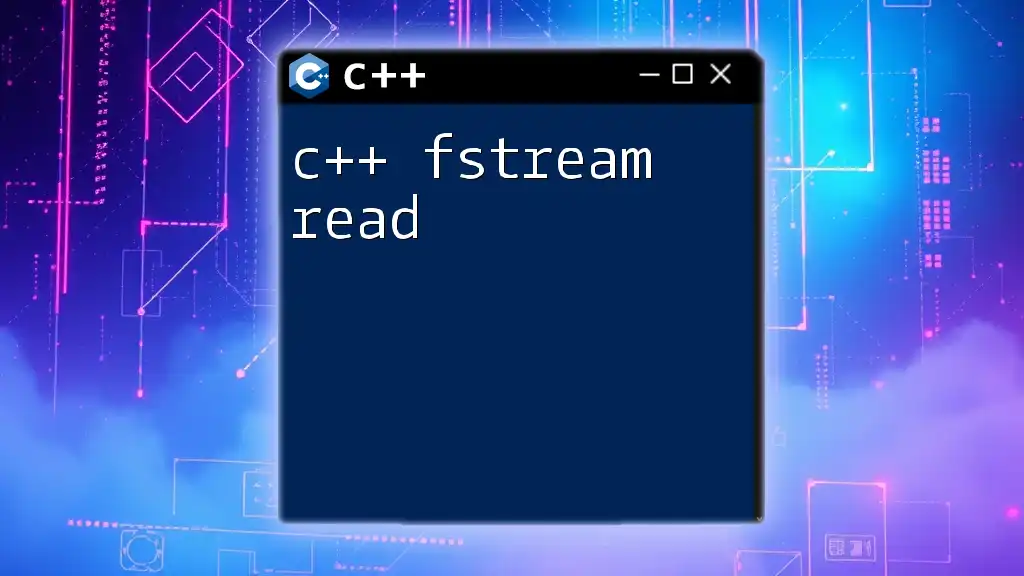
What is fstream?
The `fstream` class is a powerful component of the C++ library that allows both reading from and writing to files. It incorporates three types of file stream classes:
- ifstream: Input file stream for reading data from files.
- ofstream: Output file stream for writing data to files.
- fstream: A file stream that supports both input and output operations.
By using these classes, developers can handle file operations with greater ease, clarity, and safety.
Including the Required Header
To use `fstream`, you must include the `<fstream>` header in your program. This header contains the definitions necessary to work with file streams effectively.
Example missing this header could lead to compilation errors, indicating that file stream data types and functions are unavailable.
#include <fstream>
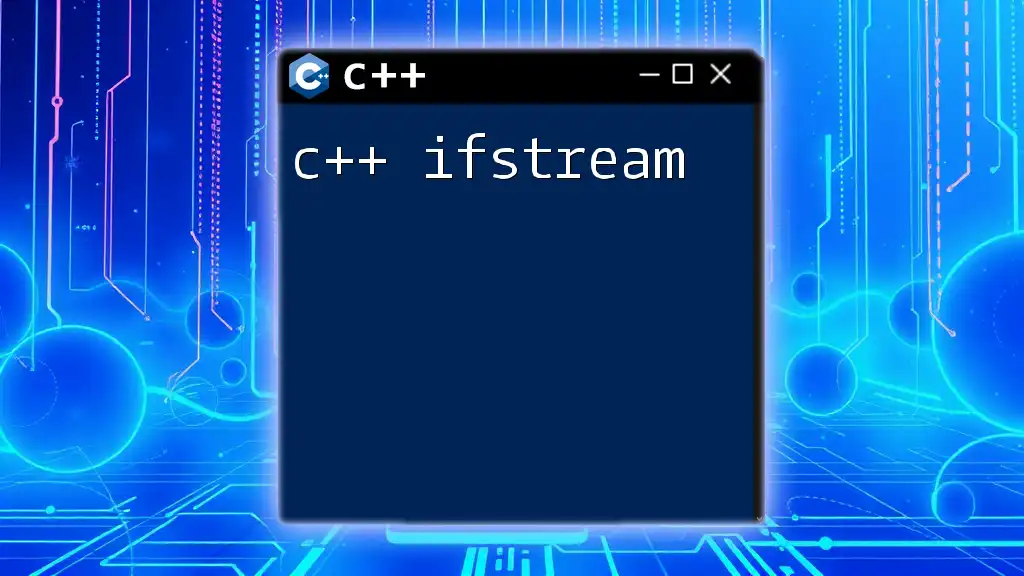
Working with ifstream
Reading from Files with ifstream
The `ifstream` class is designed to handle file inputs. It allows you to open a file and read its contents effectively. Below is a simple code snippet that demonstrates basic file reading using `ifstream`.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << '\n';
}
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this example, the `ifstream` object attempts to open "example.txt". If successful, it reads the file line by line and prints each line to the console.
Utilizing the `getline` function is crucial here, as it enables the reading of an entire line until a newline character.
Checking for Errors
When performing file operations, it is essential to verify whether the file has opened successfully. Using `if (file.is_open())` allows you to check this condition. If the file cannot be opened, you can handle the error gracefully by informing the user.
Best Practices for ifstream
To ensure safe and efficient reading from files, keep these tips in mind:
- Check for file existence: Always verify whether the file exists before attempting to open it.
- Use exception handling: Implement try-catch blocks to manage unexpected errors gracefully.
- Close the file: Always close the file after operations are completed to free resources and prevent data corruption.
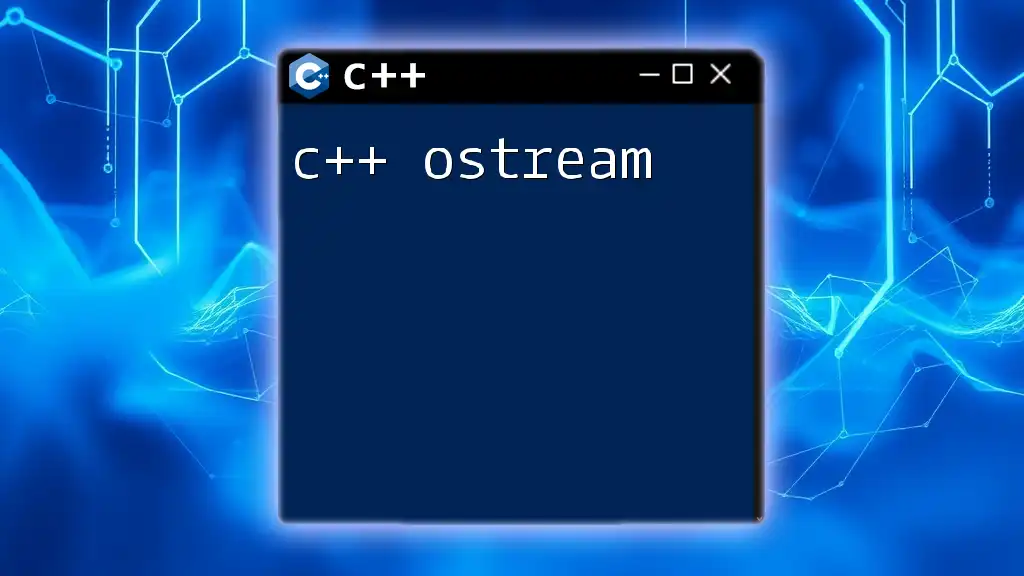
Working with ofstream
Writing to Files with ofstream
Using `ofstream`, you can easily write data to a file. Here’s a simple example of creating a new file and writing to it.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ofstream file("output.txt");
if (file.is_open()) {
file << "Hello, World!" << '\n';
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this snippet, `ofstream` opens "output.txt" for writing. If the file exists, it will be truncated to zero length; if it does not exist, it will be created. The `<<` operator is used to write data, followed by a newline character for formatting.
Appending Data to Files
If you wish to add content to an existing file rather than overwrite it, you can open the file in "append" mode. This is done by using `std::ios::app` when creating the `ofstream`.
Example of opening in append mode:
std::ofstream file("output.txt", std::ios::app);
This approach allows you to append new data without losing the previous content.
Best Practices for ofstream
To maintain a solid structure when writing data to files, follow these best practices:
- Close the file: Always close your file after finishing writing to ensure data is flushed and memory resources are released.
- Handle exceptions: Use try-catch blocks to manage unforeseen errors that may arise while writing to files.
- Format your output: To ensure readability and maintainability, format your output effectively, especially in cases where structured data is needed.
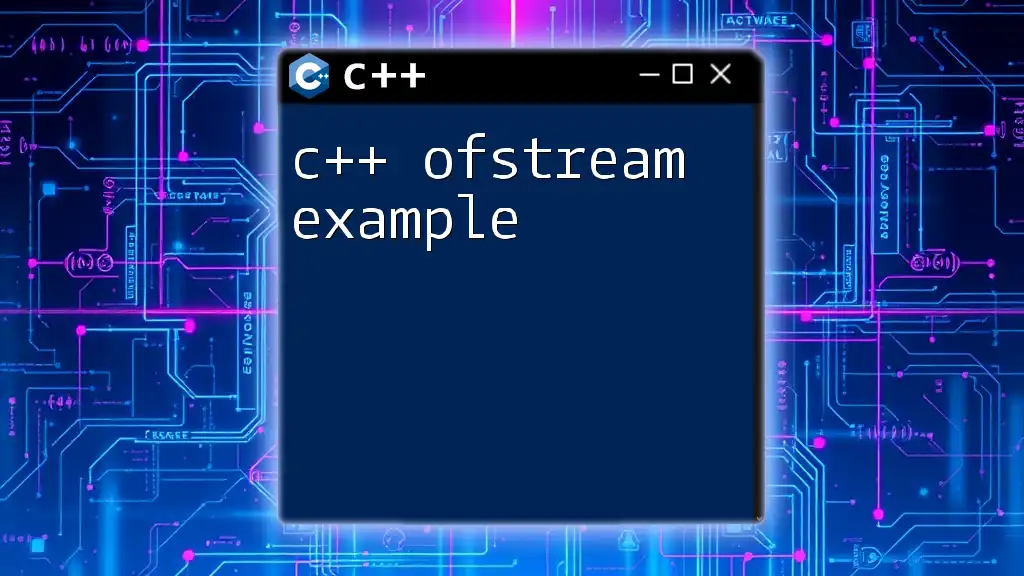
Working with fstream
Using fstream for Combined Operations
The `fstream` class enables reading from and writing to the same file within a single instance. Here is an example that demonstrates combined reading and writing.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::fstream file("example.txt", std::ios::in | std::ios::out | std::ios::app);
if (file.is_open()) {
file << "Appending this text.\n";
file.seekg(0);
std::string line;
while (getline(file, line)) {
std::cout << line << '\n';
}
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this code, the `fstream` object opens "example.txt" for both reading and writing, including the append mode. After writing new data, the `seekg(0)` function is used to move the file pointer back to the beginning of the file to read its contents.
Managing the File Pointer
Managing the file pointer is essential when working with `fstream`. The `seekg`, `seekp`, `tellg`, and `tellp` functions are helpful for repositioning and tracking the file pointer, which can determine where read or write operations will occur.
For instance, to move to the end of the file for writing, you could use:
file.seekg(0, std::ios::end); // Move to the end for writing
This functionality is vital for applications that need precise control over file data.
Closing fstream Safely
It is crucial to close the `fstream` object using `close()` to ensure all data is written properly and resources are released. Failing to close the stream might lead to data corruption or loss, especially in cases of unexpected crashes or program termination.
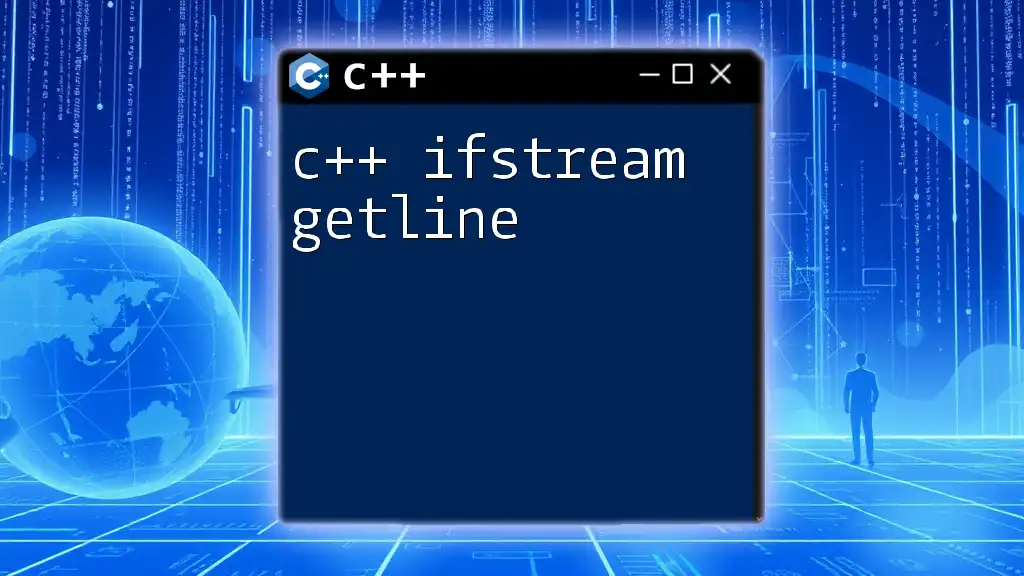
Common Errors and Troubleshooting
Debugging File Handling Issues
When working with `fstream`, some common errors include:
- File not found: Check if the specified file path is correct.
- Permission denied: Ensure your program has the necessary permissions to access the file.
- Incorrect mode: Ensure you're opening the file in the correct mode for the operations you intend to perform.
Handling Exceptions
Utilizing try-catch blocks can help you manage exceptions that arise during file operations:
std::fstream file("example.txt");
try {
if (!file) throw std::runtime_error("Failed to open file");
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
By catching exceptions, you can provide meaningful messages to the user instead of allowing the program to terminate unexpectedly.
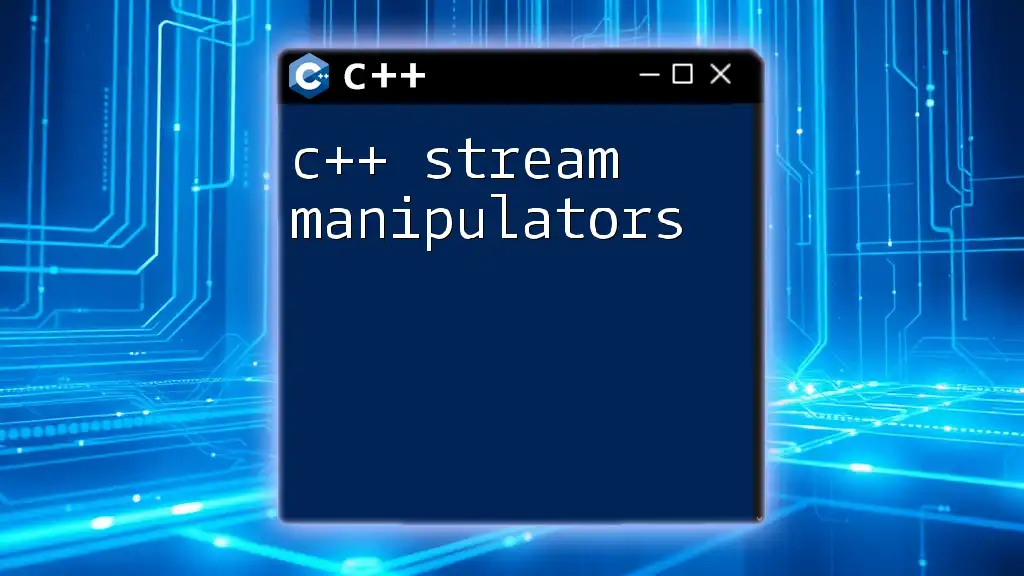
Recap of Key Points
Throughout this article, we have explored the `c++ fstream` class, which provides a versatile mechanism for file input and output. Whether you're using `ifstream`, `ofstream`, or `fstream`, proper file handling can improve your program's efficiency and reliability.
Key takeaways include:
- Utilize `ifstream` for reading, `ofstream` for writing, and `fstream` for both.
- Always check for file existence and handle errors gracefully.
- Maintain best practices by closing files and managing file pointers appropriately.
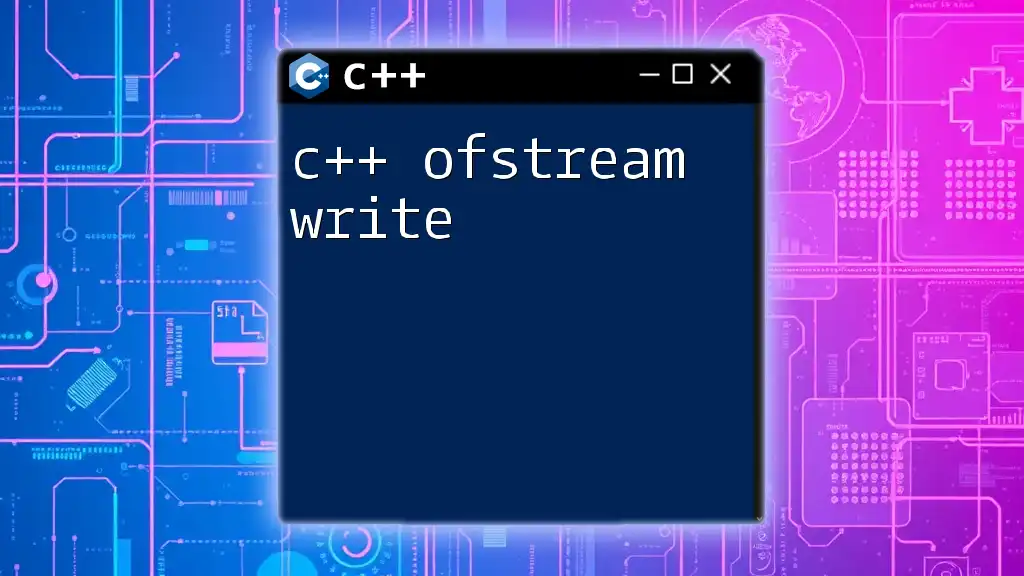
Further Learning Resources
To further enhance your understanding of `c++ fstream`, consider exploring online tutorials, courses, and books focusing on advanced file handling, serialization, and binary file operations. Engaging with diverse resources can deepen your comprehension and application of file streams in your C++ projects.
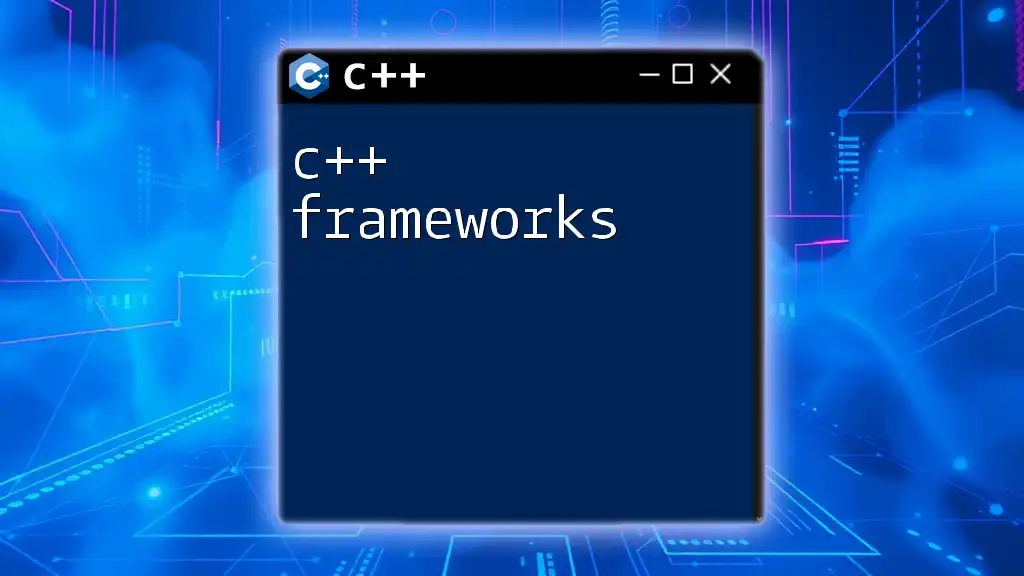
Call to Action
We invite you to share your experiences with C++ file handling, ask questions, or discuss your approach to using `fstream` in your projects. Join our community as we explore more about C++ programming and elevate your coding skills to the next level!